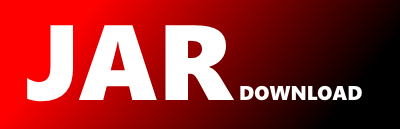
ome.xml.meta.MetadataStore Maven / Gradle / Ivy
Show all versions of ome-xml Show documentation
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.meta;
import java.util.List;
import ome.xml.model.*;
import ome.xml.model.enums.*;
import ome.xml.model.primitives.*;
import ome.units.quantity.Angle;
import ome.units.quantity.ElectricPotential;
import ome.units.quantity.Frequency;
import ome.units.quantity.Length;
import ome.units.quantity.Power;
import ome.units.quantity.Pressure;
import ome.units.quantity.Temperature;
import ome.units.quantity.Time;
import ome.units.unit.Unit;
/**
* A proxy whose responsibility it is to marshal biological image data into a
* particular storage medium.
*
* The MetadataStore
interface encompasses the metadata that
* any specific storage medium (file, relational database, etc.) should be
* expected to store into its backing data model.
*
*
The MetadataStore
interface goes hand in hand with the
* MetadataRetrieve
interface. Essentially,
* MetadataRetrieve
provides the "getter" methods for a storage
* medium, and MetadataStore
provides the "setter" methods.
*
*
Since it often makes sense for a storage medium to implement both
* interfaces, there is also an {@link IMetadata} interface encompassing
* both MetadataStore
and MetadataRetrieve
, which
* reduces the need to cast between object types.
*
*
See {@link ome.xml.meta.OMEXMLMetadata} for an example
* implementation.
*
*
Important note: It is strongly recommended that applications
* (e.g., file format readers) using MetadataStore
populate
* information in a linear order. Specifically, iterating over entities
* from "leftmost" index to "rightmost" index is required for certain
* MetadataStore
implementations such as OMERO's
* OMEROMetadataStore
. For example, when populating Image, Pixels
* and Plane information, an outer loop should iterate across
* imageIndex
, an inner loop should iterate across
* pixelsIndex
, and an innermost loop should handle
* planeIndex
. For an illustration of the ideal traversal order,
* see {@link ome.xml.meta.MetadataConverter#convertMetadata}.
*
* @author Chris Allan callan at blackcat.ca
* @author Curtis Rueden ctrueden at wisc.edu
*/
public interface MetadataStore extends BaseMetadata
{
/**
* Create root node. The action taken here is specific to the
* concrete metadata implementation.
*/
void createRoot();
/**
* Get the root node of the metadata. Note that the root node
* type will be specific to the concrete metadata
* implementation.
*
* @return the root node.
*/
MetadataRoot getRoot();
/**
* Set the root node of the metadata. Note that the root node
* type will be specific to the concrete metadata
* implementation. An exception will be thrown if the root
* node is of an incompatible type.
*
* @param root the root node.
*/
void setRoot(MetadataRoot root);
// -- Entity storage (manual definitions) --
/**
* Set the values of a MapAnnotation.
*
* @param value the MapAnnotation values to set.
* @param mapAnnotationIndex the MapAnnotation index.
*/
void setMapAnnotationValue(List value, int mapAnnotationIndex);
/**
* Set the MapAnnotation values of a GenericExcitationSource.
*
* @param map the MapAnnotation values to set.
* @param instrumentIndex the Instrument index.
*/
void setGenericExcitationSourceMap(List map, int instrumentIndex, int lightSourceIndex);
/**
* Set the MapAnnotation values of an ImagingEnvironment.
*
* @param map the MapAnnotation values to set.
* @param imageIndex the Image index.
*/
void setImagingEnvironmentMap(List map, int imageIndex);
// -- Entity storage (code generated definitions) --
/**
* Set the UUID associated with this collection of metadata.
*
* @param uuid the UUID to set.
*/
void setUUID(String uuid);
/**
* Set the Creator associated with this collection of metadata.
*
* @param creator the Creator to set.
*/
default void setCreator(String creator)
{
}
/**
* Set the AnnotationRef property of Arc.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setArcAnnotationRef(String annotation, int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Set the ID property of Arc.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcID(String id, int instrumentIndex, int lightSourceIndex);
/**
* Set the LotNumber property of Arc.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcLotNumber(String lotNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Manufacturer property of Arc.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcManufacturer(String manufacturer, int instrumentIndex, int lightSourceIndex);
/**
* Set the Model property of Arc.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcModel(String model, int instrumentIndex, int lightSourceIndex);
/**
* Set the Power property of Arc.
*
* @param power Power to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcPower(Power power, int instrumentIndex, int lightSourceIndex);
/**
* Set the SerialNumber property of Arc.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcSerialNumber(String serialNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Type property of Arc.
*
* @param type Type to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setArcType(ArcType type, int instrumentIndex, int lightSourceIndex);
/**
* Set the Base64Binary property of BinData.
*
* @param base64Binary Base64Binary to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileBinData(byte[] base64Binary, int fileAnnotationIndex);
/**
* Set the Base64Binary property of BinData.
*
* @param base64Binary Base64Binary to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskBinData(byte[] base64Binary, int ROIIndex, int shapeIndex);
/**
* Set the Base64Binary property of BinData.
*
* @param base64Binary Base64Binary to set.
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
*/
void setPixelsBinData(byte[] base64Binary, int imageIndex, int binDataIndex);
/**
* Set the BigEndian property of BinData.
*
* @param bigEndian BigEndian to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileBinDataBigEndian(Boolean bigEndian, int fileAnnotationIndex);
/**
* Set the BigEndian property of BinData.
*
* @param bigEndian BigEndian to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskBinDataBigEndian(Boolean bigEndian, int ROIIndex, int shapeIndex);
/**
* Set the BigEndian property of BinData.
*
* @param bigEndian BigEndian to set.
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
*/
void setPixelsBinDataBigEndian(Boolean bigEndian, int imageIndex, int binDataIndex);
/**
* Set the Compression property of BinData.
*
* @param compression Compression to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileBinDataCompression(Compression compression, int fileAnnotationIndex);
/**
* Set the Compression property of BinData.
*
* @param compression Compression to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskBinDataCompression(Compression compression, int ROIIndex, int shapeIndex);
/**
* Set the Compression property of BinData.
*
* @param compression Compression to set.
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
*/
void setPixelsBinDataCompression(Compression compression, int imageIndex, int binDataIndex);
/**
* Set the Length property of BinData.
*
* @param length Length to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileBinDataLength(NonNegativeLong length, int fileAnnotationIndex);
/**
* Set the Length property of BinData.
*
* @param length Length to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskBinDataLength(NonNegativeLong length, int ROIIndex, int shapeIndex);
/**
* Set the Length property of BinData.
*
* @param length Length to set.
* @param imageIndex the Image index.
* @param binDataIndex the BinData index.
*/
void setPixelsBinDataLength(NonNegativeLong length, int imageIndex, int binDataIndex);
/**
* Set the FileName property of BinaryFile.
*
* @param fileName FileName to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileFileName(String fileName, int fileAnnotationIndex);
/**
* Set the MIMEType property of BinaryFile.
*
* @param mimeType MIMEType to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileMIMEType(String mimeType, int fileAnnotationIndex);
/**
* Set the Size property of BinaryFile.
*
* @param size Size to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setBinaryFileSize(NonNegativeLong size, int fileAnnotationIndex);
/**
* Set the MetadataFile property of BinaryOnly.
*
* @param metadataFile MetadataFile to set.
*/
void setBinaryOnlyMetadataFile(String metadataFile);
/**
* Set the UUID property of BinaryOnly.
*
* @param uuid UUID to set.
*/
void setBinaryOnlyUUID(String uuid);
/**
* Set the AnnotationRef property of BooleanAnnotation.
*
* @param annotation AnnotationRef to set.
* @param booleanAnnotationIndex the BooleanAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setBooleanAnnotationAnnotationRef(String annotation, int booleanAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of BooleanAnnotation.
*
* @param annotator Annotator to set.
* @param booleanAnnotationIndex the BooleanAnnotation index.
*/
void setBooleanAnnotationAnnotator(String annotator, int booleanAnnotationIndex);
/**
* Set the Description property of BooleanAnnotation.
*
* @param description Description to set.
* @param booleanAnnotationIndex the BooleanAnnotation index.
*/
void setBooleanAnnotationDescription(String description, int booleanAnnotationIndex);
/**
* Set the ID property of BooleanAnnotation.
*
* @param id ID to set.
* @param booleanAnnotationIndex the BooleanAnnotation index.
*/
void setBooleanAnnotationID(String id, int booleanAnnotationIndex);
/**
* Set the Namespace property of BooleanAnnotation.
*
* @param namespace Namespace to set.
* @param booleanAnnotationIndex the BooleanAnnotation index.
*/
void setBooleanAnnotationNamespace(String namespace, int booleanAnnotationIndex);
/**
* Set the Value property of BooleanAnnotation.
*
* @param value Value to set.
* @param booleanAnnotationIndex the BooleanAnnotation index.
*/
void setBooleanAnnotationValue(Boolean value, int booleanAnnotationIndex);
/**
* Set the AcquisitionMode property of Channel.
*
* @param acquisitionMode AcquisitionMode to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelAcquisitionMode(AcquisitionMode acquisitionMode, int imageIndex, int channelIndex);
/**
* Set the AnnotationRef property of Channel.
*
* @param annotation AnnotationRef to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setChannelAnnotationRef(String annotation, int imageIndex, int channelIndex, int annotationRefIndex);
/**
* Set the Color property of Channel.
*
* @param color Color to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelColor(Color color, int imageIndex, int channelIndex);
/**
* Set the ContrastMethod property of Channel.
*
* @param contrastMethod ContrastMethod to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelContrastMethod(ContrastMethod contrastMethod, int imageIndex, int channelIndex);
/**
* Set the EmissionWavelength property of Channel.
*
* @param emissionWavelength EmissionWavelength to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelEmissionWavelength(Length emissionWavelength, int imageIndex, int channelIndex);
/**
* Set the ExcitationWavelength property of Channel.
*
* @param excitationWavelength ExcitationWavelength to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelExcitationWavelength(Length excitationWavelength, int imageIndex, int channelIndex);
/**
* Set the FilterSetRef property of Channel.
*
* @param filterSet FilterSetRef to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelFilterSetRef(String filterSet, int imageIndex, int channelIndex);
/**
* Set the Fluor property of Channel.
*
* @param fluor Fluor to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelFluor(String fluor, int imageIndex, int channelIndex);
/**
* Set the ID property of Channel.
*
* @param id ID to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelID(String id, int imageIndex, int channelIndex);
/**
* Set the IlluminationType property of Channel.
*
* @param illuminationType IlluminationType to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelIlluminationType(IlluminationType illuminationType, int imageIndex, int channelIndex);
/**
* Set the NDFilter property of Channel.
*
* @param ndFilter NDFilter to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelNDFilter(Double ndFilter, int imageIndex, int channelIndex);
/**
* Set the Name property of Channel.
*
* @param name Name to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelName(String name, int imageIndex, int channelIndex);
/**
* Set the PinholeSize property of Channel.
*
* @param pinholeSize PinholeSize to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelPinholeSize(Length pinholeSize, int imageIndex, int channelIndex);
/**
* Set the PockelCellSetting property of Channel.
*
* @param pockelCellSetting PockelCellSetting to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelPockelCellSetting(Integer pockelCellSetting, int imageIndex, int channelIndex);
/**
* Set the SamplesPerPixel property of Channel.
*
* @param samplesPerPixel SamplesPerPixel to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelSamplesPerPixel(PositiveInteger samplesPerPixel, int imageIndex, int channelIndex);
/**
* Set the AnnotationRef property of CommentAnnotation.
*
* @param annotation AnnotationRef to set.
* @param commentAnnotationIndex the CommentAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setCommentAnnotationAnnotationRef(String annotation, int commentAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of CommentAnnotation.
*
* @param annotator Annotator to set.
* @param commentAnnotationIndex the CommentAnnotation index.
*/
void setCommentAnnotationAnnotator(String annotator, int commentAnnotationIndex);
/**
* Set the Description property of CommentAnnotation.
*
* @param description Description to set.
* @param commentAnnotationIndex the CommentAnnotation index.
*/
void setCommentAnnotationDescription(String description, int commentAnnotationIndex);
/**
* Set the ID property of CommentAnnotation.
*
* @param id ID to set.
* @param commentAnnotationIndex the CommentAnnotation index.
*/
void setCommentAnnotationID(String id, int commentAnnotationIndex);
/**
* Set the Namespace property of CommentAnnotation.
*
* @param namespace Namespace to set.
* @param commentAnnotationIndex the CommentAnnotation index.
*/
void setCommentAnnotationNamespace(String namespace, int commentAnnotationIndex);
/**
* Set the Value property of CommentAnnotation.
*
* @param value Value to set.
* @param commentAnnotationIndex the CommentAnnotation index.
*/
void setCommentAnnotationValue(String value, int commentAnnotationIndex);
/**
* Set the AnnotationRef property of Dataset.
*
* @param annotation AnnotationRef to set.
* @param datasetIndex the Dataset index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setDatasetAnnotationRef(String annotation, int datasetIndex, int annotationRefIndex);
/**
* Set the Description property of Dataset.
*
* @param description Description to set.
* @param datasetIndex the Dataset index.
*/
void setDatasetDescription(String description, int datasetIndex);
/**
* Set the ExperimenterGroupRef property of Dataset.
*
* @param experimenterGroup ExperimenterGroupRef to set.
* @param datasetIndex the Dataset index.
*/
void setDatasetExperimenterGroupRef(String experimenterGroup, int datasetIndex);
/**
* Set the ExperimenterRef property of Dataset.
*
* @param experimenter ExperimenterRef to set.
* @param datasetIndex the Dataset index.
*/
void setDatasetExperimenterRef(String experimenter, int datasetIndex);
/**
* Set the ID property of Dataset.
*
* @param id ID to set.
* @param datasetIndex the Dataset index.
*/
void setDatasetID(String id, int datasetIndex);
/**
* Set the ImageRef property of Dataset.
*
* @param image ImageRef to set.
* @param datasetIndex the Dataset index.
* @param imageRefIndex ImageRef index (unused).
*/
void setDatasetImageRef(String image, int datasetIndex, int imageRefIndex);
/**
* Set the Name property of Dataset.
*
* @param name Name to set.
* @param datasetIndex the Dataset index.
*/
void setDatasetName(String name, int datasetIndex);
/**
* Set the AmplificationGain property of Detector.
*
* @param amplificationGain AmplificationGain to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorAmplificationGain(Double amplificationGain, int instrumentIndex, int detectorIndex);
/**
* Set the AnnotationRef property of Detector.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setDetectorAnnotationRef(String annotation, int instrumentIndex, int detectorIndex, int annotationRefIndex);
/**
* Set the Gain property of Detector.
*
* @param gain Gain to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorGain(Double gain, int instrumentIndex, int detectorIndex);
/**
* Set the ID property of Detector.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorID(String id, int instrumentIndex, int detectorIndex);
/**
* Set the LotNumber property of Detector.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorLotNumber(String lotNumber, int instrumentIndex, int detectorIndex);
/**
* Set the Manufacturer property of Detector.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorManufacturer(String manufacturer, int instrumentIndex, int detectorIndex);
/**
* Set the Model property of Detector.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorModel(String model, int instrumentIndex, int detectorIndex);
/**
* Set the Offset property of Detector.
*
* @param offset Offset to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorOffset(Double offset, int instrumentIndex, int detectorIndex);
/**
* Set the SerialNumber property of Detector.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorSerialNumber(String serialNumber, int instrumentIndex, int detectorIndex);
/**
* Set the Type property of Detector.
*
* @param type Type to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorType(DetectorType type, int instrumentIndex, int detectorIndex);
/**
* Set the Voltage property of Detector.
*
* @param voltage Voltage to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorVoltage(ElectricPotential voltage, int instrumentIndex, int detectorIndex);
/**
* Set the Zoom property of Detector.
*
* @param zoom Zoom to set.
* @param instrumentIndex the Instrument index.
* @param detectorIndex the Detector index.
*/
void setDetectorZoom(Double zoom, int instrumentIndex, int detectorIndex);
/**
* Set the Binning property of DetectorSettings.
*
* @param binning Binning to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsBinning(Binning binning, int imageIndex, int channelIndex);
/**
* Set the Gain property of DetectorSettings.
*
* @param gain Gain to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsGain(Double gain, int imageIndex, int channelIndex);
/**
* Set the ID property of DetectorSettings.
*
* @param id ID to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsID(String id, int imageIndex, int channelIndex);
/**
* Set the Integration property of DetectorSettings.
*
* @param integration Integration to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsIntegration(PositiveInteger integration, int imageIndex, int channelIndex);
/**
* Set the Offset property of DetectorSettings.
*
* @param offset Offset to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsOffset(Double offset, int imageIndex, int channelIndex);
/**
* Set the ReadOutRate property of DetectorSettings.
*
* @param readOutRate ReadOutRate to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsReadOutRate(Frequency readOutRate, int imageIndex, int channelIndex);
/**
* Set the Voltage property of DetectorSettings.
*
* @param voltage Voltage to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsVoltage(ElectricPotential voltage, int imageIndex, int channelIndex);
/**
* Set the Zoom property of DetectorSettings.
*
* @param zoom Zoom to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setDetectorSettingsZoom(Double zoom, int imageIndex, int channelIndex);
/**
* Set the AnnotationRef property of Dichroic.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setDichroicAnnotationRef(String annotation, int instrumentIndex, int dichroicIndex, int annotationRefIndex);
/**
* Set the ID property of Dichroic.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
*/
void setDichroicID(String id, int instrumentIndex, int dichroicIndex);
/**
* Set the LotNumber property of Dichroic.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
*/
void setDichroicLotNumber(String lotNumber, int instrumentIndex, int dichroicIndex);
/**
* Set the Manufacturer property of Dichroic.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
*/
void setDichroicManufacturer(String manufacturer, int instrumentIndex, int dichroicIndex);
/**
* Set the Model property of Dichroic.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
*/
void setDichroicModel(String model, int instrumentIndex, int dichroicIndex);
/**
* Set the SerialNumber property of Dichroic.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param dichroicIndex the Dichroic index.
*/
void setDichroicSerialNumber(String serialNumber, int instrumentIndex, int dichroicIndex);
/**
* Set the AnnotationRef property of DoubleAnnotation.
*
* @param annotation AnnotationRef to set.
* @param doubleAnnotationIndex the DoubleAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setDoubleAnnotationAnnotationRef(String annotation, int doubleAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of DoubleAnnotation.
*
* @param annotator Annotator to set.
* @param doubleAnnotationIndex the DoubleAnnotation index.
*/
void setDoubleAnnotationAnnotator(String annotator, int doubleAnnotationIndex);
/**
* Set the Description property of DoubleAnnotation.
*
* @param description Description to set.
* @param doubleAnnotationIndex the DoubleAnnotation index.
*/
void setDoubleAnnotationDescription(String description, int doubleAnnotationIndex);
/**
* Set the ID property of DoubleAnnotation.
*
* @param id ID to set.
* @param doubleAnnotationIndex the DoubleAnnotation index.
*/
void setDoubleAnnotationID(String id, int doubleAnnotationIndex);
/**
* Set the Namespace property of DoubleAnnotation.
*
* @param namespace Namespace to set.
* @param doubleAnnotationIndex the DoubleAnnotation index.
*/
void setDoubleAnnotationNamespace(String namespace, int doubleAnnotationIndex);
/**
* Set the Value property of DoubleAnnotation.
*
* @param value Value to set.
* @param doubleAnnotationIndex the DoubleAnnotation index.
*/
void setDoubleAnnotationValue(Double value, int doubleAnnotationIndex);
/**
* Set the AnnotationRef property of Ellipse.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setEllipseAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Ellipse.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Ellipse.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Ellipse.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Ellipse.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Ellipse.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Ellipse.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Ellipse.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the RadiusX property of Ellipse.
*
* @param radiusX RadiusX to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseRadiusX(Double radiusX, int ROIIndex, int shapeIndex);
/**
* Set the RadiusY property of Ellipse.
*
* @param radiusY RadiusY to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseRadiusY(Double radiusY, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Ellipse.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Ellipse.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Ellipse.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Ellipse.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Ellipse.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Ellipse.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Ellipse.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Ellipse.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the X property of Ellipse.
*
* @param x X to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseX(Double x, int ROIIndex, int shapeIndex);
/**
* Set the Y property of Ellipse.
*
* @param y Y to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setEllipseY(Double y, int ROIIndex, int shapeIndex);
/**
* Set the Description property of Experiment.
*
* @param description Description to set.
* @param experimentIndex the Experiment index.
*/
void setExperimentDescription(String description, int experimentIndex);
/**
* Set the ExperimenterRef property of Experiment.
*
* @param experimenter ExperimenterRef to set.
* @param experimentIndex the Experiment index.
*/
void setExperimentExperimenterRef(String experimenter, int experimentIndex);
/**
* Set the ID property of Experiment.
*
* @param id ID to set.
* @param experimentIndex the Experiment index.
*/
void setExperimentID(String id, int experimentIndex);
/**
* Set the Type property of Experiment.
*
* @param type Type to set.
* @param experimentIndex the Experiment index.
*/
void setExperimentType(ExperimentType type, int experimentIndex);
/**
* Set the AnnotationRef property of Experimenter.
*
* @param annotation AnnotationRef to set.
* @param experimenterIndex the Experimenter index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setExperimenterAnnotationRef(String annotation, int experimenterIndex, int annotationRefIndex);
/**
* Set the Email property of Experimenter.
*
* @param email Email to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterEmail(String email, int experimenterIndex);
/**
* Set the FirstName property of Experimenter.
*
* @param firstName FirstName to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterFirstName(String firstName, int experimenterIndex);
/**
* Set the ID property of Experimenter.
*
* @param id ID to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterID(String id, int experimenterIndex);
/**
* Set the Institution property of Experimenter.
*
* @param institution Institution to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterInstitution(String institution, int experimenterIndex);
/**
* Set the LastName property of Experimenter.
*
* @param lastName LastName to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterLastName(String lastName, int experimenterIndex);
/**
* Set the MiddleName property of Experimenter.
*
* @param middleName MiddleName to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterMiddleName(String middleName, int experimenterIndex);
/**
* Set the UserName property of Experimenter.
*
* @param userName UserName to set.
* @param experimenterIndex the Experimenter index.
*/
void setExperimenterUserName(String userName, int experimenterIndex);
/**
* Set the AnnotationRef property of ExperimenterGroup.
*
* @param annotation AnnotationRef to set.
* @param experimenterGroupIndex the ExperimenterGroup index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setExperimenterGroupAnnotationRef(String annotation, int experimenterGroupIndex, int annotationRefIndex);
/**
* Set the Description property of ExperimenterGroup.
*
* @param description Description to set.
* @param experimenterGroupIndex the ExperimenterGroup index.
*/
void setExperimenterGroupDescription(String description, int experimenterGroupIndex);
/**
* Set the ExperimenterRef property of ExperimenterGroup.
*
* @param experimenter ExperimenterRef to set.
* @param experimenterGroupIndex the ExperimenterGroup index.
* @param experimenterRefIndex ExperimenterRef index (unused).
*/
void setExperimenterGroupExperimenterRef(String experimenter, int experimenterGroupIndex, int experimenterRefIndex);
/**
* Set the ID property of ExperimenterGroup.
*
* @param id ID to set.
* @param experimenterGroupIndex the ExperimenterGroup index.
*/
void setExperimenterGroupID(String id, int experimenterGroupIndex);
/**
* Set the Leader property of ExperimenterGroup.
*
* @param leader Leader to set.
* @param experimenterGroupIndex the ExperimenterGroup index.
* @param leaderIndex Leader index (unused).
*/
void setExperimenterGroupLeader(String leader, int experimenterGroupIndex, int leaderIndex);
/**
* Set the Name property of ExperimenterGroup.
*
* @param name Name to set.
* @param experimenterGroupIndex the ExperimenterGroup index.
*/
void setExperimenterGroupName(String name, int experimenterGroupIndex);
/**
* Set the AnnotationRef property of Filament.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setFilamentAnnotationRef(String annotation, int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Set the ID property of Filament.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentID(String id, int instrumentIndex, int lightSourceIndex);
/**
* Set the LotNumber property of Filament.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentLotNumber(String lotNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Manufacturer property of Filament.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentManufacturer(String manufacturer, int instrumentIndex, int lightSourceIndex);
/**
* Set the Model property of Filament.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentModel(String model, int instrumentIndex, int lightSourceIndex);
/**
* Set the Power property of Filament.
*
* @param power Power to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentPower(Power power, int instrumentIndex, int lightSourceIndex);
/**
* Set the SerialNumber property of Filament.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentSerialNumber(String serialNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Type property of Filament.
*
* @param type Type to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setFilamentType(FilamentType type, int instrumentIndex, int lightSourceIndex);
/**
* Set the AnnotationRef property of FileAnnotation.
*
* @param annotation AnnotationRef to set.
* @param fileAnnotationIndex the FileAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setFileAnnotationAnnotationRef(String annotation, int fileAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of FileAnnotation.
*
* @param annotator Annotator to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setFileAnnotationAnnotator(String annotator, int fileAnnotationIndex);
/**
* Set the Description property of FileAnnotation.
*
* @param description Description to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setFileAnnotationDescription(String description, int fileAnnotationIndex);
/**
* Set the ID property of FileAnnotation.
*
* @param id ID to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setFileAnnotationID(String id, int fileAnnotationIndex);
/**
* Set the Namespace property of FileAnnotation.
*
* @param namespace Namespace to set.
* @param fileAnnotationIndex the FileAnnotation index.
*/
void setFileAnnotationNamespace(String namespace, int fileAnnotationIndex);
/**
* Set the AnnotationRef property of Filter.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setFilterAnnotationRef(String annotation, int instrumentIndex, int filterIndex, int annotationRefIndex);
/**
* Set the FilterWheel property of Filter.
*
* @param filterWheel FilterWheel to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterFilterWheel(String filterWheel, int instrumentIndex, int filterIndex);
/**
* Set the ID property of Filter.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterID(String id, int instrumentIndex, int filterIndex);
/**
* Set the LotNumber property of Filter.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterLotNumber(String lotNumber, int instrumentIndex, int filterIndex);
/**
* Set the Manufacturer property of Filter.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterManufacturer(String manufacturer, int instrumentIndex, int filterIndex);
/**
* Set the Model property of Filter.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterModel(String model, int instrumentIndex, int filterIndex);
/**
* Set the SerialNumber property of Filter.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterSerialNumber(String serialNumber, int instrumentIndex, int filterIndex);
/**
* Set the Type property of Filter.
*
* @param type Type to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setFilterType(FilterType type, int instrumentIndex, int filterIndex);
/**
* Set the DichroicRef property of FilterSet.
*
* @param dichroic DichroicRef to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
*/
void setFilterSetDichroicRef(String dichroic, int instrumentIndex, int filterSetIndex);
/**
* Set the EmissionFilterRef property of FilterSet.
*
* @param emissionFilter EmissionFilterRef to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @param emissionFilterRefIndex EmissionFilterRef index (unused).
*/
void setFilterSetEmissionFilterRef(String emissionFilter, int instrumentIndex, int filterSetIndex, int emissionFilterRefIndex);
/**
* Set the ExcitationFilterRef property of FilterSet.
*
* @param excitationFilter ExcitationFilterRef to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
* @param excitationFilterRefIndex ExcitationFilterRef index (unused).
*/
void setFilterSetExcitationFilterRef(String excitationFilter, int instrumentIndex, int filterSetIndex, int excitationFilterRefIndex);
/**
* Set the ID property of FilterSet.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
*/
void setFilterSetID(String id, int instrumentIndex, int filterSetIndex);
/**
* Set the LotNumber property of FilterSet.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
*/
void setFilterSetLotNumber(String lotNumber, int instrumentIndex, int filterSetIndex);
/**
* Set the Manufacturer property of FilterSet.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
*/
void setFilterSetManufacturer(String manufacturer, int instrumentIndex, int filterSetIndex);
/**
* Set the Model property of FilterSet.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
*/
void setFilterSetModel(String model, int instrumentIndex, int filterSetIndex);
/**
* Set the SerialNumber property of FilterSet.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param filterSetIndex the FilterSet index.
*/
void setFilterSetSerialNumber(String serialNumber, int instrumentIndex, int filterSetIndex);
/**
* Set the AnnotationRef property of Folder.
*
* @param annotation AnnotationRef to set.
* @param folderIndex the Folder index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setFolderAnnotationRef(String annotation, int folderIndex, int annotationRefIndex);
/**
* Set the Description property of Folder.
*
* @param description Description to set.
* @param folderIndex the Folder index.
*/
void setFolderDescription(String description, int folderIndex);
/**
* Set the FolderRef property of Folder.
*
* @param folder FolderRef to set.
* @param folderIndex the Folder index.
* @param folderRefIndex FolderRef index (unused).
*/
void setFolderFolderRef(String folder, int folderIndex, int folderRefIndex);
/**
* Set the ID property of Folder.
*
* @param id ID to set.
* @param folderIndex the Folder index.
*/
void setFolderID(String id, int folderIndex);
/**
* Set the ImageRef property of Folder.
*
* @param image ImageRef to set.
* @param folderIndex the Folder index.
* @param imageRefIndex ImageRef index (unused).
*/
void setFolderImageRef(String image, int folderIndex, int imageRefIndex);
/**
* Set the Name property of Folder.
*
* @param name Name to set.
* @param folderIndex the Folder index.
*/
void setFolderName(String name, int folderIndex);
/**
* Set the ROIRef property of Folder.
*
* @param roi ROIRef to set.
* @param folderIndex the Folder index.
* @param ROIRefIndex ROIRef index (unused).
*/
void setFolderROIRef(String roi, int folderIndex, int ROIRefIndex);
/**
* Set the AnnotationRef property of GenericExcitationSource.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setGenericExcitationSourceAnnotationRef(String annotation, int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Set the ID property of GenericExcitationSource.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setGenericExcitationSourceID(String id, int instrumentIndex, int lightSourceIndex);
/**
* Set the LotNumber property of GenericExcitationSource.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setGenericExcitationSourceLotNumber(String lotNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Manufacturer property of GenericExcitationSource.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setGenericExcitationSourceManufacturer(String manufacturer, int instrumentIndex, int lightSourceIndex);
/**
* Set the Model property of GenericExcitationSource.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setGenericExcitationSourceModel(String model, int instrumentIndex, int lightSourceIndex);
/**
* Set the Power property of GenericExcitationSource.
*
* @param power Power to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setGenericExcitationSourcePower(Power power, int instrumentIndex, int lightSourceIndex);
/**
* Set the SerialNumber property of GenericExcitationSource.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setGenericExcitationSourceSerialNumber(String serialNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the AcquisitionDate property of Image.
*
* @param acquisitionDate AcquisitionDate to set.
* @param imageIndex the Image index.
*/
void setImageAcquisitionDate(Timestamp acquisitionDate, int imageIndex);
/**
* Set the AnnotationRef property of Image.
*
* @param annotation AnnotationRef to set.
* @param imageIndex the Image index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setImageAnnotationRef(String annotation, int imageIndex, int annotationRefIndex);
/**
* Set the Description property of Image.
*
* @param description Description to set.
* @param imageIndex the Image index.
*/
void setImageDescription(String description, int imageIndex);
/**
* Set the ExperimentRef property of Image.
*
* @param experiment ExperimentRef to set.
* @param imageIndex the Image index.
*/
void setImageExperimentRef(String experiment, int imageIndex);
/**
* Set the ExperimenterGroupRef property of Image.
*
* @param experimenterGroup ExperimenterGroupRef to set.
* @param imageIndex the Image index.
*/
void setImageExperimenterGroupRef(String experimenterGroup, int imageIndex);
/**
* Set the ExperimenterRef property of Image.
*
* @param experimenter ExperimenterRef to set.
* @param imageIndex the Image index.
*/
void setImageExperimenterRef(String experimenter, int imageIndex);
/**
* Set the ID property of Image.
*
* @param id ID to set.
* @param imageIndex the Image index.
*/
void setImageID(String id, int imageIndex);
/**
* Set the InstrumentRef property of Image.
*
* @param instrument InstrumentRef to set.
* @param imageIndex the Image index.
*/
void setImageInstrumentRef(String instrument, int imageIndex);
/**
* Set the MicrobeamManipulationRef property of Image.
*
* @param microbeamManipulation MicrobeamManipulationRef to set.
* @param imageIndex the Image index.
* @param microbeamManipulationRefIndex MicrobeamManipulationRef index (unused).
*/
void setImageMicrobeamManipulationRef(String microbeamManipulation, int imageIndex, int microbeamManipulationRefIndex);
/**
* Set the Name property of Image.
*
* @param name Name to set.
* @param imageIndex the Image index.
*/
void setImageName(String name, int imageIndex);
/**
* Set the ROIRef property of Image.
*
* @param roi ROIRef to set.
* @param imageIndex the Image index.
* @param ROIRefIndex ROIRef index (unused).
*/
void setImageROIRef(String roi, int imageIndex, int ROIRefIndex);
/**
* Set the AirPressure property of ImagingEnvironment.
*
* @param airPressure AirPressure to set.
* @param imageIndex the Image index.
*/
void setImagingEnvironmentAirPressure(Pressure airPressure, int imageIndex);
/**
* Set the CO2Percent property of ImagingEnvironment.
*
* @param co2Percent CO2Percent to set.
* @param imageIndex the Image index.
*/
void setImagingEnvironmentCO2Percent(PercentFraction co2Percent, int imageIndex);
/**
* Set the Humidity property of ImagingEnvironment.
*
* @param humidity Humidity to set.
* @param imageIndex the Image index.
*/
void setImagingEnvironmentHumidity(PercentFraction humidity, int imageIndex);
/**
* Set the Temperature property of ImagingEnvironment.
*
* @param temperature Temperature to set.
* @param imageIndex the Image index.
*/
void setImagingEnvironmentTemperature(Temperature temperature, int imageIndex);
/**
* Set the AnnotationRef property of Instrument.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setInstrumentAnnotationRef(String annotation, int instrumentIndex, int annotationRefIndex);
/**
* Set the ID property of Instrument.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
*/
void setInstrumentID(String id, int instrumentIndex);
/**
* Set the AnnotationRef property of Label.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setLabelAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Label.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Label.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Label.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Label.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Label.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Label.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Label.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Label.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Label.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Label.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Label.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Label.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Label.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Label.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Label.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the X property of Label.
*
* @param x X to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelX(Double x, int ROIIndex, int shapeIndex);
/**
* Set the Y property of Label.
*
* @param y Y to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLabelY(Double y, int ROIIndex, int shapeIndex);
/**
* Set the AnnotationRef property of Laser.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setLaserAnnotationRef(String annotation, int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Set the FrequencyMultiplication property of Laser.
*
* @param frequencyMultiplication FrequencyMultiplication to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserFrequencyMultiplication(PositiveInteger frequencyMultiplication, int instrumentIndex, int lightSourceIndex);
/**
* Set the ID property of Laser.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserID(String id, int instrumentIndex, int lightSourceIndex);
/**
* Set the LaserMedium property of Laser.
*
* @param laserMedium LaserMedium to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserLaserMedium(LaserMedium laserMedium, int instrumentIndex, int lightSourceIndex);
/**
* Set the LotNumber property of Laser.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserLotNumber(String lotNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Manufacturer property of Laser.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserManufacturer(String manufacturer, int instrumentIndex, int lightSourceIndex);
/**
* Set the Model property of Laser.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserModel(String model, int instrumentIndex, int lightSourceIndex);
/**
* Set the PockelCell property of Laser.
*
* @param pockelCell PockelCell to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserPockelCell(Boolean pockelCell, int instrumentIndex, int lightSourceIndex);
/**
* Set the Power property of Laser.
*
* @param power Power to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserPower(Power power, int instrumentIndex, int lightSourceIndex);
/**
* Set the Pulse property of Laser.
*
* @param pulse Pulse to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserPulse(Pulse pulse, int instrumentIndex, int lightSourceIndex);
/**
* Set the Pump property of Laser.
*
* @param pump Pump to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserPump(String pump, int instrumentIndex, int lightSourceIndex);
/**
* Set the RepetitionRate property of Laser.
*
* @param repetitionRate RepetitionRate to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserRepetitionRate(Frequency repetitionRate, int instrumentIndex, int lightSourceIndex);
/**
* Set the SerialNumber property of Laser.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserSerialNumber(String serialNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Tuneable property of Laser.
*
* @param tuneable Tuneable to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserTuneable(Boolean tuneable, int instrumentIndex, int lightSourceIndex);
/**
* Set the Type property of Laser.
*
* @param type Type to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserType(LaserType type, int instrumentIndex, int lightSourceIndex);
/**
* Set the Wavelength property of Laser.
*
* @param wavelength Wavelength to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLaserWavelength(Length wavelength, int instrumentIndex, int lightSourceIndex);
/**
* Set the AnnotationRef property of LightEmittingDiode.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setLightEmittingDiodeAnnotationRef(String annotation, int instrumentIndex, int lightSourceIndex, int annotationRefIndex);
/**
* Set the ID property of LightEmittingDiode.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLightEmittingDiodeID(String id, int instrumentIndex, int lightSourceIndex);
/**
* Set the LotNumber property of LightEmittingDiode.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLightEmittingDiodeLotNumber(String lotNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the Manufacturer property of LightEmittingDiode.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLightEmittingDiodeManufacturer(String manufacturer, int instrumentIndex, int lightSourceIndex);
/**
* Set the Model property of LightEmittingDiode.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLightEmittingDiodeModel(String model, int instrumentIndex, int lightSourceIndex);
/**
* Set the Power property of LightEmittingDiode.
*
* @param power Power to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLightEmittingDiodePower(Power power, int instrumentIndex, int lightSourceIndex);
/**
* Set the SerialNumber property of LightEmittingDiode.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param lightSourceIndex the LightSource index.
*/
void setLightEmittingDiodeSerialNumber(String serialNumber, int instrumentIndex, int lightSourceIndex);
/**
* Set the AnnotationRef property of LightPath.
*
* @param annotation AnnotationRef to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setLightPathAnnotationRef(String annotation, int imageIndex, int channelIndex, int annotationRefIndex);
/**
* Set the DichroicRef property of LightPath.
*
* @param dichroic DichroicRef to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setLightPathDichroicRef(String dichroic, int imageIndex, int channelIndex);
/**
* Set the EmissionFilterRef property of LightPath.
*
* @param emissionFilter EmissionFilterRef to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param emissionFilterRefIndex EmissionFilterRef index (unused).
*/
void setLightPathEmissionFilterRef(String emissionFilter, int imageIndex, int channelIndex, int emissionFilterRefIndex);
/**
* Set the ExcitationFilterRef property of LightPath.
*
* @param excitationFilter ExcitationFilterRef to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
* @param excitationFilterRefIndex ExcitationFilterRef index (unused).
*/
void setLightPathExcitationFilterRef(String excitationFilter, int imageIndex, int channelIndex, int excitationFilterRefIndex);
/**
* Set the Attenuation property of LightSourceSettings.
*
* @param attenuation Attenuation to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelLightSourceSettingsAttenuation(PercentFraction attenuation, int imageIndex, int channelIndex);
/**
* Set the Attenuation property of LightSourceSettings.
*
* @param attenuation Attenuation to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param lightSourceSettingsIndex the LightSourceSettings index.
*/
void setMicrobeamManipulationLightSourceSettingsAttenuation(PercentFraction attenuation, int experimentIndex, int microbeamManipulationIndex, int lightSourceSettingsIndex);
/**
* Set the ID property of LightSourceSettings.
*
* @param id ID to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelLightSourceSettingsID(String id, int imageIndex, int channelIndex);
/**
* Set the ID property of LightSourceSettings.
*
* @param id ID to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param lightSourceSettingsIndex the LightSourceSettings index.
*/
void setMicrobeamManipulationLightSourceSettingsID(String id, int experimentIndex, int microbeamManipulationIndex, int lightSourceSettingsIndex);
/**
* Set the Wavelength property of LightSourceSettings.
*
* @param wavelength Wavelength to set.
* @param imageIndex the Image index.
* @param channelIndex the Channel index.
*/
void setChannelLightSourceSettingsWavelength(Length wavelength, int imageIndex, int channelIndex);
/**
* Set the Wavelength property of LightSourceSettings.
*
* @param wavelength Wavelength to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param lightSourceSettingsIndex the LightSourceSettings index.
*/
void setMicrobeamManipulationLightSourceSettingsWavelength(Length wavelength, int experimentIndex, int microbeamManipulationIndex, int lightSourceSettingsIndex);
/**
* Set the AnnotationRef property of Line.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setLineAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Line.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Line.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Line.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Line.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Line.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Line.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Line.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the MarkerEnd property of Line.
*
* @param markerEnd MarkerEnd to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineMarkerEnd(Marker markerEnd, int ROIIndex, int shapeIndex);
/**
* Set the MarkerStart property of Line.
*
* @param markerStart MarkerStart to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineMarkerStart(Marker markerStart, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Line.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Line.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Line.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Line.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Line.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Line.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Line.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Line.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the X1 property of Line.
*
* @param x1 X1 to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineX1(Double x1, int ROIIndex, int shapeIndex);
/**
* Set the X2 property of Line.
*
* @param x2 X2 to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineX2(Double x2, int ROIIndex, int shapeIndex);
/**
* Set the Y1 property of Line.
*
* @param y1 Y1 to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineY1(Double y1, int ROIIndex, int shapeIndex);
/**
* Set the Y2 property of Line.
*
* @param y2 Y2 to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setLineY2(Double y2, int ROIIndex, int shapeIndex);
/**
* Set the AnnotationRef property of ListAnnotation.
*
* @param annotation AnnotationRef to set.
* @param listAnnotationIndex the ListAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setListAnnotationAnnotationRef(String annotation, int listAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of ListAnnotation.
*
* @param annotator Annotator to set.
* @param listAnnotationIndex the ListAnnotation index.
*/
void setListAnnotationAnnotator(String annotator, int listAnnotationIndex);
/**
* Set the Description property of ListAnnotation.
*
* @param description Description to set.
* @param listAnnotationIndex the ListAnnotation index.
*/
void setListAnnotationDescription(String description, int listAnnotationIndex);
/**
* Set the ID property of ListAnnotation.
*
* @param id ID to set.
* @param listAnnotationIndex the ListAnnotation index.
*/
void setListAnnotationID(String id, int listAnnotationIndex);
/**
* Set the Namespace property of ListAnnotation.
*
* @param namespace Namespace to set.
* @param listAnnotationIndex the ListAnnotation index.
*/
void setListAnnotationNamespace(String namespace, int listAnnotationIndex);
/**
* Set the AnnotationRef property of LongAnnotation.
*
* @param annotation AnnotationRef to set.
* @param longAnnotationIndex the LongAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setLongAnnotationAnnotationRef(String annotation, int longAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of LongAnnotation.
*
* @param annotator Annotator to set.
* @param longAnnotationIndex the LongAnnotation index.
*/
void setLongAnnotationAnnotator(String annotator, int longAnnotationIndex);
/**
* Set the Description property of LongAnnotation.
*
* @param description Description to set.
* @param longAnnotationIndex the LongAnnotation index.
*/
void setLongAnnotationDescription(String description, int longAnnotationIndex);
/**
* Set the ID property of LongAnnotation.
*
* @param id ID to set.
* @param longAnnotationIndex the LongAnnotation index.
*/
void setLongAnnotationID(String id, int longAnnotationIndex);
/**
* Set the Namespace property of LongAnnotation.
*
* @param namespace Namespace to set.
* @param longAnnotationIndex the LongAnnotation index.
*/
void setLongAnnotationNamespace(String namespace, int longAnnotationIndex);
/**
* Set the Value property of LongAnnotation.
*
* @param value Value to set.
* @param longAnnotationIndex the LongAnnotation index.
*/
void setLongAnnotationValue(Long value, int longAnnotationIndex);
/**
* Set the AnnotationRef property of MapAnnotation.
*
* @param annotation AnnotationRef to set.
* @param mapAnnotationIndex the MapAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setMapAnnotationAnnotationRef(String annotation, int mapAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of MapAnnotation.
*
* @param annotator Annotator to set.
* @param mapAnnotationIndex the MapAnnotation index.
*/
void setMapAnnotationAnnotator(String annotator, int mapAnnotationIndex);
/**
* Set the Description property of MapAnnotation.
*
* @param description Description to set.
* @param mapAnnotationIndex the MapAnnotation index.
*/
void setMapAnnotationDescription(String description, int mapAnnotationIndex);
/**
* Set the ID property of MapAnnotation.
*
* @param id ID to set.
* @param mapAnnotationIndex the MapAnnotation index.
*/
void setMapAnnotationID(String id, int mapAnnotationIndex);
/**
* Set the Namespace property of MapAnnotation.
*
* @param namespace Namespace to set.
* @param mapAnnotationIndex the MapAnnotation index.
*/
void setMapAnnotationNamespace(String namespace, int mapAnnotationIndex);
/**
* Set the AnnotationRef property of Mask.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setMaskAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Mask.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Mask.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Mask.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Mask.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Mask.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the Height property of Mask.
*
* @param height Height to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskHeight(Double height, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Mask.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Mask.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Mask.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Mask.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Mask.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Mask.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Mask.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Mask.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Mask.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Mask.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the Width property of Mask.
*
* @param width Width to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskWidth(Double width, int ROIIndex, int shapeIndex);
/**
* Set the X property of Mask.
*
* @param x X to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskX(Double x, int ROIIndex, int shapeIndex);
/**
* Set the Y property of Mask.
*
* @param y Y to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setMaskY(Double y, int ROIIndex, int shapeIndex);
/**
* Set the Description property of MicrobeamManipulation.
*
* @param description Description to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
*/
void setMicrobeamManipulationDescription(String description, int experimentIndex, int microbeamManipulationIndex);
/**
* Set the ExperimenterRef property of MicrobeamManipulation.
*
* @param experimenter ExperimenterRef to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
*/
void setMicrobeamManipulationExperimenterRef(String experimenter, int experimentIndex, int microbeamManipulationIndex);
/**
* Set the ID property of MicrobeamManipulation.
*
* @param id ID to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
*/
void setMicrobeamManipulationID(String id, int experimentIndex, int microbeamManipulationIndex);
/**
* Set the ROIRef property of MicrobeamManipulation.
*
* @param roi ROIRef to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
* @param ROIRefIndex ROIRef index (unused).
*/
void setMicrobeamManipulationROIRef(String roi, int experimentIndex, int microbeamManipulationIndex, int ROIRefIndex);
/**
* Set the Type property of MicrobeamManipulation.
*
* @param type Type to set.
* @param experimentIndex the Experiment index.
* @param microbeamManipulationIndex the MicrobeamManipulation index.
*/
void setMicrobeamManipulationType(MicrobeamManipulationType type, int experimentIndex, int microbeamManipulationIndex);
/**
* Set the LotNumber property of Microscope.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
*/
void setMicroscopeLotNumber(String lotNumber, int instrumentIndex);
/**
* Set the Manufacturer property of Microscope.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
*/
void setMicroscopeManufacturer(String manufacturer, int instrumentIndex);
/**
* Set the Model property of Microscope.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
*/
void setMicroscopeModel(String model, int instrumentIndex);
/**
* Set the SerialNumber property of Microscope.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
*/
void setMicroscopeSerialNumber(String serialNumber, int instrumentIndex);
/**
* Set the Type property of Microscope.
*
* @param type Type to set.
* @param instrumentIndex the Instrument index.
*/
void setMicroscopeType(MicroscopeType type, int instrumentIndex);
/**
* Set the AnnotationRef property of Objective.
*
* @param annotation AnnotationRef to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setObjectiveAnnotationRef(String annotation, int instrumentIndex, int objectiveIndex, int annotationRefIndex);
/**
* Set the CalibratedMagnification property of Objective.
*
* @param calibratedMagnification CalibratedMagnification to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveCalibratedMagnification(Double calibratedMagnification, int instrumentIndex, int objectiveIndex);
/**
* Set the Correction property of Objective.
*
* @param correction Correction to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveCorrection(Correction correction, int instrumentIndex, int objectiveIndex);
/**
* Set the ID property of Objective.
*
* @param id ID to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveID(String id, int instrumentIndex, int objectiveIndex);
/**
* Set the Immersion property of Objective.
*
* @param immersion Immersion to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveImmersion(Immersion immersion, int instrumentIndex, int objectiveIndex);
/**
* Set the Iris property of Objective.
*
* @param iris Iris to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveIris(Boolean iris, int instrumentIndex, int objectiveIndex);
/**
* Set the LensNA property of Objective.
*
* @param lensNA LensNA to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveLensNA(Double lensNA, int instrumentIndex, int objectiveIndex);
/**
* Set the LotNumber property of Objective.
*
* @param lotNumber LotNumber to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveLotNumber(String lotNumber, int instrumentIndex, int objectiveIndex);
/**
* Set the Manufacturer property of Objective.
*
* @param manufacturer Manufacturer to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveManufacturer(String manufacturer, int instrumentIndex, int objectiveIndex);
/**
* Set the Model property of Objective.
*
* @param model Model to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveModel(String model, int instrumentIndex, int objectiveIndex);
/**
* Set the NominalMagnification property of Objective.
*
* @param nominalMagnification NominalMagnification to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveNominalMagnification(Double nominalMagnification, int instrumentIndex, int objectiveIndex);
/**
* Set the SerialNumber property of Objective.
*
* @param serialNumber SerialNumber to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveSerialNumber(String serialNumber, int instrumentIndex, int objectiveIndex);
/**
* Set the WorkingDistance property of Objective.
*
* @param workingDistance WorkingDistance to set.
* @param instrumentIndex the Instrument index.
* @param objectiveIndex the Objective index.
*/
void setObjectiveWorkingDistance(Length workingDistance, int instrumentIndex, int objectiveIndex);
/**
* Set the CorrectionCollar property of ObjectiveSettings.
*
* @param correctionCollar CorrectionCollar to set.
* @param imageIndex the Image index.
*/
void setObjectiveSettingsCorrectionCollar(Double correctionCollar, int imageIndex);
/**
* Set the ID property of ObjectiveSettings.
*
* @param id ID to set.
* @param imageIndex the Image index.
*/
void setObjectiveSettingsID(String id, int imageIndex);
/**
* Set the Medium property of ObjectiveSettings.
*
* @param medium Medium to set.
* @param imageIndex the Image index.
*/
void setObjectiveSettingsMedium(Medium medium, int imageIndex);
/**
* Set the RefractiveIndex property of ObjectiveSettings.
*
* @param refractiveIndex RefractiveIndex to set.
* @param imageIndex the Image index.
*/
void setObjectiveSettingsRefractiveIndex(Double refractiveIndex, int imageIndex);
/**
* Set the BigEndian property of Pixels.
*
* @param bigEndian BigEndian to set.
* @param imageIndex the Image index.
*/
void setPixelsBigEndian(Boolean bigEndian, int imageIndex);
/**
* Set the DimensionOrder property of Pixels.
*
* @param dimensionOrder DimensionOrder to set.
* @param imageIndex the Image index.
*/
void setPixelsDimensionOrder(DimensionOrder dimensionOrder, int imageIndex);
/**
* Set the ID property of Pixels.
*
* @param id ID to set.
* @param imageIndex the Image index.
*/
void setPixelsID(String id, int imageIndex);
/**
* Set the Interleaved property of Pixels.
*
* @param interleaved Interleaved to set.
* @param imageIndex the Image index.
*/
void setPixelsInterleaved(Boolean interleaved, int imageIndex);
/**
* Set the PhysicalSizeX property of Pixels.
*
* @param physicalSizeX PhysicalSizeX to set.
* @param imageIndex the Image index.
*/
void setPixelsPhysicalSizeX(Length physicalSizeX, int imageIndex);
/**
* Set the PhysicalSizeY property of Pixels.
*
* @param physicalSizeY PhysicalSizeY to set.
* @param imageIndex the Image index.
*/
void setPixelsPhysicalSizeY(Length physicalSizeY, int imageIndex);
/**
* Set the PhysicalSizeZ property of Pixels.
*
* @param physicalSizeZ PhysicalSizeZ to set.
* @param imageIndex the Image index.
*/
void setPixelsPhysicalSizeZ(Length physicalSizeZ, int imageIndex);
/**
* Set the SignificantBits property of Pixels.
*
* @param significantBits SignificantBits to set.
* @param imageIndex the Image index.
*/
void setPixelsSignificantBits(PositiveInteger significantBits, int imageIndex);
/**
* Set the SizeC property of Pixels.
*
* @param sizeC SizeC to set.
* @param imageIndex the Image index.
*/
void setPixelsSizeC(PositiveInteger sizeC, int imageIndex);
/**
* Set the SizeT property of Pixels.
*
* @param sizeT SizeT to set.
* @param imageIndex the Image index.
*/
void setPixelsSizeT(PositiveInteger sizeT, int imageIndex);
/**
* Set the SizeX property of Pixels.
*
* @param sizeX SizeX to set.
* @param imageIndex the Image index.
*/
void setPixelsSizeX(PositiveInteger sizeX, int imageIndex);
/**
* Set the SizeY property of Pixels.
*
* @param sizeY SizeY to set.
* @param imageIndex the Image index.
*/
void setPixelsSizeY(PositiveInteger sizeY, int imageIndex);
/**
* Set the SizeZ property of Pixels.
*
* @param sizeZ SizeZ to set.
* @param imageIndex the Image index.
*/
void setPixelsSizeZ(PositiveInteger sizeZ, int imageIndex);
/**
* Set the TimeIncrement property of Pixels.
*
* @param timeIncrement TimeIncrement to set.
* @param imageIndex the Image index.
*/
void setPixelsTimeIncrement(Time timeIncrement, int imageIndex);
/**
* Set the Type property of Pixels.
*
* @param type Type to set.
* @param imageIndex the Image index.
*/
void setPixelsType(PixelType type, int imageIndex);
/**
* Set the AnnotationRef property of Plane.
*
* @param annotation AnnotationRef to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setPlaneAnnotationRef(String annotation, int imageIndex, int planeIndex, int annotationRefIndex);
/**
* Set the DeltaT property of Plane.
*
* @param deltaT DeltaT to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlaneDeltaT(Time deltaT, int imageIndex, int planeIndex);
/**
* Set the ExposureTime property of Plane.
*
* @param exposureTime ExposureTime to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlaneExposureTime(Time exposureTime, int imageIndex, int planeIndex);
/**
* Set the HashSHA1 property of Plane.
*
* @param hashSHA1 HashSHA1 to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlaneHashSHA1(String hashSHA1, int imageIndex, int planeIndex);
/**
* Set the PositionX property of Plane.
*
* @param positionX PositionX to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlanePositionX(Length positionX, int imageIndex, int planeIndex);
/**
* Set the PositionY property of Plane.
*
* @param positionY PositionY to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlanePositionY(Length positionY, int imageIndex, int planeIndex);
/**
* Set the PositionZ property of Plane.
*
* @param positionZ PositionZ to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlanePositionZ(Length positionZ, int imageIndex, int planeIndex);
/**
* Set the TheC property of Plane.
*
* @param theC TheC to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlaneTheC(NonNegativeInteger theC, int imageIndex, int planeIndex);
/**
* Set the TheT property of Plane.
*
* @param theT TheT to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlaneTheT(NonNegativeInteger theT, int imageIndex, int planeIndex);
/**
* Set the TheZ property of Plane.
*
* @param theZ TheZ to set.
* @param imageIndex the Image index.
* @param planeIndex the Plane index.
*/
void setPlaneTheZ(NonNegativeInteger theZ, int imageIndex, int planeIndex);
/**
* Set the AnnotationRef property of Plate.
*
* @param annotation AnnotationRef to set.
* @param plateIndex the Plate index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setPlateAnnotationRef(String annotation, int plateIndex, int annotationRefIndex);
/**
* Set the ColumnNamingConvention property of Plate.
*
* @param columnNamingConvention ColumnNamingConvention to set.
* @param plateIndex the Plate index.
*/
void setPlateColumnNamingConvention(NamingConvention columnNamingConvention, int plateIndex);
/**
* Set the Columns property of Plate.
*
* @param columns Columns to set.
* @param plateIndex the Plate index.
*/
void setPlateColumns(PositiveInteger columns, int plateIndex);
/**
* Set the Description property of Plate.
*
* @param description Description to set.
* @param plateIndex the Plate index.
*/
void setPlateDescription(String description, int plateIndex);
/**
* Set the ExternalIdentifier property of Plate.
*
* @param externalIdentifier ExternalIdentifier to set.
* @param plateIndex the Plate index.
*/
void setPlateExternalIdentifier(String externalIdentifier, int plateIndex);
/**
* Set the FieldIndex property of Plate.
*
* @param fieldIndex FieldIndex to set.
* @param plateIndex the Plate index.
*/
void setPlateFieldIndex(NonNegativeInteger fieldIndex, int plateIndex);
/**
* Set the ID property of Plate.
*
* @param id ID to set.
* @param plateIndex the Plate index.
*/
void setPlateID(String id, int plateIndex);
/**
* Set the Name property of Plate.
*
* @param name Name to set.
* @param plateIndex the Plate index.
*/
void setPlateName(String name, int plateIndex);
/**
* Set the RowNamingConvention property of Plate.
*
* @param rowNamingConvention RowNamingConvention to set.
* @param plateIndex the Plate index.
*/
void setPlateRowNamingConvention(NamingConvention rowNamingConvention, int plateIndex);
/**
* Set the Rows property of Plate.
*
* @param rows Rows to set.
* @param plateIndex the Plate index.
*/
void setPlateRows(PositiveInteger rows, int plateIndex);
/**
* Set the Status property of Plate.
*
* @param status Status to set.
* @param plateIndex the Plate index.
*/
void setPlateStatus(String status, int plateIndex);
/**
* Set the WellOriginX property of Plate.
*
* @param wellOriginX WellOriginX to set.
* @param plateIndex the Plate index.
*/
void setPlateWellOriginX(Length wellOriginX, int plateIndex);
/**
* Set the WellOriginY property of Plate.
*
* @param wellOriginY WellOriginY to set.
* @param plateIndex the Plate index.
*/
void setPlateWellOriginY(Length wellOriginY, int plateIndex);
/**
* Set the AnnotationRef property of PlateAcquisition.
*
* @param annotation AnnotationRef to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setPlateAcquisitionAnnotationRef(String annotation, int plateIndex, int plateAcquisitionIndex, int annotationRefIndex);
/**
* Set the Description property of PlateAcquisition.
*
* @param description Description to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
*/
void setPlateAcquisitionDescription(String description, int plateIndex, int plateAcquisitionIndex);
/**
* Set the EndTime property of PlateAcquisition.
*
* @param endTime EndTime to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
*/
void setPlateAcquisitionEndTime(Timestamp endTime, int plateIndex, int plateAcquisitionIndex);
/**
* Set the ID property of PlateAcquisition.
*
* @param id ID to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
*/
void setPlateAcquisitionID(String id, int plateIndex, int plateAcquisitionIndex);
/**
* Set the MaximumFieldCount property of PlateAcquisition.
*
* @param maximumFieldCount MaximumFieldCount to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
*/
void setPlateAcquisitionMaximumFieldCount(PositiveInteger maximumFieldCount, int plateIndex, int plateAcquisitionIndex);
/**
* Set the Name property of PlateAcquisition.
*
* @param name Name to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
*/
void setPlateAcquisitionName(String name, int plateIndex, int plateAcquisitionIndex);
/**
* Set the StartTime property of PlateAcquisition.
*
* @param startTime StartTime to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
*/
void setPlateAcquisitionStartTime(Timestamp startTime, int plateIndex, int plateAcquisitionIndex);
/**
* Set the WellSampleRef property of PlateAcquisition.
*
* @param wellSample WellSampleRef to set.
* @param plateIndex the Plate index.
* @param plateAcquisitionIndex the PlateAcquisition index.
* @param wellSampleRefIndex WellSampleRef index (unused).
*/
void setPlateAcquisitionWellSampleRef(String wellSample, int plateIndex, int plateAcquisitionIndex, int wellSampleRefIndex);
/**
* Set the AnnotationRef property of Point.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setPointAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Point.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Point.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Point.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Point.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Point.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Point.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Point.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Point.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Point.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Point.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Point.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Point.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Point.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Point.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Point.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the X property of Point.
*
* @param x X to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointX(Double x, int ROIIndex, int shapeIndex);
/**
* Set the Y property of Point.
*
* @param y Y to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPointY(Double y, int ROIIndex, int shapeIndex);
/**
* Set the AnnotationRef property of Polygon.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setPolygonAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Polygon.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Polygon.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Polygon.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Polygon.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Polygon.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Polygon.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Polygon.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the Points property of Polygon.
*
* @param points Points to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonPoints(String points, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Polygon.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Polygon.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Polygon.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Polygon.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Polygon.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Polygon.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Polygon.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Polygon.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolygonTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the AnnotationRef property of Polyline.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setPolylineAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Polyline.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Polyline.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Polyline.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Polyline.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Polyline.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Polyline.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Polyline.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the MarkerEnd property of Polyline.
*
* @param markerEnd MarkerEnd to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineMarkerEnd(Marker markerEnd, int ROIIndex, int shapeIndex);
/**
* Set the MarkerStart property of Polyline.
*
* @param markerStart MarkerStart to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineMarkerStart(Marker markerStart, int ROIIndex, int shapeIndex);
/**
* Set the Points property of Polyline.
*
* @param points Points to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylinePoints(String points, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Polyline.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Polyline.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Polyline.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Polyline.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Polyline.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Polyline.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Polyline.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Polyline.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setPolylineTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the AnnotationRef property of Project.
*
* @param annotation AnnotationRef to set.
* @param projectIndex the Project index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setProjectAnnotationRef(String annotation, int projectIndex, int annotationRefIndex);
/**
* Set the DatasetRef property of Project.
*
* @param dataset DatasetRef to set.
* @param projectIndex the Project index.
* @param datasetRefIndex DatasetRef index (unused).
*/
void setProjectDatasetRef(String dataset, int projectIndex, int datasetRefIndex);
/**
* Set the Description property of Project.
*
* @param description Description to set.
* @param projectIndex the Project index.
*/
void setProjectDescription(String description, int projectIndex);
/**
* Set the ExperimenterGroupRef property of Project.
*
* @param experimenterGroup ExperimenterGroupRef to set.
* @param projectIndex the Project index.
*/
void setProjectExperimenterGroupRef(String experimenterGroup, int projectIndex);
/**
* Set the ExperimenterRef property of Project.
*
* @param experimenter ExperimenterRef to set.
* @param projectIndex the Project index.
*/
void setProjectExperimenterRef(String experimenter, int projectIndex);
/**
* Set the ID property of Project.
*
* @param id ID to set.
* @param projectIndex the Project index.
*/
void setProjectID(String id, int projectIndex);
/**
* Set the Name property of Project.
*
* @param name Name to set.
* @param projectIndex the Project index.
*/
void setProjectName(String name, int projectIndex);
/**
* Set the AnnotationRef property of ROI.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setROIAnnotationRef(String annotation, int ROIIndex, int annotationRefIndex);
/**
* Set the Description property of ROI.
*
* @param description Description to set.
* @param ROIIndex the ROI index.
*/
void setROIDescription(String description, int ROIIndex);
/**
* Set the ID property of ROI.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
*/
void setROIID(String id, int ROIIndex);
/**
* Set the Name property of ROI.
*
* @param name Name to set.
* @param ROIIndex the ROI index.
*/
void setROIName(String name, int ROIIndex);
/**
* Set the AnnotationRef property of Reagent.
*
* @param annotation AnnotationRef to set.
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setReagentAnnotationRef(String annotation, int screenIndex, int reagentIndex, int annotationRefIndex);
/**
* Set the Description property of Reagent.
*
* @param description Description to set.
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
*/
void setReagentDescription(String description, int screenIndex, int reagentIndex);
/**
* Set the ID property of Reagent.
*
* @param id ID to set.
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
*/
void setReagentID(String id, int screenIndex, int reagentIndex);
/**
* Set the Name property of Reagent.
*
* @param name Name to set.
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
*/
void setReagentName(String name, int screenIndex, int reagentIndex);
/**
* Set the ReagentIdentifier property of Reagent.
*
* @param reagentIdentifier ReagentIdentifier to set.
* @param screenIndex the Screen index.
* @param reagentIndex the Reagent index.
*/
void setReagentReagentIdentifier(String reagentIdentifier, int screenIndex, int reagentIndex);
/**
* Set the AnnotationRef property of Rectangle.
*
* @param annotation AnnotationRef to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setRectangleAnnotationRef(String annotation, int ROIIndex, int shapeIndex, int annotationRefIndex);
/**
* Set the FillColor property of Rectangle.
*
* @param fillColor FillColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleFillColor(Color fillColor, int ROIIndex, int shapeIndex);
/**
* Set the FillRule property of Rectangle.
*
* @param fillRule FillRule to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleFillRule(FillRule fillRule, int ROIIndex, int shapeIndex);
/**
* Set the FontFamily property of Rectangle.
*
* @param fontFamily FontFamily to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleFontFamily(FontFamily fontFamily, int ROIIndex, int shapeIndex);
/**
* Set the FontSize property of Rectangle.
*
* @param fontSize FontSize to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleFontSize(Length fontSize, int ROIIndex, int shapeIndex);
/**
* Set the FontStyle property of Rectangle.
*
* @param fontStyle FontStyle to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleFontStyle(FontStyle fontStyle, int ROIIndex, int shapeIndex);
/**
* Set the Height property of Rectangle.
*
* @param height Height to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleHeight(Double height, int ROIIndex, int shapeIndex);
/**
* Set the ID property of Rectangle.
*
* @param id ID to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleID(String id, int ROIIndex, int shapeIndex);
/**
* Set the Locked property of Rectangle.
*
* @param locked Locked to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleLocked(Boolean locked, int ROIIndex, int shapeIndex);
/**
* Set the StrokeColor property of Rectangle.
*
* @param strokeColor StrokeColor to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleStrokeColor(Color strokeColor, int ROIIndex, int shapeIndex);
/**
* Set the StrokeDashArray property of Rectangle.
*
* @param strokeDashArray StrokeDashArray to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleStrokeDashArray(String strokeDashArray, int ROIIndex, int shapeIndex);
/**
* Set the StrokeWidth property of Rectangle.
*
* @param strokeWidth StrokeWidth to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleStrokeWidth(Length strokeWidth, int ROIIndex, int shapeIndex);
/**
* Set the Text property of Rectangle.
*
* @param text Text to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleText(String text, int ROIIndex, int shapeIndex);
/**
* Set the TheC property of Rectangle.
*
* @param theC TheC to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleTheC(NonNegativeInteger theC, int ROIIndex, int shapeIndex);
/**
* Set the TheT property of Rectangle.
*
* @param theT TheT to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleTheT(NonNegativeInteger theT, int ROIIndex, int shapeIndex);
/**
* Set the TheZ property of Rectangle.
*
* @param theZ TheZ to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleTheZ(NonNegativeInteger theZ, int ROIIndex, int shapeIndex);
/**
* Set the Transform property of Rectangle.
*
* @param transform Transform to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleTransform(AffineTransform transform, int ROIIndex, int shapeIndex);
/**
* Set the Width property of Rectangle.
*
* @param width Width to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleWidth(Double width, int ROIIndex, int shapeIndex);
/**
* Set the X property of Rectangle.
*
* @param x X to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleX(Double x, int ROIIndex, int shapeIndex);
/**
* Set the Y property of Rectangle.
*
* @param y Y to set.
* @param ROIIndex the ROI index.
* @param shapeIndex the Shape index.
*/
void setRectangleY(Double y, int ROIIndex, int shapeIndex);
/**
* Set the RightsHeld property of Rights.
*
* @param rightsHeld RightsHeld to set.
*/
void setRightsRightsHeld(String rightsHeld);
/**
* Set the RightsHolder property of Rights.
*
* @param rightsHolder RightsHolder to set.
*/
void setRightsRightsHolder(String rightsHolder);
/**
* Set the AnnotationRef property of Screen.
*
* @param annotation AnnotationRef to set.
* @param screenIndex the Screen index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setScreenAnnotationRef(String annotation, int screenIndex, int annotationRefIndex);
/**
* Set the Description property of Screen.
*
* @param description Description to set.
* @param screenIndex the Screen index.
*/
void setScreenDescription(String description, int screenIndex);
/**
* Set the ID property of Screen.
*
* @param id ID to set.
* @param screenIndex the Screen index.
*/
void setScreenID(String id, int screenIndex);
/**
* Set the Name property of Screen.
*
* @param name Name to set.
* @param screenIndex the Screen index.
*/
void setScreenName(String name, int screenIndex);
/**
* Set the PlateRef property of Screen.
*
* @param plate PlateRef to set.
* @param screenIndex the Screen index.
* @param plateRefIndex PlateRef index (unused).
*/
void setScreenPlateRef(String plate, int screenIndex, int plateRefIndex);
/**
* Set the ProtocolDescription property of Screen.
*
* @param protocolDescription ProtocolDescription to set.
* @param screenIndex the Screen index.
*/
void setScreenProtocolDescription(String protocolDescription, int screenIndex);
/**
* Set the ProtocolIdentifier property of Screen.
*
* @param protocolIdentifier ProtocolIdentifier to set.
* @param screenIndex the Screen index.
*/
void setScreenProtocolIdentifier(String protocolIdentifier, int screenIndex);
/**
* Set the ReagentSetDescription property of Screen.
*
* @param reagentSetDescription ReagentSetDescription to set.
* @param screenIndex the Screen index.
*/
void setScreenReagentSetDescription(String reagentSetDescription, int screenIndex);
/**
* Set the ReagentSetIdentifier property of Screen.
*
* @param reagentSetIdentifier ReagentSetIdentifier to set.
* @param screenIndex the Screen index.
*/
void setScreenReagentSetIdentifier(String reagentSetIdentifier, int screenIndex);
/**
* Set the Type property of Screen.
*
* @param type Type to set.
* @param screenIndex the Screen index.
*/
void setScreenType(String type, int screenIndex);
/**
* Set the Name property of StageLabel.
*
* @param name Name to set.
* @param imageIndex the Image index.
*/
void setStageLabelName(String name, int imageIndex);
/**
* Set the X property of StageLabel.
*
* @param x X to set.
* @param imageIndex the Image index.
*/
void setStageLabelX(Length x, int imageIndex);
/**
* Set the Y property of StageLabel.
*
* @param y Y to set.
* @param imageIndex the Image index.
*/
void setStageLabelY(Length y, int imageIndex);
/**
* Set the Z property of StageLabel.
*
* @param z Z to set.
* @param imageIndex the Image index.
*/
void setStageLabelZ(Length z, int imageIndex);
/**
* Set the AnnotationRef property of TagAnnotation.
*
* @param annotation AnnotationRef to set.
* @param tagAnnotationIndex the TagAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setTagAnnotationAnnotationRef(String annotation, int tagAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of TagAnnotation.
*
* @param annotator Annotator to set.
* @param tagAnnotationIndex the TagAnnotation index.
*/
void setTagAnnotationAnnotator(String annotator, int tagAnnotationIndex);
/**
* Set the Description property of TagAnnotation.
*
* @param description Description to set.
* @param tagAnnotationIndex the TagAnnotation index.
*/
void setTagAnnotationDescription(String description, int tagAnnotationIndex);
/**
* Set the ID property of TagAnnotation.
*
* @param id ID to set.
* @param tagAnnotationIndex the TagAnnotation index.
*/
void setTagAnnotationID(String id, int tagAnnotationIndex);
/**
* Set the Namespace property of TagAnnotation.
*
* @param namespace Namespace to set.
* @param tagAnnotationIndex the TagAnnotation index.
*/
void setTagAnnotationNamespace(String namespace, int tagAnnotationIndex);
/**
* Set the Value property of TagAnnotation.
*
* @param value Value to set.
* @param tagAnnotationIndex the TagAnnotation index.
*/
void setTagAnnotationValue(String value, int tagAnnotationIndex);
/**
* Set the AnnotationRef property of TermAnnotation.
*
* @param annotation AnnotationRef to set.
* @param termAnnotationIndex the TermAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setTermAnnotationAnnotationRef(String annotation, int termAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of TermAnnotation.
*
* @param annotator Annotator to set.
* @param termAnnotationIndex the TermAnnotation index.
*/
void setTermAnnotationAnnotator(String annotator, int termAnnotationIndex);
/**
* Set the Description property of TermAnnotation.
*
* @param description Description to set.
* @param termAnnotationIndex the TermAnnotation index.
*/
void setTermAnnotationDescription(String description, int termAnnotationIndex);
/**
* Set the ID property of TermAnnotation.
*
* @param id ID to set.
* @param termAnnotationIndex the TermAnnotation index.
*/
void setTermAnnotationID(String id, int termAnnotationIndex);
/**
* Set the Namespace property of TermAnnotation.
*
* @param namespace Namespace to set.
* @param termAnnotationIndex the TermAnnotation index.
*/
void setTermAnnotationNamespace(String namespace, int termAnnotationIndex);
/**
* Set the Value property of TermAnnotation.
*
* @param value Value to set.
* @param termAnnotationIndex the TermAnnotation index.
*/
void setTermAnnotationValue(String value, int termAnnotationIndex);
/**
* Set the FirstC property of TiffData.
*
* @param firstC FirstC to set.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setTiffDataFirstC(NonNegativeInteger firstC, int imageIndex, int tiffDataIndex);
/**
* Set the FirstT property of TiffData.
*
* @param firstT FirstT to set.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setTiffDataFirstT(NonNegativeInteger firstT, int imageIndex, int tiffDataIndex);
/**
* Set the FirstZ property of TiffData.
*
* @param firstZ FirstZ to set.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setTiffDataFirstZ(NonNegativeInteger firstZ, int imageIndex, int tiffDataIndex);
/**
* Set the IFD property of TiffData.
*
* @param ifd IFD to set.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setTiffDataIFD(NonNegativeInteger ifd, int imageIndex, int tiffDataIndex);
/**
* Set the PlaneCount property of TiffData.
*
* @param planeCount PlaneCount to set.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setTiffDataPlaneCount(NonNegativeInteger planeCount, int imageIndex, int tiffDataIndex);
/**
* Set the AnnotationRef property of TimestampAnnotation.
*
* @param annotation AnnotationRef to set.
* @param timestampAnnotationIndex the TimestampAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setTimestampAnnotationAnnotationRef(String annotation, int timestampAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of TimestampAnnotation.
*
* @param annotator Annotator to set.
* @param timestampAnnotationIndex the TimestampAnnotation index.
*/
void setTimestampAnnotationAnnotator(String annotator, int timestampAnnotationIndex);
/**
* Set the Description property of TimestampAnnotation.
*
* @param description Description to set.
* @param timestampAnnotationIndex the TimestampAnnotation index.
*/
void setTimestampAnnotationDescription(String description, int timestampAnnotationIndex);
/**
* Set the ID property of TimestampAnnotation.
*
* @param id ID to set.
* @param timestampAnnotationIndex the TimestampAnnotation index.
*/
void setTimestampAnnotationID(String id, int timestampAnnotationIndex);
/**
* Set the Namespace property of TimestampAnnotation.
*
* @param namespace Namespace to set.
* @param timestampAnnotationIndex the TimestampAnnotation index.
*/
void setTimestampAnnotationNamespace(String namespace, int timestampAnnotationIndex);
/**
* Set the Value property of TimestampAnnotation.
*
* @param value Value to set.
* @param timestampAnnotationIndex the TimestampAnnotation index.
*/
void setTimestampAnnotationValue(Timestamp value, int timestampAnnotationIndex);
/**
* Set the CutIn property of TransmittanceRange.
*
* @param cutIn CutIn to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setTransmittanceRangeCutIn(Length cutIn, int instrumentIndex, int filterIndex);
/**
* Set the CutInTolerance property of TransmittanceRange.
*
* @param cutInTolerance CutInTolerance to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setTransmittanceRangeCutInTolerance(Length cutInTolerance, int instrumentIndex, int filterIndex);
/**
* Set the CutOut property of TransmittanceRange.
*
* @param cutOut CutOut to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setTransmittanceRangeCutOut(Length cutOut, int instrumentIndex, int filterIndex);
/**
* Set the CutOutTolerance property of TransmittanceRange.
*
* @param cutOutTolerance CutOutTolerance to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setTransmittanceRangeCutOutTolerance(Length cutOutTolerance, int instrumentIndex, int filterIndex);
/**
* Set the Transmittance property of TransmittanceRange.
*
* @param transmittance Transmittance to set.
* @param instrumentIndex the Instrument index.
* @param filterIndex the Filter index.
*/
void setTransmittanceRangeTransmittance(PercentFraction transmittance, int instrumentIndex, int filterIndex);
/**
* Set the text value of UUID.
*
* @param value text value.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setUUIDValue(String value, int imageIndex, int tiffDataIndex);
/**
* Set the FileName property of UUID.
*
* @param fileName FileName to set.
* @param imageIndex the Image index.
* @param tiffDataIndex the TiffData index.
*/
void setUUIDFileName(String fileName, int imageIndex, int tiffDataIndex);
/**
* Set the AnnotationRef property of Well.
*
* @param annotation AnnotationRef to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setWellAnnotationRef(String annotation, int plateIndex, int wellIndex, int annotationRefIndex);
/**
* Set the Color property of Well.
*
* @param color Color to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellColor(Color color, int plateIndex, int wellIndex);
/**
* Set the Column property of Well.
*
* @param column Column to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellColumn(NonNegativeInteger column, int plateIndex, int wellIndex);
/**
* Set the ExternalDescription property of Well.
*
* @param externalDescription ExternalDescription to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellExternalDescription(String externalDescription, int plateIndex, int wellIndex);
/**
* Set the ExternalIdentifier property of Well.
*
* @param externalIdentifier ExternalIdentifier to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellExternalIdentifier(String externalIdentifier, int plateIndex, int wellIndex);
/**
* Set the ID property of Well.
*
* @param id ID to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellID(String id, int plateIndex, int wellIndex);
/**
* Set the ReagentRef property of Well.
*
* @param reagent ReagentRef to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellReagentRef(String reagent, int plateIndex, int wellIndex);
/**
* Set the Row property of Well.
*
* @param row Row to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellRow(NonNegativeInteger row, int plateIndex, int wellIndex);
/**
* Set the Type property of Well.
*
* @param type Type to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
*/
void setWellType(String type, int plateIndex, int wellIndex);
/**
* Set the ID property of WellSample.
*
* @param id ID to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
*/
void setWellSampleID(String id, int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Set the ImageRef property of WellSample.
*
* @param image ImageRef to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
*/
void setWellSampleImageRef(String image, int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Set the Index property of WellSample.
*
* @param index Index to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
*/
void setWellSampleIndex(NonNegativeInteger index, int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Set the PositionX property of WellSample.
*
* @param positionX PositionX to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
*/
void setWellSamplePositionX(Length positionX, int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Set the PositionY property of WellSample.
*
* @param positionY PositionY to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
*/
void setWellSamplePositionY(Length positionY, int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Set the Timepoint property of WellSample.
*
* @param timepoint Timepoint to set.
* @param plateIndex the Plate index.
* @param wellIndex the Well index.
* @param wellSampleIndex the WellSample index.
*/
void setWellSampleTimepoint(Timestamp timepoint, int plateIndex, int wellIndex, int wellSampleIndex);
/**
* Set the AnnotationRef property of XMLAnnotation.
*
* @param annotation AnnotationRef to set.
* @param XMLAnnotationIndex the XMLAnnotation index.
* @param annotationRefIndex AnnotationRef index (unused).
*/
void setXMLAnnotationAnnotationRef(String annotation, int XMLAnnotationIndex, int annotationRefIndex);
/**
* Set the Annotator property of XMLAnnotation.
*
* @param annotator Annotator to set.
* @param XMLAnnotationIndex the XMLAnnotation index.
*/
void setXMLAnnotationAnnotator(String annotator, int XMLAnnotationIndex);
/**
* Set the Description property of XMLAnnotation.
*
* @param description Description to set.
* @param XMLAnnotationIndex the XMLAnnotation index.
*/
void setXMLAnnotationDescription(String description, int XMLAnnotationIndex);
/**
* Set the ID property of XMLAnnotation.
*
* @param id ID to set.
* @param XMLAnnotationIndex the XMLAnnotation index.
*/
void setXMLAnnotationID(String id, int XMLAnnotationIndex);
/**
* Set the Namespace property of XMLAnnotation.
*
* @param namespace Namespace to set.
* @param XMLAnnotationIndex the XMLAnnotation index.
*/
void setXMLAnnotationNamespace(String namespace, int XMLAnnotationIndex);
/**
* Set the Value property of XMLAnnotation.
*
* @param value Value to set.
* @param XMLAnnotationIndex the XMLAnnotation index.
*/
void setXMLAnnotationValue(String value, int XMLAnnotationIndex);
}