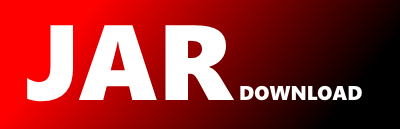
net.sf.saxon.s9api.XPathSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.s9api;
import net.sf.saxon.om.Item;
import net.sf.saxon.om.ValueRepresentation;
import net.sf.saxon.sxpath.XPathDynamicContext;
import net.sf.saxon.sxpath.XPathExpression;
import net.sf.saxon.sxpath.XPathVariable;
import net.sf.saxon.trans.XPathException;
import net.sf.saxon.value.SequenceExtent;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* An XPathSelector represents a compiled and loaded XPath expression ready for execution.
* The XPathSelector holds details of the dynamic evaluation context for the XPath expression.
*/
@SuppressWarnings({"ForeachStatement"})
public class XPathSelector implements Iterable {
private XPathExpression exp;
private XPathDynamicContext dynamicContext;
private List declaredVariables;
// protected constructor
protected XPathSelector(XPathExpression exp,
ArrayList declaredVariables) {
this.exp = exp;
this.declaredVariables = declaredVariables;
dynamicContext = exp.createDynamicContext(null);
}
/**
* Set the context item for evaluating the XPath expression.
* This may be either a node or an atomic value. Most commonly it will be a document node,
* which might be constructed using the {@link DocumentBuilder#build} method.
*
* @param item The context item for evaluating the expression. Must not be null.
*/
public void setContextItem(XdmItem item) throws SaxonApiException {
try {
dynamicContext.setContextItem((Item)item.getUnderlyingValue());
} catch (XPathException e) {
throw new SaxonApiException(e);
}
}
/**
* Get the context item used for evaluating the XPath expression.
* This may be either a node or an atomic value. Most commonly it will be a document node,
* which might be constructed using the Build method of the DocumentBuilder object.
*
* @return The context item for evaluating the expression, or null if no context item
* has been set.
*/
public XdmItem getContextItem() {
return XdmItem.wrapItem(dynamicContext.getContextItem());
}
/**
* Set the value of a variable
*
* @param name The name of the variable. This must match the name of a variable
* that was declared to the XPathCompiler. No error occurs if the expression does not
* actually reference a variable with this name.
* @param value The value to be given to the variable.
* @throws SaxonApiException if the variable has not been declared or if the type of the value
* supplied does not conform to the required type that was specified when the variable was declared
*/
public void setVariable(QName name, XdmValue value) throws SaxonApiException {
XPathVariable var = null;
for (XPathVariable v : declaredVariables) {
if (v.getVariableQName().equals(name.getStructuredQName())) {
var = v;
break;
}
}
if (var == null) {
throw new SaxonApiException(
new XPathException("Variable has not been declared: " + name));
}
try {
dynamicContext.setVariable(var, value.getUnderlyingValue());
} catch (XPathException e) {
throw new SaxonApiException(e);
}
}
/**
* Evaluate the expression, returning the result as an XdmValue
(that is,
* a sequence of nodes and/or atomic values).
*
* Note: Although a singleton result may be represented as an XdmItem
, there is
* no guarantee that this will always be the case. If you know that the expression will return at
* most one node or atomic value, it is best to use the evaluateSingle
method, which
* does guarantee that an XdmItem
(or null) will be returned.
*
* @return An XdmValue
representing the results of the expression.
* @throws SaxonApiException if a dynamic error occurs during the expression evaluation.
*/
public XdmValue evaluate() throws SaxonApiException {
ValueRepresentation value;
try {
value = SequenceExtent.makeSequenceExtent(exp.iterate(dynamicContext));
} catch (XPathException e) {
throw new SaxonApiException(e);
}
return XdmValue.wrap(value);
}
/**
* Evaluate the XPath expression, returning the result as an XdmItem
(that is,
* a single node or atomic value).
*
* @return an XdmItem
representing the result of the expression, or null if the expression
* returns an empty sequence. If the expression returns a sequence of more than one item,
* any items after the first are ignored.
* @throws SaxonApiException if a dynamic error occurs during the expression evaluation.
*/
public XdmItem evaluateSingle() throws SaxonApiException {
try {
Item i = exp.evaluateSingle(dynamicContext);
if (i == null) {
return null;
}
return (XdmItem) XdmValue.wrap(i);
} catch (XPathException e) {
throw new SaxonApiException(e);
}
}
/**
* Evaluate the expression, returning the result as an Iterator
(that is,
* an iterator over a sequence of nodes and/or atomic values).
*
* Because an XPathSelector
is an {@link Iterable}, it is possible to
* iterate over the result using a Java 5 "for each" expression, for example:
*
*
* XPathCompiler compiler = processor.newXPathCompiler();
* XPathSelector seq = compiler.compile("1 to 20").load();
* for (XdmItem item : seq) {
* System.err.println(item);
* }
*
*
* @return An iterator over the sequence that represents the results of the expression.
* Each object in this sequence will be an instance of XdmItem
. Note
* that the expression may be evaluated lazily, which means that a successful response
* from this method does not imply that the expression has executed successfully: failures
* may be reported later while retrieving items from the iterator.
* @throws SaxonApiUncheckedException
* if a dynamic error occurs during XPath evaluation that
* can be detected at this point. It is also possible that an SaxonApiUncheckedException will
* be thrown by the hasNext()
method of the returned iterator.
*/
public Iterator iterator() throws SaxonApiUncheckedException {
try {
return new XdmSequenceIterator(exp.iterate(dynamicContext));
} catch (XPathException e) {
throw new SaxonApiUncheckedException(e);
}
}
}
//
// The contents of this file are subject to the Mozilla Public License Version 1.0 (the "License");
// you may not use this file except in compliance with the License. You may obtain a copy of the
// License at http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS" basis,
// WITHOUT WARRANTY OF ANY KIND, either express or implied.
// See the License for the specific language governing rights and limitations under the License.
//
// The Original Code is: all this file
//
// The Initial Developer of the Original Code is Michael H. Kay.
//
// Contributor(s):
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy