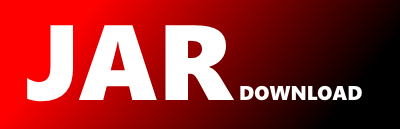
org.openlr.map.PathImpl Maven / Gradle / Ivy
package org.openlr.map;
import lombok.EqualsAndHashCode;
import lombok.ToString;
import org.locationtech.jts.geom.LineString;
import org.openlr.geo.Geo;
import java.util.ArrayList;
import java.util.List;
@EqualsAndHashCode
@ToString
class PathImpl> implements Path {
private final List lines;
private final double positiveOffset;
private final double negativeOffset;
@EqualsAndHashCode.Exclude
@ToString.Exclude
private final Geo geo;
PathImpl(List lines, double positiveOffset, double negativeOffset, Geo geo) {
this.lines = lines;
this.positiveOffset = positiveOffset;
this.negativeOffset = negativeOffset;
this.geo = geo;
}
@Override
public List getLines() {
return lines;
}
@Override
public double getPositiveOffset() {
return positiveOffset;
}
@Override
public double getNegativeOffset() {
return negativeOffset;
}
@Override
public PointAlongLine getStart() {
L line = lines.get(0);
return new PointAlongLineImpl<>(line, positiveOffset, geo);
}
@Override
public PointAlongLine getEnd() {
L line = lines.get(lines.size() - 1);
return new PointAlongLineImpl<>(line, line.getLength() - negativeOffset, geo);
}
@Override
public LineString getGeometry() {
List lineStrings = new ArrayList<>();
double totalLength = 0;
for (L line : lines) {
lineStrings.add(line.getGeometry());
totalLength += line.getLength();
}
LineString totalLineString = geo.joinLineStrings(lineStrings);
double startFraction = positiveOffset / totalLength;
double stopFraction = (totalLength - negativeOffset) / totalLength;
return geo.sliceLineString(totalLineString, startFraction, stopFraction);
}
@Override
public double getLength() {
double totalLength = lines.stream()
.mapToDouble(Line::getLength)
.sum();
return totalLength - positiveOffset - negativeOffset;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy