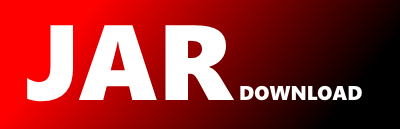
net.sf.saxon.pattern.NodeKindTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.pattern;
import net.sf.saxon.om.NodeInfo;
import net.sf.saxon.tinytree.TinyTree;
import net.sf.saxon.type.*;
/**
* NodeTest is an interface that enables a test of whether a node has a particular
* name and kind. A NodeKindTest matches the node kind only.
*
* @author Michael H. Kay
*/
public class NodeKindTest extends NodeTest {
public static final NodeKindTest DOCUMENT = new NodeKindTest(Type.DOCUMENT);
public static final NodeKindTest ELEMENT = new NodeKindTest(Type.ELEMENT);
public static final NodeKindTest ATTRIBUTE = new NodeKindTest(Type.ATTRIBUTE);
public static final NodeKindTest TEXT = new NodeKindTest(Type.TEXT);
public static final NodeKindTest COMMENT = new NodeKindTest(Type.COMMENT);
public static final NodeKindTest PROCESSING_INSTRUCTION = new NodeKindTest(Type.PROCESSING_INSTRUCTION);
public static final NodeKindTest NAMESPACE = new NodeKindTest(Type.NAMESPACE);
private int kind;
private NodeKindTest(int nodeKind) {
kind = nodeKind;
}
/**
* Make a test for a given kind of node
*/
public static NodeTest makeNodeKindTest(int kind) {
switch (kind) {
case Type.DOCUMENT:
return DOCUMENT;
case Type.ELEMENT:
return ELEMENT;
case Type.ATTRIBUTE:
return ATTRIBUTE;
case Type.COMMENT:
return COMMENT;
case Type.TEXT:
return TEXT;
case Type.PROCESSING_INSTRUCTION:
return PROCESSING_INSTRUCTION;
case Type.NAMESPACE:
return NAMESPACE;
case Type.NODE:
return AnyNodeTest.getInstance();
default:
throw new IllegalArgumentException("Unknown node kind in NodeKindTest");
}
}
/**
* Test whether this node test is satisfied by a given node
* @param nodeKind The type of node to be matched
* @param fingerprint identifies the expanded name of the node to be matched
*/
public boolean matches(int nodeKind, int fingerprint, int annotation) {
return (kind == nodeKind);
}
/**
* Test whether this node test is satisfied by a given node on a TinyTree. The node
* must be a document, element, text, comment, or processing instruction node.
* This method is provided
* so that when navigating a TinyTree a node can be rejected without
* actually instantiating a NodeInfo object.
*
* @param tree the TinyTree containing the node
* @param nodeNr the number of the node within the TinyTree
* @return true if the node matches the NodeTest, otherwise false
*/
public boolean matches(TinyTree tree, int nodeNr) {
return tree.getNodeKind(nodeNr) == kind;
}
/**
* Test whether this node test is satisfied by a given node. This alternative
* method is used in the case of nodes where calculating the fingerprint is expensive,
* for example DOM or JDOM nodes.
* @param node the node to be matched
*/
public boolean matches(NodeInfo node) {
return node.getNodeKind() == kind;
}
/**
* Determine the default priority of this node test when used on its own as a Pattern
*/
public final double getDefaultPriority() {
return -0.5;
}
/**
* Determine the types of nodes to which this pattern applies. Used for optimisation.
* @return the type of node matched by this pattern. e.g. Type.ELEMENT or Type.TEXT
*/
public int getPrimitiveType() {
return kind;
}
/**
* Get a mask indicating which kinds of nodes this NodeTest can match. This is a combination
* of bits: 1<
© 2015 - 2025 Weber Informatics LLC | Privacy Policy