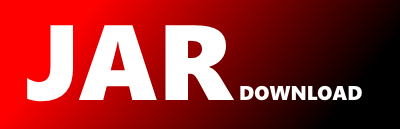
org.openlr.encoder.EncoderImpl Maven / Gradle / Ivy
package org.openlr.encoder;
import org.openlr.location.*;
import org.openlr.locationreference.*;
import org.openlr.map.Line;
class EncoderImpl implements Encoder {
private final PathEncoder pathEncoder;
private final PointAlongLineEncoder pointAlongLineEncoder;
private final LocationReferenceFactory locationReferenceFactory;
public EncoderImpl(PathEncoder pathEncoder, PointAlongLineEncoder pointAlongLineEncoder, LocationReferenceFactory locationReferenceFactory) {
this.pathEncoder = pathEncoder;
this.pointAlongLineEncoder = pointAlongLineEncoder;
this.locationReferenceFactory = locationReferenceFactory;
}
@Override
public LocationReference encode(Location location) {
if (location instanceof LineLocation) {
return encode((LineLocation) location);
}
else if (location instanceof GeoCoordinateLocation) {
return encode((GeoCoordinateLocation) location);
}
else if (location instanceof PointAlongLineLocation) {
return encode((PointAlongLineLocation) location);
}
else if (location instanceof PointOfInterestWithAccessPointLocation) {
return encode((PointOfInterestWithAccessPointLocation) location);
}
else if (location instanceof CircleLocation) {
return encode((CircleLocation) location);
}
else if (location instanceof PolygonLocation) {
return encode((PolygonLocation) location);
}
else if (location instanceof RectangleLocation) {
return encode((RectangleLocation) location);
}
else if (location instanceof GridLocation) {
return encode((GridLocation) location);
}
else if (location instanceof ClosedLineLocation) {
return encode((ClosedLineLocation) location);
}
else {
throw new IllegalArgumentException("Unknown location type");
}
}
@Override
public > LineLocationReference encode(LineLocation lineLocation) {
PathEncoderResult result = pathEncoder.encode(lineLocation.getPath());
return locationReferenceFactory.createLineLocationReference(
result.getLocationReferencePoints(),
result.getRelativePositiveOffset(),
result.getRelativeNegativeOffset());
}
@Override
public GeoCoordinateLocationReference encode(GeoCoordinateLocation geoCoordinateLocation) {
return locationReferenceFactory.createGeoCoordinateLocationReference(geoCoordinateLocation.getCoordinate());
}
@Override
public > PointAlongLineLocationReference encode(PointAlongLineLocation pointAlongLineLocation) {
PointAlongLineEncoderResult result = pointAlongLineEncoder.encode(pointAlongLineLocation.getPointAlongLine());
return locationReferenceFactory.createPointAlongLineLocationReference(
result.getFirstLocationReferencePoint(),
result.getLastLocationReferencePoint(),
result.getRelativePositiveOffset(),
pointAlongLineLocation.getOrientation(),
pointAlongLineLocation.getSideOfRoad());
}
@Override
public > PointOfInterestWithAccessPointLocationReference encode(PointOfInterestWithAccessPointLocation pointOfInterestWithAccessPointLocation) {
PointAlongLineEncoderResult result = pointAlongLineEncoder.encode(pointOfInterestWithAccessPointLocation.getAccessPoint());
return locationReferenceFactory.createPointOfInterestWithAccessPointLocationReference(
result.getFirstLocationReferencePoint(),
result.getLastLocationReferencePoint(),
result.getRelativePositiveOffset(),
pointOfInterestWithAccessPointLocation.getOrientation(),
pointOfInterestWithAccessPointLocation.getSideOfRoad(),
pointOfInterestWithAccessPointLocation.getCoordinate());
}
@Override
public CircleLocationReference encode(CircleLocation circleLocation) {
return locationReferenceFactory.createCircleLocationReference(circleLocation.getCenter(), circleLocation.getRadius());
}
@Override
public PolygonLocationReference encode(PolygonLocation polygonLocation) {
return locationReferenceFactory.createPolygonLocationReference(polygonLocation.getCoordinates());
}
@Override
public RectangleLocationReference encode(RectangleLocation rectangleLocation) {
return locationReferenceFactory.createRectangleLocationReference(rectangleLocation.getLowerLeft(), rectangleLocation.getUpperRight());
}
@Override
public GridLocationReference encode(GridLocation gridLocation) {
return locationReferenceFactory.createGridLocationReference(gridLocation.getLowerLeft(), gridLocation.getUpperRight(), gridLocation.getNumberOfColumns(), gridLocation.getNumberOfRows());
}
@Override
public > ClosedLineLocationReference encode(ClosedLineLocation closedLineLocation) {
PathEncoderResult result = pathEncoder.encode(closedLineLocation.getPath());
return locationReferenceFactory.createClosedLineLocationReference(result.getLocationReferencePoints());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy