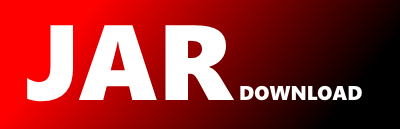
net.sf.saxon.instruct.Instruction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.instruct;
import net.sf.saxon.Configuration;
import net.sf.saxon.Controller;
import net.sf.saxon.event.PipelineConfiguration;
import net.sf.saxon.event.SequenceOutputter;
import net.sf.saxon.expr.*;
import net.sf.saxon.om.EmptyIterator;
import net.sf.saxon.om.Item;
import net.sf.saxon.om.SequenceIterator;
import net.sf.saxon.om.SingletonIterator;
import net.sf.saxon.trace.InstructionInfo;
import net.sf.saxon.trans.XPathException;
import net.sf.saxon.type.ItemType;
import net.sf.saxon.type.Type;
import net.sf.saxon.type.TypeHierarchy;
import javax.xml.transform.SourceLocator;
/**
* Abstract superclass for all instructions in the compiled stylesheet.
* This represents a compiled instruction, and as such, the minimum information is
* retained from the original stylesheet.
* Note: this class implements SourceLocator: that is, it can identify where in the stylesheet
* the source instruction was located.
*/
public abstract class Instruction extends Expression implements SourceLocator, TailCallReturner {
/**
* Constructor
*/
public Instruction() {}
/**
* An implementation of Expression must provide at least one of the methods evaluateItem(), iterate(), or process().
* This method indicates which of these methods is prefered. For instructions this is the process() method.
*/
public int getImplementationMethod() {
return Expression.PROCESS_METHOD;
}
/**
* Get the namecode of the instruction for use in diagnostics
* @return a code identifying the instruction: typically but not always
* the fingerprint of a name in the XSLT namespace
*/
public int getInstructionNameCode() {
return -1;
}
/**
* Get the item type of the items returned by evaluating this instruction
* @return the static item type of the instruction
* @param th the type hierarchy cache
*/
public ItemType getItemType(TypeHierarchy th) {
return Type.ITEM_TYPE;
}
/**
* Get the cardinality of the sequence returned by evaluating this instruction
* @return the static cardinality
*/
public int computeCardinality() {
return StaticProperty.ALLOWS_ZERO_OR_MORE;
}
/**
* ProcessLeavingTail: called to do the real work of this instruction. This method
* must be implemented in each subclass. The results of the instruction are written
* to the current Receiver, which can be obtained via the Controller.
* @param context The dynamic context of the transformation, giving access to the current node,
* the current variables, etc.
* @return null if the instruction has completed execution; or a TailCall indicating
* a function call or template call that is delegated to the caller, to be made after the stack has
* been unwound so as to save stack space.
*/
public abstract TailCall processLeavingTail(XPathContext context) throws XPathException;
/**
* Process the instruction, without returning any tail calls
* @param context The dynamic context, giving access to the current node,
* the current variables, etc.
*/
public void process(XPathContext context) throws XPathException {
try {
TailCall tc = processLeavingTail(context);
while (tc != null) {
tc = tc.processLeavingTail();
}
} catch (XPathException err) {
err.maybeSetLocation(this);
throw err;
}
}
/**
* Get a SourceLocator identifying the location of this instruction
* @return the location of this instruction in the source stylesheet or query
*/
public SourceLocator getSourceLocator() {
return this;
}
/**
* Construct an exception with diagnostic information. Note that this method
* returns the exception, it does not throw it: that is up to the caller.
* @param loc the location of the error
* @param error The exception containing information about the error
* @param context The controller of the transformation
* @return an exception based on the supplied exception, but with location information
* added relating to this instruction
*/
protected static XPathException dynamicError(SourceLocator loc, XPathException error, XPathContext context) {
if (error instanceof TerminationException) {
return error;
}
error.maybeSetLocation(loc);
error.maybeSetContext(context);
return error;
}
/**
* Assemble a ParameterSet. Method used by instructions that have xsl:with-param
* children. This method is used for the non-tunnel parameters.
* @param context the XPath dynamic context
* @param actualParams the set of with-param parameters that specify tunnel="no"
* @return a ParameterSet
*/
protected static ParameterSet assembleParams(XPathContext context,
WithParam[] actualParams)
throws XPathException {
if (actualParams == null || actualParams.length == 0) {
return null;
}
ParameterSet params = new ParameterSet(actualParams.length);
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy