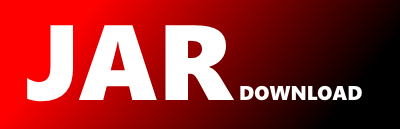
org.openlca.proto.ProtoProject Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: olca.proto
package org.openlca.proto;
/**
* Protobuf type {@code protolca.ProtoProject}
*/
public final class ProtoProject extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protolca.ProtoProject)
ProtoProjectOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProtoProject.newBuilder() to construct.
private ProtoProject(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProtoProject() {
id_ = "";
name_ = "";
description_ = "";
version_ = "";
lastChange_ = "";
type_ = 0;
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
library_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ProtoProject();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProtoProject(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
version_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
lastChange_ = s;
break;
}
case 48: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 58: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (category_ != null) {
subBuilder = category_.toBuilder();
}
category_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(category_);
category_ = subBuilder.buildPartial();
}
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tags_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
tags_.add(s);
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
library_ = s;
break;
}
case 82: {
org.openlca.proto.ProtoRef.Builder subBuilder = null;
if (impactMethod_ != null) {
subBuilder = impactMethod_.toBuilder();
}
impactMethod_ = input.readMessage(org.openlca.proto.ProtoRef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(impactMethod_);
impactMethod_ = subBuilder.buildPartial();
}
break;
}
case 90: {
org.openlca.proto.ProtoNwSet.Builder subBuilder = null;
if (nwSet_ != null) {
subBuilder = nwSet_.toBuilder();
}
nwSet_ = input.readMessage(org.openlca.proto.ProtoNwSet.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(nwSet_);
nwSet_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tags_ = tags_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoProject_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoProject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openlca.proto.ProtoProject.class, org.openlca.proto.ProtoProject.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
*
* The name of the entity.
*
*
* string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* The name of the entity.
*
*
* string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
private volatile java.lang.Object description_;
/**
*
* The description of the entity.
*
*
* string description = 3;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* The description of the entity.
*
*
* string description = 3;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 4;
private volatile java.lang.Object version_;
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @return The version.
*/
@java.lang.Override
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @return The bytes for version.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LAST_CHANGE_FIELD_NUMBER = 5;
private volatile java.lang.Object lastChange_;
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @return The lastChange.
*/
@java.lang.Override
public java.lang.String getLastChange() {
java.lang.Object ref = lastChange_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastChange_ = s;
return s;
}
}
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @return The bytes for lastChange.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLastChangeBytes() {
java.lang.Object ref = lastChange_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lastChange_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 6;
private int type_;
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @return The type.
*/
@java.lang.Override public org.openlca.proto.ProtoType getType() {
@SuppressWarnings("deprecation")
org.openlca.proto.ProtoType result = org.openlca.proto.ProtoType.valueOf(type_);
return result == null ? org.openlca.proto.ProtoType.UNRECOGNIZED : result;
}
public static final int CATEGORY_FIELD_NUMBER = 7;
private org.openlca.proto.ProtoRef category_;
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
* @return Whether the category field is set.
*/
@java.lang.Override
public boolean hasCategory() {
return category_ != null;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
* @return The category.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getCategory() {
return category_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : category_;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getCategoryOrBuilder() {
return getCategory();
}
public static final int TAGS_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList tags_;
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @return A list containing the tags.
*/
public com.google.protobuf.ProtocolStringList
getTagsList() {
return tags_;
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @return The count of tags.
*/
public int getTagsCount() {
return tags_.size();
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param index The index of the element to return.
* @return The tags at the given index.
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param index The index of the value to return.
* @return The bytes of the tags at the given index.
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
public static final int LIBRARY_FIELD_NUMBER = 9;
private volatile java.lang.Object library_;
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @return The library.
*/
@java.lang.Override
public java.lang.String getLibrary() {
java.lang.Object ref = library_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
library_ = s;
return s;
}
}
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @return The bytes for library.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLibraryBytes() {
java.lang.Object ref = library_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
library_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMPACT_METHOD_FIELD_NUMBER = 10;
private org.openlca.proto.ProtoRef impactMethod_;
/**
* .protolca.ProtoRef impact_method = 10;
* @return Whether the impactMethod field is set.
*/
@java.lang.Override
public boolean hasImpactMethod() {
return impactMethod_ != null;
}
/**
* .protolca.ProtoRef impact_method = 10;
* @return The impactMethod.
*/
@java.lang.Override
public org.openlca.proto.ProtoRef getImpactMethod() {
return impactMethod_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : impactMethod_;
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
@java.lang.Override
public org.openlca.proto.ProtoRefOrBuilder getImpactMethodOrBuilder() {
return getImpactMethod();
}
public static final int NW_SET_FIELD_NUMBER = 11;
private org.openlca.proto.ProtoNwSet nwSet_;
/**
* .protolca.ProtoNwSet nw_set = 11;
* @return Whether the nwSet field is set.
*/
@java.lang.Override
public boolean hasNwSet() {
return nwSet_ != null;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
* @return The nwSet.
*/
@java.lang.Override
public org.openlca.proto.ProtoNwSet getNwSet() {
return nwSet_ == null ? org.openlca.proto.ProtoNwSet.getDefaultInstance() : nwSet_;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
@java.lang.Override
public org.openlca.proto.ProtoNwSetOrBuilder getNwSetOrBuilder() {
return getNwSet();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, description_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(version_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, version_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(lastChange_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, lastChange_);
}
if (type_ != org.openlca.proto.ProtoType.Undefined.getNumber()) {
output.writeEnum(6, type_);
}
if (category_ != null) {
output.writeMessage(7, getCategory());
}
for (int i = 0; i < tags_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, tags_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(library_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, library_);
}
if (impactMethod_ != null) {
output.writeMessage(10, getImpactMethod());
}
if (nwSet_ != null) {
output.writeMessage(11, getNwSet());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, description_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(version_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, version_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(lastChange_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, lastChange_);
}
if (type_ != org.openlca.proto.ProtoType.Undefined.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, type_);
}
if (category_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCategory());
}
{
int dataSize = 0;
for (int i = 0; i < tags_.size(); i++) {
dataSize += computeStringSizeNoTag(tags_.getRaw(i));
}
size += dataSize;
size += 1 * getTagsList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(library_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, library_);
}
if (impactMethod_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getImpactMethod());
}
if (nwSet_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getNwSet());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openlca.proto.ProtoProject)) {
return super.equals(obj);
}
org.openlca.proto.ProtoProject other = (org.openlca.proto.ProtoProject) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getName()
.equals(other.getName())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (!getVersion()
.equals(other.getVersion())) return false;
if (!getLastChange()
.equals(other.getLastChange())) return false;
if (type_ != other.type_) return false;
if (hasCategory() != other.hasCategory()) return false;
if (hasCategory()) {
if (!getCategory()
.equals(other.getCategory())) return false;
}
if (!getTagsList()
.equals(other.getTagsList())) return false;
if (!getLibrary()
.equals(other.getLibrary())) return false;
if (hasImpactMethod() != other.hasImpactMethod()) return false;
if (hasImpactMethod()) {
if (!getImpactMethod()
.equals(other.getImpactMethod())) return false;
}
if (hasNwSet() != other.hasNwSet()) return false;
if (hasNwSet()) {
if (!getNwSet()
.equals(other.getNwSet())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
hash = (37 * hash) + LAST_CHANGE_FIELD_NUMBER;
hash = (53 * hash) + getLastChange().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasCategory()) {
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getCategory().hashCode();
}
if (getTagsCount() > 0) {
hash = (37 * hash) + TAGS_FIELD_NUMBER;
hash = (53 * hash) + getTagsList().hashCode();
}
hash = (37 * hash) + LIBRARY_FIELD_NUMBER;
hash = (53 * hash) + getLibrary().hashCode();
if (hasImpactMethod()) {
hash = (37 * hash) + IMPACT_METHOD_FIELD_NUMBER;
hash = (53 * hash) + getImpactMethod().hashCode();
}
if (hasNwSet()) {
hash = (37 * hash) + NW_SET_FIELD_NUMBER;
hash = (53 * hash) + getNwSet().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openlca.proto.ProtoProject parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoProject parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoProject parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoProject parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoProject parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoProject parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoProject parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoProject parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openlca.proto.ProtoProject parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoProject parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openlca.proto.ProtoProject parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoProject parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openlca.proto.ProtoProject prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code protolca.ProtoProject}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protolca.ProtoProject)
org.openlca.proto.ProtoProjectOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoProject_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoProject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openlca.proto.ProtoProject.class, org.openlca.proto.ProtoProject.Builder.class);
}
// Construct using org.openlca.proto.ProtoProject.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
name_ = "";
description_ = "";
version_ = "";
lastChange_ = "";
type_ = 0;
if (categoryBuilder_ == null) {
category_ = null;
} else {
category_ = null;
categoryBuilder_ = null;
}
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
library_ = "";
if (impactMethodBuilder_ == null) {
impactMethod_ = null;
} else {
impactMethod_ = null;
impactMethodBuilder_ = null;
}
if (nwSetBuilder_ == null) {
nwSet_ = null;
} else {
nwSet_ = null;
nwSetBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoProject_descriptor;
}
@java.lang.Override
public org.openlca.proto.ProtoProject getDefaultInstanceForType() {
return org.openlca.proto.ProtoProject.getDefaultInstance();
}
@java.lang.Override
public org.openlca.proto.ProtoProject build() {
org.openlca.proto.ProtoProject result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openlca.proto.ProtoProject buildPartial() {
org.openlca.proto.ProtoProject result = new org.openlca.proto.ProtoProject(this);
int from_bitField0_ = bitField0_;
result.id_ = id_;
result.name_ = name_;
result.description_ = description_;
result.version_ = version_;
result.lastChange_ = lastChange_;
result.type_ = type_;
if (categoryBuilder_ == null) {
result.category_ = category_;
} else {
result.category_ = categoryBuilder_.build();
}
if (((bitField0_ & 0x00000001) != 0)) {
tags_ = tags_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tags_ = tags_;
result.library_ = library_;
if (impactMethodBuilder_ == null) {
result.impactMethod_ = impactMethod_;
} else {
result.impactMethod_ = impactMethodBuilder_.build();
}
if (nwSetBuilder_ == null) {
result.nwSet_ = nwSet_;
} else {
result.nwSet_ = nwSetBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openlca.proto.ProtoProject) {
return mergeFrom((org.openlca.proto.ProtoProject)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openlca.proto.ProtoProject other) {
if (other == org.openlca.proto.ProtoProject.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
onChanged();
}
if (!other.getLastChange().isEmpty()) {
lastChange_ = other.lastChange_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasCategory()) {
mergeCategory(other.getCategory());
}
if (!other.tags_.isEmpty()) {
if (tags_.isEmpty()) {
tags_ = other.tags_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTagsIsMutable();
tags_.addAll(other.tags_);
}
onChanged();
}
if (!other.getLibrary().isEmpty()) {
library_ = other.library_;
onChanged();
}
if (other.hasImpactMethod()) {
mergeImpactMethod(other.getImpactMethod());
}
if (other.hasNwSet()) {
mergeNwSet(other.getNwSet());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.openlca.proto.ProtoProject parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.openlca.proto.ProtoProject) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* The reference ID (typically an UUID) of the entity.
*
*
* string id = 1 [json_name = "@id"];
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* The name of the entity.
*
*
* string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the entity.
*
*
* string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the entity.
*
*
* string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* The name of the entity.
*
*
* string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* The name of the entity.
*
*
* string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* The description of the entity.
*
*
* string description = 3;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The description of the entity.
*
*
* string description = 3;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The description of the entity.
*
*
* string description = 3;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* The description of the entity.
*
*
* string description = 3;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* The description of the entity.
*
*
* string description = 3;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object version_ = "";
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @return The version.
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @return The bytes for version.
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
return this;
}
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @return This builder for chaining.
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
onChanged();
return this;
}
/**
*
* A version number in MAJOR.MINOR.PATCH format where the MINOR and PATCH
* fields are optional and the fields may have leading zeros (so 01.00.00 is
* the same as 1.0.0 or 1).
*
*
* string version = 4;
* @param value The bytes for version to set.
* @return This builder for chaining.
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
version_ = value;
onChanged();
return this;
}
private java.lang.Object lastChange_ = "";
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @return The lastChange.
*/
public java.lang.String getLastChange() {
java.lang.Object ref = lastChange_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
lastChange_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @return The bytes for lastChange.
*/
public com.google.protobuf.ByteString
getLastChangeBytes() {
java.lang.Object ref = lastChange_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
lastChange_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @param value The lastChange to set.
* @return This builder for chaining.
*/
public Builder setLastChange(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
lastChange_ = value;
onChanged();
return this;
}
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @return This builder for chaining.
*/
public Builder clearLastChange() {
lastChange_ = getDefaultInstance().getLastChange();
onChanged();
return this;
}
/**
*
* The timestamp when the entity was changed the last time.
*
*
* string last_change = 5;
* @param value The bytes for lastChange to set.
* @return This builder for chaining.
*/
public Builder setLastChangeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
lastChange_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @return The type.
*/
@java.lang.Override
public org.openlca.proto.ProtoType getType() {
@SuppressWarnings("deprecation")
org.openlca.proto.ProtoType result = org.openlca.proto.ProtoType.valueOf(type_);
return result == null ? org.openlca.proto.ProtoType.UNRECOGNIZED : result;
}
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.openlca.proto.ProtoType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The type name of the respective entity.
*
*
* .protolca.ProtoType type = 6 [json_name = "@type"];
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private org.openlca.proto.ProtoRef category_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> categoryBuilder_;
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
* @return Whether the category field is set.
*/
public boolean hasCategory() {
return categoryBuilder_ != null || category_ != null;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
* @return The category.
*/
public org.openlca.proto.ProtoRef getCategory() {
if (categoryBuilder_ == null) {
return category_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : category_;
} else {
return categoryBuilder_.getMessage();
}
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
public Builder setCategory(org.openlca.proto.ProtoRef value) {
if (categoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
category_ = value;
onChanged();
} else {
categoryBuilder_.setMessage(value);
}
return this;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
public Builder setCategory(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (categoryBuilder_ == null) {
category_ = builderForValue.build();
onChanged();
} else {
categoryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
public Builder mergeCategory(org.openlca.proto.ProtoRef value) {
if (categoryBuilder_ == null) {
if (category_ != null) {
category_ =
org.openlca.proto.ProtoRef.newBuilder(category_).mergeFrom(value).buildPartial();
} else {
category_ = value;
}
onChanged();
} else {
categoryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
public Builder clearCategory() {
if (categoryBuilder_ == null) {
category_ = null;
onChanged();
} else {
category_ = null;
categoryBuilder_ = null;
}
return this;
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
public org.openlca.proto.ProtoRef.Builder getCategoryBuilder() {
onChanged();
return getCategoryFieldBuilder().getBuilder();
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
public org.openlca.proto.ProtoRefOrBuilder getCategoryOrBuilder() {
if (categoryBuilder_ != null) {
return categoryBuilder_.getMessageOrBuilder();
} else {
return category_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : category_;
}
}
/**
*
* The category of the entity.
*
*
* .protolca.ProtoRef category = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getCategoryFieldBuilder() {
if (categoryBuilder_ == null) {
categoryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getCategory(),
getParentForChildren(),
isClean());
category_ = null;
}
return categoryBuilder_;
}
private com.google.protobuf.LazyStringList tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTagsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tags_ = new com.google.protobuf.LazyStringArrayList(tags_);
bitField0_ |= 0x00000001;
}
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @return A list containing the tags.
*/
public com.google.protobuf.ProtocolStringList
getTagsList() {
return tags_.getUnmodifiableView();
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @return The count of tags.
*/
public int getTagsCount() {
return tags_.size();
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param index The index of the element to return.
* @return The tags at the given index.
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param index The index of the value to return.
* @return The bytes of the tags at the given index.
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param index The index to set the value at.
* @param value The tags to set.
* @return This builder for chaining.
*/
public Builder setTags(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.set(index, value);
onChanged();
return this;
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param value The tags to add.
* @return This builder for chaining.
*/
public Builder addTags(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param values The tags to add.
* @return This builder for chaining.
*/
public Builder addAllTags(
java.lang.Iterable values) {
ensureTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tags_);
onChanged();
return this;
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @return This builder for chaining.
*/
public Builder clearTags() {
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* A list of optional tags. A tag is just a string which should not contain
* commas (and other special characters).
*
*
* repeated string tags = 8;
* @param value The bytes of the tags to add.
* @return This builder for chaining.
*/
public Builder addTagsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
private java.lang.Object library_ = "";
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @return The library.
*/
public java.lang.String getLibrary() {
java.lang.Object ref = library_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
library_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @return The bytes for library.
*/
public com.google.protobuf.ByteString
getLibraryBytes() {
java.lang.Object ref = library_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
library_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @param value The library to set.
* @return This builder for chaining.
*/
public Builder setLibrary(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
library_ = value;
onChanged();
return this;
}
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @return This builder for chaining.
*/
public Builder clearLibrary() {
library_ = getDefaultInstance().getLibrary();
onChanged();
return this;
}
/**
*
* If this entity is part of a library, this field contains the identifier of
* that library. The identifier is typically just the combination of the
* library name and version.
*
*
* string library = 9;
* @param value The bytes for library to set.
* @return This builder for chaining.
*/
public Builder setLibraryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
library_ = value;
onChanged();
return this;
}
private org.openlca.proto.ProtoRef impactMethod_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder> impactMethodBuilder_;
/**
* .protolca.ProtoRef impact_method = 10;
* @return Whether the impactMethod field is set.
*/
public boolean hasImpactMethod() {
return impactMethodBuilder_ != null || impactMethod_ != null;
}
/**
* .protolca.ProtoRef impact_method = 10;
* @return The impactMethod.
*/
public org.openlca.proto.ProtoRef getImpactMethod() {
if (impactMethodBuilder_ == null) {
return impactMethod_ == null ? org.openlca.proto.ProtoRef.getDefaultInstance() : impactMethod_;
} else {
return impactMethodBuilder_.getMessage();
}
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
public Builder setImpactMethod(org.openlca.proto.ProtoRef value) {
if (impactMethodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
impactMethod_ = value;
onChanged();
} else {
impactMethodBuilder_.setMessage(value);
}
return this;
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
public Builder setImpactMethod(
org.openlca.proto.ProtoRef.Builder builderForValue) {
if (impactMethodBuilder_ == null) {
impactMethod_ = builderForValue.build();
onChanged();
} else {
impactMethodBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
public Builder mergeImpactMethod(org.openlca.proto.ProtoRef value) {
if (impactMethodBuilder_ == null) {
if (impactMethod_ != null) {
impactMethod_ =
org.openlca.proto.ProtoRef.newBuilder(impactMethod_).mergeFrom(value).buildPartial();
} else {
impactMethod_ = value;
}
onChanged();
} else {
impactMethodBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
public Builder clearImpactMethod() {
if (impactMethodBuilder_ == null) {
impactMethod_ = null;
onChanged();
} else {
impactMethod_ = null;
impactMethodBuilder_ = null;
}
return this;
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
public org.openlca.proto.ProtoRef.Builder getImpactMethodBuilder() {
onChanged();
return getImpactMethodFieldBuilder().getBuilder();
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
public org.openlca.proto.ProtoRefOrBuilder getImpactMethodOrBuilder() {
if (impactMethodBuilder_ != null) {
return impactMethodBuilder_.getMessageOrBuilder();
} else {
return impactMethod_ == null ?
org.openlca.proto.ProtoRef.getDefaultInstance() : impactMethod_;
}
}
/**
* .protolca.ProtoRef impact_method = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>
getImpactMethodFieldBuilder() {
if (impactMethodBuilder_ == null) {
impactMethodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoRef, org.openlca.proto.ProtoRef.Builder, org.openlca.proto.ProtoRefOrBuilder>(
getImpactMethod(),
getParentForChildren(),
isClean());
impactMethod_ = null;
}
return impactMethodBuilder_;
}
private org.openlca.proto.ProtoNwSet nwSet_;
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoNwSet, org.openlca.proto.ProtoNwSet.Builder, org.openlca.proto.ProtoNwSetOrBuilder> nwSetBuilder_;
/**
* .protolca.ProtoNwSet nw_set = 11;
* @return Whether the nwSet field is set.
*/
public boolean hasNwSet() {
return nwSetBuilder_ != null || nwSet_ != null;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
* @return The nwSet.
*/
public org.openlca.proto.ProtoNwSet getNwSet() {
if (nwSetBuilder_ == null) {
return nwSet_ == null ? org.openlca.proto.ProtoNwSet.getDefaultInstance() : nwSet_;
} else {
return nwSetBuilder_.getMessage();
}
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
public Builder setNwSet(org.openlca.proto.ProtoNwSet value) {
if (nwSetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nwSet_ = value;
onChanged();
} else {
nwSetBuilder_.setMessage(value);
}
return this;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
public Builder setNwSet(
org.openlca.proto.ProtoNwSet.Builder builderForValue) {
if (nwSetBuilder_ == null) {
nwSet_ = builderForValue.build();
onChanged();
} else {
nwSetBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
public Builder mergeNwSet(org.openlca.proto.ProtoNwSet value) {
if (nwSetBuilder_ == null) {
if (nwSet_ != null) {
nwSet_ =
org.openlca.proto.ProtoNwSet.newBuilder(nwSet_).mergeFrom(value).buildPartial();
} else {
nwSet_ = value;
}
onChanged();
} else {
nwSetBuilder_.mergeFrom(value);
}
return this;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
public Builder clearNwSet() {
if (nwSetBuilder_ == null) {
nwSet_ = null;
onChanged();
} else {
nwSet_ = null;
nwSetBuilder_ = null;
}
return this;
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
public org.openlca.proto.ProtoNwSet.Builder getNwSetBuilder() {
onChanged();
return getNwSetFieldBuilder().getBuilder();
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
public org.openlca.proto.ProtoNwSetOrBuilder getNwSetOrBuilder() {
if (nwSetBuilder_ != null) {
return nwSetBuilder_.getMessageOrBuilder();
} else {
return nwSet_ == null ?
org.openlca.proto.ProtoNwSet.getDefaultInstance() : nwSet_;
}
}
/**
* .protolca.ProtoNwSet nw_set = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoNwSet, org.openlca.proto.ProtoNwSet.Builder, org.openlca.proto.ProtoNwSetOrBuilder>
getNwSetFieldBuilder() {
if (nwSetBuilder_ == null) {
nwSetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.openlca.proto.ProtoNwSet, org.openlca.proto.ProtoNwSet.Builder, org.openlca.proto.ProtoNwSetOrBuilder>(
getNwSet(),
getParentForChildren(),
isClean());
nwSet_ = null;
}
return nwSetBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protolca.ProtoProject)
}
// @@protoc_insertion_point(class_scope:protolca.ProtoProject)
private static final org.openlca.proto.ProtoProject DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openlca.proto.ProtoProject();
}
public static org.openlca.proto.ProtoProject getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProtoProject parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProtoProject(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openlca.proto.ProtoProject getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy