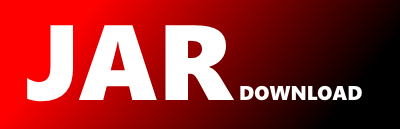
org.openlca.proto.ProtoExchangeOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: olca.proto
package org.openlca.proto;
public interface ProtoExchangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:protolca.ProtoExchange)
com.google.protobuf.MessageOrBuilder {
/**
*
* Indicates whether this exchange is an avoided product.
*
*
* bool avoided_product = 1;
* @return The avoidedProduct.
*/
boolean getAvoidedProduct();
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return The costFormula.
*/
java.lang.String getCostFormula();
/**
*
* A formula for calculating the costs of this exchange.
*
*
* string cost_formula = 2;
* @return The bytes for costFormula.
*/
com.google.protobuf.ByteString
getCostFormulaBytes();
/**
*
* The costs of this exchange.
*
*
* double cost_value = 3;
* @return The costValue.
*/
double getCostValue();
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
* @return Whether the currency field is set.
*/
boolean hasCurrency();
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
* @return The currency.
*/
org.openlca.proto.ProtoRef getCurrency();
/**
*
* The currency in which the costs of this exchange are given.
*
*
* .protolca.ProtoRef currency = 4;
*/
org.openlca.proto.ProtoRefOrBuilder getCurrencyOrBuilder();
/**
*
* The process internal ID of the exchange. This is used to identify
* exchanges unambiguously within a process (e.g. when linking exchanges in a
* product system where multiple exchanges with the same flow are allowed).
* The value should be >= 1.
*
*
* int32 internal_id = 5;
* @return The internalId.
*/
int getInternalId();
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
* @return Whether the flow field is set.
*/
boolean hasFlow();
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
* @return The flow.
*/
org.openlca.proto.ProtoRef getFlow();
/**
*
* The reference to the flow of the exchange.
*
*
* .protolca.ProtoRef flow = 6;
*/
org.openlca.proto.ProtoRefOrBuilder getFlowOrBuilder();
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
* @return Whether the flowProperty field is set.
*/
boolean hasFlowProperty();
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
* @return The flowProperty.
*/
org.openlca.proto.ProtoRef getFlowProperty();
/**
*
* The quantity in which the amount is given.
*
*
* .protolca.ProtoRef flow_property = 7;
*/
org.openlca.proto.ProtoRefOrBuilder getFlowPropertyOrBuilder();
/**
* bool input = 8;
* @return The input.
*/
boolean getInput();
/**
*
* Indicates whether the exchange is the quantitative reference of the
* process.
*
*
* bool quantitative_reference = 9;
* @return The quantitativeReference.
*/
boolean getQuantitativeReference();
/**
* double base_uncertainty = 10;
* @return The baseUncertainty.
*/
double getBaseUncertainty();
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
* @return Whether the defaultProvider field is set.
*/
boolean hasDefaultProvider();
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
* @return The defaultProvider.
*/
org.openlca.proto.ProtoRef getDefaultProvider();
/**
*
* A default provider is a [Process] that is linked as the provider of a
* product input or the waste treatment provider of a waste output. It is
* just an optional default setting which can be also ignored when building
* product systems in openLCA. The user is always free to link processes in
* product systems ignoring these defaults (but the flows and flow directions
* have to match of course).
*
*
* .protolca.ProtoRef default_provider = 11;
*/
org.openlca.proto.ProtoRefOrBuilder getDefaultProviderOrBuilder();
/**
* double amount = 12;
* @return The amount.
*/
double getAmount();
/**
* string amount_formula = 13;
* @return The amountFormula.
*/
java.lang.String getAmountFormula();
/**
* string amount_formula = 13;
* @return The bytes for amountFormula.
*/
com.google.protobuf.ByteString
getAmountFormulaBytes();
/**
* .protolca.ProtoRef unit = 14;
* @return Whether the unit field is set.
*/
boolean hasUnit();
/**
* .protolca.ProtoRef unit = 14;
* @return The unit.
*/
org.openlca.proto.ProtoRef getUnit();
/**
* .protolca.ProtoRef unit = 14;
*/
org.openlca.proto.ProtoRefOrBuilder getUnitOrBuilder();
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return The dqEntry.
*/
java.lang.String getDqEntry();
/**
*
* A data quality entry like `(1;3;2;5;1)`. The entry is a vector of data
* quality values that need to match the data quality scheme for flow inputs
* and outputs that is assigned to the [Process]. In such a scheme the data
* quality indicators have fixed positions and the respective values in the
* `dqEntry` vector map to these positions.
*
*
* string dq_entry = 15;
* @return The bytes for dqEntry.
*/
com.google.protobuf.ByteString
getDqEntryBytes();
/**
* .protolca.ProtoUncertainty uncertainty = 16;
* @return Whether the uncertainty field is set.
*/
boolean hasUncertainty();
/**
* .protolca.ProtoUncertainty uncertainty = 16;
* @return The uncertainty.
*/
org.openlca.proto.ProtoUncertainty getUncertainty();
/**
* .protolca.ProtoUncertainty uncertainty = 16;
*/
org.openlca.proto.ProtoUncertaintyOrBuilder getUncertaintyOrBuilder();
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return The description.
*/
java.lang.String getDescription();
/**
*
* A general comment about the input or output.
*
*
* string description = 17;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy