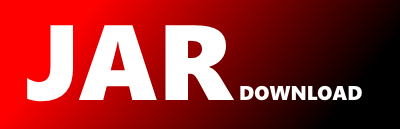
org.opengis.referencing.datum.DatumFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geoapi Show documentation
Show all versions of geoapi Show documentation
The development community in building GIS solutions is sustaining an enormous level
of effort. The GeoAPI project aims to reduce duplication and increase interoperability
by providing neutral, interface-only APIs derived from OGC/ISO Standards.
/*
* GeoAPI - Java interfaces for OGC/ISO standards
* http://www.geoapi.org
*
* Copyright (C) 2004-2011 Open Geospatial Consortium, Inc.
* All Rights Reserved. http://www.opengeospatial.org/ogc/legal
*
* Permission to use, copy, and modify this software and its documentation, with
* or without modification, for any purpose and without fee or royalty is hereby
* granted, provided that you include the following on ALL copies of the software
* and documentation or portions thereof, including modifications, that you make:
*
* 1. The full text of this NOTICE in a location viewable to users of the
* redistributed or derivative work.
* 2. Notice of any changes or modifications to the OGC files, including the
* date changes were made.
*
* THIS SOFTWARE AND DOCUMENTATION IS PROVIDED "AS IS," AND COPYRIGHT HOLDERS MAKE
* NO REPRESENTATIONS OR WARRANTIES, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED
* TO, WARRANTIES OF MERCHANTABILITY OR FITNESS FOR ANY PARTICULAR PURPOSE OR THAT
* THE USE OF THE SOFTWARE OR DOCUMENTATION WILL NOT INFRINGE ANY THIRD PARTY
* PATENTS, COPYRIGHTS, TRADEMARKS OR OTHER RIGHTS.
*
* COPYRIGHT HOLDERS WILL NOT BE LIABLE FOR ANY DIRECT, INDIRECT, SPECIAL OR
* CONSEQUENTIAL DAMAGES ARISING OUT OF ANY USE OF THE SOFTWARE OR DOCUMENTATION.
*
* The name and trademarks of copyright holders may NOT be used in advertising or
* publicity pertaining to the software without specific, written prior permission.
* Title to copyright in this software and any associated documentation will at all
* times remain with copyright holders.
*/
package org.opengis.referencing.datum;
import java.util.Map;
import java.util.Date;
import javax.measure.Unit;
import javax.measure.quantity.Angle;
import javax.measure.quantity.Length;
import org.opengis.referencing.ObjectFactory;
import org.opengis.util.FactoryException;
import org.opengis.annotation.UML;
import static org.opengis.annotation.Specification.*;
/**
* Builds up complex {@linkplain Datum datums} from simpler objects or values.
* {@code DatumFactory} allows applications to make {@linkplain Datum datums}
* that cannot be created by a {@link DatumAuthorityFactory}. This factory is very
* flexible, whereas the authority factory is easier to use.
* So {@link DatumAuthorityFactory} can be used to make "standard" datums, and
* {@code DatumFactory} can be used to make "special" datums.
*
* @author Martin Desruisseaux (IRD)
* @version 3.0.1
* @since 1.0
*
* @see org.opengis.referencing.cs.CSFactory
* @see org.opengis.referencing.crs.CRSFactory
*/
@UML(identifier="CS_CoordinateSystemFactory", specification=OGC_01009)
public interface DatumFactory extends ObjectFactory {
/**
* Creates an engineering datum.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @return The datum for the given properties.
* @throws FactoryException if the object creation failed.
*/
@UML(identifier="createLocalDatum", specification=OGC_01009)
EngineeringDatum createEngineeringDatum(Map properties)
throws FactoryException;
/**
* Creates geodetic datum from ellipsoid and (optionally) Bursa-Wolf parameters.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param ellipsoid Ellipsoid to use in new geodetic datum.
* @param primeMeridian Prime meridian to use in new geodetic datum.
* @return The datum for the given properties.
* @throws FactoryException if the object creation failed.
*/
@UML(identifier="createHorizontalDatum", specification=OGC_01009)
GeodeticDatum createGeodeticDatum(Map properties,
Ellipsoid ellipsoid,
PrimeMeridian primeMeridian) throws FactoryException;
/**
* Creates an image datum.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param pixelInCell Specification of the way the image grid is associated
* with the image data attributes.
* @return The datum for the given properties.
* @throws FactoryException if the object creation failed.
*/
ImageDatum createImageDatum(Map properties,
PixelInCell pixelInCell) throws FactoryException;
/**
* Creates a temporal datum from an enumerated type value.
*
*
*
* Warning: The argument type of this method may change
* in GeoAPI 3.1 release. It may be replaced by a type matching more closely
* either ISO 19108 (Temporal Schema) or ISO 19103.
*
*
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param origin The date and time origin of this temporal datum.
* @return The datum for the given properties.
* @throws FactoryException if the object creation failed.
*/
TemporalDatum createTemporalDatum(Map properties,
Date origin) throws FactoryException;
/**
* Creates a vertical datum from an enumerated type value.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param type The type of this vertical datum (often "geoidal").
* @return The datum for the given properties.
* @throws FactoryException if the object creation failed.
*/
@UML(identifier="createVerticalDatum", specification=OGC_01009)
VerticalDatum createVerticalDatum(Map properties,
VerticalDatumType type) throws FactoryException;
/**
* Creates an ellipsoid from radius values.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param semiMajorAxis Equatorial radius in supplied linear units.
* @param semiMinorAxis Polar radius in supplied linear units.
* @param unit Linear units of ellipsoid axes.
* @return The ellipsoid for the given properties.
* @throws FactoryException if the object creation failed.
*/
@UML(identifier="createEllipsoid", specification=OGC_01009)
Ellipsoid createEllipsoid(Map properties,
double semiMajorAxis,
double semiMinorAxis,
Unit unit) throws FactoryException;
/**
* Creates an ellipsoid from an major radius, and inverse flattening.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param semiMajorAxis Equatorial radius in supplied linear units.
* @param inverseFlattening Eccentricity of ellipsoid.
* @param unit Linear units of major axis.
* @return The ellipsoid for the given properties.
* @throws FactoryException if the object creation failed.
*/
@UML(identifier="createFlattenedSphere", specification=OGC_01009)
Ellipsoid createFlattenedSphere(Map properties,
double semiMajorAxis,
double inverseFlattening,
Unit unit) throws FactoryException;
/**
* Creates a prime meridian, relative to Greenwich.
*
* @param properties Name and other properties to give to the new object.
* Available properties are {@linkplain ObjectFactory listed there}.
* @param longitude Longitude of prime meridian in supplied angular units East of Greenwich.
* @param unit Angular units of longitude.
* @return The prime meridian for the given properties.
* @throws FactoryException if the object creation failed.
*/
@UML(identifier="createPrimeMeridian", specification=OGC_01009)
PrimeMeridian createPrimeMeridian(Map properties,
double longitude,
Unit unit) throws FactoryException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy