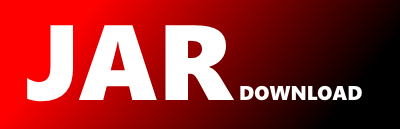
org.openlca.proto.ProtoExchange Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: olca.proto
package org.openlca.proto;
/**
*
* An Exchange is an input or output of a [Flow] in a [Process]. The amount of
* an exchange is given in a specific unit of a quantity ([FlowProperty]) of
* the flow. The allowed units and flow properties that can be used for a flow
* in an exchange are defined by the flow property information in that flow
* (see also the [FlowPropertyFactor] type).
*
*
* Protobuf type {@code protolca.ProtoExchange}
*/
public final class ProtoExchange extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protolca.ProtoExchange)
ProtoExchangeOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProtoExchange.newBuilder() to construct.
private ProtoExchange(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProtoExchange() {
costFormula_ = "";
amountFormula_ = "";
dqEntry_ = "";
description_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ProtoExchange();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProtoExchange(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
avoidedProduct_ = input.readBool();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
costFormula_ = s;
break;
}
case 25: {
costValue_ = input.readDouble();
break;
}
case 34: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (currency_ != null) {
subBuilder = currency_.toBuilder();
}
currency_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(currency_);
currency_ = subBuilder.buildPartial();
}
break;
}
case 40: {
internalId_ = input.readInt32();
break;
}
case 50: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (flow_ != null) {
subBuilder = flow_.toBuilder();
}
flow_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(flow_);
flow_ = subBuilder.buildPartial();
}
break;
}
case 58: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (flowProperty_ != null) {
subBuilder = flowProperty_.toBuilder();
}
flowProperty_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(flowProperty_);
flowProperty_ = subBuilder.buildPartial();
}
break;
}
case 64: {
input_ = input.readBool();
break;
}
case 72: {
quantitativeReference_ = input.readBool();
break;
}
case 81: {
baseUncertainty_ = input.readDouble();
break;
}
case 90: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (defaultProvider_ != null) {
subBuilder = defaultProvider_.toBuilder();
}
defaultProvider_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(defaultProvider_);
defaultProvider_ = subBuilder.buildPartial();
}
break;
}
case 97: {
amount_ = input.readDouble();
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
amountFormula_ = s;
break;
}
case 114: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (unit_ != null) {
subBuilder = unit_.toBuilder();
}
unit_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(unit_);
unit_ = subBuilder.buildPartial();
}
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
dqEntry_ = s;
break;
}
case 130: {
org.openlca.proto.ProtoUncertainty.Builder subBuilder = null;
if (uncertainty_ != null) {
subBuilder = uncertainty_.toBuilder();
}
uncertainty_ = input.readMessage(org.openlca.proto.ProtoUncertainty.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(uncertainty_);
uncertainty_ = subBuilder.buildPartial();
}
break;
}
case 138: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoExchange_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoExchange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openlca.proto.ProtoExchange.class, org.openlca.proto.ProtoExchange.Builder.class);
}
public static final int AVOIDED_PRODUCT_FIELD_NUMBER = 1;
private boolean avoidedProduct_;
/**
*
* Indicates whether this exchange is an avoided product.
*
*
* bool avoided_product = 1;
* @return The avoidedProduct.
*/
@java.lang.Override
public boolean getAvoidedProduct() {
return avoidedProduct_;
}
public static final int COST_FORMULA_FIELD_NUMBER = 2;
private volatile java.lang.Object costFormula_;
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return The costFormula.
*/
@java.lang.Override
public java.lang.String getCostFormula() {
java.lang.Object ref = costFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
costFormula_ = s;
return s;
}
}
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return The bytes for costFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCostFormulaBytes() {
java.lang.Object ref = costFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
costFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COST_VALUE_FIELD_NUMBER = 3;
private double costValue_;
/**
*
* The costs of this exchange.
*
*
* double cost_value = 3;
* @return The costValue.
*/
@java.lang.Override
public double getCostValue() {
return costValue_;
}
public static final int CURRENCY_FIELD_NUMBER = 4;
private org.openlca.proto.ProtoRef currency_;
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
* @return Whether the currency field is set.
*/
@java.lang.Override
public boolean hasCurrency() {
return currency_ != null;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
* @return The currency.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getCurrency() {
return currency_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : currency_;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getCurrencyOrBuilder() {
return getCurrency();
}
public static final int INTERNAL_ID_FIELD_NUMBER = 5;
private int internalId_;
/**
*
* The process internal ID of the exchange. This is used to identify
* exchanges unambiguously within a process (e.g. when linking exchanges in a
* product system where multiple exchanges with the same flow are allowed).
* The value should be >= 1.
*
*
* int32 internal_id = 5;
* @return The internalId.
*/
@java.lang.Override
public int getInternalId() {
return internalId_;
}
public static final int FLOW_FIELD_NUMBER = 6;
private org.openlca.proto.ProtoRef flow_;
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
* @return Whether the flow field is set.
*/
@java.lang.Override
public boolean hasFlow() {
return flow_ != null;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
* @return The flow.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getFlow() {
return flow_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : flow_;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getFlowOrBuilder() {
return getFlow();
}
public static final int FLOW_PROPERTY_FIELD_NUMBER = 7;
private org.openlca.proto.ProtoRef flowProperty_;
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
* @return Whether the flowProperty field is set.
*/
@java.lang.Override
public boolean hasFlowProperty() {
return flowProperty_ != null;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
* @return The flowProperty.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getFlowProperty() {
return flowProperty_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : flowProperty_;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getFlowPropertyOrBuilder() {
return getFlowProperty();
}
public static final int INPUT_FIELD_NUMBER = 8;
private boolean input_;
/**
* bool input = 8;
* @return The input.
*/
@java.lang.Override
public boolean getInput() {
return input_;
}
public static final int QUANTITATIVE_REFERENCE_FIELD_NUMBER = 9;
private boolean quantitativeReference_;
/**
*
* Indicates whether the exchange is the quantitative reference of the
* process.
*
*
* bool quantitative_reference = 9;
* @return The quantitativeReference.
*/
@java.lang.Override
public boolean getQuantitativeReference() {
return quantitativeReference_;
}
public static final int BASE_UNCERTAINTY_FIELD_NUMBER = 10;
private double baseUncertainty_;
/**
* double base_uncertainty = 10;
* @return The baseUncertainty.
*/
@java.lang.Override
public double getBaseUncertainty() {
return baseUncertainty_;
}
public static final int DEFAULT_PROVIDER_FIELD_NUMBER = 11;
private org.openlca.proto.ProtoRef defaultProvider_;
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
* @return Whether the defaultProvider field is set.
*/
@java.lang.Override
public boolean hasDefaultProvider() {
return defaultProvider_ != null;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
* @return The defaultProvider.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getDefaultProvider() {
return defaultProvider_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : defaultProvider_;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getDefaultProviderOrBuilder() {
return getDefaultProvider();
}
public static final int AMOUNT_FIELD_NUMBER = 12;
private double amount_;
/**
* double amount = 12;
* @return The amount.
*/
@java.lang.Override
public double getAmount() {
return amount_;
}
public static final int AMOUNT_FORMULA_FIELD_NUMBER = 13;
private volatile java.lang.Object amountFormula_;
/**
* string amount_formula = 13;
* @return The amountFormula.
*/
@java.lang.Override
public java.lang.String getAmountFormula() {
java.lang.Object ref = amountFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
amountFormula_ = s;
return s;
}
}
/**
* string amount_formula = 13;
* @return The bytes for amountFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAmountFormulaBytes() {
java.lang.Object ref = amountFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
amountFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNIT_FIELD_NUMBER = 14;
private org.openlca.proto.ProtoRef unit_;
/**
* .protolca.ProtoRef unit = 14;
* @return Whether the unit field is set.
*/
@java.lang.Override
public boolean hasUnit() {
return unit_ != null;
}
/**
* .protolca.ProtoRef unit = 14;
* @return The unit.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getUnit() {
return unit_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : unit_;
}
/**
* .protolca.ProtoRef unit = 14;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getUnitOrBuilder() {
return getUnit();
}
public static final int DQ_ENTRY_FIELD_NUMBER = 15;
private volatile java.lang.Object dqEntry_;
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return The dqEntry.
*/
@java.lang.Override
public java.lang.String getDqEntry() {
java.lang.Object ref = dqEntry_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dqEntry_ = s;
return s;
}
}
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return The bytes for dqEntry.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDqEntryBytes() {
java.lang.Object ref = dqEntry_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dqEntry_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNCERTAINTY_FIELD_NUMBER = 16;
private org.openlca.proto.ProtoUncertainty uncertainty_;
/**
* .protolca.ProtoUncertainty uncertainty = 16;
* @return Whether the uncertainty field is set.
*/
@java.lang.Override
public boolean hasUncertainty() {
return uncertainty_ != null;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
* @return The uncertainty.
*/
@java.lang.Override
public org.openlca.proto.ProtoUncertainty getUncertainty() {
return uncertainty_ == null ? org.openlca.proto.ProtoUncertainty.getDefaultInstance() : uncertainty_;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
@java.lang.Override
public org.openlca.proto.ProtoUncertaintyOrBuilder getUncertaintyOrBuilder() {
return getUncertainty();
}
public static final int DESCRIPTION_FIELD_NUMBER = 17;
private volatile java.lang.Object description_;
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (avoidedProduct_ != false) {
output.writeBool(1, avoidedProduct_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(costFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, costFormula_);
}
if (costValue_ != 0D) {
output.writeDouble(3, costValue_);
}
if (currency_ != null) {
output.writeMessage(4, getCurrency());
}
if (internalId_ != 0) {
output.writeInt32(5, internalId_);
}
if (flow_ != null) {
output.writeMessage(6, getFlow());
}
if (flowProperty_ != null) {
output.writeMessage(7, getFlowProperty());
}
if (input_ != false) {
output.writeBool(8, input_);
}
if (quantitativeReference_ != false) {
output.writeBool(9, quantitativeReference_);
}
if (baseUncertainty_ != 0D) {
output.writeDouble(10, baseUncertainty_);
}
if (defaultProvider_ != null) {
output.writeMessage(11, getDefaultProvider());
}
if (amount_ != 0D) {
output.writeDouble(12, amount_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(amountFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, amountFormula_);
}
if (unit_ != null) {
output.writeMessage(14, getUnit());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(dqEntry_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, dqEntry_);
}
if (uncertainty_ != null) {
output.writeMessage(16, getUncertainty());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, description_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (avoidedProduct_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, avoidedProduct_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(costFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, costFormula_);
}
if (costValue_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, costValue_);
}
if (currency_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getCurrency());
}
if (internalId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, internalId_);
}
if (flow_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getFlow());
}
if (flowProperty_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getFlowProperty());
}
if (input_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, input_);
}
if (quantitativeReference_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, quantitativeReference_);
}
if (baseUncertainty_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(10, baseUncertainty_);
}
if (defaultProvider_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getDefaultProvider());
}
if (amount_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(12, amount_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(amountFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, amountFormula_);
}
if (unit_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getUnit());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(dqEntry_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, dqEntry_);
}
if (uncertainty_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getUncertainty());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, description_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openlca.proto.ProtoExchange)) {
return super.equals(obj);
}
org.openlca.proto.ProtoExchange other = (org.openlca.proto.ProtoExchange) obj;
if (getAvoidedProduct()
!= other.getAvoidedProduct()) return false;
if (!getCostFormula()
.equals(other.getCostFormula())) return false;
if (java.lang.Double.doubleToLongBits(getCostValue())
!= java.lang.Double.doubleToLongBits(
other.getCostValue())) return false;
if (hasCurrency() != other.hasCurrency()) return false;
if (hasCurrency()) {
if (!getCurrency()
.equals(other.getCurrency())) return false;
}
if (getInternalId()
!= other.getInternalId()) return false;
if (hasFlow() != other.hasFlow()) return false;
if (hasFlow()) {
if (!getFlow()
.equals(other.getFlow())) return false;
}
if (hasFlowProperty() != other.hasFlowProperty()) return false;
if (hasFlowProperty()) {
if (!getFlowProperty()
.equals(other.getFlowProperty())) return false;
}
if (getInput()
!= other.getInput()) return false;
if (getQuantitativeReference()
!= other.getQuantitativeReference()) return false;
if (java.lang.Double.doubleToLongBits(getBaseUncertainty())
!= java.lang.Double.doubleToLongBits(
other.getBaseUncertainty())) return false;
if (hasDefaultProvider() != other.hasDefaultProvider()) return false;
if (hasDefaultProvider()) {
if (!getDefaultProvider()
.equals(other.getDefaultProvider())) return false;
}
if (java.lang.Double.doubleToLongBits(getAmount())
!= java.lang.Double.doubleToLongBits(
other.getAmount())) return false;
if (!getAmountFormula()
.equals(other.getAmountFormula())) return false;
if (hasUnit() != other.hasUnit()) return false;
if (hasUnit()) {
if (!getUnit()
.equals(other.getUnit())) return false;
}
if (!getDqEntry()
.equals(other.getDqEntry())) return false;
if (hasUncertainty() != other.hasUncertainty()) return false;
if (hasUncertainty()) {
if (!getUncertainty()
.equals(other.getUncertainty())) return false;
}
if (!getDescription()
.equals(other.getDescription())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + AVOIDED_PRODUCT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAvoidedProduct());
hash = (37 * hash) + COST_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getCostFormula().hashCode();
hash = (37 * hash) + COST_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCostValue()));
if (hasCurrency()) {
hash = (37 * hash) + CURRENCY_FIELD_NUMBER;
hash = (53 * hash) + getCurrency().hashCode();
}
hash = (37 * hash) + INTERNAL_ID_FIELD_NUMBER;
hash = (53 * hash) + getInternalId();
if (hasFlow()) {
hash = (37 * hash) + FLOW_FIELD_NUMBER;
hash = (53 * hash) + getFlow().hashCode();
}
if (hasFlowProperty()) {
hash = (37 * hash) + FLOW_PROPERTY_FIELD_NUMBER;
hash = (53 * hash) + getFlowProperty().hashCode();
}
hash = (37 * hash) + INPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getInput());
hash = (37 * hash) + QUANTITATIVE_REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getQuantitativeReference());
hash = (37 * hash) + BASE_UNCERTAINTY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getBaseUncertainty()));
if (hasDefaultProvider()) {
hash = (37 * hash) + DEFAULT_PROVIDER_FIELD_NUMBER;
hash = (53 * hash) + getDefaultProvider().hashCode();
}
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getAmount()));
hash = (37 * hash) + AMOUNT_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getAmountFormula().hashCode();
if (hasUnit()) {
hash = (37 * hash) + UNIT_FIELD_NUMBER;
hash = (53 * hash) + getUnit().hashCode();
}
hash = (37 * hash) + DQ_ENTRY_FIELD_NUMBER;
hash = (53 * hash) + getDqEntry().hashCode();
if (hasUncertainty()) {
hash = (37 * hash) + UNCERTAINTY_FIELD_NUMBER;
hash = (53 * hash) + getUncertainty().hashCode();
}
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openlca.proto.ProtoExchange parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoExchange parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoExchange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoExchange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoExchange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoExchange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoExchange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoExchange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openlca.proto.ProtoExchange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoExchange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openlca.proto.ProtoExchange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoExchange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openlca.proto.ProtoExchange prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* An Exchange is an input or output of a [Flow] in a [Process]. The amount of
* an exchange is given in a specific unit of a quantity ([FlowProperty]) of
* the flow. The allowed units and flow properties that can be used for a flow
* in an exchange are defined by the flow property information in that flow
* (see also the [FlowPropertyFactor] type).
*
*
* Protobuf type {@code protolca.ProtoExchange}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protolca.ProtoExchange)
org.openlca.proto.ProtoExchangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoExchange_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoExchange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openlca.proto.ProtoExchange.class, org.openlca.proto.ProtoExchange.Builder.class);
}
// Construct using org.openlca.proto.ProtoExchange.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
avoidedProduct_ = false;
costFormula_ = "";
costValue_ = 0D;
if (currencyBuilder_ == null) {
currency_ = null;
} else {
currency_ = null;
currencyBuilder_ = null;
}
internalId_ = 0;
if (flowBuilder_ == null) {
flow_ = null;
} else {
flow_ = null;
flowBuilder_ = null;
}
if (flowPropertyBuilder_ == null) {
flowProperty_ = null;
} else {
flowProperty_ = null;
flowPropertyBuilder_ = null;
}
input_ = false;
quantitativeReference_ = false;
baseUncertainty_ = 0D;
if (defaultProviderBuilder_ == null) {
defaultProvider_ = null;
} else {
defaultProvider_ = null;
defaultProviderBuilder_ = null;
}
amount_ = 0D;
amountFormula_ = "";
if (unitBuilder_ == null) {
unit_ = null;
} else {
unit_ = null;
unitBuilder_ = null;
}
dqEntry_ = "";
if (uncertaintyBuilder_ == null) {
uncertainty_ = null;
} else {
uncertainty_ = null;
uncertaintyBuilder_ = null;
}
description_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoExchange_descriptor;
}
@java.lang.Override
public org.openlca.proto.ProtoExchange getDefaultInstanceForType() {
return org.openlca.proto.ProtoExchange.getDefaultInstance();
}
@java.lang.Override
public org.openlca.proto.ProtoExchange build() {
org.openlca.proto.ProtoExchange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openlca.proto.ProtoExchange buildPartial() {
org.openlca.proto.ProtoExchange result = new org.openlca.proto.ProtoExchange(this);
result.avoidedProduct_ = avoidedProduct_;
result.costFormula_ = costFormula_;
result.costValue_ = costValue_;
if (currencyBuilder_ == null) {
result.currency_ = currency_;
} else {
result.currency_ = currencyBuilder_.build();
}
result.internalId_ = internalId_;
if (flowBuilder_ == null) {
result.flow_ = flow_;
} else {
result.flow_ = flowBuilder_.build();
}
if (flowPropertyBuilder_ == null) {
result.flowProperty_ = flowProperty_;
} else {
result.flowProperty_ = flowPropertyBuilder_.build();
}
result.input_ = input_;
result.quantitativeReference_ = quantitativeReference_;
result.baseUncertainty_ = baseUncertainty_;
if (defaultProviderBuilder_ == null) {
result.defaultProvider_ = defaultProvider_;
} else {
result.defaultProvider_ = defaultProviderBuilder_.build();
}
result.amount_ = amount_;
result.amountFormula_ = amountFormula_;
if (unitBuilder_ == null) {
result.unit_ = unit_;
} else {
result.unit_ = unitBuilder_.build();
}
result.dqEntry_ = dqEntry_;
if (uncertaintyBuilder_ == null) {
result.uncertainty_ = uncertainty_;
} else {
result.uncertainty_ = uncertaintyBuilder_.build();
}
result.description_ = description_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openlca.proto.ProtoExchange) {
return mergeFrom((org.openlca.proto.ProtoExchange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openlca.proto.ProtoExchange other) {
if (other == org.openlca.proto.ProtoExchange.getDefaultInstance()) return this;
if (other.getAvoidedProduct() != false) {
setAvoidedProduct(other.getAvoidedProduct());
}
if (!other.getCostFormula().isEmpty()) {
costFormula_ = other.costFormula_;
onChanged();
}
if (other.getCostValue() != 0D) {
setCostValue(other.getCostValue());
}
if (other.hasCurrency()) {
mergeCurrency(other.getCurrency());
}
if (other.getInternalId() != 0) {
setInternalId(other.getInternalId());
}
if (other.hasFlow()) {
mergeFlow(other.getFlow());
}
if (other.hasFlowProperty()) {
mergeFlowProperty(other.getFlowProperty());
}
if (other.getInput() != false) {
setInput(other.getInput());
}
if (other.getQuantitativeReference() != false) {
setQuantitativeReference(other.getQuantitativeReference());
}
if (other.getBaseUncertainty() != 0D) {
setBaseUncertainty(other.getBaseUncertainty());
}
if (other.hasDefaultProvider()) {
mergeDefaultProvider(other.getDefaultProvider());
}
if (other.getAmount() != 0D) {
setAmount(other.getAmount());
}
if (!other.getAmountFormula().isEmpty()) {
amountFormula_ = other.amountFormula_;
onChanged();
}
if (other.hasUnit()) {
mergeUnit(other.getUnit());
}
if (!other.getDqEntry().isEmpty()) {
dqEntry_ = other.dqEntry_;
onChanged();
}
if (other.hasUncertainty()) {
mergeUncertainty(other.getUncertainty());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.openlca.proto.ProtoExchange parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.openlca.proto.ProtoExchange) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean avoidedProduct_ ;
/**
*
* Indicates whether this exchange is an avoided product.
*
*
* bool avoided_product = 1;
* @return The avoidedProduct.
*/
@java.lang.Override
public boolean getAvoidedProduct() {
return avoidedProduct_;
}
/**
*
* Indicates whether this exchange is an avoided product.
*
*
* bool avoided_product = 1;
* @param value The avoidedProduct to set.
* @return This builder for chaining.
*/
public Builder setAvoidedProduct(boolean value) {
avoidedProduct_ = value;
onChanged();
return this;
}
/**
*
* Indicates whether this exchange is an avoided product.
*
*
* bool avoided_product = 1;
* @return This builder for chaining.
*/
public Builder clearAvoidedProduct() {
avoidedProduct_ = false;
onChanged();
return this;
}
private java.lang.Object costFormula_ = "";
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return The costFormula.
*/
public java.lang.String getCostFormula() {
java.lang.Object ref = costFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
costFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return The bytes for costFormula.
*/
public com.google.protobuf.ByteString
getCostFormulaBytes() {
java.lang.Object ref = costFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
costFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @param value The costFormula to set.
* @return This builder for chaining.
*/
public Builder setCostFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
costFormula_ = value;
onChanged();
return this;
}
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return This builder for chaining.
*/
public Builder clearCostFormula() {
costFormula_ = getDefaultInstance().getCostFormula();
onChanged();
return this;
}
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @param value The bytes for costFormula to set.
* @return This builder for chaining.
*/
public Builder setCostFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
costFormula_ = value;
onChanged();
return this;
}
private double costValue_ ;
/**
*
* The costs of this exchange.
*
*
* double cost_value = 3;
* @return The costValue.
*/
@java.lang.Override
public double getCostValue() {
return costValue_;
}
/**
*
* The costs of this exchange.
*
*
* double cost_value = 3;
* @param value The costValue to set.
* @return This builder for chaining.
*/
public Builder setCostValue(double value) {
costValue_ = value;
onChanged();
return this;
}
/**
*
* The costs of this exchange.
*
*
* double cost_value = 3;
* @return This builder for chaining.
*/
public Builder clearCostValue() {
costValue_ = 0D;
onChanged();
return this;
}
private org.openlca.proto.ProtoRef currency_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> currencyBuilder_;
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
* @return Whether the currency field is set.
*/
public boolean hasCurrency() {
return currencyBuilder_ != null || currency_ != null;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
* @return The currency.
*/
public org.openlca.proto.ProtoRef getCurrency() {
if (currencyBuilder_ == null) {
return currency_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : currency_;
} else {
return currencyBuilder_.getMessage();
}
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
public Builder setCurrency(org.openlca.proto.ProtoRef value) {
if (currencyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
currency_ = value;
onChanged();
} else {
currencyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
public Builder setCurrency(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (currencyBuilder_ == null) {
currency_ = builderForValue.build();
onChanged();
} else {
currencyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
public Builder mergeCurrency(org.openlca.proto.ProtoRef value) {
if (currencyBuilder_ == null) {
if (currency_ != null) {
currency_ =
org.openlca.proto.ProtoRef.newBuilder(currency_).mergeFrom(value).buildPartial();
} else {
currency_ = value;
}
onChanged();
} else {
currencyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
public Builder clearCurrency() {
if (currencyBuilder_ == null) {
currency_ = null;
onChanged();
} else {
currency_ = null;
currencyBuilder_ = null;
}
return this;
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
public org.openlca.proto.ProtoRef.Builder getCurrencyBuilder() {
onChanged();
return getCurrencyFieldBuilder().getBuilder();
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
public org.openlca.proto.ProtoRefOrBuilder getCurrencyOrBuilder() {
if (currencyBuilder_ != null) {
return currencyBuilder_.getMessageOrBuilder();
} else {
return currency_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : currency_;
}
}
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getCurrencyFieldBuilder() {
if (currencyBuilder_ == null) {
currencyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getCurrency(),
getParentForChildren(),
isClean());
currency_ = null;
}
return currencyBuilder_;
}
private int internalId_ ;
/**
*
* The process internal ID of the exchange. This is used to identify
* exchanges unambiguously within a process (e.g. when linking exchanges in a
* product system where multiple exchanges with the same flow are allowed).
* The value should be >= 1.
*
*
* int32 internal_id = 5;
* @return The internalId.
*/
@java.lang.Override
public int getInternalId() {
return internalId_;
}
/**
*
* The process internal ID of the exchange. This is used to identify
* exchanges unambiguously within a process (e.g. when linking exchanges in a
* product system where multiple exchanges with the same flow are allowed).
* The value should be >= 1.
*
*
* int32 internal_id = 5;
* @param value The internalId to set.
* @return This builder for chaining.
*/
public Builder setInternalId(int value) {
internalId_ = value;
onChanged();
return this;
}
/**
*
* The process internal ID of the exchange. This is used to identify
* exchanges unambiguously within a process (e.g. when linking exchanges in a
* product system where multiple exchanges with the same flow are allowed).
* The value should be >= 1.
*
*
* int32 internal_id = 5;
* @return This builder for chaining.
*/
public Builder clearInternalId() {
internalId_ = 0;
onChanged();
return this;
}
private org.openlca.proto.ProtoRef flow_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> flowBuilder_;
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
* @return Whether the flow field is set.
*/
public boolean hasFlow() {
return flowBuilder_ != null || flow_ != null;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
* @return The flow.
*/
public org.openlca.proto.ProtoRef getFlow() {
if (flowBuilder_ == null) {
return flow_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : flow_;
} else {
return flowBuilder_.getMessage();
}
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
public Builder setFlow(org.openlca.proto.ProtoRef value) {
if (flowBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
flow_ = value;
onChanged();
} else {
flowBuilder_.setMessage(value);
}
return this;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
public Builder setFlow(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (flowBuilder_ == null) {
flow_ = builderForValue.build();
onChanged();
} else {
flowBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
public Builder mergeFlow(org.openlca.proto.ProtoRef value) {
if (flowBuilder_ == null) {
if (flow_ != null) {
flow_ =
org.openlca.proto.ProtoRef.newBuilder(flow_).mergeFrom(value).buildPartial();
} else {
flow_ = value;
}
onChanged();
} else {
flowBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
public Builder clearFlow() {
if (flowBuilder_ == null) {
flow_ = null;
onChanged();
} else {
flow_ = null;
flowBuilder_ = null;
}
return this;
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
public org.openlca.proto.ProtoRef.Builder getFlowBuilder() {
onChanged();
return getFlowFieldBuilder().getBuilder();
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
public org.openlca.proto.ProtoRefOrBuilder getFlowOrBuilder() {
if (flowBuilder_ != null) {
return flowBuilder_.getMessageOrBuilder();
} else {
return flow_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : flow_;
}
}
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getFlowFieldBuilder() {
if (flowBuilder_ == null) {
flowBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getFlow(),
getParentForChildren(),
isClean());
flow_ = null;
}
return flowBuilder_;
}
private org.openlca.proto.ProtoRef flowProperty_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> flowPropertyBuilder_;
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
* @return Whether the flowProperty field is set.
*/
public boolean hasFlowProperty() {
return flowPropertyBuilder_ != null || flowProperty_ != null;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
* @return The flowProperty.
*/
public org.openlca.proto.ProtoRef getFlowProperty() {
if (flowPropertyBuilder_ == null) {
return flowProperty_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : flowProperty_;
} else {
return flowPropertyBuilder_.getMessage();
}
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
public Builder setFlowProperty(org.openlca.proto.ProtoRef value) {
if (flowPropertyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
flowProperty_ = value;
onChanged();
} else {
flowPropertyBuilder_.setMessage(value);
}
return this;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
public Builder setFlowProperty(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (flowPropertyBuilder_ == null) {
flowProperty_ = builderForValue.build();
onChanged();
} else {
flowPropertyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
public Builder mergeFlowProperty(org.openlca.proto.ProtoRef value) {
if (flowPropertyBuilder_ == null) {
if (flowProperty_ != null) {
flowProperty_ =
org.openlca.proto.ProtoRef.newBuilder(flowProperty_).mergeFrom(value).buildPartial();
} else {
flowProperty_ = value;
}
onChanged();
} else {
flowPropertyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
public Builder clearFlowProperty() {
if (flowPropertyBuilder_ == null) {
flowProperty_ = null;
onChanged();
} else {
flowProperty_ = null;
flowPropertyBuilder_ = null;
}
return this;
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
public org.openlca.proto.ProtoRef.Builder getFlowPropertyBuilder() {
onChanged();
return getFlowPropertyFieldBuilder().getBuilder();
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
public org.openlca.proto.ProtoRefOrBuilder getFlowPropertyOrBuilder() {
if (flowPropertyBuilder_ != null) {
return flowPropertyBuilder_.getMessageOrBuilder();
} else {
return flowProperty_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : flowProperty_;
}
}
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getFlowPropertyFieldBuilder() {
if (flowPropertyBuilder_ == null) {
flowPropertyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getFlowProperty(),
getParentForChildren(),
isClean());
flowProperty_ = null;
}
return flowPropertyBuilder_;
}
private boolean input_ ;
/**
* bool input = 8;
* @return The input.
*/
@java.lang.Override
public boolean getInput() {
return input_;
}
/**
* bool input = 8;
* @param value The input to set.
* @return This builder for chaining.
*/
public Builder setInput(boolean value) {
input_ = value;
onChanged();
return this;
}
/**
* bool input = 8;
* @return This builder for chaining.
*/
public Builder clearInput() {
input_ = false;
onChanged();
return this;
}
private boolean quantitativeReference_ ;
/**
*
* Indicates whether the exchange is the quantitative reference of the
* process.
*
*
* bool quantitative_reference = 9;
* @return The quantitativeReference.
*/
@java.lang.Override
public boolean getQuantitativeReference() {
return quantitativeReference_;
}
/**
*
* Indicates whether the exchange is the quantitative reference of the
* process.
*
*
* bool quantitative_reference = 9;
* @param value The quantitativeReference to set.
* @return This builder for chaining.
*/
public Builder setQuantitativeReference(boolean value) {
quantitativeReference_ = value;
onChanged();
return this;
}
/**
*
* Indicates whether the exchange is the quantitative reference of the
* process.
*
*
* bool quantitative_reference = 9;
* @return This builder for chaining.
*/
public Builder clearQuantitativeReference() {
quantitativeReference_ = false;
onChanged();
return this;
}
private double baseUncertainty_ ;
/**
* double base_uncertainty = 10;
* @return The baseUncertainty.
*/
@java.lang.Override
public double getBaseUncertainty() {
return baseUncertainty_;
}
/**
* double base_uncertainty = 10;
* @param value The baseUncertainty to set.
* @return This builder for chaining.
*/
public Builder setBaseUncertainty(double value) {
baseUncertainty_ = value;
onChanged();
return this;
}
/**
* double base_uncertainty = 10;
* @return This builder for chaining.
*/
public Builder clearBaseUncertainty() {
baseUncertainty_ = 0D;
onChanged();
return this;
}
private org.openlca.proto.ProtoRef defaultProvider_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> defaultProviderBuilder_;
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
* @return Whether the defaultProvider field is set.
*/
public boolean hasDefaultProvider() {
return defaultProviderBuilder_ != null || defaultProvider_ != null;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
* @return The defaultProvider.
*/
public org.openlca.proto.ProtoRef getDefaultProvider() {
if (defaultProviderBuilder_ == null) {
return defaultProvider_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : defaultProvider_;
} else {
return defaultProviderBuilder_.getMessage();
}
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
public Builder setDefaultProvider(org.openlca.proto.ProtoRef value) {
if (defaultProviderBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
defaultProvider_ = value;
onChanged();
} else {
defaultProviderBuilder_.setMessage(value);
}
return this;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
public Builder setDefaultProvider(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (defaultProviderBuilder_ == null) {
defaultProvider_ = builderForValue.build();
onChanged();
} else {
defaultProviderBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
public Builder mergeDefaultProvider(org.openlca.proto.ProtoRef value) {
if (defaultProviderBuilder_ == null) {
if (defaultProvider_ != null) {
defaultProvider_ =
org.openlca.proto.ProtoRef.newBuilder(defaultProvider_).mergeFrom(value).buildPartial();
} else {
defaultProvider_ = value;
}
onChanged();
} else {
defaultProviderBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
public Builder clearDefaultProvider() {
if (defaultProviderBuilder_ == null) {
defaultProvider_ = null;
onChanged();
} else {
defaultProvider_ = null;
defaultProviderBuilder_ = null;
}
return this;
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
public org.openlca.proto.ProtoRef.Builder getDefaultProviderBuilder() {
onChanged();
return getDefaultProviderFieldBuilder().getBuilder();
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
public org.openlca.proto.ProtoRefOrBuilder getDefaultProviderOrBuilder() {
if (defaultProviderBuilder_ != null) {
return defaultProviderBuilder_.getMessageOrBuilder();
} else {
return defaultProvider_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : defaultProvider_;
}
}
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getDefaultProviderFieldBuilder() {
if (defaultProviderBuilder_ == null) {
defaultProviderBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getDefaultProvider(),
getParentForChildren(),
isClean());
defaultProvider_ = null;
}
return defaultProviderBuilder_;
}
private double amount_ ;
/**
* double amount = 12;
* @return The amount.
*/
@java.lang.Override
public double getAmount() {
return amount_;
}
/**
* double amount = 12;
* @param value The amount to set.
* @return This builder for chaining.
*/
public Builder setAmount(double value) {
amount_ = value;
onChanged();
return this;
}
/**
* double amount = 12;
* @return This builder for chaining.
*/
public Builder clearAmount() {
amount_ = 0D;
onChanged();
return this;
}
private java.lang.Object amountFormula_ = "";
/**
* string amount_formula = 13;
* @return The amountFormula.
*/
public java.lang.String getAmountFormula() {
java.lang.Object ref = amountFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
amountFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string amount_formula = 13;
* @return The bytes for amountFormula.
*/
public com.google.protobuf.ByteString
getAmountFormulaBytes() {
java.lang.Object ref = amountFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
amountFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string amount_formula = 13;
* @param value The amountFormula to set.
* @return This builder for chaining.
*/
public Builder setAmountFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
amountFormula_ = value;
onChanged();
return this;
}
/**
* string amount_formula = 13;
* @return This builder for chaining.
*/
public Builder clearAmountFormula() {
amountFormula_ = getDefaultInstance().getAmountFormula();
onChanged();
return this;
}
/**
* string amount_formula = 13;
* @param value The bytes for amountFormula to set.
* @return This builder for chaining.
*/
public Builder setAmountFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
amountFormula_ = value;
onChanged();
return this;
}
private org.openlca.proto.ProtoRef unit_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> unitBuilder_;
/**
* .protolca.ProtoRef unit = 14;
* @return Whether the unit field is set.
*/
public boolean hasUnit() {
return unitBuilder_ != null || unit_ != null;
}
/**
* .protolca.ProtoRef unit = 14;
* @return The unit.
*/
public org.openlca.proto.ProtoRef getUnit() {
if (unitBuilder_ == null) {
return unit_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : unit_;
} else {
return unitBuilder_.getMessage();
}
}
/**
* .protolca.ProtoRef unit = 14;
*/
public Builder setUnit(org.openlca.proto.ProtoRef value) {
if (unitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
unit_ = value;
onChanged();
} else {
unitBuilder_.setMessage(value);
}
return this;
}
/**
* .protolca.ProtoRef unit = 14;
*/
public Builder setUnit(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (unitBuilder_ == null) {
unit_ = builderForValue.build();
onChanged();
} else {
unitBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protolca.ProtoRef unit = 14;
*/
public Builder mergeUnit(org.openlca.proto.ProtoRef value) {
if (unitBuilder_ == null) {
if (unit_ != null) {
unit_ =
org.openlca.proto.ProtoRef.newBuilder(unit_).mergeFrom(value).buildPartial();
} else {
unit_ = value;
}
onChanged();
} else {
unitBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protolca.ProtoRef unit = 14;
*/
public Builder clearUnit() {
if (unitBuilder_ == null) {
unit_ = null;
onChanged();
} else {
unit_ = null;
unitBuilder_ = null;
}
return this;
}
/**
* .protolca.ProtoRef unit = 14;
*/
public org.openlca.proto.ProtoRef.Builder getUnitBuilder() {
onChanged();
return getUnitFieldBuilder().getBuilder();
}
/**
* .protolca.ProtoRef unit = 14;
*/
public org.openlca.proto.ProtoRefOrBuilder getUnitOrBuilder() {
if (unitBuilder_ != null) {
return unitBuilder_.getMessageOrBuilder();
} else {
return unit_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : unit_;
}
}
/**
* .protolca.ProtoRef unit = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getUnitFieldBuilder() {
if (unitBuilder_ == null) {
unitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getUnit(),
getParentForChildren(),
isClean());
unit_ = null;
}
return unitBuilder_;
}
private java.lang.Object dqEntry_ = "";
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return The dqEntry.
*/
public java.lang.String getDqEntry() {
java.lang.Object ref = dqEntry_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dqEntry_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return The bytes for dqEntry.
*/
public com.google.protobuf.ByteString
getDqEntryBytes() {
java.lang.Object ref = dqEntry_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dqEntry_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @param value The dqEntry to set.
* @return This builder for chaining.
*/
public Builder setDqEntry(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
dqEntry_ = value;
onChanged();
return this;
}
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return This builder for chaining.
*/
public Builder clearDqEntry() {
dqEntry_ = getDefaultInstance().getDqEntry();
onChanged();
return this;
}
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @param value The bytes for dqEntry to set.
* @return This builder for chaining.
*/
public Builder setDqEntryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
dqEntry_ = value;
onChanged();
return this;
}
private org.openlca.proto.ProtoUncertainty uncertainty_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoUncertainty, org.openlca.proto.ProtoUncertainty.Builder, org.openlca.proto.ProtoUncertaintyOrBuilder> uncertaintyBuilder_;
/**
* .protolca.ProtoUncertainty uncertainty = 16;
* @return Whether the uncertainty field is set.
*/
public boolean hasUncertainty() {
return uncertaintyBuilder_ != null || uncertainty_ != null;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
* @return The uncertainty.
*/
public org.openlca.proto.ProtoUncertainty getUncertainty() {
if (uncertaintyBuilder_ == null) {
return uncertainty_ == null ? org.openlca.proto.ProtoUncertainty.getDefaultInstance() : uncertainty_;
} else {
return uncertaintyBuilder_.getMessage();
}
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
public Builder setUncertainty(org.openlca.proto.ProtoUncertainty value) {
if (uncertaintyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
uncertainty_ = value;
onChanged();
} else {
uncertaintyBuilder_.setMessage(value);
}
return this;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
public Builder setUncertainty(
org.openlca.proto.ProtoUncertainty.Builder builderForValue) {
if (uncertaintyBuilder_ == null) {
uncertainty_ = builderForValue.build();
onChanged();
} else {
uncertaintyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
public Builder mergeUncertainty(org.openlca.proto.ProtoUncertainty value) {
if (uncertaintyBuilder_ == null) {
if (uncertainty_ != null) {
uncertainty_ =
org.openlca.proto.ProtoUncertainty.newBuilder(uncertainty_).mergeFrom(value).buildPartial();
} else {
uncertainty_ = value;
}
onChanged();
} else {
uncertaintyBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
public Builder clearUncertainty() {
if (uncertaintyBuilder_ == null) {
uncertainty_ = null;
onChanged();
} else {
uncertainty_ = null;
uncertaintyBuilder_ = null;
}
return this;
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
public org.openlca.proto.ProtoUncertainty.Builder getUncertaintyBuilder() {
onChanged();
return getUncertaintyFieldBuilder().getBuilder();
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
public org.openlca.proto.ProtoUncertaintyOrBuilder getUncertaintyOrBuilder() {
if (uncertaintyBuilder_ != null) {
return uncertaintyBuilder_.getMessageOrBuilder();
} else {
return uncertainty_ == null ?
org.openlca.proto.ProtoUncertainty.getDefaultInstance() : uncertainty_;
}
}
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoUncertainty, org.openlca.proto.ProtoUncertainty.Builder, org.openlca.proto.ProtoUncertaintyOrBuilder>
getUncertaintyFieldBuilder() {
if (uncertaintyBuilder_ == null) {
uncertaintyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoUncertainty, org.openlca.proto.ProtoUncertainty.Builder, org.openlca.proto.ProtoUncertaintyOrBuilder>(
getUncertainty(),
getParentForChildren(),
isClean());
uncertainty_ = null;
}
return uncertaintyBuilder_;
}
private java.lang.Object description_ = "";
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protolca.ProtoExchange)
}
// @@protoc_insertion_point(class_scope:protolca.ProtoExchange)
private static final org.openlca.proto.ProtoExchange DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openlca.proto.ProtoExchange();
}
public static org.openlca.proto.ProtoExchange getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProtoExchange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProtoExchange(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openlca.proto.ProtoExchange getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy