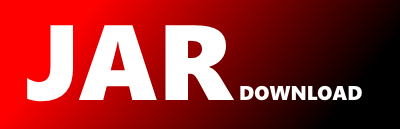
org.openimaj.lsh.functions.DoublePStableFactory Maven / Gradle / Ivy
/*
AUTOMATICALLY GENERATED BY jTemp FROM
/Users/jsh2/Work/openimaj/target/checkout/machine-learning/nearest-neighbour/src/main/jtemp/org/openimaj/lsh/functions/#T#PStableFactory.jtemp
*/
/**
* Copyright (c) 2011, The University of Southampton and the individual contributors.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* * Neither the name of the University of Southampton nor the names of its
* contributors may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
* ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON
* ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.openimaj.lsh.functions;
import org.openimaj.citation.annotation.Reference;
import org.openimaj.citation.annotation.ReferenceType;
import org.openimaj.util.array.SparseDoubleArray;
import org.openimaj.util.array.SparseDoubleArray.Entry;
import cern.jet.random.engine.MersenneTwister;
/**
* Base class for hashing schemes based on P-Stable distributions. The hash
* functions are of the form h(x) = floor((ax + b) / w).
*
* @author Jonathon Hare ([email protected])
*/
@Reference(
type = ReferenceType.Inproceedings,
author = { "Datar, Mayur", "Immorlica, Nicole", "Indyk, Piotr", "Mirrokni, Vahab S." },
title = "Locality-sensitive hashing scheme based on p-stable distributions",
year = "2004",
booktitle = "Proceedings of the twentieth annual symposium on Computational geometry",
pages = { "253", "", "262" },
url = "http://doi.acm.org/10.1145/997817.997857",
publisher = "ACM",
series = "SCG '04"
)
public abstract class DoublePStableFactory extends DoubleHashFunctionFactory {
protected abstract class PStableFunction extends DoubleHashFunction {
protected double[] r;
protected double b;
PStableFunction(MersenneTwister rng) {
super(rng);
}
@Override
public final int computeHashCode(double[] point) {
double val = 0;
for (int i = 0; i < point.length; i++) {
val += point[i] * r[i];
}
val = (val + b) / w;
return (int) Math.floor(val);
}
@Override
public int computeHashCode(SparseDoubleArray array) {
double val = 0;
for (Entry e : array.entries()) {
val += e.value * r[e.index];
}
val = (val + b) / w;
return (int) Math.floor(val);
}
}
double w;
/**
* Construct with the given parameters.
*
* @param ndims
* number of dimensions of the data
* @param rng
* the random number generator
* @param w
* the width parameter
*/
public DoublePStableFactory(int ndims, MersenneTwister rng, double w) {
super(ndims, rng);
this.w = w;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy