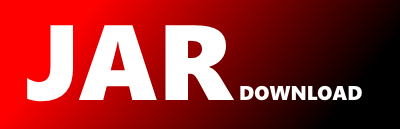
net.sf.saxon.om.Axis Maven / Gradle / Ivy
Go to download
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
Please wait ...