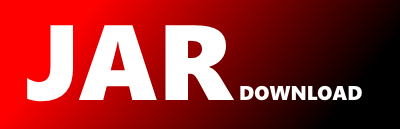
org.openl.ie.constrainer.IntExp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.openl.rules.constrainer Show documentation
Show all versions of org.openl.rules.constrainer Show documentation
OpenL Constraint Programming engine
package org.openl.ie.constrainer;
///////////////////////////////////////////////////////////////////////////////
/*
* Copyright Exigen Group 1998, 1999, 2000
* 320 Amboy Ave., Metuchen, NJ, 08840, USA, www.exigengroup.com
*
* The copyright to the computer program(s) herein
* is the property of Exigen Group, USA. All rights reserved.
* The program(s) may be used and/or copied only with
* the written permission of Exigen Group
* or in accordance with the terms and conditions
* stipulated in the agreement/contract under which
* the program(s) have been supplied.
*/
///////////////////////////////////////////////////////////////////////////////
//
//: IntExp.java
//
/**
* An interface for the constrained integer expression. Any arithmetic operation where the operands are the integer
* variables/expressions returns the integer expression.
*/
public interface IntExp extends Expression {
/**
* An interface used in the iteration of the domain of the integer expression.
*
* @see #iterateDomain
*/
interface IntDomainIterator {
/**
* Processes each value contained within domain one by one (by convention from lowest to highest value).
*
* It should return false
to stop iteration or true
to continue.
*
* @see IntExp#iterateDomain
*/
boolean doSomethingOrStop(int val) throws Failure;
}
/**
* The minimum value of the int type. Note that MIN_VALUE == -MAX_VALUE
is not true for
* java.lang.Integer
.
*/
int MIN_VALUE = -Integer.MAX_VALUE;
/**
* The largest value of type int.
*/
int MAX_VALUE = Integer.MAX_VALUE;
/**
* Returns the expression: (this + value)
.
*/
IntExp add(int value);
/**
* Returns the expression: (this + exp)
.
*/
IntExp add(IntExp exp);
/**
* Returns true if this expression is bound. An expression is considered bound if contains only one value in its
* domain.
*
* @return true
if this expression is bound: it's domain has only one value.
*/
boolean bound();
/**
* Returns true if the domain of this expression contains the value.
*
* NOTE: in some cases it is not possible (or feasible) to calculate contains
with 100% accuracy.
* We also cannot guarantee a consistency between contains
and size
method for all
* expressions. In other words if contains(value)
returns true
it might happen that value
* does not belong to the expression's domain. The opposite (false
) is always true :).
*
* @return true
if the expression contains the value in it's domain.
*/
boolean contains(int value);
/**
* Returns the display string for the current domain of this expression.
*/
String domainToString();
/**
* Returns the boolean expression: (this == value)
.
*/
IntBoolExp eq(int value);
/**
* Returns the constraint: (this == value)
.
*/
Constraint equals(int value);
/**
* Returns the boolean expression: (this >= value)
.
*/
IntBoolExp ge(int value);
/**
* Returns the boolean expression: (this > value)
.
*/
IntBoolExp gt(int value);
/**
* Returns the boolean expression: (this > exp)
.
*/
IntBoolExp gt(IntExp exp);
/**
* Iterates the domain of this expression by calling doSomethingOrStop(int val)
of
* IntDomainIterator
.
*
* @see IntDomainIterator
* @see IntDomainIterator#doSomethingOrStop
*/
void iterateDomain(IntDomainIterator it) throws Failure;
/**
* Returns the boolean expression: (this <= value)
.
*/
IntBoolExp le(int value);
/**
* Returns the boolean expression: (this < value)
.
*/
IntBoolExp lt(int value);
/**
* Returns the boolean expression: (this < exp)
.
*/
IntBoolExp lt(IntExp exp);
/**
* Returns the largest value of the domain of this expression.
*/
int max();
/**
* Returns the smallest value of the domain of this expression.
*/
int min();
/**
* Removes the range [min..max] from the domain of this expression.
*
* @param min range minimum value.
* @param max range maxinum value.
* @throws Failure if domain becomes empty.
*/
void removeRange(int min, int max) throws Failure;
/**
* Removes the value from the domain of this expression.
*
* @throws Failure if domain becomes empty.
*/
void removeValue(int value) throws Failure;
/**
* Sets the maximum value for this expression.
*
* @param max new maximum value.
* @throws Failure if domain becomes empty.
*/
void setMax(int max) throws Failure;
/**
* Sets the minimum value for this expression.
*
* @param min new miminum value.
* @throws Failure if domain becomes empty.
*/
void setMin(int min) throws Failure;
/**
* Bounds this variable to be equal to the value.
*
* @param value a value to be set.
* @throws Failure when: !this.contains(value)
.
* @see #bound
*/
void setValue(int value) throws Failure;
/**
* Returns the size (cardinality) of the domain of this expression. The domain's size is the number of integer
* values the domain.
*
* @return the number of values in the domain of this variable.
*/
int size();
/**
* Returns the value this expression if it is bound.
*
* @return the value of this expresion.
* @throws Failure if this expresionis not bound.
*
* @see #bound
*/
int value() throws Failure;
/**
*
* @return value without checking bound(); should be called only after the caller checked the bounds already
*/
int valueUnsafe();
} // ~IntExp
© 2015 - 2024 Weber Informatics LLC | Privacy Policy