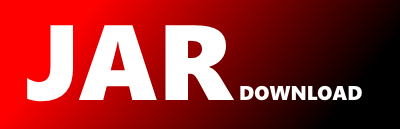
org.opengis.cite.geotiff11.util.TiffDump Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ets-geotiff11 Show documentation
Show all versions of ets-geotiff11 Show documentation
Describe purpose of test suite.
The newest version!
package org.opengis.cite.geotiff11.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* A (rough) wrapper class for the output of TiffDump.exe
*
* @author Dustin Jutras, AGC
*/
public class TiffDump {
/**
*
* main.
*
* @param args an array of {@link java.lang.String} objects
*/
public static void main(String[] args) {
TiffDump td;
String test = "Tile L(ength (0x323) SHORT (3) 231<256 789 87 45>\\r\\n";
try {
td = new TiffDump(new String(
"c:/Users/RDAGCDLJ/Documents/FY20/GeoTIFF/example_tiffs/COG_DEF_OR/LC08_L1TP_016030_20140602_20170305_01_T1_B1.TIF:\r\n"
+ "Magic: 0x4949 Version: 0x2a \r\n"
+ "Directory 0: offset 8 (0x8) next 3034 (0xbda)\r\n"
+ "ImageWidth (256) SHORT (3) 1<7901>\r\n" + "ImageLength (257) SHORT (3) 1<8011>\r\n"
+ "BitsPerSample (258) SHORT (3) 1<16>\r\n" + "Compression (259) SHORT (3) 1<8>\r\n"
+ "Photometric (262) SHORT (3) 1<1>\r\n" + "SamplesPerPixel (277) SHORT (3) 1<1>\r\n"
+ "PlanarConfig (284) SHORT (3) 1<1>\r\n" + "Predictor (317) SHORT (3) 1<1>\r\n"
+ "TileWidth (322) SHORT (3) 1<512>\r\n" + "TileLength (323) SHORT (3) 1<512>\r\n"
+ "SampleFormat (339) SHORT (3) 1<1>\r\n" + "33550 (0x830e) DOUBLE (12) 3<30 30 0>\r\n"
+ "33922 (0x8482) DOUBLE (12) 6<0 0 0 217200 4.9029e+06 0>\r\n"
+ "34735 (0x87af) SHORT (3) 64<1 1 0 14 1024 0 1 1 1025 0 1 2 1026 34737 33 0 2048 0 1 32767 2049 34737 84 33 2054 0 1 9102 2055 34736 1 0 2057 34736 1 1 2059 34736 1 2 2061 34736 1 3 3072 0 1 32767 3073 34737 406 117 3074 0 1 16018 3076 0 1 9001 0 0 0 0>\r\n"
+ "34736 (0x87b0) DOUBLE (12) 4<0.0174533 6.37814e+06 298.257 0>\r\n"
+ "34737 (0x87b1) ASCII (2) 524\r\n"));
System.out.println(td);
System.out.println(td.getDirectory(0));
System.out.println(td.getDirectory(0).getTag("34737"));
System.out.println();
}
catch (Exception e) {
e.printStackTrace();
}
}
/**
* A class representing a directory within a tiff
*
* @author Dustin Jutras, AGC
*
*/
public class Directory {
private int index;
private int offset;
private int next;
private List tags = new ArrayList<>();
public Directory(String directoryLine) {
List info = new ArrayList(Arrays.asList(directoryLine.replace(":", "").split(" ")));
index = Integer.parseInt(info.get(info.indexOf("directory") + 1).trim());
offset = Integer.parseInt(info.get(info.indexOf("offset") + 1).trim());
next = Integer.parseInt(info.get(info.indexOf("next") + 1).trim());
}
public void addTag(String line) {
this.tags.add(new Tag(line));
}
public Tag getTag(String s) {
for (Tag tag : tags) {
if (tag.getName().equals(s.toLowerCase())) {
return tag;
}
}
return null;
}
public Tag getTag(int value) {
for (Tag tag : tags) {
if (tag.getNameValue() == value) {
return tag;
}
}
return null;
}
public List getTags() {
return tags;
}
public int getOffset() {
return offset;
}
public int getNext() {
return next;
}
@Override
public String toString() {
String tagsString = "";
for (Tag tag : tags) {
tagsString += tag.toString() + "\n";
}
return String.format("Directory %d:\nOffset: %d\tNext: %d\nTags:\n%s\n%s\n", index, offset, next,
tagHeaderString(), tagsString);
}
public boolean hasTag(int tag) {
return getTag(tag) != null;
}
}
/**
* A class representing a tag within a tiff
*
* @author Dustin Jutras, AGC
*
*/
public class Tag {
private String line;
private String name, type;
private int nameValue, typeValue, count;
private String valuesAsString;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy