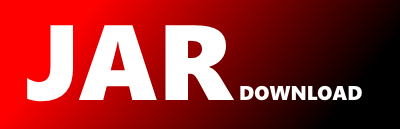
ome.xml.model.Label Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ome-xml Show documentation
Show all versions of ome-xml Show documentation
A library for working with OME-XML metadata structures.
The newest version!
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.model;
import java.util.ArrayList;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import ome.xml.model.enums.*;
import ome.xml.model.enums.handlers.*;
import ome.xml.model.primitives.*;
import ome.units.quantity.*;
import ome.units.unit.Unit;
public class Label extends Shape
{
// Base: Shape -- Name: Label -- Type: Label -- modelBaseType: Shape -- langBaseType: Object
// -- Constants --
public static final String NAMESPACE = "http://www.openmicroscopy.org/Schemas/OME/2016-06";
/** Logger for this class. */
private static final Logger LOGGER =
LoggerFactory.getLogger(Label.class);
// -- Instance variables --
// X property
private Double x;
// Y property
private Double y;
// -- Constructors --
/** Default constructor. */
public Label()
{
super();
}
/**
* Constructs Label recursively from an XML DOM tree.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public Label(Element element, OMEModel model)
throws EnumerationException
{
update(element, model);
}
/** Copy constructor. */
public Label(Label orig)
{
super(orig);
x = orig.x;
y = orig.y;
}
// -- Custom content from Label specific template --
// -- OMEModelObject API methods --
/**
* Updates Label recursively from an XML DOM tree. NOTE: No
* properties are removed, only added or updated.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public void update(Element element, OMEModel model)
throws EnumerationException
{
super.update(element, model);
if (element.hasAttribute("X"))
{
// Attribute property X
setX(Double.valueOf(
element.getAttribute("X")));
}
if (element.hasAttribute("Y"))
{
// Attribute property Y
setY(Double.valueOf(
element.getAttribute("Y")));
}
}
// -- Label API methods --
public boolean link(Reference reference, OMEModelObject o)
{
return super.link(reference, o);
}
// Property X
public Double getX()
{
return x;
}
public void setX(Double x)
{
this.x = x;
}
// Property Y
public Double getY()
{
return y;
}
public void setY(Double y)
{
this.y = y;
}
public Element asXMLElement(Document document)
{
return asXMLElement(document, null);
}
public Element asXMLElement(Document document, Element Label_element)
{
// Creating XML block for Label
if (Label_element == null)
{
Label_element =
document.createElementNS(NAMESPACE, "Label");
}
// Ensure any base annotations add their Elements first
super.asXMLElement(document, Label_element);
if (x != null)
{
// Attribute property X
Label_element.setAttribute("X", x.toString());
}
if (y != null)
{
// Attribute property Y
Label_element.setAttribute("Y", y.toString());
}
return Label_element;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy