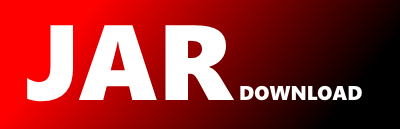
org.openl.rules.ui.ProjectModel Maven / Gradle / Ivy
The newest version!
package org.openl.rules.ui;
import java.io.File;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import org.apache.commons.lang.ArrayUtils;
import org.apache.commons.lang.StringUtils;
import org.openl.CompiledOpenClass;
import org.openl.OpenL;
import org.openl.conf.ClassLoaderFactory;
import org.openl.conf.OpenLConfiguration;
import org.openl.message.OpenLMessage;
import org.openl.message.OpenLMessages;
import org.openl.message.Severity;
import org.openl.rules.dependency.graph.DependencyRulesGraph;
import org.openl.rules.lang.xls.IXlsTableNames;
import org.openl.rules.lang.xls.XlsNodeTypes;
import org.openl.rules.lang.xls.XlsWorkbookListener;
import org.openl.rules.lang.xls.XlsWorkbookSourceCodeModule;
import org.openl.rules.lang.xls.XlsWorkbookSourceHistoryListener;
import org.openl.rules.lang.xls.binding.XlsMetaInfo;
import org.openl.rules.lang.xls.syntax.TableSyntaxNode;
import org.openl.rules.lang.xls.syntax.TableSyntaxNodeAdapter;
import org.openl.rules.lang.xls.syntax.WorkbookSyntaxNode;
import org.openl.rules.lang.xls.syntax.XlsModuleSyntaxNode;
import org.openl.rules.project.ModulesCache;
import org.openl.rules.project.abstraction.RulesProject;
import org.openl.rules.project.instantiation.ReloadType;
import org.openl.rules.project.instantiation.RulesInstantiationStrategy;
import org.openl.rules.project.model.Module;
import org.openl.rules.project.resolving.ResolvingStrategy;
import org.openl.rules.project.resolving.RulesProjectResolver;
import org.openl.rules.search.IOpenLSearch;
import org.openl.rules.search.ISearchTableRow;
import org.openl.rules.search.OpenLAdvancedSearchResult;
import org.openl.rules.search.OpenLAdvancedSearchResultViewer;
import org.openl.rules.search.OpenLBussinessSearchResult;
import org.openl.rules.search.OpenLSavedSearch;
import org.openl.rules.search.OpenLAdvancedSearchResult.TableAndRows;
import org.openl.rules.table.CompositeGrid;
import org.openl.rules.table.IGridTable;
import org.openl.rules.table.IOpenLTable;
import org.openl.rules.table.OpenLTable;
import org.openl.rules.table.xls.XlsCellStyle2;
import org.openl.rules.table.xls.XlsSheetGridHelper;
import org.openl.rules.table.xls.XlsSheetGridImporter;
import org.openl.rules.table.xls.XlsSheetGridModel;
import org.openl.rules.table.xls.XlsUrlParser;
import org.openl.rules.table.xls.XlsUrlUtils;
import org.openl.rules.tableeditor.model.TableEditorModel;
import org.openl.rules.testmethod.TestSuiteMethod;
import org.openl.rules.testmethod.TestUnit;
import org.openl.rules.testmethod.TestUnitsResults;
import org.openl.rules.types.OpenMethodDispatcher;
import org.openl.rules.ui.tests.results.RanTestsResults;
import org.openl.rules.ui.tests.results.Test;
import org.openl.rules.ui.tree.OpenMethodsGroupTreeNodeBuilder;
import org.openl.rules.ui.tree.ProjectTreeNode;
import org.openl.rules.ui.tree.TreeBuilder;
import org.openl.rules.ui.tree.TreeCache;
import org.openl.rules.ui.tree.TreeNodeBuilder;
import org.openl.rules.validation.properties.dimentional.DispatcherTablesBuilder;
import org.openl.rules.ui.RecentlyVisitedTables;
import org.openl.source.SourceHistoryManager;
import org.openl.syntax.code.Dependency;
import org.openl.syntax.code.DependencyType;
import org.openl.syntax.exception.SyntaxNodeException;
import org.openl.syntax.impl.IdentifierNode;
import org.openl.types.IMemberMetaInfo;
import org.openl.types.IOpenClass;
import org.openl.types.IOpenMethod;
import org.openl.types.NullOpenClass;
import org.openl.types.impl.IBenchmarkableMethod;
import org.openl.util.Log;
import org.openl.util.RuntimeExceptionWrapper;
import org.openl.util.benchmark.Benchmark;
import org.openl.util.benchmark.BenchmarkInfo;
import org.openl.util.benchmark.BenchmarkUnit;
import org.openl.util.export.IExporter;
import org.openl.util.tree.ITreeElement;
import org.openl.vm.IRuntimeEnv;
import org.openl.vm.SimpleVM;
import org.openl.vm.trace.Tracer;
public class ProjectModel {
/**
* Compiled rules with errors. Representation of wrapper.
*/
private CompiledOpenClass compiledOpenClass;
private Module moduleInfo;
private ModulesCache modulesCache = new ModulesCache();
private ProjectIndexer indexer;
private WebStudio studio;
private ColorFilterHolder filterHolder = new ColorFilterHolder();
private OpenLSavedSearch[] savedSearches;
private ProjectTreeNode projectRoot = null;
private TreeCache> idTreeCache = new TreeCache>();
private TreeCache uriTreeCache = new TreeCache();
private DependencyRulesGraph dependencyGraph;
private SourceHistoryManager historyManager;
private RecentlyVisitedTables recentlyVisitedTables = new RecentlyVisitedTables();
public ProjectModel(WebStudio studio) {
this.studio = studio;
}
public RulesProject getProject() {
return studio.getCurrentProject();
}
public BenchmarkInfo benchmarkElement(String elementUri, final String testName, String testID,
final String testDescr, int ms) throws Exception {
BenchmarkUnit bu = null;
if (StringUtils.isBlank(testName)) {
IOpenMethod m = getMethod(elementUri);
return benchmarkMethod(m, ms);
}
final RanTestsResults atr = getRunMethods(elementUri);
final int tid = Integer.parseInt(testID);
final IRuntimeEnv env = new SimpleVM().getRuntimeEnv();
final Object target = compiledOpenClass.getOpenClassWithErrors().newInstance(env);
bu = new BenchmarkUnit() {
@Override
public String getName() {
return testDescr;
}
@Override
public void run() throws Exception {
throw new RuntimeException();
}
@Override
public void runNtimes(int times) throws Exception {
try {
atr.run(testName, tid, target, env, times);
} catch (Throwable t) {
Log.error("Error during Method run: ", t);
throw RuntimeExceptionWrapper.wrap(t);
}
}
@Override
public String[] unitName() {
return new String[] { testName + ":" + tid };
}
};
BenchmarkUnit[] buu = { bu };
return new Benchmark(buu).runUnit(bu, ms, false);
}
public BenchmarkInfo benchmarkMethod(final IOpenMethod m, int ms) throws Exception {
final IRuntimeEnv env = new SimpleVM().getRuntimeEnv();
final Object target = compiledOpenClass.getOpenClassWithErrors().newInstance(env);
ClassLoader currentContextClassLoader = Thread.currentThread().getContextClassLoader();
try {
Thread.currentThread().setContextClassLoader(compiledOpenClass.getClassLoader());
final Object[] params = {};
// Object res = null;
BenchmarkUnit bu = null;
try {
if (m instanceof IBenchmarkableMethod) {
final IBenchmarkableMethod bm = (IBenchmarkableMethod) m;
bu = new BenchmarkUnit() {
@Override
public String getName() {
return bm.getBenchmarkName();
}
@Override
public int nUnitRuns() {
return bm.nUnitRuns();
}
@Override
protected void run() throws Exception {
throw new RuntimeException();
}
@Override
public void runNtimes(int times) throws Exception {
bm.invokeBenchmark(target, params, env, times);
}
@Override
public String[] unitName() {
return bm.unitName();
}
};
} else {
bu = new BenchmarkUnit() {
@Override
public String getName() {
return m.getName();
}
@Override
protected void run() throws Exception {
m.invoke(target, params, env);
}
};
}
BenchmarkUnit[] buu = { bu };
return new Benchmark(buu).runUnit(bu, ms, false);
} catch (Throwable t) {
Log.error("Run Error:", t);
return new BenchmarkInfo(t, bu, bu.getName());
}
} finally {
Thread.currentThread().setContextClassLoader(currentContextClassLoader);
}
}
private Object convertTestResult(Object res) {
if (res == null) {
return null;
}
Class> clazz = res.getClass();
if (!(clazz == TestUnitsResults.class)) {
return res;
}
TestUnitsResults tr = (TestUnitsResults) res;
Object[] unitResults = new Object[tr.getNumberOfTestUnits()];
int i = 0;
for (TestUnit testUnit : tr.getTestUnits()) {
unitResults[i] = testUnit.getActualResult();
i++;
}
return unitResults;
}
public TableSyntaxNode findAnyTableNodeByLocation(XlsUrlParser p1) {
TableSyntaxNode[] nodes = getTableSyntaxNodes();
for (int i = 0; i < nodes.length; i++) {
if (nodes[i].getType().equals(XlsNodeTypes.XLS_DT.toString())
&& XlsUrlUtils.intersectsByLocation(p1, nodes[i].getGridTable().getUri())) {
return nodes[i];
}
}
return null;
}
public TableSyntaxNode findNode(String url) {
XlsUrlParser parsedUrl = new XlsUrlParser();
parsedUrl.parse(url);
if (parsedUrl.range == null) {
return null;
}
return findNode(parsedUrl);
}
public String findTableUri(String partialUri) {
TableSyntaxNode tableSyntaxNode = findNode(partialUri);
if (tableSyntaxNode != null) {
return tableSyntaxNode.getUri();
}
return null;
}
public TableSyntaxNode findNode(XlsUrlParser p1) {
TableSyntaxNode[] nodes = getTableSyntaxNodes();
for (int i = 0; i < nodes.length; i++) {
if (XlsUrlUtils.intersects(p1, nodes[i].getGridTable().getUri())) {
return nodes[i];
}
}
return null;
}
public List getAllValidatedNodes() {
if (compiledOpenClass == null) {
return Collections.emptyList();
}
TableSyntaxNode[] nodes = getTableSyntaxNodes();
List list = new ArrayList();
for (int i = 0; i < nodes.length; i++) {
TableSyntaxNode tsn = nodes[i];
if (tsn.getType() == XlsNodeTypes.XLS_DT.toString()) {
if (tsn.getErrors() == null) {
if (tsn.getTableProperties() != null) {
if ("on".equals(tsn.getTableProperties().getValidateDT())) {
list.add(tsn);
}
}
}
}
}
return list;
}
public String getTreeNodeId(ITreeElement> treeNode) {
return idTreeCache.getKey(treeNode);
}
public String getTreeNodeId(String uri) {
ProjectTreeNode node = uriTreeCache.getNode(uri);
String nodeId = idTreeCache.getKey(node);
return nodeId;
}
public ProjectTreeNode getTreeNodeById(String id) {
return (ProjectTreeNode) idTreeCache.getNode(id);
}
public ProjectTreeNode getTreeNodeByUri(String uri) {
return uriTreeCache.getNode(uri);
}
public ColorFilterHolder getFilterHolder() {
return filterHolder;
}
/**
* @return Returns the indexer.
*/
public ProjectIndexer getIndexer() {
return indexer;
}
public IOpenMethod getMethod(String elementUri) {
TableSyntaxNode tsn = getNode(elementUri);
if (tsn == null) {
return null;
}
return getMethod(tsn);
}
public List getTargetMethods(String testOrRunUri) {
List targetMethods = new ArrayList();
IOpenMethod testMethod = getMethod(testOrRunUri);
if (testMethod instanceof TestSuiteMethod) {
IOpenMethod targetMethod = ((TestSuiteMethod) testMethod).getTestedMethod();
// Overloaded methods
if (targetMethod instanceof OpenMethodDispatcher) {
List overloadedMethods = ((OpenMethodDispatcher) targetMethod).getCandidates();
targetMethods.addAll(overloadedMethods);
} else {
targetMethods.add(targetMethod);
}
}
return targetMethods;
}
public List getTargetTables(String testOrRunUri) {
List targetTables = new ArrayList();
List targetMethods = getTargetMethods(testOrRunUri);
for (IOpenMethod targetMethod : targetMethods) {
if (targetMethod != null) {
IMemberMetaInfo methodInfo = targetMethod.getInfo();
if (methodInfo != null) {
TableSyntaxNode tsn = (TableSyntaxNode) methodInfo.getSyntaxNode();
IOpenLTable targetTable = new TableSyntaxNodeAdapter(tsn);
targetTables.add(targetTable);
}
}
}
return targetTables;
}
public IOpenMethod getMethod(TableSyntaxNode tsn) {
if(!isProjectCompiledSuccessfully()) {
return null;
}
IOpenClass openClass = compiledOpenClass.getOpenClassWithErrors();
for (IOpenMethod method : openClass.getMethods()) {
IOpenMethod resolvedMethod = null;
if (method instanceof OpenMethodDispatcher) {
resolvedMethod = resolveMethodDispatcher((OpenMethodDispatcher) method, tsn);
} else {
resolvedMethod = resolveMethod(method, tsn);
}
if (resolvedMethod != null) {
return resolvedMethod;
}
}
return null;
}
private IOpenMethod resolveMethodDispatcher(OpenMethodDispatcher method, TableSyntaxNode syntaxNode) {
List candidates = method.getCandidates();
for (IOpenMethod candidate : candidates) {
IOpenMethod resolvedMethod = resolveMethod(candidate, syntaxNode);
if (resolvedMethod != null) {
return method;
}
}
return null;
}
private IOpenMethod resolveMethod(IOpenMethod method, TableSyntaxNode syntaxNode) {
if (isInstanceOfTable(method, syntaxNode)) {
return method;
}
return null;
}
/**
* Checks that {@link IOpenMethod} object is instance that represents the
* given {@TableSyntaxNode} object. Actually,
* {@link IOpenMethod} object must have the same syntax node as given one.
* If given method is instance of {@link OpenMethodDispatcher}
* false
value will be returned.
*
* @param method method to check
* @param syntaxNode syntax node
* @return true
if {@link IOpenMethod} object represents the
* given table syntax node; false
- otherwise
*/
private boolean isInstanceOfTable(IOpenMethod method, TableSyntaxNode syntaxNode) {
IMemberMetaInfo metaInfo = method.getInfo();
return (metaInfo != null && metaInfo.getSyntaxNode() == syntaxNode);
}
public TableSyntaxNode getNode(String elementUri) {
TableSyntaxNode tsn = null;
if (elementUri != null) {
ProjectTreeNode pte = getTreeNodeByUri(elementUri);
if (pte != null) {
tsn = (TableSyntaxNode) pte.getObject();
}
if (tsn == null) {
tsn = findNode(elementUri);
}
}
return tsn;
}
public synchronized ITreeElement> getProjectTree() {
if (projectRoot == null) {
buildProjectTree();
}
return projectRoot;
}
public RanTestsResults getRunMethods(String elementUri) {
IOpenMethod m = getMethod(elementUri);
if (m == null) {
return null;
}
IOpenMethod[] runners = ProjectHelper.runners(m);
String[] names = new String[runners.length];
for (int i = 0; i < runners.length; i++) {
names[i] = ProjectHelper.createTestName(runners[i]);
}
return new RanTestsResults(runners, names);
}
public OpenLSavedSearch[] getSavedSearches() {
if (savedSearches == null && isReady()) {
TableSyntaxNode[] nodes = getTableSyntaxNodes();
List savedSearches = new ArrayList();
for (TableSyntaxNode node : nodes) {
if (node.getType().equals(XlsNodeTypes.XLS_PERSISTENT.toString())) {
String code = node.getHeader().getModule().getCode();
if ((IXlsTableNames.PERSISTENCE_TABLE + " " + OpenLSavedSearch.class.getName()).equals(code)) {
OpenLSavedSearch savedSearch = new OpenLSavedSearch().restore(new XlsSheetGridImporter(
(XlsSheetGridModel) node.getGridTable().getGrid(), node));
savedSearches.add(savedSearch);
}
}
}
this.savedSearches = savedSearches.toArray(new OpenLSavedSearch[savedSearches.size()]);
}
return savedSearches;
}
public List getAdvancedSearchResults(Object searchResult) {
List searchResults = new ArrayList();
if (searchResult instanceof OpenLAdvancedSearchResult) {
TableAndRows[] tr = ((OpenLAdvancedSearchResult) searchResult).getFoundTableAndRows();
OpenLAdvancedSearchResultViewer searchViewer = new OpenLAdvancedSearchResultViewer();
for (int i = 0; i < tr.length; i++) {
ISearchTableRow[] rows = tr[i].getRows();
if (rows.length > 0) {
TableSyntaxNode tsn = tr[i].getTsn();
String tableUri = tsn.getUri();
CompositeGrid cg = searchViewer.makeGrid(rows);
IGridTable gridTable = cg != null ? cg.asGridTable() : null;
OpenLTable newTable = new OpenLTable();
newTable.setGridTable(gridTable);
newTable.setUri(tableUri);
newTable.setProperties(tsn.getTableProperties());
searchResults.add(newTable);
}
}
}
return searchResults;
}
public List getBussinessSearchResults(Object searchResult) {
List searchResults = new ArrayList();
if (searchResult instanceof OpenLBussinessSearchResult) {
List foundTables = ((OpenLBussinessSearchResult) searchResult).getFoundTables();
for (TableSyntaxNode foundTable : foundTables) {
searchResults.add(new TableSyntaxNodeAdapter(foundTable));
}
}
return searchResults;
}
public WebStudio getStudio() {
return studio;
}
public IOpenLTable getTable(String elementUri) {
TableSyntaxNode tsn = getNode(elementUri);
if (tsn != null) {
return new TableSyntaxNodeAdapter(tsn);
}
return null;
}
public IGridTable getGridTable(String elementUri) {
TableSyntaxNode tsn = getNode(elementUri);
return tsn == null ? null : tsn.getGridTable();
}
public String getTableView(String view) {
return view == null ? studio.getMode().getTableMode() : view;
}
public RanTestsResults getTestsRunner(IOpenMethod[] testMethods) {
String[] names = new String[testMethods.length];
for (int i = 0; i < testMethods.length; i++) {
names[i] = ProjectHelper.createTestName(testMethods[i]);
}
return new RanTestsResults(testMethods, names);
}
/**
* Get runnable tests for tested method by uri.
* Runnable tests - tests with filled rules rows data for testing its functionality (with test cases).
* If you need to get all test methods, including and empty ones, use {@link #getAllTestMethods(String).
*
* @param elementUri
* @return
*/
public RanTestsResults getTestMethods(String elementUri) {
IOpenMethod[] testMethods = null;
IOpenMethod method = getMethod(elementUri);
if (method != null) {
if (ProjectHelper.isTester(method)) {
testMethods = new IOpenMethod[] {method};
} else {
testMethods = ProjectHelper.testers(method);
}
if (ArrayUtils.isNotEmpty(testMethods)) {
return getTestsRunner(testMethods);
}
}
return null;
}
/**
* Gets all test methods for method by uri.
*
* @param elementUri
* @return all test methods, including tests with test cases, runs with filled runs, tests without cases(empty),
* runs without any parameters and tests without cases and runs.
*/
public RanTestsResults getAllTestMethods(String elementUri) {
IOpenMethod[] testMethods = null;
IOpenMethod method = getMethod(elementUri);
if (method != null) {
testMethods = ProjectHelper.allTesters(method);
if (ArrayUtils.isNotEmpty(testMethods)) {
return getTestsRunner(testMethods);
}
}
return null;
}
public RanTestsResults getAllTestMethods() {
if (isProjectCompiledSuccessfully()) {
IOpenMethod[] testMethods = ProjectHelper.allTesters(compiledOpenClass.getOpenClassWithErrors());
return getTestsRunner(testMethods);
}
return null;
}
// TODO Refactor
private WorkbookSyntaxNode[] getWorkbookNodes() {
if (!isProjectCompiledSuccessfully()) {
return null;
}
if (compiledOpenClass != null) {
XlsModuleSyntaxNode xlsModuleNode = ((XlsMetaInfo) compiledOpenClass.getOpenClassWithErrors().getMetaInfo())
.getXlsModuleNode();
WorkbookSyntaxNode[] workbookNodes = xlsModuleNode.getWorkbookSyntaxNodes();
return workbookNodes;
}
return null;
}
public boolean isSourceModified() {
WorkbookSyntaxNode[] workbookNodes = getWorkbookNodes();
if (workbookNodes != null) {
for (WorkbookSyntaxNode node : workbookNodes) {
if (node.getWorkbookSourceCodeModule().isModified()) {
return true;
}
}
}
return false;
}
public CompiledOpenClass getCompiledOpenClass() {
return compiledOpenClass;
}
/**
* @return Returns the wrapperInfo.
*/
public Module getModuleInfo() {
return moduleInfo;
}
public XlsModuleSyntaxNode getXlsModuleNode() {
if (!isProjectCompiledSuccessfully()) {
return null;
}
XlsMetaInfo xmi = (XlsMetaInfo) compiledOpenClass.getOpenClassWithErrors().getMetaInfo();
XlsModuleSyntaxNode xsn = xmi.getXlsModuleNode();
return xsn;
}
/**
* Returns if current project is read only.
*
* @return true
if project is read only.
*/
public boolean isEditable() {
RulesProject project = getProject();
if (project != null) {
return project.isCheckedOut() || project.isLocalOnly();
}
return false;
}
public boolean isReady() {
return compiledOpenClass != null;
}
public boolean isMethodHasParams(String elementUri) {
IOpenMethod m = getMethod(elementUri);
if (m == null) {
return false;
}
return ProjectHelper.isMethodHasParams(m);
}
public boolean isTestable(String elementUri) {
IOpenMethod m = getMethod(elementUri);
if (m == null) {
return false;
}
return ProjectHelper.isTestable(m);
}
public boolean isTestable(TableSyntaxNode tsn) {
IOpenMethod m = getMethod(tsn);
if (m == null) {
return false;
}
return ProjectHelper.isTestable(m);
}
public synchronized void buildProjectTree() {
if (compiledOpenClass == null) {
return;
}
ProjectTreeNode root = makeProjectTreeRoot();
TableSyntaxNode[] tableSyntaxNodes = getTableSyntaxNodes();
OverloadedMethodsDictionary methodNodesDictionary = makeMethodNodesDictionary(tableSyntaxNodes);
TreeBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy