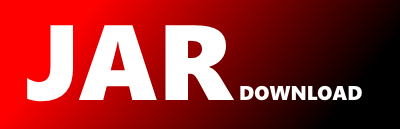
org.openlca.proto.ProtoUncertainty Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: olca.proto
package org.openlca.proto;
/**
*
* Defines the parameter values of an uncertainty distribution. Depending on
* the uncertainty distribution type different parameters could be used.
*
*
* Protobuf type {@code protolca.ProtoUncertainty}
*/
public final class ProtoUncertainty extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:protolca.ProtoUncertainty)
ProtoUncertaintyOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProtoUncertainty.newBuilder() to construct.
private ProtoUncertainty(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProtoUncertainty() {
distributionType_ = 0;
meanFormula_ = "";
geomMeanFormula_ = "";
minimumFormula_ = "";
sdFormula_ = "";
geomSdFormula_ = "";
modeFormula_ = "";
maximumFormula_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ProtoUncertainty();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProtoUncertainty(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
distributionType_ = rawValue;
break;
}
case 17: {
mean_ = input.readDouble();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
meanFormula_ = s;
break;
}
case 33: {
geomMean_ = input.readDouble();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
geomMeanFormula_ = s;
break;
}
case 49: {
minimum_ = input.readDouble();
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
minimumFormula_ = s;
break;
}
case 65: {
sd_ = input.readDouble();
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
sdFormula_ = s;
break;
}
case 81: {
geomSd_ = input.readDouble();
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
geomSdFormula_ = s;
break;
}
case 97: {
mode_ = input.readDouble();
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
modeFormula_ = s;
break;
}
case 113: {
maximum_ = input.readDouble();
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
maximumFormula_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoUncertainty_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoUncertainty_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openlca.proto.ProtoUncertainty.class, org.openlca.proto.ProtoUncertainty.Builder.class);
}
public static final int DISTRIBUTION_TYPE_FIELD_NUMBER = 1;
private int distributionType_;
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @return The enum numeric value on the wire for distributionType.
*/
@java.lang.Override public int getDistributionTypeValue() {
return distributionType_;
}
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @return The distributionType.
*/
@java.lang.Override public org.openlca.proto.ProtoUncertaintyType getDistributionType() {
@SuppressWarnings("deprecation")
org.openlca.proto.ProtoUncertaintyType result = org.openlca.proto.ProtoUncertaintyType.valueOf(distributionType_);
return result == null ? org.openlca.proto.ProtoUncertaintyType.UNRECOGNIZED : result;
}
public static final int MEAN_FIELD_NUMBER = 2;
private double mean_;
/**
*
* The arithmetic mean (used for normal distributions).
*
*
* double mean = 2;
* @return The mean.
*/
@java.lang.Override
public double getMean() {
return mean_;
}
public static final int MEAN_FORMULA_FIELD_NUMBER = 3;
private volatile java.lang.Object meanFormula_;
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @return The meanFormula.
*/
@java.lang.Override
public java.lang.String getMeanFormula() {
java.lang.Object ref = meanFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
meanFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @return The bytes for meanFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMeanFormulaBytes() {
java.lang.Object ref = meanFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
meanFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GEOM_MEAN_FIELD_NUMBER = 4;
private double geomMean_;
/**
*
* The geometric mean value (used for log-normal distributions).
*
*
* double geom_mean = 4;
* @return The geomMean.
*/
@java.lang.Override
public double getGeomMean() {
return geomMean_;
}
public static final int GEOM_MEAN_FORMULA_FIELD_NUMBER = 5;
private volatile java.lang.Object geomMeanFormula_;
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @return The geomMeanFormula.
*/
@java.lang.Override
public java.lang.String getGeomMeanFormula() {
java.lang.Object ref = geomMeanFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
geomMeanFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @return The bytes for geomMeanFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getGeomMeanFormulaBytes() {
java.lang.Object ref = geomMeanFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
geomMeanFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MINIMUM_FIELD_NUMBER = 6;
private double minimum_;
/**
*
* The minimum value (used for uniform and triangle distributions).
*
*
* double minimum = 6;
* @return The minimum.
*/
@java.lang.Override
public double getMinimum() {
return minimum_;
}
public static final int MINIMUM_FORMULA_FIELD_NUMBER = 7;
private volatile java.lang.Object minimumFormula_;
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @return The minimumFormula.
*/
@java.lang.Override
public java.lang.String getMinimumFormula() {
java.lang.Object ref = minimumFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
minimumFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @return The bytes for minimumFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMinimumFormulaBytes() {
java.lang.Object ref = minimumFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
minimumFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SD_FIELD_NUMBER = 8;
private double sd_;
/**
*
* The arithmetic standard deviation (used for normal distributions).
*
*
* double sd = 8;
* @return The sd.
*/
@java.lang.Override
public double getSd() {
return sd_;
}
public static final int SD_FORMULA_FIELD_NUMBER = 9;
private volatile java.lang.Object sdFormula_;
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @return The sdFormula.
*/
@java.lang.Override
public java.lang.String getSdFormula() {
java.lang.Object ref = sdFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sdFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @return The bytes for sdFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSdFormulaBytes() {
java.lang.Object ref = sdFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sdFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GEOM_SD_FIELD_NUMBER = 10;
private double geomSd_;
/**
*
* The geometric standard deviation (used for log-normal distributions).
*
*
* double geom_sd = 10;
* @return The geomSd.
*/
@java.lang.Override
public double getGeomSd() {
return geomSd_;
}
public static final int GEOM_SD_FORMULA_FIELD_NUMBER = 11;
private volatile java.lang.Object geomSdFormula_;
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @return The geomSdFormula.
*/
@java.lang.Override
public java.lang.String getGeomSdFormula() {
java.lang.Object ref = geomSdFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
geomSdFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @return The bytes for geomSdFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getGeomSdFormulaBytes() {
java.lang.Object ref = geomSdFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
geomSdFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MODE_FIELD_NUMBER = 12;
private double mode_;
/**
*
* The most likely value (used for triangle distributions).
*
*
* double mode = 12;
* @return The mode.
*/
@java.lang.Override
public double getMode() {
return mode_;
}
public static final int MODE_FORMULA_FIELD_NUMBER = 13;
private volatile java.lang.Object modeFormula_;
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @return The modeFormula.
*/
@java.lang.Override
public java.lang.String getModeFormula() {
java.lang.Object ref = modeFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
modeFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @return The bytes for modeFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getModeFormulaBytes() {
java.lang.Object ref = modeFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
modeFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAXIMUM_FIELD_NUMBER = 14;
private double maximum_;
/**
*
* The maximum value (used for uniform and triangle distributions).
*
*
* double maximum = 14;
* @return The maximum.
*/
@java.lang.Override
public double getMaximum() {
return maximum_;
}
public static final int MAXIMUM_FORMULA_FIELD_NUMBER = 15;
private volatile java.lang.Object maximumFormula_;
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @return The maximumFormula.
*/
@java.lang.Override
public java.lang.String getMaximumFormula() {
java.lang.Object ref = maximumFormula_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
maximumFormula_ = s;
return s;
}
}
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @return The bytes for maximumFormula.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMaximumFormulaBytes() {
java.lang.Object ref = maximumFormula_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
maximumFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (distributionType_ != org.openlca.proto.ProtoUncertaintyType.UNDEFINED_UNCERTAINTY_TYPE.getNumber()) {
output.writeEnum(1, distributionType_);
}
if (mean_ != 0D) {
output.writeDouble(2, mean_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(meanFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, meanFormula_);
}
if (geomMean_ != 0D) {
output.writeDouble(4, geomMean_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(geomMeanFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, geomMeanFormula_);
}
if (minimum_ != 0D) {
output.writeDouble(6, minimum_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(minimumFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, minimumFormula_);
}
if (sd_ != 0D) {
output.writeDouble(8, sd_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sdFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, sdFormula_);
}
if (geomSd_ != 0D) {
output.writeDouble(10, geomSd_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(geomSdFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, geomSdFormula_);
}
if (mode_ != 0D) {
output.writeDouble(12, mode_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(modeFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, modeFormula_);
}
if (maximum_ != 0D) {
output.writeDouble(14, maximum_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(maximumFormula_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, maximumFormula_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (distributionType_ != org.openlca.proto.ProtoUncertaintyType.UNDEFINED_UNCERTAINTY_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, distributionType_);
}
if (mean_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, mean_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(meanFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, meanFormula_);
}
if (geomMean_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, geomMean_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(geomMeanFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, geomMeanFormula_);
}
if (minimum_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(6, minimum_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(minimumFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, minimumFormula_);
}
if (sd_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(8, sd_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sdFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, sdFormula_);
}
if (geomSd_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(10, geomSd_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(geomSdFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, geomSdFormula_);
}
if (mode_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(12, mode_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(modeFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, modeFormula_);
}
if (maximum_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(14, maximum_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(maximumFormula_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, maximumFormula_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openlca.proto.ProtoUncertainty)) {
return super.equals(obj);
}
org.openlca.proto.ProtoUncertainty other = (org.openlca.proto.ProtoUncertainty) obj;
if (distributionType_ != other.distributionType_) return false;
if (java.lang.Double.doubleToLongBits(getMean())
!= java.lang.Double.doubleToLongBits(
other.getMean())) return false;
if (!getMeanFormula()
.equals(other.getMeanFormula())) return false;
if (java.lang.Double.doubleToLongBits(getGeomMean())
!= java.lang.Double.doubleToLongBits(
other.getGeomMean())) return false;
if (!getGeomMeanFormula()
.equals(other.getGeomMeanFormula())) return false;
if (java.lang.Double.doubleToLongBits(getMinimum())
!= java.lang.Double.doubleToLongBits(
other.getMinimum())) return false;
if (!getMinimumFormula()
.equals(other.getMinimumFormula())) return false;
if (java.lang.Double.doubleToLongBits(getSd())
!= java.lang.Double.doubleToLongBits(
other.getSd())) return false;
if (!getSdFormula()
.equals(other.getSdFormula())) return false;
if (java.lang.Double.doubleToLongBits(getGeomSd())
!= java.lang.Double.doubleToLongBits(
other.getGeomSd())) return false;
if (!getGeomSdFormula()
.equals(other.getGeomSdFormula())) return false;
if (java.lang.Double.doubleToLongBits(getMode())
!= java.lang.Double.doubleToLongBits(
other.getMode())) return false;
if (!getModeFormula()
.equals(other.getModeFormula())) return false;
if (java.lang.Double.doubleToLongBits(getMaximum())
!= java.lang.Double.doubleToLongBits(
other.getMaximum())) return false;
if (!getMaximumFormula()
.equals(other.getMaximumFormula())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DISTRIBUTION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + distributionType_;
hash = (37 * hash) + MEAN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getMean()));
hash = (37 * hash) + MEAN_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getMeanFormula().hashCode();
hash = (37 * hash) + GEOM_MEAN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getGeomMean()));
hash = (37 * hash) + GEOM_MEAN_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getGeomMeanFormula().hashCode();
hash = (37 * hash) + MINIMUM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getMinimum()));
hash = (37 * hash) + MINIMUM_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getMinimumFormula().hashCode();
hash = (37 * hash) + SD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSd()));
hash = (37 * hash) + SD_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getSdFormula().hashCode();
hash = (37 * hash) + GEOM_SD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getGeomSd()));
hash = (37 * hash) + GEOM_SD_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getGeomSdFormula().hashCode();
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getMode()));
hash = (37 * hash) + MODE_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getModeFormula().hashCode();
hash = (37 * hash) + MAXIMUM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getMaximum()));
hash = (37 * hash) + MAXIMUM_FORMULA_FIELD_NUMBER;
hash = (53 * hash) + getMaximumFormula().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openlca.proto.ProtoUncertainty parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoUncertainty parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.openlca.proto.ProtoUncertainty parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openlca.proto.ProtoUncertainty prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Defines the parameter values of an uncertainty distribution. Depending on
* the uncertainty distribution type different parameters could be used.
*
*
* Protobuf type {@code protolca.ProtoUncertainty}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:protolca.ProtoUncertainty)
org.openlca.proto.ProtoUncertaintyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoUncertainty_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoUncertainty_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openlca.proto.ProtoUncertainty.class, org.openlca.proto.ProtoUncertainty.Builder.class);
}
// Construct using org.openlca.proto.ProtoUncertainty.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
distributionType_ = 0;
mean_ = 0D;
meanFormula_ = "";
geomMean_ = 0D;
geomMeanFormula_ = "";
minimum_ = 0D;
minimumFormula_ = "";
sd_ = 0D;
sdFormula_ = "";
geomSd_ = 0D;
geomSdFormula_ = "";
mode_ = 0D;
modeFormula_ = "";
maximum_ = 0D;
maximumFormula_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openlca.proto.Proto.internal_static_protolca_ProtoUncertainty_descriptor;
}
@java.lang.Override
public org.openlca.proto.ProtoUncertainty getDefaultInstanceForType() {
return org.openlca.proto.ProtoUncertainty.getDefaultInstance();
}
@java.lang.Override
public org.openlca.proto.ProtoUncertainty build() {
org.openlca.proto.ProtoUncertainty result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openlca.proto.ProtoUncertainty buildPartial() {
org.openlca.proto.ProtoUncertainty result = new org.openlca.proto.ProtoUncertainty(this);
result.distributionType_ = distributionType_;
result.mean_ = mean_;
result.meanFormula_ = meanFormula_;
result.geomMean_ = geomMean_;
result.geomMeanFormula_ = geomMeanFormula_;
result.minimum_ = minimum_;
result.minimumFormula_ = minimumFormula_;
result.sd_ = sd_;
result.sdFormula_ = sdFormula_;
result.geomSd_ = geomSd_;
result.geomSdFormula_ = geomSdFormula_;
result.mode_ = mode_;
result.modeFormula_ = modeFormula_;
result.maximum_ = maximum_;
result.maximumFormula_ = maximumFormula_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openlca.proto.ProtoUncertainty) {
return mergeFrom((org.openlca.proto.ProtoUncertainty)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openlca.proto.ProtoUncertainty other) {
if (other == org.openlca.proto.ProtoUncertainty.getDefaultInstance()) return this;
if (other.distributionType_ != 0) {
setDistributionTypeValue(other.getDistributionTypeValue());
}
if (other.getMean() != 0D) {
setMean(other.getMean());
}
if (!other.getMeanFormula().isEmpty()) {
meanFormula_ = other.meanFormula_;
onChanged();
}
if (other.getGeomMean() != 0D) {
setGeomMean(other.getGeomMean());
}
if (!other.getGeomMeanFormula().isEmpty()) {
geomMeanFormula_ = other.geomMeanFormula_;
onChanged();
}
if (other.getMinimum() != 0D) {
setMinimum(other.getMinimum());
}
if (!other.getMinimumFormula().isEmpty()) {
minimumFormula_ = other.minimumFormula_;
onChanged();
}
if (other.getSd() != 0D) {
setSd(other.getSd());
}
if (!other.getSdFormula().isEmpty()) {
sdFormula_ = other.sdFormula_;
onChanged();
}
if (other.getGeomSd() != 0D) {
setGeomSd(other.getGeomSd());
}
if (!other.getGeomSdFormula().isEmpty()) {
geomSdFormula_ = other.geomSdFormula_;
onChanged();
}
if (other.getMode() != 0D) {
setMode(other.getMode());
}
if (!other.getModeFormula().isEmpty()) {
modeFormula_ = other.modeFormula_;
onChanged();
}
if (other.getMaximum() != 0D) {
setMaximum(other.getMaximum());
}
if (!other.getMaximumFormula().isEmpty()) {
maximumFormula_ = other.maximumFormula_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.openlca.proto.ProtoUncertainty parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.openlca.proto.ProtoUncertainty) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int distributionType_ = 0;
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @return The enum numeric value on the wire for distributionType.
*/
@java.lang.Override public int getDistributionTypeValue() {
return distributionType_;
}
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @param value The enum numeric value on the wire for distributionType to set.
* @return This builder for chaining.
*/
public Builder setDistributionTypeValue(int value) {
distributionType_ = value;
onChanged();
return this;
}
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @return The distributionType.
*/
@java.lang.Override
public org.openlca.proto.ProtoUncertaintyType getDistributionType() {
@SuppressWarnings("deprecation")
org.openlca.proto.ProtoUncertaintyType result = org.openlca.proto.ProtoUncertaintyType.valueOf(distributionType_);
return result == null ? org.openlca.proto.ProtoUncertaintyType.UNRECOGNIZED : result;
}
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @param value The distributionType to set.
* @return This builder for chaining.
*/
public Builder setDistributionType(org.openlca.proto.ProtoUncertaintyType value) {
if (value == null) {
throw new NullPointerException();
}
distributionType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The uncertainty distribution type
*
*
* .protolca.ProtoUncertaintyType distribution_type = 1;
* @return This builder for chaining.
*/
public Builder clearDistributionType() {
distributionType_ = 0;
onChanged();
return this;
}
private double mean_ ;
/**
*
* The arithmetic mean (used for normal distributions).
*
*
* double mean = 2;
* @return The mean.
*/
@java.lang.Override
public double getMean() {
return mean_;
}
/**
*
* The arithmetic mean (used for normal distributions).
*
*
* double mean = 2;
* @param value The mean to set.
* @return This builder for chaining.
*/
public Builder setMean(double value) {
mean_ = value;
onChanged();
return this;
}
/**
*
* The arithmetic mean (used for normal distributions).
*
*
* double mean = 2;
* @return This builder for chaining.
*/
public Builder clearMean() {
mean_ = 0D;
onChanged();
return this;
}
private java.lang.Object meanFormula_ = "";
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @return The meanFormula.
*/
public java.lang.String getMeanFormula() {
java.lang.Object ref = meanFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
meanFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @return The bytes for meanFormula.
*/
public com.google.protobuf.ByteString
getMeanFormulaBytes() {
java.lang.Object ref = meanFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
meanFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @param value The meanFormula to set.
* @return This builder for chaining.
*/
public Builder setMeanFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
meanFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @return This builder for chaining.
*/
public Builder clearMeanFormula() {
meanFormula_ = getDefaultInstance().getMeanFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the arithmetic mean.
*
*
* string mean_formula = 3;
* @param value The bytes for meanFormula to set.
* @return This builder for chaining.
*/
public Builder setMeanFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
meanFormula_ = value;
onChanged();
return this;
}
private double geomMean_ ;
/**
*
* The geometric mean value (used for log-normal distributions).
*
*
* double geom_mean = 4;
* @return The geomMean.
*/
@java.lang.Override
public double getGeomMean() {
return geomMean_;
}
/**
*
* The geometric mean value (used for log-normal distributions).
*
*
* double geom_mean = 4;
* @param value The geomMean to set.
* @return This builder for chaining.
*/
public Builder setGeomMean(double value) {
geomMean_ = value;
onChanged();
return this;
}
/**
*
* The geometric mean value (used for log-normal distributions).
*
*
* double geom_mean = 4;
* @return This builder for chaining.
*/
public Builder clearGeomMean() {
geomMean_ = 0D;
onChanged();
return this;
}
private java.lang.Object geomMeanFormula_ = "";
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @return The geomMeanFormula.
*/
public java.lang.String getGeomMeanFormula() {
java.lang.Object ref = geomMeanFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
geomMeanFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @return The bytes for geomMeanFormula.
*/
public com.google.protobuf.ByteString
getGeomMeanFormulaBytes() {
java.lang.Object ref = geomMeanFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
geomMeanFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @param value The geomMeanFormula to set.
* @return This builder for chaining.
*/
public Builder setGeomMeanFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
geomMeanFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @return This builder for chaining.
*/
public Builder clearGeomMeanFormula() {
geomMeanFormula_ = getDefaultInstance().getGeomMeanFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the geometric mean.
*
*
* string geom_mean_formula = 5;
* @param value The bytes for geomMeanFormula to set.
* @return This builder for chaining.
*/
public Builder setGeomMeanFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
geomMeanFormula_ = value;
onChanged();
return this;
}
private double minimum_ ;
/**
*
* The minimum value (used for uniform and triangle distributions).
*
*
* double minimum = 6;
* @return The minimum.
*/
@java.lang.Override
public double getMinimum() {
return minimum_;
}
/**
*
* The minimum value (used for uniform and triangle distributions).
*
*
* double minimum = 6;
* @param value The minimum to set.
* @return This builder for chaining.
*/
public Builder setMinimum(double value) {
minimum_ = value;
onChanged();
return this;
}
/**
*
* The minimum value (used for uniform and triangle distributions).
*
*
* double minimum = 6;
* @return This builder for chaining.
*/
public Builder clearMinimum() {
minimum_ = 0D;
onChanged();
return this;
}
private java.lang.Object minimumFormula_ = "";
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @return The minimumFormula.
*/
public java.lang.String getMinimumFormula() {
java.lang.Object ref = minimumFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
minimumFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @return The bytes for minimumFormula.
*/
public com.google.protobuf.ByteString
getMinimumFormulaBytes() {
java.lang.Object ref = minimumFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
minimumFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @param value The minimumFormula to set.
* @return This builder for chaining.
*/
public Builder setMinimumFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
minimumFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @return This builder for chaining.
*/
public Builder clearMinimumFormula() {
minimumFormula_ = getDefaultInstance().getMinimumFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the minimum value.
*
*
* string minimum_formula = 7;
* @param value The bytes for minimumFormula to set.
* @return This builder for chaining.
*/
public Builder setMinimumFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
minimumFormula_ = value;
onChanged();
return this;
}
private double sd_ ;
/**
*
* The arithmetic standard deviation (used for normal distributions).
*
*
* double sd = 8;
* @return The sd.
*/
@java.lang.Override
public double getSd() {
return sd_;
}
/**
*
* The arithmetic standard deviation (used for normal distributions).
*
*
* double sd = 8;
* @param value The sd to set.
* @return This builder for chaining.
*/
public Builder setSd(double value) {
sd_ = value;
onChanged();
return this;
}
/**
*
* The arithmetic standard deviation (used for normal distributions).
*
*
* double sd = 8;
* @return This builder for chaining.
*/
public Builder clearSd() {
sd_ = 0D;
onChanged();
return this;
}
private java.lang.Object sdFormula_ = "";
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @return The sdFormula.
*/
public java.lang.String getSdFormula() {
java.lang.Object ref = sdFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sdFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @return The bytes for sdFormula.
*/
public com.google.protobuf.ByteString
getSdFormulaBytes() {
java.lang.Object ref = sdFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sdFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @param value The sdFormula to set.
* @return This builder for chaining.
*/
public Builder setSdFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sdFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @return This builder for chaining.
*/
public Builder clearSdFormula() {
sdFormula_ = getDefaultInstance().getSdFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the arithmetic standard deviation.
*
*
* string sd_formula = 9;
* @param value The bytes for sdFormula to set.
* @return This builder for chaining.
*/
public Builder setSdFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sdFormula_ = value;
onChanged();
return this;
}
private double geomSd_ ;
/**
*
* The geometric standard deviation (used for log-normal distributions).
*
*
* double geom_sd = 10;
* @return The geomSd.
*/
@java.lang.Override
public double getGeomSd() {
return geomSd_;
}
/**
*
* The geometric standard deviation (used for log-normal distributions).
*
*
* double geom_sd = 10;
* @param value The geomSd to set.
* @return This builder for chaining.
*/
public Builder setGeomSd(double value) {
geomSd_ = value;
onChanged();
return this;
}
/**
*
* The geometric standard deviation (used for log-normal distributions).
*
*
* double geom_sd = 10;
* @return This builder for chaining.
*/
public Builder clearGeomSd() {
geomSd_ = 0D;
onChanged();
return this;
}
private java.lang.Object geomSdFormula_ = "";
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @return The geomSdFormula.
*/
public java.lang.String getGeomSdFormula() {
java.lang.Object ref = geomSdFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
geomSdFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @return The bytes for geomSdFormula.
*/
public com.google.protobuf.ByteString
getGeomSdFormulaBytes() {
java.lang.Object ref = geomSdFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
geomSdFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @param value The geomSdFormula to set.
* @return This builder for chaining.
*/
public Builder setGeomSdFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
geomSdFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @return This builder for chaining.
*/
public Builder clearGeomSdFormula() {
geomSdFormula_ = getDefaultInstance().getGeomSdFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the geometric standard deviation.
*
*
* string geom_sd_formula = 11;
* @param value The bytes for geomSdFormula to set.
* @return This builder for chaining.
*/
public Builder setGeomSdFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
geomSdFormula_ = value;
onChanged();
return this;
}
private double mode_ ;
/**
*
* The most likely value (used for triangle distributions).
*
*
* double mode = 12;
* @return The mode.
*/
@java.lang.Override
public double getMode() {
return mode_;
}
/**
*
* The most likely value (used for triangle distributions).
*
*
* double mode = 12;
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(double value) {
mode_ = value;
onChanged();
return this;
}
/**
*
* The most likely value (used for triangle distributions).
*
*
* double mode = 12;
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = 0D;
onChanged();
return this;
}
private java.lang.Object modeFormula_ = "";
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @return The modeFormula.
*/
public java.lang.String getModeFormula() {
java.lang.Object ref = modeFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
modeFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @return The bytes for modeFormula.
*/
public com.google.protobuf.ByteString
getModeFormulaBytes() {
java.lang.Object ref = modeFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
modeFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @param value The modeFormula to set.
* @return This builder for chaining.
*/
public Builder setModeFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
modeFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @return This builder for chaining.
*/
public Builder clearModeFormula() {
modeFormula_ = getDefaultInstance().getModeFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the most likely value.
*
*
* string mode_formula = 13;
* @param value The bytes for modeFormula to set.
* @return This builder for chaining.
*/
public Builder setModeFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
modeFormula_ = value;
onChanged();
return this;
}
private double maximum_ ;
/**
*
* The maximum value (used for uniform and triangle distributions).
*
*
* double maximum = 14;
* @return The maximum.
*/
@java.lang.Override
public double getMaximum() {
return maximum_;
}
/**
*
* The maximum value (used for uniform and triangle distributions).
*
*
* double maximum = 14;
* @param value The maximum to set.
* @return This builder for chaining.
*/
public Builder setMaximum(double value) {
maximum_ = value;
onChanged();
return this;
}
/**
*
* The maximum value (used for uniform and triangle distributions).
*
*
* double maximum = 14;
* @return This builder for chaining.
*/
public Builder clearMaximum() {
maximum_ = 0D;
onChanged();
return this;
}
private java.lang.Object maximumFormula_ = "";
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @return The maximumFormula.
*/
public java.lang.String getMaximumFormula() {
java.lang.Object ref = maximumFormula_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
maximumFormula_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @return The bytes for maximumFormula.
*/
public com.google.protobuf.ByteString
getMaximumFormulaBytes() {
java.lang.Object ref = maximumFormula_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
maximumFormula_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @param value The maximumFormula to set.
* @return This builder for chaining.
*/
public Builder setMaximumFormula(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
maximumFormula_ = value;
onChanged();
return this;
}
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @return This builder for chaining.
*/
public Builder clearMaximumFormula() {
maximumFormula_ = getDefaultInstance().getMaximumFormula();
onChanged();
return this;
}
/**
*
* A mathematical formula for the maximum value.
*
*
* string maximum_formula = 15;
* @param value The bytes for maximumFormula to set.
* @return This builder for chaining.
*/
public Builder setMaximumFormulaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
maximumFormula_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:protolca.ProtoUncertainty)
}
// @@protoc_insertion_point(class_scope:protolca.ProtoUncertainty)
private static final org.openlca.proto.ProtoUncertainty DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openlca.proto.ProtoUncertainty();
}
public static org.openlca.proto.ProtoUncertainty getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProtoUncertainty parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProtoUncertainty(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openlca.proto.ProtoUncertainty getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy