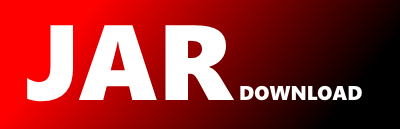
org.openl.rules.maven.GenerateMojo Maven / Gradle / Ivy
package org.openl.rules.maven;
/*
* Copyright 2001-2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.io.File;
import java.util.List;
import org.apache.commons.io.FilenameUtils;
import org.apache.maven.model.Resource;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.openl.OpenL;
import org.openl.conf.ant.JavaAntTask;
import org.openl.conf.ant.JavaInterfaceAntTask;
/**
* Generate OpenL interface, domain classes, project descriptor and unit tests
*/
@Mojo(name = "generate", defaultPhase = LifecyclePhase.GENERATE_SOURCES)
public class GenerateMojo extends BaseOpenLMojo {
/**
* Tasks that will generate classes.
*
* Object Properties
*
*
* Name
* Type
* Required
* Description
*
*
* srcFile
* String
* true
* Reference to the Excel file for which an interface class must be
* generated.
*
*
* targetClass
* String
* true
* Full name of the interface class to be generated. OpenL Tablets
* WebStudio recognizes modules in projects by interface classes and uses
* their names in the user interface. If there are multiple wrappers with
* identical names, only one of them is recognized as a module in OpenL
* Tablets WebStudio.
*
*
* displayName
* String
* false
* End user oriented title of the file that appears in OpenL Tablets
* WebStudio. Default value is Excel file name without extension.
*
*
* targetSrcDir
* String
* false
* Folder where the generated interface class must be placed. For
* example: "src/main/java". Default value is:
* "${project.build.sourceDirectory}"
*
*
* openlName
* String
* false
* OpenL configuration to be used. For OpenL Tablets, the following
* value must always be used: org.openl.xls. Default value is:
* "org.openl.xls"
*
*
* userHome
* String
* false
* Location of user-defined resources relative to the current OpenL
* Tablets project. Default value is: "."
*
*
* userClassPath
* String
* false
* Reference to the folder with additional compiled classes imported
* by the module when the interface is generated. Default value is:
* null.
*
*
* ignoreTestMethods
* boolean
* false
* If true, test methods will not be added to interface class. Used
* only in JavaInterfaceAntTask. Default value is: true.
*
*
* generateUnitTests
* boolean
* false
* Overwrites base {@link #generateUnitTests} value
*
*
* unitTestTemplatePath
* String
* false
* Overwrites base {@link #unitTestTemplatePath} value
*
*
* overwriteUnitTests
* boolean
* false
* Overwrites base {@link #overwriteUnitTests} value
*
*
*
*/
@Parameter(required = true)
private JavaAntTask[] generateInterfaces;
/**
* If true, rules.xml will be generated if it doesn't exist. If
* false, rules.xml will not be generated. Default value is "true".
* @see #overwriteProjectDescriptor
*/
@Parameter(defaultValue = "true")
private boolean createProjectDescriptor;
/**
* If true, rules.xml will be overwritten on each run. If
* false, rules.xml generation will be skipped if it exists.
* Makes sense only if {@link #createProjectDescriptor} == true.
* Default value is "true".
* @see #createProjectDescriptor
*/
@Parameter(defaultValue = "true")
private boolean overwriteProjectDescriptor;
/**
* Default project name in rules.xml. If omitted, the name of the first
* module in the project is used. Used only if createProjectDescriptor ==
* true.
*/
@Parameter
private String projectName;
/**
* Default classpath entries in rules.xml. Default value is {"."} Used only
* if createProjectDescriptor == true.
*/
@Parameter
private String[] classpaths = { "." };
/**
* If true, JUnit tests for OpenL Tablets Test tables will be generated.
* Default value is "false"
*/
@Parameter(defaultValue = "false")
private Boolean generateUnitTests;
/**
* Path to Velocity template for generated unit tests.
* If omitted, default template will be used.
* Available in template variables:
*
*
* Name
* Description
*
*
* openlInterfacePackage
* Package of generated interface class
*
*
* openlInterfaceClass
* Generated interface class name
*
*
* testMethodNames
* Available test method names
*
*
* projectRoot
* Root directory of OpenL project
*
*
* srcFile
* Reference to the Excel file for which an interface class must be
* generated.
*
*
* StringUtils
* Apache commons utility class
*
*
*/
@Parameter(defaultValue = "org/openl/rules/maven/JUnitTestTemplate.vm")
private String unitTestTemplatePath;
/**
* If true, existing JUnit tests will be overwritten. If false, only absent tests will be generated, others will be skipped.
*/
@Parameter(defaultValue = "false")
private Boolean overwriteUnitTests;
@Override
public void execute() throws MojoExecutionException {
if (getLog().isInfoEnabled()) {
getLog().info("Running OpenL GenerateMojo...");
}
for (JavaAntTask task : generateInterfaces) {
if (getLog().isInfoEnabled()) {
getLog().info(String.format("Generating classes for module '%s'...", task.getSrcFile()));
}
initDefaultValues(task);
task.execute();
}
}
private void initDefaultValues(JavaAntTask task) {
if (task.getOpenlName() == null) {
task.setOpenlName(OpenL.OPENL_JAVA_RULE_NAME);
}
if (task.getTargetSrcDir() == null) {
task.setTargetSrcDir(project.getBuild().getSourceDirectory());
}
if (task.getDisplayName() == null) {
task.setDisplayName(FilenameUtils.getBaseName(task.getSrcFile()));
}
initResourcePath(task);
if (task instanceof JavaInterfaceAntTask) {
JavaInterfaceAntTask interfaceTask = (JavaInterfaceAntTask) task;
initCreateProjectDescriptorState(interfaceTask);
interfaceTask.setDefaultProjectName(projectName);
interfaceTask.setDefaultClasspaths(classpaths);
if (task instanceof GenerateInterface) {
GenerateInterface generateInterface = (GenerateInterface) task;
generateInterface.setLog(getLog());
generateInterface.setTestSourceDirectory(project.getBuild().getTestSourceDirectory());
if (generateInterface.getGenerateUnitTests() == null) {
generateInterface.setGenerateUnitTests(generateUnitTests);
}
if (generateInterface.getUnitTestTemplatePath() == null) {
generateInterface.setUnitTestTemplatePath(unitTestTemplatePath);
}
if (generateInterface.getOverwriteUnitTests() == null) {
generateInterface.setOverwriteUnitTests(overwriteUnitTests);
}
}
}
}
private void initCreateProjectDescriptorState(JavaInterfaceAntTask task) {
if (createProjectDescriptor) {
if (new File(task.getResourcesPath(), "rules.xml").exists()) {
task.setCreateProjectDescriptor(overwriteProjectDescriptor);
return;
}
}
task.setCreateProjectDescriptor(createProjectDescriptor);
}
private void initResourcePath(JavaAntTask task) {
String srcFile = task.getSrcFile().replace("\\", "/");
String baseDir = project.getBasedir().getAbsolutePath();
String directory = getSubDirectory(baseDir, openlResourcesDirectory).replace("\\", "/");
if (srcFile.startsWith(directory)) {
srcFile = getSubDirectory(directory, srcFile);
task.setResourcesPath(directory);
task.setSrcFile(srcFile);
return;
}
@SuppressWarnings("unchecked")
List resources = (List) project.getResources();
for (Resource resource : resources) {
String resourceDirectory = resource.getDirectory();
resourceDirectory = getSubDirectory(baseDir, resourceDirectory).replace("\\", "/");
if (srcFile.startsWith(resourceDirectory)) {
srcFile = getSubDirectory(resourceDirectory, srcFile);
task.setResourcesPath(resourceDirectory);
task.setSrcFile(srcFile);
break;
}
}
}
private String getSubDirectory(String baseDir, String resourceDirectory) {
if (resourceDirectory.startsWith(baseDir)) {
resourceDirectory = resourceDirectory.substring(resourceDirectory.lastIndexOf(baseDir) + baseDir.length());
resourceDirectory = removeSlashFromBeginning(resourceDirectory);
}
return resourceDirectory;
}
private String removeSlashFromBeginning(String resourceDirectory) {
if (resourceDirectory.startsWith("/") || resourceDirectory.startsWith("\\")) {
resourceDirectory = resourceDirectory.substring(1);
}
return resourceDirectory;
}
}