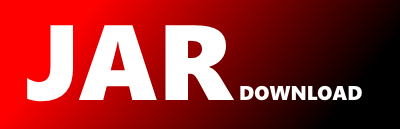
com.wombat.mamda.MamdaQuoteUpdate Maven / Gradle / Ivy
/* $Id$
*
* OpenMAMA: The open middleware agnostic messaging API
* Copyright (C) 2012 NYSE Technologies, Inc.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*/
package com.wombat.mamda;
import com.wombat.mama.*;
/**
* MamdaQuoteUpdate is an interface that provides access to fields
* related to quote updates.
*/
public interface MamdaQuoteUpdate extends MamdaBasicEvent
{
/**
* @return Bid price. The highest price that the representative
* party/group is willing to pay to buy the security. For most
* feeds, this size is represented in round lots.
*/
MamaPrice getBidPrice();
/**
* @return the bid price Field State
*/
short getBidPriceFieldState();
/**
* @return Total share size available for the current
* bid price. For most feeds, this size is represented in round
* lots.
*/
double getBidSize();
/**
* @return the bid size Field State
*/
short getBidSizeFieldState();
/**
* @return The identifier of the market participant (e.g. exchange
* or market maker) contributing the bid price field.
*/
String getBidPartId();
/**
* @return the bid part ID Field State
*/
short getBidPartIdFieldState();
/**
* @return Ask price. The lowest price that the representative
* party/group is willing to take to sell the security. For most
* feeds, this size is represented in round lots.
*/
MamaPrice getAskPrice();
/**
* @return the ask price Field State
*/
short getAskPriceFieldState();
/**
* @return Total share size available for the current
* ask price. For most feeds, this size is represented in round
* lots.
*/
double getAskSize();
/**
* @return the ask size Field State
*/
short getAskSizeFieldState();
/**
* @return The identifier of the market participant (e.g. exchange
* or market maker) contributing the ask price field.
*/
String getAskPartId();
/**
* @return the ask part ID Field State
*/
short getAskPartIdFieldState();
/**
* @return A normalized set of qualifiers for the last quote for
* the security. This field may contain multiple string values,
* separated by the colon(:) character.
*
*
* Value Meaning
*
* Normal
* Regular quote; no special condition
*
*
* DepthAsk
* Depth on ask side
*
*
* DepthBid
* Depth on bid side
*
*
* SlowQuoteOnAskSide
* This indicates that a market participants Ask is in a slow
* (CTA) mode. While in this mode, automated
* execution is not eligible on the Ask side and can be
* traded through pursuant to Regulation NMS requirements.
*
*
* SlowQuoteOnBidSide
* This indicates that a market participants Bid is in a slow
* (CTA) mode. While in this mode, automated
* execution is not eligible on the Bid side and can be
* traded through pursuant to Regulation NMS
* requirements.
*
*
* Fast
* Fast trading
*
*
* NonFirm
* Non-firm quote
*
*
* Rotation
* OPRA only. Quote relates to a trading rotation (Where a participant rotates through various clients that they are trading for)
*
*
* Auto
* Automatic trade
*
*
* Inactive
*
*
*
* SpecBid
* Specialist bid
*
*
* SpecAsk
* Specialist ask
*
*
* OneSided
* One sided. No orders, or only market orders, exist on one side
* of the book.
*
*
* PassiveMarketMaker
* Market Maker is both underwriter and buyer of security.
*
*
* LockedMarket
* Locked market - Bid is equal to Ask for OTCBB issues
*
*
*
* Crossed
* Crossed market - Bid is greater than Ask for OTCBB
*
*
*
* Synd
* Syndicate bid
*
*
* PreSynd
* Pre-syndicate bid
*
*
* Penalty
* Penalty bid
*
*
* UnsolBid
* Unsolicited bid
*
*
* UnsolAsk
* Unsolicited ask
*
*
* UnsolQuote
* Unsolicited quote
*
*
* Empty
* Empty quote (no quote)
*
*
* XpressBid
* NYSE LiquidityQuote Xpress bid indicator
*
*
* XpressAsk
* NYSE LiquidityQuote Xpress ask indicator
*
*
* BestOrder
*
*
*
* WillSell
*
*
*
* WillBuy
*
*
*
* AnyOrder
*
*
*
* MktOnly
* Market orders only.
*
*
* ManualAsk
* This indicates that a market participants Ask is in a manual
* (NASDAQ) mode. While in this mode, automated
* execution is not eligible on the Ask side and can be traded through
* pursuant to Regulation NMS requirements.
*
*
* ManualBid
* This indicates that a market participants Bid is in a manual
* (NASDAQ) mode. While in this mode, automated
* execution is not eligible on the Bid side and can be
* traded through pursuant to Regulation NMS requirements.
*
*
* AutomaticAsk
* This indicates that the marlet participant's Ask is in
* automatic mode - we generally send a combination of the last four
* (e.g. ManualAsk:AutomaticBid or ManualAsk;ManualBid)
*
*
* AutomaticBid
* This indicates that the marlet participant's Ask is in
* automatic mode - we generally send a combination of the last four
* (e.g. ManualAsk:AutomaticBid or ManualAsk;ManualBid)
*
*
* Closing
* Closing quote.
*
*
* Unknown
* Quote condition unknown.
*
*
*/
String getQuoteQual();
/**
* @return the quote qual Field State
*/
short getQuoteQualFieldState();
/**
* The exchange specific non normalized quote qualifier.
*
* @return The exchange specific quote qualifier.
* @see #getQuoteQual()
*/
String getQuoteQualNative ();
/**
* @return the quote qual native Field State
*/
short getQuoteQualNativeFieldState();
/**
* NASDAQ Bid Tick Indicator for Short Sale Rule Compliance.
* National Bid Tick Indicator based on changes to the bid price of the
* National Best Bid or Offer (National BBO).
*
*
* Value Meaning
*
* U
* Up Tick. The current Best Bid Price price is higher than the
* previous Best Bid Price for the given NNM security.
*
*
* D
* Down Tick. The current Best Bid Price price is lower than the
* previous Best Bid Price for the given NNM security.
*
*
* N
* No Tick. The NASD Short Sale Rule does not apply to issue (i.e.
* NASDAQ SmallCap listed security).
*
*
* Z
* Unset - default value within the API
*
*
*
* @return The tick bid indicator.
*/
char getShortSaleBidTick ();
/**
* @return the short sale bid tick Field State
*/
short getShortSaleBidTickFieldState();
/**
* get the ShortSaleCircuitBreaker
* @return ShortSaleCircuitBreaker
*
* - return values:
* - Blank: Short Sale Restriction Not in Effect.
* - A: Short Sale Restriction Activiated.
* - C: Short Sale Restriction Continued.
* - D: Sale Restriction Deactivated.
* - E: Sale Restriction in Effect.
*
*/
public char getShortSaleCircuitBreaker();
/**
* @return the reason Field State
*/
public short getShortSaleCircuitBreakerFieldState();
MamaDateTime getAskTime();
/**
* @return the ask time Field State
*/
short getAskTimeFieldState();
MamaDateTime getBidTime();
/**
* @return the bid time Field State
*/
short getBidTimeFieldState();
String getAskIndicator();
/**
* @return the ask indicator Field State
*/
short getAskIndicatorFieldState();
String getBidIndicator();
/**
* @return the bid indicator Field State
*/
short getBidIndicatorFieldState();
long getAskUpdateCount();
/**
* @return the ask update count Field State
*/
short getAskUpdateCountFieldState();
long getBidUpdateCount();
/**
* @return the bid update count Field State
*/
short getBidUpdateCountFieldState();
double getAskYield();
/**
* @return the ask yield Field State
*/
short getAskYieldFieldState();
double getBidYield();
/**
* @return the bid yield Field State
*/
short getBidYieldFieldState();
/**
* @return the quote Ask depth
**/
double getAskDepth();
/**
* @return the quote bid depth
**/
double getBidDepth();
/**
* @return the ask depth Field State
*/
short getAskDepthFieldState();
/**
* @return the bid depth Field State
*/
short getBidDepthFieldState();
String getBidSizesList();
/**
* @return the bid sizes list Field State
*/
short getBidSizesListFieldState();
String getAskSizesList();
/**
* @return the ask sizes list Field State
*/
short getAskSizesListFieldState();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy