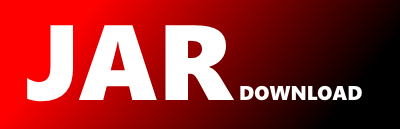
org.openl.util.ce.impl.SequenceActivity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.openl.rules.ce Show documentation
Show all versions of org.openl.rules.ce Show documentation
OpenL Tablets Concurrent Edition
package org.openl.util.ce.impl;
import java.util.ArrayList;
import java.util.List;
import org.openl.util.ce.IActivity;
import org.openl.util.ce.ICallableActivity;
import org.openl.util.ce.IInvokableActivity;
import org.openl.util.ce.IScheduledActivity;
import org.openl.vm.IRuntimeEnv;
public class SequenceActivity implements IScheduledActivity {
int id;
int precedentSize;
List dependents = new ArrayList();
IScheduledActivity[] activities;
boolean isInvokable;
public SequenceActivity(IScheduledActivity[] activities, boolean isInvokable) {
this.activities = activities;
this.isInvokable = isInvokable;
}
@Override
public IActivity activity() {
if (isInvokable)
return invokableActivity();
return callableActivity();
}
private ICallableActivity> callableActivity() {
return new ICallableActivity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy