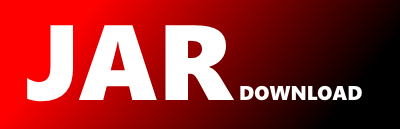
org.openl.OpenL Maven / Gradle / Ivy
/*
* Created on May 30, 2003
*
* Developed by Intelligent ChoicePoint Inc. 2003
*/
package org.openl;
import java.io.File;
import java.util.Map;
import org.apache.commons.collections4.map.ReferenceMap;
import org.openl.cache.GenericKey;
import org.openl.conf.IOpenLBuilder;
import org.openl.conf.IOpenLConfiguration;
import org.openl.conf.IUserContext;
import org.openl.conf.OpenConfigurationException;
import org.openl.conf.OpenLConfigurator;
import org.openl.conf.UserContext;
/**
* This class describes OpenL engine context abstraction that used during
* compilation process.
*
* The class OpenL implements both factory(static) methods for creating OpenL
* instances and actual OpenL functionality. Each instance of OpenL should be
* considered as a Language Configuration(LC). You may have as many LCs in your
* application as you want. Current OpenL architecture allows to have different
* OpenL configurations in separate class loaders, so they will not interfere
* with each other. It allows, for example, to have 2 LCs using different SAX or
* DOM parser implementation.
*
* The actual work is done by class OpenLConfigurator.
*
* @see OpenLConfigurator
* @author snshor
*/
public class OpenL {
public static final String OPENL_J_NAME = "org.openl.j";
public static final String OPENL_JAVA_NAME = "org.openl.rules.java";
public static final String OPENL_JAVA_CE_NAME = "org.openl.rules.java.ce";
public static final String OPENL_JAVA_RULE_NAME = "org.openl.xls";
private static final String DEFAULT_USER_HOME = ".";
private static OpenLConfigurator config = new OpenLConfigurator();
// Soft references to values are used to prevent memory leak
private static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy