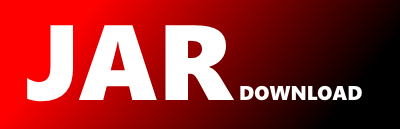
javafx.scene.AccessibleAttribute Maven / Gradle / Ivy
/*
* Copyright (c) 2014, 2017, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package javafx.scene;
import java.time.LocalDate;
import javafx.collections.ObservableList;
import javafx.geometry.Bounds;
import javafx.geometry.Orientation;
import javafx.scene.input.KeyCombination;
import javafx.scene.text.Font;
/**
* This enum describes the attributes that an assistive technology
* such as a screen reader can request from the scene graph.
*
* The {@link AccessibleRole} dictates the set of attributes that
* the screen reader will request for a particular control. For
* example, a slider is expected to return a double that represents
* the current value.
*
* Attributes may have any number of parameters, depending on the particular attribute.
*
* When the value of an attribute is changed by a node, it must notify the assistive technology
* using {@link Node#notifyAccessibleAttributeChanged(AccessibleAttribute)}. This will allow
* the screen reader to inform the user that a value has changed. The most common form of
* notification is focus change.
*
*
* @see Node#queryAccessibleAttribute(AccessibleAttribute, Object...)
* @see Node#notifyAccessibleAttributeChanged(AccessibleAttribute)
* @see AccessibleRole
* @see AccessibleAttribute#ROLE
*
* @since JavaFX 8u40
*/
public enum AccessibleAttribute {
/**
* Returns the accelerator for the node.
*
* - Used by: Menu, MenuItem, RadioMenuItem, and others
* - Needs notify: no
* - Return Type: {@link KeyCombination}
* - Parameters:
*
*/
ACCELERATOR(KeyCombination.class),
/**
* Returns the bounds for the node.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link Bounds}
* - Parameters:
*
*/
BOUNDS(Bounds.class),
/**
* Returns the array of bounding rectangles for the given character range.
*
* - Used by: TextField and TextArea
* - Needs notify: no
* - Return Type: {@link Bounds}[]
* - Parameters:
*
* - {@link Integer} the start offset
* - {@link Integer} the end offset
*
*
*
*/
BOUNDS_FOR_RANGE(Bounds[].class),
/**
* Returns the caret offset for the node.
*
* - Used by: TextField and TextArea
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
CARET_OFFSET(Integer.class),
/**
* Returns the children for the node.
*
* - Used by: Parent
* - Needs notify: no
* - Return Type: {@link javafx.collections.ObservableList}<{@link Node}>
* - Parameters:
*
*/
CHILDREN(ObservableList.class),
/**
* Returns the column at the given index.
*
* - Used by: TableView and TreeTableView
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
* - {@link Integer} the index
*
*
*
*/
COLUMN_AT_INDEX(Node.class),
/**
* Returns the cell at the given row and column indices.
*
* - Used by: TableView and TreeTableView
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
* - {@link Integer} the row index
* - {@link Integer} the column index
*
*
*
*/
CELL_AT_ROW_COLUMN(Node.class),
/**
* Returns the column count for the node.
*
* - Used by: TableView and TreeTableView
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
COLUMN_COUNT(Integer.class),
/**
* Returns the column index for the node.
*
* - Used by: TableCell and TreeTableCell
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
COLUMN_INDEX(Integer.class),
/**
* Returns the contents of the node.
*
* - Used by: ScrollPane
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
CONTENTS(Node.class),
/**
* Returns true if the node is disabled, otherwise false.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
DISABLED(Boolean.class),
/**
* Returns the depth of a row in the disclosure hierarchy.
*
* - Used by: TreeItem and TreeTableRow
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
DISCLOSURE_LEVEL(Integer.class),
/**
* Returns the local date for the node.
*
* - Used by: DatePicker
* - Needs notify: no
* - Return Type: {@link LocalDate}
* - Parameters:
*
*/
DATE(LocalDate.class),
/**
* Returns true if the node is editable, otherwise false.
*
* - Used by: TextField, ComboBox, and others
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
EDITABLE(Boolean.class),
/**
* Returns true if the node is expanded, otherwise false.
*
* - Used by: TreeItem, TitledPane, and others
* - Needs notify: yes
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
EXPANDED(Boolean.class),
/**
* Returns the focus item.
*
* Used for controls such as TabPane, TableView, ListView
* where the assistive technology focus is different from the
* normal focus node. For example, a table control will have focus,
* while a cell inside the table might have the screen reader focus.
*
*
* - Used by: ListView, TabPane, and others
* - Needs notify: yes
* - Return Type: {@link Node}
* - Parameters:
*
*/
FOCUS_ITEM(Node.class),
/**
* Returns the focus node.
* Type: Node
*
* When this attribute is requested from the Scene, the default implementation
* returns {@link Scene#focusOwnerProperty()}.
*
*
* - Used by: Scene
* - Needs notify: yes
* - Return Type: {@link Node}
* - Parameters:
*
*/
FOCUS_NODE(Node.class),
/**
* Returns true if the node is focused, otherwise false.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
FOCUSED(Boolean.class),
/**
* Returns the font for the node.
*
* - Used by: TextField and TextArea
* - Needs notify: no
* - Return Type: {@link Font}
* - Parameters:
*
*/
FONT(Font.class),
/**
* Returns the header for the node.
*
* - Used by: TableView and TreeTableView
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
HEADER(Node.class),
/**
* Returns the help text for the node.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link String}
* - Parameters:
*
*/
HELP(String.class),
/**
* Returns the horizontal scroll bar for the node.
*
* - Used by: ListView, ScrollPane, and others
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
HORIZONTAL_SCROLLBAR(Node.class),
/**
* Returns true of the node is indeterminaite, otherwise false.
*
* - Used by: CheckBox and ProgressIndicator
* - Needs notify: yes
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
INDETERMINATE(Boolean.class),
/**
* Returns the item at the given index.
*
* - Used by: TabPane, ListView, and others
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
* - {@link Integer} the index
*
*
*
*/
ITEM_AT_INDEX(Node.class),
/**
* Returns the item count for the node.
*
* - Used by: TabPane, ListView, and others
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
ITEM_COUNT(Integer.class),
/**
* Returns the index for the node.
*
* - Used by: ListItem, TableRow, and others
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
INDEX(Integer.class),
/**
* Returns the node that is the label for this node.
* When {@link javafx.scene.control.Label#labelForProperty() labelFor} is set,
* the default implementation of {@code LABELED_BY} uses this
* relationship to return the appropriate node to the screen
* reader.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
LABELED_BY(Node.class),
/**
* Returns true if the node is a leaf element, otherwise false.
*
* - Used by: TreeItem and TreeTableRow
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
LEAF(Boolean.class),
/**
* Returns the line end offset of the given line index.
*
* - Used by: TextArea
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
* - {@link Integer} the line index
*
*
*
*/
LINE_END(Integer.class),
/**
* Returns the line index of the given character offset.
*
* - Used by: TextArea
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
* - {@link Integer} the character offset
*
*
*
*/
LINE_FOR_OFFSET(Integer.class),
/**
* Returns the line start offset of the given line index.
*
* - Used by: TextArea
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
* - {@link Integer} the line index
*
*
*
*/
LINE_START(Integer.class),
/**
* Returns the minimum value for the node.
*
* - Used by: Slider, ScrollBar, and others
* - Needs notify: no
* - Return Type: {@link Double}
* - Parameters:
*
*/
MIN_VALUE(Double.class),
/**
* Returns the maximum value for the node.
*
* - Used by: Slider, ScrollBar, and others
* - Needs notify: no
* - Return Type: {@link Double}
* - Parameters:
*
*/
MAX_VALUE(Double.class),
/**
* Returns the mnemonic for the node.
*
* - Used by: Menu, MenuItem, CheckMenuItem, and others
* - Needs notify: no
* - Return Type: {@link String}
* - Parameters:
*
*/
MNEMONIC(String.class),
/**
* Returns true if the node allows for multiple selection, otherwise false.
*
* - Used by: ListView, TableView, and others
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
MULTIPLE_SELECTION(Boolean.class),
/**
* Returns the node at the given location.
*
* - Used by: Scene
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
* - {@link javafx.geometry.Point2D} the point location
*
*
*
*/
NODE_AT_POINT(Node.class),
/**
* Returns the character offset at the given location.
*
* - Used by: TextField and TextArea
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
* - {@link javafx.geometry.Point2D} the point location
*
*
*
*/
OFFSET_AT_POINT(Integer.class),
/**
* Returns the orientation of the node.
*
* - Used by: ScrolBar and Slider
* - Needs notify: no
* - Return Type: {@link javafx.geometry.Orientation}
* - Parameters:
*
*/
ORIENTATION(Orientation.class),
/**
* Return the overflow button for the node.
*
* - Used by: Toolbar
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
OVERFLOW_BUTTON(Node.class),
/**
* Returns the parent for the node.
*
* - Used by: Node
* - Needs notify: yes
* - Return Type: {@link Parent}
* - Parameters:
*
*/
PARENT(Parent.class),
/**
* Returns the parent menu for the node.
*
* - Used by: ContextMenu
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
PARENT_MENU(Node.class),
/**
* Returns the role for the node.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link AccessibleRole}
* - Parameters:
*
*/
ROLE(AccessibleRole.class),
/**
* Returns the role description for the node.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link String}
* - Parameters:
*
*/
ROLE_DESCRIPTION(String.class),
/**
* Returns the row at the given index.
*
* - Used by: TableView, TreeView, and TreeTableView
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
* - {@link Integer} the row index
*
*
*
*/
ROW_AT_INDEX(Node.class),
/**
* Returns the row count for the node.
*
* - Used by: TableView, TreeView, and TreeTableView
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
ROW_COUNT(Integer.class),
/**
* Returns the row index of the node.
*
* - Used by: TableCell, TreeItem, and TreeTableCell
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
ROW_INDEX(Integer.class),
/**
* Returns the scene for the node.
*
* - Used by: Node
* - Needs notify: no
* - Return Type: {@link Scene}
* - Parameters:
*
*/
SCENE(Scene.class),
/**
* Returns true if the node is selected, otherwise false.
*
* - Used by: CheckBox, TreeItem, and others
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
SELECTED(Boolean.class),
/**
* Returns the list of selected items for the node.
*
* - Used by: ListView, TableView, and others
* - Needs notify: no
* - Return Type: {@link javafx.collections.ObservableList}<{@link Node}>
* - Parameters:
*
*/
SELECTED_ITEMS(ObservableList.class),
/**
* Returns the text selection end offset for the node.
*
* - Used by: TextField and TextArea
* - Needs notify: yes
* - Return Type: {@link Integer}
* - Parameters:
*
*/
SELECTION_END(Integer.class),
/**
* Returns the text selection start offset for the node.
*
* - Used by: TextField and TextArea
* - Needs notify: yes
* - Return Type: {@link Integer}
* - Parameters:
*
*/
SELECTION_START(Integer.class),
/**
* Returns the sub menu for the node.
*
* - Used by: Menu
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
SUBMENU(Node.class),
/**
* Returns the text for the node.
* E.g.
*
* - ComboBox returns a string representation of the current selected item.
*
- TextField returns the contents of the text field.
*
*
*
* - Used by: Node
* - Needs notify: yes
* - Return Type: {@link String}
* - Parameters:
*
*/
TEXT(String.class),
/**
* Returns a tree item at the given index, relative to its TREE_ITEM_PARENT.
*
* - Used by: TreeItem and TreeTableRow
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
* - {@link Integer} the index
*
*
*
*/
TREE_ITEM_AT_INDEX(Node.class),
/**
* Returns the tree item count for the node, relative to its TREE_ITEM_PARENT.
*
* - Used by: TreeItem and TreeTableRow
* - Needs notify: no
* - Return Type: {@link Integer}
* - Parameters:
*
*/
TREE_ITEM_COUNT(Integer.class),
/**
* Returns the parent item for the item, or null if the item is the root.
*
* - Used by: TreeItem and TreeTableRow
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
TREE_ITEM_PARENT(Node.class),
/**
* Returns the value for the node.
*
* - Used by: Slider, ScrollBar, Thumb, and others
* - Needs notify: yes
* - Return Type: {@link Double}
* - Parameters:
*
*/
VALUE(Double.class),
/**
* Returns the vertical scroll bar for the node.
*
* - Used by: ListView, ScrollPane, and others
* - Needs notify: no
* - Return Type: {@link Node}
* - Parameters:
*
*/
VERTICAL_SCROLLBAR(Node.class),
/**
* Returns true if node is visible, otherwise false.
*
* - Used by: Node and ContextMenu
* - Needs notify: yes
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
VISIBLE(Boolean.class),
/**
* Returns true if the node has been visited, otherwise false.
*
* - Used by: Hyperlink
* - Needs notify: no
* - Return Type: {@link Boolean}
* - Parameters:
*
*/
VISITED(Boolean.class),
;
private Class> returnClass;
AccessibleAttribute(Class> returnClass) {
this.returnClass = returnClass;
}
public Class> getReturnType() {
return returnClass;
}
}