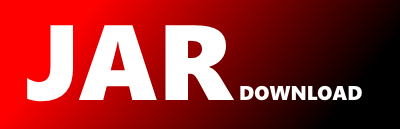
org.openl.rules.ui.tree.VersionedTreeNode Maven / Gradle / Ivy
The newest version!
package org.openl.rules.ui.tree;
import java.util.Iterator;
import java.util.TreeMap;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.openl.rules.lang.xls.syntax.TableSyntaxNode;
import org.openl.rules.ui.IProjectTypes;
import org.openl.util.conf.Version;
/**
* Folder tree node that represents table with several versions. If node has
* several versions then folder node will be linked with one of its child. If
* node has only one version then it will be leaf in tree.
*
* Linked child is child with "active" table(there is only one "active" table
* among all version of the table) or the child with greatest version.
*
* @author PUdalau
*/
public class VersionedTreeNode extends ProjectTreeNode {
private static Log LOG = LogFactory.getLog(VersionedTreeNode.class);
private TableSyntaxNode linkedChild;
public VersionedTreeNode(String[] displayName, TableSyntaxNode table) {
super(displayName, IProjectTypes.PT_TABLE + "." + table.getType(), null, null, 0, null);
}
@Override
public String getType() {
return IProjectTypes.PT_TABLE + "." + linkedChild.getType();
}
@Override
public String getUri() {
return linkedChild.getUri();
}
@Override
public Object getProblems() {
return linkedChild.getErrors() != null ? linkedChild.getErrors() : linkedChild.getValidationResult();
}
@Override
public boolean hasProblems() {
return getProblems() != null;
}
@Override
public TableSyntaxNode getTableSyntaxNode() {
return linkedChild;
}
@Override
public TreeMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy