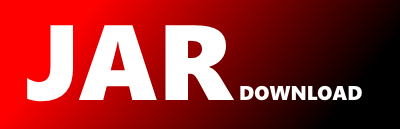
org.openl.rules.spring.openapi.OpenApiUtils Maven / Gradle / Ivy
The newest version!
package org.openl.rules.spring.openapi;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.lang.reflect.TypeVariable;
import java.util.Optional;
import java.util.Set;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpSession;
import io.swagger.v3.oas.annotations.Hidden;
import io.swagger.v3.oas.annotations.Operation;
import org.springframework.core.GenericTypeResolver;
import org.springframework.core.MethodParameter;
import org.springframework.core.ResolvableType;
import org.springframework.core.annotation.AnnotationUtils;
import org.springframework.core.io.Resource;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartRequest;
import org.openl.util.StringUtils;
public final class OpenApiUtils {
private static final Set> FILE_TYPES = Set.of(Resource.class, MultipartFile.class, MultipartRequest.class);
private static final Set> TYPES_TO_IGNORE = Set
.of(ServletRequest.class, ServletResponse.class, HttpSession.class);
private OpenApiUtils() {
}
/**
*
*
* @return
*/
public static String getRequestBasePath() {
var requestAttrs = RequestContextHolder.getRequestAttributes();
if (requestAttrs instanceof ServletRequestAttributes) {
final var request = ((ServletRequestAttributes) requestAttrs).getRequest();
final var scheme = request.getScheme();
final var port = request.getServerPort();
final var url = new StringBuilder(scheme).append("://").append(request.getServerName());
if ("http".equals(scheme) && port != 80 || "https".equals(scheme) && port != 443) {
url.append(':').append(request.getServerPort());
}
Optional.of(request.getContextPath()).filter(StringUtils::isNotBlank).ifPresent(url::append);
Optional.of(request.getServletPath()).filter(StringUtils::isNotBlank).ifPresent(url::append);
return url.toString();
}
return null;
}
public static boolean isFile(Type type) {
var rawClass = ResolvableType.forType(type).getRawClass();
if (rawClass != null) {
return FILE_TYPES.stream().anyMatch(clazz -> clazz.isAssignableFrom(rawClass));
}
return false;
}
public static boolean isVoid(Type type) {
var cl = ResolvableType.forType(type).getRawClass();
if (cl != null) {
return cl == Void.class || cl == void.class;
}
return false;
}
public static Type getType(MethodParameter methodParameter) {
var genericType = methodParameter.getGenericParameterType();
if (genericType instanceof ParameterizedType || genericType instanceof TypeVariable) {
return GenericTypeResolver.resolveType(genericType, methodParameter.getContainingClass());
} else {
return methodParameter.getParameterType();
}
}
public static Type getReturnType(Method method) {
return getType(new MethodParameter(method, -1));
}
public static boolean isIgnorableType(Type type) {
var cl = ResolvableType.forType(type).getRawClass();
if (cl != null) {
return TYPES_TO_IGNORE.stream().anyMatch(clazz -> clazz.isAssignableFrom(cl));
}
return false;
}
public static boolean isHiddenApiMethod(Method method) {
var anno = AnnotationUtils.findAnnotation(method, Operation.class);
return anno != null && anno.hidden() || AnnotationUtils.findAnnotation(method,
Hidden.class) != null || isHidden(method.getDeclaringClass());
}
public static boolean isHidden(Class> cl) {
return AnnotationUtils.findAnnotation(cl, Hidden.class) != null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy