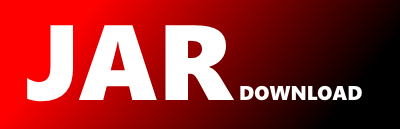
net.sf.saxon.expr.PairIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.expr;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* An iterator over a pair of objects (typically sub-expressions of an expression)
*/
public class PairIterator implements Iterator {
private Object one;
private Object two;
private int pos = 0;
/**
* Create an iterator over two objects
* @param one the first object to be returned
* @param two the second object to be returned
*/
public PairIterator(Object one, Object two) {
this.one = one;
this.two = two;
}
/**
* Returns true if the iteration has more elements. (In other
* words, returns true if next would return an element
* rather than throwing an exception.)
*
* @return true if the iterator has more elements.
*/
public boolean hasNext() {
return pos<2;
}
/**
* Returns the next element in the iteration.
*
* @return the next element in the iteration.
* @exception NoSuchElementException iteration has no more elements.
*/
public Object next() {
switch (pos++) {
case 0: return one;
case 1: return two;
default: throw new NoSuchElementException();
}
}
/**
*
* Removes from the underlying collection the last element returned by the
* iterator (optional operation). This method can be called only once per
* call to next. The behavior of an iterator is unspecified if
* the underlying collection is modified while the iteration is in
* progress in any way other than by calling this method.
*
* @exception UnsupportedOperationException if the remove
* operation is not supported by this Iterator.
* @exception IllegalStateException if the next method has not
* yet been called, or the remove method has already
* been called after the last call to the next
* method.
*/
public void remove() {
throw new UnsupportedOperationException();
}
}
//
// The contents of this file are subject to the Mozilla Public License Version 1.0 (the "License");
// you may not use this file except in compliance with the License. You may obtain a copy of the
// License at http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS" basis,
// WITHOUT WARRANTY OF ANY KIND, either express or implied.
// See the License for the specific language governing rights and limitations under the License.
//
// The Original Code is: all this file.
//
// The Initial Developer of the Original Code is Michael H. Kay.
//
// Contributor(s): Michael Kay
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy