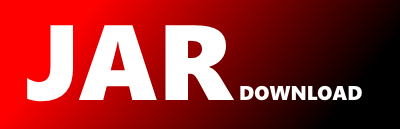
javafx.scene.media.package-info Maven / Gradle / Ivy
/*
* Copyright (c) 2013, 2024, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
/**
* Provides the set of classes for integrating audio and video into Java FX
* Applications. The primary use for this package is media playback. There are
* three principal classes in the media package:
* {@link javafx.scene.media.Media Media},
* {@link javafx.scene.media.MediaPlayer MediaPlayer}, and
* {@link javafx.scene.media.MediaView MediaView}.
*
* Contents
*
* - Supported Media Types
* - Supported Protocols
* - Supported Metadata Tags
* - Playing Media in Java FX
*
*
*
* Supported Media Types
*
* Java FX supports a number of different media types. A media type is considered to
* be the combination of a container format and one or more encodings. In some
* cases the container format might simply be an elementary stream containing the
* encoded data.
*
* Supported Encoding Types
*
*
* An encoding type specifies how sampled audio or video data are stored. Usually
* the encoding type implies a particular compression algorithm. The following
* table indicates the encoding types supported by Java FX Media.
*
*
*
* Media Encoding Table
* Encoding Type Description
* AAC Audio Advanced Audio Coding audio compression
* MP3 Audio
* Raw MPEG-1, 2, and 2.5 audio; layers I, II, and III; all supported
* combinations of sampling frequencies and bit rates. Note: File must contain at least 3 MP3 frames.
*
* PCM Audio Uncompressed, raw audio samples
* H.264/AVC Video H.264/MPEG-4 Part 10 / AVC (Advanced Video Coding)
* video compression
* H.265/HEVC Video H.265/MPEG-H Part 2 / HEVC (High Efficiency Video Coding)
* video compression
*
*
* Supported Container Types
*
*
* A container type specifies the file format used to store the encoded audio,
* video, and other media data. Each container type is associated with one or more
* MIME types, file extensions, and file signatures (the initial bytes in the file).
* The following table indicates the combination of container and encoding types
* supported by Java FX Media.
*
*
*
* Media Container / Encoding Types Table
* Container Description Video Encoding
* Audio Encoding MIME Type File Extension
* AIFF Audio Interchange File Format N/A
* PCM audio/x-aiff .aif, .aiff
* HLS (*) MP2T HTTP Live Streaming (audiovisual) H.264/AVC
* AAC application/vnd.apple.mpegurl, audio/mpegurl .m3u8
* HLS (*) MP3 HTTP Live Streaming (audio-only) N/A
* MP3 application/vnd.apple.mpegurl, audio/mpegurl .m3u8
* HLS (*) fMP4 HTTP Live Streaming (audiovisual) H.264/AVC
* AAC application/vnd.apple.mpegurl, audio/mpegurl .m3u8
* HLS (*) fMP4 HTTP Live Streaming (audiovisual) H.265/HEVC
* AAC application/vnd.apple.mpegurl, audio/mpegurl .m3u8
* HLS (*) fMP4 HTTP Live Streaming (audio-only) N/A
* AAC application/vnd.apple.mpegurl, audio/mpegurl .m3u8
* HLS (*) AAC HTTP Live Streaming (audio-only) N/A
* AAC application/vnd.apple.mpegurl, audio/mpegurl .m3u8
* MP3 MPEG-1, 2, 2.5 raw audio stream possibly with ID3 metadata v2.3 or v2.4
* N/A MP3 audio/mpeg .mp3
* MP4 MPEG-4 Part 14 H.264/AVC
* AAC video/mp4, audio/x-m4a, video/x-m4v .mp4, .m4a, .m4v
* MP4 MPEG-4 Part 14 H.265/HEVC
* AAC video/mp4, audio/x-m4a, video/x-m4v .mp4, .m4a, .m4v
* WAV Waveform Audio Format N/A
* PCM audio/x-wav .wav
*
*
*
(*) HLS is a protocol rather than a container type but is included here to
* aggregate similar attributes.
*
*
* Supported Protocols
*
*
* Supported Protocols Table
* Protocol Description Reference
*
* FILE
* Protocol for URI representation of local files
* java.net.URI
*
*
* HTTP
* Hypertext transfer protocol for representation of remote files
* java.net.URI
*
*
* HTTPS
* Hypertext transfer protocol secure for representation of remote files
* java.net.URI
*
*
* JAR
* Representation of media entries in files accessible via the FILE, HTTP or HTTPS protocols
* java.net.JarURLConnection
*
*
* HTTP Live Streaming (HLS)
* Playlist-based media streaming via HTTP or HTTPS
* HTTP Live Streaming (RFC 8216)
*
*
*
* MPEG-4 Playback via HTTP
*
* It is recommended that MPEG-4 media to be played over HTTP or HTTPS be formatted such that the
* headers required to decode the stream appear at the beginning of the file. Otherwise,
* playback might stall until the entire file is downloaded.
*
* HTTP Live Streaming (HLS)
*
* HLS playback handles sources with these characteristics:
*
*
* - On-demand and live playlists.
* - Elementary MP3 and AAC audio streams (audio/mpegurl).
* - Multiplexed MP2T streams (application/vnd.apple.mpegurl) with one AAC audio and one H.264/AVC video track.
* - Multiplexed fMP4 streams (application/vnd.apple.mpegurl) with one AAC audio and one H.264/AVC or H.265/HEVC video track.
* - Playlists with integer or float duration.
* - Additional audio renditions via #EXT-X-MEDIA tag:
*
* - MP2T streams with one H.264/AVC video track and elementary AAC audio stream via #EXT-X-MEDIA tag.
* - fMP4 streams with one H.264/AVC or H.265/HEVC video track and elementary AAC audio stream via #EXT-X-MEDIA tag.
* - fMP4 streams with one H.264/AVC or H.265/HEVC video track and fMP4 streams with one AAC audio track via #EXT-X-MEDIA tag.
*
*
*
*
* Sources which do not conform to this basic profile are not guaranteed to be handled.
* The playlist contains information about the streams comprising the source and is
* downloaded at the start of playback. Switching between alternate streams, bit rates,
* and video resolutions is handled automatically as a function of network conditions.
*
*
*
* Supported Metadata Tags
*
* A media container may also include certain metadata which describe the media in
* the file. The Java FX Media API makes the metadata available via the
* {@link javafx.scene.media.Media#getMetadata()} method. The keys in this mapping
* are referred to as tags with the tags supported by Java FX Media listed in
* the following table. Note that which tags are available for a given media source
* depend on the metadata actually stored in that source, i.e., not all tags are
* guaranteed to be available.
*
*
* "Metadata Keys and Tags Table
* Container Tag (type String) Type Description
* MP3 raw metadata Map<String,ByteBuffer> The raw metadata according to the appropriate media specification. The key "ID3" maps to MP3 ID3v2 metadata.
* MP3 album artist java.lang.String The artist for the overall album, possibly "Various Artists" for compilations.
* MP3 album java.lang.String The name of the album.
* MP3 artist java.lang.String The artist of the track.
* MP3 comment-N java.lang.String A comment where N is a 0-relative index. Comment format: ContentDescription[lng]=Comment
* MP3 composer java.lang.String The composer of the track.
* MP3 year java.lang.Integer The year the track was recorded.
* MP3 disc count java.lang.Integer The number of discs in the album.
* MP3 disc number java.lang.Integer The 1-relative index of the disc on which this track appears.
* MP3 duration javafx.util.Duration The duration of the track.
* MP3 genre java.lang.String The genre of the track, for example, "Classical," "Darkwave," or "Jazz."
* MP3 image javafx.scene.image.Image The album cover.
* MP3 title java.lang.String The name of the track.
* MP3 track count java.lang.Integer The number of tracks on the album.
* MP3 track number java.lang.Integer The 1-relative index of this track on the disc.
*
*
*
* Playing Media in Java FX
* Basic Playback
*
* The basic steps required to play media in Java FX are:
*
*
* - Create a {@link javafx.scene.media.Media} object for the desired media source.
* - Create a {@link javafx.scene.media.MediaPlayer} object from the
Media
object.
* - Create a {@link javafx.scene.media.MediaView} object.
* - Add the
MediaPlayer
to the MediaView
.
* - Add the
MediaView
to the scene graph.
* - Invoke {@link javafx.scene.media.MediaPlayer#play()}.
*
* The foregoing steps are illustrated by the sample code in the MediaView
* class documentation. Some things which should be noted are:
*
* - One
Media
object may be shared among multiple MediaPlayer
s.
* - One
MediaPlayer
may be shared amoung multiple MediaView
s.
* - Media may be played directly by a
MediaPlayer
* without creating a MediaView
although a view is required for display.
* - Instead of
MediaPlayer.play()
,
* {@link javafx.scene.media.MediaPlayer#setAutoPlay MediaPlayer.setAutoPlay(true)}
* may be used to request that playing start as soon as possible.
* MediaPlayer
has several operational states defined by
* {@link javafx.scene.media.MediaPlayer.Status}.
* - Audio-only media may instead be played using {@link javafx.scene.media.AudioClip}
* (recommended for low latency playback of short clips).
*
* Error Handling
*
* Errors using Java FX Media may be either synchronous or asynchronous. In general
* synchronous errors will manifest themselves as a Java Exception
and
* asynchronous errors will cause a Java FX property to be set. In the latter case
* either the error
property may be observed directly, an
* onError
callback registered, or possibly both.
*
* The main sources of synchronous errors are
* {@link javafx.scene.media.Media#Media Media()} and
* {@link javafx.scene.media.MediaPlayer#MediaPlayer MediaPlayer()}.
* The asynchronous error properties are
* {@link javafx.scene.media.Media#errorProperty Media.error} and
* {@link javafx.scene.media.MediaPlayer#errorProperty MediaPlayer.error}, and the
* asynchronous error callbacks
* {@link javafx.scene.media.Media#onErrorProperty Media.onError},
* {@link javafx.scene.media.MediaPlayer#onErrorProperty MediaPlayer.onError}, and
* {@link javafx.scene.media.MediaView#onErrorProperty MediaView.onError}.
*
*
* Some errors might be duplicated. For example, a MediaPlayer
will
* propagate an error that it encounters to its associated Media
, and
* a MediaPlayer
to all its associated MediaView
s. As a
* consequence, it is possible to receive multiple notifications of the occurrence
* of a given error, depending on which properties are monitored.
*
*
* The following code snippet illustrates error handling with media:
* {@code
* String source;
* Media media;
* MediaPlayer mediaPlayer;
* MediaView mediaView;
* try {
* media = new Media(source);
* if (media.getError() == null) {
* media.setOnError(new Runnable() {
* public void run() {
* // Handle asynchronous error in Media object.
* }
* });
* try {
* mediaPlayer = new MediaPlayer(media);
* if (mediaPlayer.getError() == null) {
* mediaPlayer.setOnError(new Runnable() {
* public void run() {
* // Handle asynchronous error in MediaPlayer object.
* }
* });
* mediaView = new MediaView(mediaPlayer);
* mediaView.setOnError(new EventHandler() {
* public void handle(MediaErrorEvent t) {
* // Handle asynchronous error in MediaView.
* }
* });
* } else {
* // Handle synchronous error creating MediaPlayer.
* }
* } catch (Exception mediaPlayerException) {
* // Handle exception in MediaPlayer constructor.
* }
* } else {
* // Handle synchronous error creating Media.
* }
* } catch (Exception mediaException) {
* // Handle exception in Media constructor.
* }
* }
*/
package javafx.scene.media;