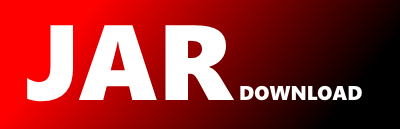
org.openjsse.sun.security.ssl.OpenJSSE Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openjsse Show documentation
Show all versions of openjsse Show documentation
OpenJSSE delivers a TLS 1.3 JSSE provider for Java SE 8
The newest version!
/*
* Copyright 2019 Azul Systems, Inc.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package org.openjsse.sun.security.ssl;
import java.security.*;
import sun.security.action.GetPropertyAction;
import sun.security.x509.AlgorithmId;
import sun.security.util.ObjectIdentifier;
import java.lang.reflect.Field;
import java.util.Map;
/**
* The JSSE provider.
*
* The RSA implementation has been removed from JSSE, but we still need to
* register the same algorithms for compatibility. We just point to the RSA
* implementation in the SunRsaSign provider. This works because all classes
* are in the bootclasspath and therefore loaded by the same classloader.
*
* OpenJSSE now supports an experimental FIPS compliant mode when used with an
* appropriate FIPS certified crypto provider. In FIPS mode, we:
* . allow only TLS 1.0 or later
* . allow only FIPS approved ciphersuites
* . perform all crypto in the FIPS crypto provider
*
* It is currently not possible to use both FIPS compliant OpenJSSE and
* standard JSSE at the same time because of the various static data structures
* we use.
*
* However, we do want to allow FIPS mode to be enabled at runtime and without
* editing the java.security file. That means we need to allow
* Security.removeProvider("OpenJSSE") to work, which creates an instance of
* this class in non-FIPS mode. That is why we delay the selection of the mode
* as long as possible. This is until we open an SSL/TLS connection and the
* data structures need to be initialized or until OpenJSSE is initialized in
* FIPS mode.
*
*/
public abstract class OpenJSSE extends Provider {
public static final double PROVIDER_VER;
private static final long serialVersionUID = 3231825739635378733L;
private static String info;
private static String fipsInfo =
"JDK JSSE provider (FIPS mode, crypto provider ";
// tri-valued flag:
// null := no final decision made
// false := data structures initialized in non-FIPS mode
// true := data structures initialized in FIPS mode
private static Boolean fips;
// the FIPS certificate crypto provider that we use to perform all crypto
// operations. null in non-FIPS mode
static java.security.Provider cryptoProvider;
static {
PROVIDER_VER = Double.parseDouble(System.getProperty("java.specification.version"));
info = "JDK JSSE provider" +
"(PKCS12, SunX509/PKIX key/trust factories, " +
"SSLv3/TLSv1/TLSv1.1/TLSv1.2/TLSv1.3)";
}
protected static synchronized boolean isFIPS() {
if (fips == null) {
fips = false;
}
return fips;
}
// ensure we can use FIPS mode using the specified crypto provider.
// enable FIPS mode if not already enabled.
private static synchronized void ensureFIPS(java.security.Provider p) {
if (fips == null) {
fips = true;
cryptoProvider = p;
} else {
if (fips == false) {
throw new ProviderException
("OpenJSSE already initialized in non-FIPS mode");
}
if (cryptoProvider != p) {
throw new ProviderException
("OpenJSSE already initialized with FIPS crypto provider "
+ cryptoProvider);
}
}
}
// standard constructor
@SuppressWarnings( "deprecation" )
protected OpenJSSE() {
super("OpenJSSE", PROVIDER_VER, info);
subclassCheck();
if (Boolean.TRUE.equals(fips)) {
throw new ProviderException
("OpenJSSE is already initialized in FIPS mode");
}
registerAlgorithms(false);
}
// preferred constructor to enable FIPS mode at runtime
protected OpenJSSE(java.security.Provider cryptoProvider){
this(checkNull(cryptoProvider), cryptoProvider.getName());
}
// constructor to enable FIPS mode from java.security file
protected OpenJSSE(String cryptoProvider){
this(null, checkNull(cryptoProvider));
}
private static T checkNull(T t) {
if (t == null) {
throw new ProviderException("cryptoProvider must not be null");
}
return t;
}
@SuppressWarnings( "deprecation" )
private OpenJSSE(java.security.Provider cryptoProvider,
String providerName) {
super("OpenJSSE", PROVIDER_VER, fipsInfo + providerName + ")");
subclassCheck();
if (cryptoProvider == null) {
// Calling Security.getProvider() will cause other providers to be
// loaded. That is not good but unavoidable here.
cryptoProvider = Security.getProvider(providerName);
if (cryptoProvider == null) {
throw new ProviderException
("Crypto provider not installed: " + providerName);
}
}
ensureFIPS(cryptoProvider);
registerAlgorithms(true);
}
@SuppressWarnings("unchecked")
private void registerAlgorithms(final boolean isfips) {
AccessController.doPrivileged(new PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy