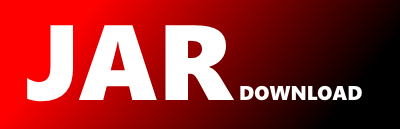
net.sf.saxon.instruct.ElementCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.instruct;
import net.sf.saxon.Controller;
import net.sf.saxon.value.Cardinality;
import net.sf.saxon.evpull.*;
import net.sf.saxon.event.*;
import net.sf.saxon.expr.*;
import net.sf.saxon.om.Item;
import net.sf.saxon.om.NodeInfo;
import net.sf.saxon.om.StandardNames;
import net.sf.saxon.om.Validation;
import net.sf.saxon.pattern.NodeKindTest;
import net.sf.saxon.pattern.NodeTest;
import net.sf.saxon.pull.*;
import net.sf.saxon.trans.XPathException;
import net.sf.saxon.type.ItemType;
import net.sf.saxon.type.Type;
import net.sf.saxon.type.TypeHierarchy;
import net.sf.saxon.type.ValidationException;
/**
* An instruction that creates an element node. There are two subtypes, FixedElement
* for use where the name is known statically, and Element where it is computed
* dynamically. To allow use in both XSLT and XQuery, the class acts both as an
* Instruction and as an Expression.
*/
public abstract class ElementCreator extends ParentNodeConstructor {
/**
* The inheritNamespaces flag indicates that the namespace nodes on the element created by this instruction
* are to be inherited (copied) on the children of this element. That is, if this flag is false, the child
* elements must carry a namespace undeclaration for all the namespaces on the parent, unless they are
* redeclared in some way.
*/
protected boolean inheritNamespaces = true;
/**
* Flag set to true if validation=preserve and no schema type supplied for validation
*/
protected boolean preservingTypes = true;
/**
* Construct an ElementCreator. Exists for the benefit of subclasses.
*/
public ElementCreator() { }
/**
* Get the item type of the value returned by this instruction
* @return the item type
* @param th the type hierarchy cache
*/
public ItemType getItemType(TypeHierarchy th) {
return NodeKindTest.ELEMENT;
}
/**
* Determine whether this elementCreator performs validation or strips type annotations
* @return false if the instruction performs validation of the constructed output or if it strips
* type annotations, otherwise true
*/
public boolean isPreservingTypes() {
return preservingTypes;
}
/**
* Determine whether the inherit namespaces flag is set
* @return true if namespaces constructed on a parent element are to be inherited by its children
*/
public boolean isInheritNamespaces() {
return inheritNamespaces;
}
/**
* Get the static properties of this expression (other than its type). The result is
* bit-signficant. These properties are used for optimizations. In general, if
* property bit is set, it is true, but if it is unset, the value is unknown.
*
* @return a set of flags indicating static properties of this expression
*/
public int computeSpecialProperties() {
return super.computeSpecialProperties() |
StaticProperty.SINGLE_DOCUMENT_NODESET;
}
/**
* Set the validation mode for the new element
*/
public void setValidationMode(int mode) {
super.setValidationMode(mode);
if (mode != Validation.PRESERVE) {
preservingTypes = false;
}
}
/**
* Suppress validation on contained element constructors, on the grounds that the parent element
* is already performing validation. The default implementation does nothing.
*/
public void suppressValidation(int validationMode) {
if (validation == validationMode) {
setValidationMode(Validation.PRESERVE);
}
}
/**
* Check statically whether the content of the element creates attributes or namespaces
* after creating any child nodes
* @param env the static context
* @throws XPathException
*/
protected void checkContentSequence(StaticContext env) throws XPathException {
if (content instanceof Block) {
TypeHierarchy th = env.getConfiguration().getTypeHierarchy();
Expression[] components = ((Block)content).getChildren();
boolean foundChild = false;
boolean foundPossibleChild = false;
int childNodeKinds = (1<{@x}{@y}
if (components[i] instanceof ValueOf &&
((ValueOf)components[i]).select instanceof StringLiteral) {
String value = (((StringLiteral)((ValueOf)components[i]).select).getStringValue());
if (value.length() == 0) {
// continue; // not an error
} else {
foundChild = true;
}
} else {
foundPossibleChild = true;
}
} else if ((possibleNodeKinds & ~childNodeKinds) == 0) {
if (maybeEmpty) {
foundPossibleChild = true;
} else {
foundChild = true;
}
} else if (foundChild && possibleNodeKinds == 1<
© 2015 - 2025 Weber Informatics LLC | Privacy Policy