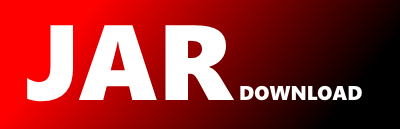
org.opengis.cite.ogcapifeatures10.EtsAssert Maven / Gradle / Ivy
package org.opengis.cite.ogcapifeatures10;
import java.util.List;
import org.locationtech.jts.geom.Envelope;
import org.locationtech.jts.geom.Geometry;
import org.opengis.cite.ogcapifeatures10.conformance.crs.query.crs.CoordinateSystem;
import static org.opengis.cite.ogcapifeatures10.OgcApiFeatures10.DEFAULT_CRS;
import static org.opengis.cite.ogcapifeatures10.OgcApiFeatures10.DEFAULT_CRS_WITH_HEIGHT;
/**
* Provides a set of custom assertion methods.
*
* @author Lyn Goltz
*/
public class EtsAssert {
private static Envelope CRS84_BBOX = new Envelope(-180, 180, -90, 90);
/**
*
* assertTrue.
*
* @param valueToAssert the boolean to assert to be true
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertTrue(boolean valueToAssert, String failureMsg) {
if (!valueToAssert)
throw new AssertionError(failureMsg);
}
/**
*
* assertFalse.
*
* @param valueToAssert the boolean to assert to be false
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertFalse(boolean valueToAssert, String failureMsg) {
if (valueToAssert)
throw new AssertionError(failureMsg);
}
/**
*
* 1. For http-URIs (starting with http:) validate that the string conforms to the syntax specified
* by RFC 7230, section 2.7.1.
* 2. For https-URIs (starting with https:) validate that the string conforms to the syntax specified
* by RFC 7230, section 2.7.2.
* 3. For URNs (starting with urn:) validate that the string conforms to the syntax specified
* by RFC 8141, section 2.
* 4. For OGC URNs (starting with urn:ogc:def:crs:) and OGC http-URIs (starting with http://www.opengis.net/def/crs/)
* validate that the string conforms to the syntax specified by OGC Name Type Specification - definitions - part 1 – basic name.
*
* @param coordinateSystem the coordinate system to assert as valid identifier (see
* above). If null
an AssertionError is thrown.
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertValidCrsIdentifier(CoordinateSystem coordinateSystem, String failureMsg) {
if (coordinateSystem == null)
throw new AssertionError(failureMsg);
if (!coordinateSystem.isValid())
throw new AssertionError(failureMsg);
}
/**
* Assert that one of the default CRS
*
*
* * http://www.opengis.net/def/crs/OGC/1.3/CRS84 (for coordinates without height)
* * http://www.opengis.net/def/crs/OGC/0/CRS84h (for coordinates with height)
*
*
* is in the list of passed crs.
* @param valueToAssert list of CRS which should contain the default crs, never
* null
* @param failureMsg the message to throw in case of a failure, should not be
* null
* @return the default CRS
*/
public static CoordinateSystem assertDefaultCrs(List valueToAssert, String failureMsg) {
if (valueToAssert.contains(OgcApiFeatures10.DEFAULT_CRS_CODE))
return DEFAULT_CRS;
if (valueToAssert.contains(OgcApiFeatures10.DEFAULT_CRS_WITH_HEIGHT_CODE))
return DEFAULT_CRS_WITH_HEIGHT;
throw new AssertionError(failureMsg);
}
/**
* Assert that the first is one of the default CRS
*
*
* * http://www.opengis.net/def/crs/OGC/1.3/CRS84 (for coordinates without height)
* * http://www.opengis.net/def/crs/OGC/0/CRS84h (for coordinates with height)
*
* @param valueToAssert list of CRS which should contain the default crs, never
* null
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertDefaultCrsAtFirst(List valueToAssert, String failureMsg) {
if (!valueToAssert.isEmpty()) {
String firstCrs = valueToAssert.get(0);
if (OgcApiFeatures10.DEFAULT_CRS_CODE.equals(firstCrs))
return;
if (OgcApiFeatures10.DEFAULT_CRS_WITH_HEIGHT_CODE.equals(firstCrs))
return;
}
throw new AssertionError(failureMsg);
}
/**
* Assert that the passed string is one of the the default CRS
*
*
* * http://www.opengis.net/def/crs/OGC/1.3/CRS84 (for coordinates without height)
* * http://www.opengis.net/def/crs/OGC/0/CRS84h (for coordinates with height)
*
* @param crsHeaderValue CRS which should be the default crs, never null
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertDefaultCrs(String crsHeaderValue, String failureMsg) {
if (!crsHeaderValue.matches("<" + OgcApiFeatures10.DEFAULT_CRS_CODE + ">")
&& !crsHeaderValue.matches("<" + OgcApiFeatures10.DEFAULT_CRS_WITH_HEIGHT_CODE + ">")) {
throw new AssertionError(failureMsg);
}
}
/**
*
* assertCrsHeader.
*
* @param crsHeaderValue the value from the header, never null
* @param expectedCrs th expected value, never null
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertCrsHeader(String crsHeaderValue, CoordinateSystem expectedCrs, String failureMsg) {
if (!crsHeaderValue.matches(expectedCrs.getAsHeaderValue())) {
throw new AssertionError(failureMsg);
}
}
/**
* Assert that one of the default CRS
*
*
* * http://www.opengis.net/def/crs/OGC/1.3/CRS84 (for coordinates without height)
* * http://www.opengis.net/def/crs/OGC/0/CRS84h (for coordinates with height)
*
* @param crsHeaderValue the value from the header, never null
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertDefaultCrsHeader(String crsHeaderValue, String failureMsg) {
if (!crsHeaderValue.matches("<" + OgcApiFeatures10.DEFAULT_CRS_CODE + ">")
&& !crsHeaderValue.matches("<" + OgcApiFeatures10.DEFAULT_CRS_WITH_HEIGHT_CODE + ">")) {
throw new AssertionError(failureMsg);
}
}
/**
* Assert that the passed geometry is in the valid area of CRS84.
* @param geometry to check, may be null
* @param failureMsg the message to throw in case of a failure, should not be
* null
*/
public static void assertInCrs84(Geometry geometry, String failureMsg) {
if (geometry == null)
return;
if (!CRS84_BBOX.contains(geometry.getEnvelopeInternal())) {
throw new AssertionError(failureMsg);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy