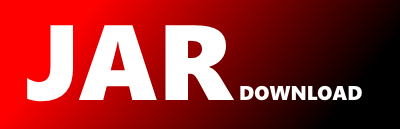
org.openlr.binary.BinaryMarshallerImpl Maven / Gradle / Ivy
package org.openlr.binary;
import org.openlr.binary.reader.LocationReferenceReader;
import org.openlr.binary.writer.LocationReferenceWriter;
import org.openlr.locationreference.LocationReference;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.Base64;
class BinaryMarshallerImpl implements BinaryMarshaller {
private final Base64.Decoder base64Decoder;
private final Base64.Encoder base64Encoder;
private final LocationReferenceReader locationReferenceReader;
private final LocationReferenceWriter locationReferenceWriter;
BinaryMarshallerImpl(Base64.Decoder base64Decoder, Base64.Encoder base64Encoder, LocationReferenceReader locationReferenceReader, LocationReferenceWriter locationReferenceWriter) {
this.base64Decoder = base64Decoder;
this.base64Encoder = base64Encoder;
this.locationReferenceReader = locationReferenceReader;
this.locationReferenceWriter = locationReferenceWriter;
}
@Override
public LocationReference unmarshall(ByteArrayInputStream inputStream) throws BinaryMarshallerException {
try {
return locationReferenceReader.read(inputStream);
}
catch (IOException e) {
throw new BinaryMarshallerException("Unable to unmarshall location reference", e);
}
}
@Override
public T unmarshall(ByteArrayInputStream inputStream, Class type) throws BinaryMarshallerException {
return (T) unmarshall(inputStream);
}
@Override
public LocationReference unmarshall(byte[] bytes) throws BinaryMarshallerException {
try (ByteArrayInputStream inputStream = new ByteArrayInputStream(bytes)){
return unmarshall(inputStream);
}
catch (IOException e) {
throw new BinaryMarshallerException("Unable to unmarshall location reference", e);
}
}
@Override
public T unmarshall(byte[] bytes, Class type) throws BinaryMarshallerException {
return (T) unmarshall(bytes);
}
@Override
public LocationReference unmarshall(String base64String) throws BinaryMarshallerException {
try {
byte[] bytes = base64Decoder.decode(base64String);
return unmarshall(bytes);
}
catch (IllegalArgumentException e) {
throw new BinaryMarshallerException("Unable to unmarshall location reference", e);
}
}
@Override
public T unmarshall(String base64String, Class type) throws BinaryMarshallerException {
return (T) unmarshall(base64String);
}
@Override
public void marshall(LocationReference locationReference, ByteArrayOutputStream outputStream) throws BinaryMarshallerException {
try {
locationReferenceWriter.write(locationReference, outputStream);
}
catch (IOException e) {
throw new BinaryMarshallerException("Unable to marshall location reference", e);
}
}
@Override
public byte[] marshall(LocationReference locationReference) throws BinaryMarshallerException {
try (ByteArrayOutputStream outputStream = new ByteArrayOutputStream()) {
marshall(locationReference, outputStream);
return outputStream.toByteArray();
}
catch (IOException e) {
throw new BinaryMarshallerException("Unable to marshall location reference", e);
}
}
@Override
public String marshallToBase64String(LocationReference locationReference) throws BinaryMarshallerException {
byte[] bytes = marshall(locationReference);
return base64Encoder.encodeToString(bytes);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy