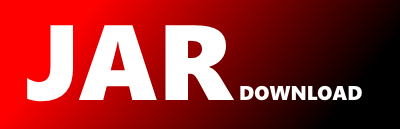
ome.xml.model.Annotation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ome-xml Show documentation
Show all versions of ome-xml Show documentation
A library for working with OME-XML metadata structures.
The newest version!
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.model;
import java.util.ArrayList;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import ome.xml.model.enums.*;
import ome.xml.model.enums.handlers.*;
import ome.xml.model.primitives.*;
import ome.units.quantity.*;
import ome.units.unit.Unit;
public abstract class Annotation extends AbstractOMEModelObject
{
// Base: -- Name: Annotation -- Type: Annotation -- modelBaseType: AbstractOMEModelObject -- langBaseType: Object
// -- Constants --
public static final String NAMESPACE = "http://www.openmicroscopy.org/Schemas/OME/2016-06";
/** Logger for this class. */
private static final Logger LOGGER =
LoggerFactory.getLogger(Annotation.class);
// -- Instance variables --
// ID property
private String id;
// Namespace property
private String namespace;
// Annotator property
private String annotator;
// Description property
private String description;
// AnnotationRef reference (occurs more than once)
private List annotationLinks = new ReferenceList<>();
// Image_BackReference back reference (occurs more than once)
private List imageLinks = new ReferenceList<>();
// Plane_BackReference back reference (occurs more than once)
private List planeLinks = new ReferenceList<>();
// Channel_BackReference back reference (occurs more than once)
private List channelLinks = new ReferenceList<>();
// Instrument_BackReference back reference (occurs more than once)
private List instrumentLinks = new ReferenceList<>();
// LightSource_BackReference back reference (occurs more than once)
private List lightSourceLinks = new ReferenceList<>();
// Project_BackReference back reference (occurs more than once)
private List projectLinks = new ReferenceList<>();
// ExperimenterGroup_BackReference back reference (occurs more than once)
private List experimenterGroupLinks = new ReferenceList<>();
// Dataset_BackReference back reference (occurs more than once)
private List datasetLinks = new ReferenceList<>();
// Experimenter_BackReference back reference (occurs more than once)
private List experimenterLinks = new ReferenceList<>();
// Folder_BackReference back reference (occurs more than once)
private List folderLinks = new ReferenceList<>();
// Objective_BackReference back reference (occurs more than once)
private List objectiveLinks = new ReferenceList<>();
// Detector_BackReference back reference (occurs more than once)
private List detectorLinks = new ReferenceList<>();
// Filter_BackReference back reference (occurs more than once)
private List filterLinks = new ReferenceList<>();
// Dichroic_BackReference back reference (occurs more than once)
private List dichroicLinks = new ReferenceList<>();
// LightPath_BackReference back reference (occurs more than once)
private List lightPathLinks = new ReferenceList<>();
// ROI_BackReference back reference (occurs more than once)
private List roiLinks = new ReferenceList<>();
// Shape_BackReference back reference (occurs more than once)
private List shapeLinks = new ReferenceList<>();
// Plate_BackReference back reference (occurs more than once)
private List plateLinks = new ReferenceList<>();
// Reagent_BackReference back reference (occurs more than once)
private List reagentLinks = new ReferenceList<>();
// Screen_BackReference back reference (occurs more than once)
private List screenLinks = new ReferenceList<>();
// PlateAcquisition_BackReference back reference (occurs more than once)
private List plateAcquisitionLinks = new ReferenceList<>();
// Well_BackReference back reference (occurs more than once)
private List wellLinks = new ReferenceList<>();
// -- Constructors --
/** Default constructor. */
public Annotation()
{
}
/**
* Constructs Annotation recursively from an XML DOM tree.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public Annotation(Element element, OMEModel model)
throws EnumerationException
{
update(element, model);
}
/** Copy constructor. */
public Annotation(Annotation orig)
{
id = orig.id;
namespace = orig.namespace;
annotator = orig.annotator;
description = orig.description;
annotationLinks = orig.annotationLinks;
imageLinks = orig.imageLinks;
planeLinks = orig.planeLinks;
channelLinks = orig.channelLinks;
instrumentLinks = orig.instrumentLinks;
lightSourceLinks = orig.lightSourceLinks;
projectLinks = orig.projectLinks;
experimenterGroupLinks = orig.experimenterGroupLinks;
datasetLinks = orig.datasetLinks;
experimenterLinks = orig.experimenterLinks;
folderLinks = orig.folderLinks;
objectiveLinks = orig.objectiveLinks;
detectorLinks = orig.detectorLinks;
filterLinks = orig.filterLinks;
dichroicLinks = orig.dichroicLinks;
lightPathLinks = orig.lightPathLinks;
roiLinks = orig.roiLinks;
shapeLinks = orig.shapeLinks;
plateLinks = orig.plateLinks;
reagentLinks = orig.reagentLinks;
screenLinks = orig.screenLinks;
plateAcquisitionLinks = orig.plateAcquisitionLinks;
wellLinks = orig.wellLinks;
}
// -- Custom content from Annotation specific template --
// -- OMEModelObject API methods --
/**
* Updates Annotation recursively from an XML DOM tree. NOTE: No
* properties are removed, only added or updated.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public void update(Element element, OMEModel model)
throws EnumerationException
{
super.update(element, model);
if (!element.hasAttribute("ID") && getID() == null)
{
// TODO: Should be its own exception
throw new RuntimeException(
"Annotation missing required ID property.");
}
if (element.hasAttribute("ID"))
{
// ID property
setID(String.valueOf(
element.getAttribute("ID")));
// Adding this model object to the model handler
model.addModelObject(getID(), this);
}
if (element.hasAttribute("Namespace"))
{
// Attribute property Namespace
setNamespace(String.valueOf(
element.getAttribute("Namespace")));
}
if (element.hasAttribute("Annotator"))
{
// Attribute property Annotator
setAnnotator(String.valueOf(
element.getAttribute("Annotator")));
}
List Description_nodeList =
getChildrenByTagName(element, "Description");
if (Description_nodeList.size() > 1)
{
// TODO: Should be its own Exception
throw new RuntimeException(String.format(
"Description node list size %d != 1",
Description_nodeList.size()));
}
else if (Description_nodeList.size() != 0)
{
// Element property Description which is not complex (has no
// sub-elements)
setDescription(
String.valueOf(Description_nodeList.get(0).getTextContent()));
}
// Element reference AnnotationRef
List AnnotationRef_nodeList =
getChildrenByTagName(element, "AnnotationRef");
for (Element AnnotationRef_element : AnnotationRef_nodeList)
{
AnnotationRef annotationLinks_reference = new AnnotationRef();
annotationLinks_reference.setID(AnnotationRef_element.getAttribute("ID"));
model.addReference(this, annotationLinks_reference);
}
}
// -- Annotation API methods --
public boolean link(Reference reference, OMEModelObject o)
{
if (reference instanceof AnnotationRef)
{
Annotation o_casted = (Annotation) o;
annotationLinks.add(o_casted);
return true;
}
return super.link(reference, o);
}
// Property ID
public String getID()
{
return id;
}
public void setID(String id)
{
this.id = id;
}
// Property Namespace
public String getNamespace()
{
return namespace;
}
public void setNamespace(String namespace)
{
this.namespace = namespace;
}
// Property Annotator
public String getAnnotator()
{
return annotator;
}
public void setAnnotator(String annotator)
{
this.annotator = annotator;
}
// Property Description
public String getDescription()
{
return description;
}
public void setDescription(String description)
{
this.description = description;
}
// Reference which occurs more than once
public int sizeOfLinkedAnnotationList()
{
return annotationLinks.size();
}
public List copyLinkedAnnotationList()
{
return new ArrayList<>(annotationLinks);
}
public Annotation getLinkedAnnotation(int index)
{
return annotationLinks.get(index);
}
public Annotation setLinkedAnnotation(int index, Annotation o)
{
return annotationLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkAnnotation(Annotation o)
{
return annotationLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkAnnotation(Annotation o)
{
return annotationLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedImageList()
{
return imageLinks.size();
}
public List copyLinkedImageList()
{
return new ArrayList<>(imageLinks);
}
public Image getLinkedImage(int index)
{
return imageLinks.get(index);
}
public Image setLinkedImage(int index, Image o)
{
return imageLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkImage(Image o)
{
return imageLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkImage(Image o)
{
return imageLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedPlaneList()
{
return planeLinks.size();
}
public List copyLinkedPlaneList()
{
return new ArrayList<>(planeLinks);
}
public Plane getLinkedPlane(int index)
{
return planeLinks.get(index);
}
public Plane setLinkedPlane(int index, Plane o)
{
return planeLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkPlane(Plane o)
{
return planeLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkPlane(Plane o)
{
return planeLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedChannelList()
{
return channelLinks.size();
}
public List copyLinkedChannelList()
{
return new ArrayList<>(channelLinks);
}
public Channel getLinkedChannel(int index)
{
return channelLinks.get(index);
}
public Channel setLinkedChannel(int index, Channel o)
{
return channelLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkChannel(Channel o)
{
return channelLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkChannel(Channel o)
{
return channelLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedInstrumentList()
{
return instrumentLinks.size();
}
public List copyLinkedInstrumentList()
{
return new ArrayList<>(instrumentLinks);
}
public Instrument getLinkedInstrument(int index)
{
return instrumentLinks.get(index);
}
public Instrument setLinkedInstrument(int index, Instrument o)
{
return instrumentLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkInstrument(Instrument o)
{
return instrumentLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkInstrument(Instrument o)
{
return instrumentLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedLightSourceList()
{
return lightSourceLinks.size();
}
public List copyLinkedLightSourceList()
{
return new ArrayList<>(lightSourceLinks);
}
public LightSource getLinkedLightSource(int index)
{
return lightSourceLinks.get(index);
}
public LightSource setLinkedLightSource(int index, LightSource o)
{
return lightSourceLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkLightSource(LightSource o)
{
return lightSourceLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkLightSource(LightSource o)
{
return lightSourceLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedProjectList()
{
return projectLinks.size();
}
public List copyLinkedProjectList()
{
return new ArrayList<>(projectLinks);
}
public Project getLinkedProject(int index)
{
return projectLinks.get(index);
}
public Project setLinkedProject(int index, Project o)
{
return projectLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkProject(Project o)
{
return projectLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkProject(Project o)
{
return projectLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedExperimenterGroupList()
{
return experimenterGroupLinks.size();
}
public List copyLinkedExperimenterGroupList()
{
return new ArrayList<>(experimenterGroupLinks);
}
public ExperimenterGroup getLinkedExperimenterGroup(int index)
{
return experimenterGroupLinks.get(index);
}
public ExperimenterGroup setLinkedExperimenterGroup(int index, ExperimenterGroup o)
{
return experimenterGroupLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkExperimenterGroup(ExperimenterGroup o)
{
return experimenterGroupLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkExperimenterGroup(ExperimenterGroup o)
{
return experimenterGroupLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedDatasetList()
{
return datasetLinks.size();
}
public List copyLinkedDatasetList()
{
return new ArrayList<>(datasetLinks);
}
public Dataset getLinkedDataset(int index)
{
return datasetLinks.get(index);
}
public Dataset setLinkedDataset(int index, Dataset o)
{
return datasetLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkDataset(Dataset o)
{
return datasetLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkDataset(Dataset o)
{
return datasetLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedExperimenterList()
{
return experimenterLinks.size();
}
public List copyLinkedExperimenterList()
{
return new ArrayList<>(experimenterLinks);
}
public Experimenter getLinkedExperimenter(int index)
{
return experimenterLinks.get(index);
}
public Experimenter setLinkedExperimenter(int index, Experimenter o)
{
return experimenterLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkExperimenter(Experimenter o)
{
return experimenterLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkExperimenter(Experimenter o)
{
return experimenterLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedFolderList()
{
return folderLinks.size();
}
public List copyLinkedFolderList()
{
return new ArrayList<>(folderLinks);
}
public Folder getLinkedFolder(int index)
{
return folderLinks.get(index);
}
public Folder setLinkedFolder(int index, Folder o)
{
return folderLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkFolder(Folder o)
{
return folderLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkFolder(Folder o)
{
return folderLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedObjectiveList()
{
return objectiveLinks.size();
}
public List copyLinkedObjectiveList()
{
return new ArrayList<>(objectiveLinks);
}
public Objective getLinkedObjective(int index)
{
return objectiveLinks.get(index);
}
public Objective setLinkedObjective(int index, Objective o)
{
return objectiveLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkObjective(Objective o)
{
return objectiveLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkObjective(Objective o)
{
return objectiveLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedDetectorList()
{
return detectorLinks.size();
}
public List copyLinkedDetectorList()
{
return new ArrayList<>(detectorLinks);
}
public Detector getLinkedDetector(int index)
{
return detectorLinks.get(index);
}
public Detector setLinkedDetector(int index, Detector o)
{
return detectorLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkDetector(Detector o)
{
return detectorLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkDetector(Detector o)
{
return detectorLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedFilterList()
{
return filterLinks.size();
}
public List copyLinkedFilterList()
{
return new ArrayList<>(filterLinks);
}
public Filter getLinkedFilter(int index)
{
return filterLinks.get(index);
}
public Filter setLinkedFilter(int index, Filter o)
{
return filterLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkFilter(Filter o)
{
return filterLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkFilter(Filter o)
{
return filterLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedDichroicList()
{
return dichroicLinks.size();
}
public List copyLinkedDichroicList()
{
return new ArrayList<>(dichroicLinks);
}
public Dichroic getLinkedDichroic(int index)
{
return dichroicLinks.get(index);
}
public Dichroic setLinkedDichroic(int index, Dichroic o)
{
return dichroicLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkDichroic(Dichroic o)
{
return dichroicLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkDichroic(Dichroic o)
{
return dichroicLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedLightPathList()
{
return lightPathLinks.size();
}
public List copyLinkedLightPathList()
{
return new ArrayList<>(lightPathLinks);
}
public LightPath getLinkedLightPath(int index)
{
return lightPathLinks.get(index);
}
public LightPath setLinkedLightPath(int index, LightPath o)
{
return lightPathLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkLightPath(LightPath o)
{
return lightPathLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkLightPath(LightPath o)
{
return lightPathLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedROIList()
{
return roiLinks.size();
}
public List copyLinkedROIList()
{
return new ArrayList<>(roiLinks);
}
public ROI getLinkedROI(int index)
{
return roiLinks.get(index);
}
public ROI setLinkedROI(int index, ROI o)
{
return roiLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkROI(ROI o)
{
return roiLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkROI(ROI o)
{
return roiLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedShapeList()
{
return shapeLinks.size();
}
public List copyLinkedShapeList()
{
return new ArrayList<>(shapeLinks);
}
public Shape getLinkedShape(int index)
{
return shapeLinks.get(index);
}
public Shape setLinkedShape(int index, Shape o)
{
return shapeLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkShape(Shape o)
{
return shapeLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkShape(Shape o)
{
return shapeLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedPlateList()
{
return plateLinks.size();
}
public List copyLinkedPlateList()
{
return new ArrayList<>(plateLinks);
}
public Plate getLinkedPlate(int index)
{
return plateLinks.get(index);
}
public Plate setLinkedPlate(int index, Plate o)
{
return plateLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkPlate(Plate o)
{
return plateLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkPlate(Plate o)
{
return plateLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedReagentList()
{
return reagentLinks.size();
}
public List copyLinkedReagentList()
{
return new ArrayList<>(reagentLinks);
}
public Reagent getLinkedReagent(int index)
{
return reagentLinks.get(index);
}
public Reagent setLinkedReagent(int index, Reagent o)
{
return reagentLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkReagent(Reagent o)
{
return reagentLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkReagent(Reagent o)
{
return reagentLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedScreenList()
{
return screenLinks.size();
}
public List copyLinkedScreenList()
{
return new ArrayList<>(screenLinks);
}
public Screen getLinkedScreen(int index)
{
return screenLinks.get(index);
}
public Screen setLinkedScreen(int index, Screen o)
{
return screenLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkScreen(Screen o)
{
return screenLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkScreen(Screen o)
{
return screenLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedPlateAcquisitionList()
{
return plateAcquisitionLinks.size();
}
public List copyLinkedPlateAcquisitionList()
{
return new ArrayList<>(plateAcquisitionLinks);
}
public PlateAcquisition getLinkedPlateAcquisition(int index)
{
return plateAcquisitionLinks.get(index);
}
public PlateAcquisition setLinkedPlateAcquisition(int index, PlateAcquisition o)
{
return plateAcquisitionLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkPlateAcquisition(PlateAcquisition o)
{
return plateAcquisitionLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkPlateAcquisition(PlateAcquisition o)
{
return plateAcquisitionLinks.remove(o);
}
// Reference which occurs more than once
public int sizeOfLinkedWellList()
{
return wellLinks.size();
}
public List copyLinkedWellList()
{
return new ArrayList<>(wellLinks);
}
public Well getLinkedWell(int index)
{
return wellLinks.get(index);
}
public Well setLinkedWell(int index, Well o)
{
return wellLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkWell(Well o)
{
return wellLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkWell(Well o)
{
return wellLinks.remove(o);
}
public Element asXMLElement(Document document)
{
return asXMLElement(document, null);
}
public Element asXMLElement(Document document, Element Annotation_element)
{
// Creating XML block for Annotation
if (Annotation_element == null)
{
Annotation_element =
document.createElementNS(NAMESPACE, "Annotation");
}
// Ensure any base annotations add their Elements first
super.asXMLElement(document, Annotation_element);
if (id != null)
{
// Attribute property ID
Annotation_element.setAttribute("ID", id);
}
if (namespace != null)
{
// Attribute property Namespace
Annotation_element.setAttribute("Namespace", namespace);
}
if (annotator != null)
{
// Attribute property Annotator
Annotation_element.setAttribute("Annotator", annotator);
}
if (description != null)
{
// Element property Description which is not complex (has no
// sub-elements)
Element description_element =
document.createElementNS(NAMESPACE, "Description");
description_element.setTextContent(description);
Annotation_element.appendChild(description_element);
}
if (annotationLinks != null)
{
// Reference property AnnotationRef which occurs more than once
for (Annotation annotationLinks_value : annotationLinks)
{
AnnotationRef o = new AnnotationRef();
o.setID(annotationLinks_value.getID());
Element child =
document.createElementNS(NAMESPACE, "AnnotationRef");
o.asXMLElement(document, child);
Annotation_element.appendChild(child);
}
}
// *** IGNORING *** Skipped back reference Image_BackReference
// *** IGNORING *** Skipped back reference Plane_BackReference
// *** IGNORING *** Skipped back reference Channel_BackReference
// *** IGNORING *** Skipped back reference Instrument_BackReference
// *** IGNORING *** Skipped back reference LightSource_BackReference
// *** IGNORING *** Skipped back reference Project_BackReference
// *** IGNORING *** Skipped back reference ExperimenterGroup_BackReference
// *** IGNORING *** Skipped back reference Dataset_BackReference
// *** IGNORING *** Skipped back reference Experimenter_BackReference
// *** IGNORING *** Skipped back reference Folder_BackReference
// *** IGNORING *** Skipped back reference Objective_BackReference
// *** IGNORING *** Skipped back reference Detector_BackReference
// *** IGNORING *** Skipped back reference Filter_BackReference
// *** IGNORING *** Skipped back reference Dichroic_BackReference
// *** IGNORING *** Skipped back reference LightPath_BackReference
// *** IGNORING *** Skipped back reference ROI_BackReference
// *** IGNORING *** Skipped back reference Shape_BackReference
// *** IGNORING *** Skipped back reference Plate_BackReference
// *** IGNORING *** Skipped back reference Reagent_BackReference
// *** IGNORING *** Skipped back reference Screen_BackReference
// *** IGNORING *** Skipped back reference PlateAcquisition_BackReference
// *** IGNORING *** Skipped back reference Well_BackReference
return Annotation_element;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy