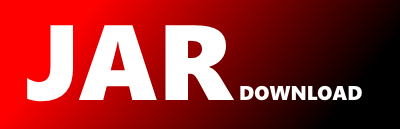
ome.xml.model.Reagent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ome-xml Show documentation
Show all versions of ome-xml Show documentation
A library for working with OME-XML metadata structures.
The newest version!
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.model;
import java.util.ArrayList;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import ome.xml.model.enums.*;
import ome.xml.model.enums.handlers.*;
import ome.xml.model.primitives.*;
import ome.units.quantity.*;
import ome.units.unit.Unit;
public class Reagent extends AbstractOMEModelObject
{
// Base: -- Name: Reagent -- Type: Reagent -- modelBaseType: AbstractOMEModelObject -- langBaseType: Object
// -- Constants --
public static final String NAMESPACE = "http://www.openmicroscopy.org/Schemas/OME/2016-06";
/** Logger for this class. */
private static final Logger LOGGER =
LoggerFactory.getLogger(Reagent.class);
// -- Instance variables --
// ID property
private String id;
// Name property
private String name;
// ReagentIdentifier property
private String reagentIdentifier;
// Description property
private String description;
// AnnotationRef reference (occurs more than once)
private List annotationLinks = new ReferenceList<>();
// Screen_BackReference back reference
private Screen screen;
// Well_BackReference back reference (occurs more than once)
private List wells = new ReferenceList<>();
// -- Constructors --
/** Default constructor. */
public Reagent()
{
}
/**
* Constructs Reagent recursively from an XML DOM tree.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public Reagent(Element element, OMEModel model)
throws EnumerationException
{
update(element, model);
}
/** Copy constructor. */
public Reagent(Reagent orig)
{
id = orig.id;
name = orig.name;
reagentIdentifier = orig.reagentIdentifier;
description = orig.description;
annotationLinks = orig.annotationLinks;
screen = orig.screen;
wells = orig.wells;
}
// -- Custom content from Reagent specific template --
// -- OMEModelObject API methods --
/**
* Updates Reagent recursively from an XML DOM tree. NOTE: No
* properties are removed, only added or updated.
* @param element Root of the XML DOM tree to construct a model object
* graph from.
* @param model Handler for the OME model which keeps track of instances
* and references seen during object population.
* @throws EnumerationException If there is an error instantiating an
* enumeration during model object creation.
*/
public void update(Element element, OMEModel model)
throws EnumerationException
{
super.update(element, model);
if (!element.hasAttribute("ID") && getID() == null)
{
// TODO: Should be its own exception
throw new RuntimeException(
"Reagent missing required ID property.");
}
if (element.hasAttribute("ID"))
{
// ID property
setID(String.valueOf(
element.getAttribute("ID")));
// Adding this model object to the model handler
model.addModelObject(getID(), this);
}
if (element.hasAttribute("Name"))
{
// Attribute property Name
setName(String.valueOf(
element.getAttribute("Name")));
}
if (element.hasAttribute("ReagentIdentifier"))
{
// Attribute property ReagentIdentifier
setReagentIdentifier(String.valueOf(
element.getAttribute("ReagentIdentifier")));
}
List Description_nodeList =
getChildrenByTagName(element, "Description");
if (Description_nodeList.size() > 1)
{
// TODO: Should be its own Exception
throw new RuntimeException(String.format(
"Description node list size %d != 1",
Description_nodeList.size()));
}
else if (Description_nodeList.size() != 0)
{
// Element property Description which is not complex (has no
// sub-elements)
setDescription(
String.valueOf(Description_nodeList.get(0).getTextContent()));
}
// Element reference AnnotationRef
List AnnotationRef_nodeList =
getChildrenByTagName(element, "AnnotationRef");
for (Element AnnotationRef_element : AnnotationRef_nodeList)
{
AnnotationRef annotationLinks_reference = new AnnotationRef();
annotationLinks_reference.setID(AnnotationRef_element.getAttribute("ID"));
model.addReference(this, annotationLinks_reference);
}
}
// -- Reagent API methods --
public boolean link(Reference reference, OMEModelObject o)
{
if (reference instanceof AnnotationRef)
{
Annotation o_casted = (Annotation) o;
o_casted.linkReagent(this);
annotationLinks.add(o_casted);
return true;
}
return super.link(reference, o);
}
// Property ID
public String getID()
{
return id;
}
public void setID(String id)
{
this.id = id;
}
// Property Name
public String getName()
{
return name;
}
public void setName(String name)
{
this.name = name;
}
// Property ReagentIdentifier
public String getReagentIdentifier()
{
return reagentIdentifier;
}
public void setReagentIdentifier(String reagentIdentifier)
{
this.reagentIdentifier = reagentIdentifier;
}
// Property Description
public String getDescription()
{
return description;
}
public void setDescription(String description)
{
this.description = description;
}
// Reference which occurs more than once
public int sizeOfLinkedAnnotationList()
{
return annotationLinks.size();
}
public List copyLinkedAnnotationList()
{
return new ArrayList<>(annotationLinks);
}
public Annotation getLinkedAnnotation(int index)
{
return annotationLinks.get(index);
}
public Annotation setLinkedAnnotation(int index, Annotation o)
{
return annotationLinks.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkAnnotation(Annotation o)
{
o.linkReagent(this);
return annotationLinks.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkAnnotation(Annotation o)
{
o.unlinkReagent(this);
return annotationLinks.remove(o);
}
// Property Screen_BackReference
public Screen getScreen()
{
return screen;
}
public void setScreen(Screen screen_BackReference)
{
this.screen = screen_BackReference;
}
// Reference which occurs more than once
public int sizeOfLinkedWellList()
{
return wells.size();
}
public List copyLinkedWellList()
{
return new ArrayList<>(wells);
}
public Well getLinkedWell(int index)
{
return wells.get(index);
}
public Well setLinkedWell(int index, Well o)
{
return wells.set(index, o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean linkWell(Well o)
{
return wells.add(o);
}
@SuppressWarnings("UnusedReturnValue")
public boolean unlinkWell(Well o)
{
return wells.remove(o);
}
public Element asXMLElement(Document document)
{
return asXMLElement(document, null);
}
public Element asXMLElement(Document document, Element Reagent_element)
{
// Creating XML block for Reagent
if (Reagent_element == null)
{
Reagent_element =
document.createElementNS(NAMESPACE, "Reagent");
}
// Ensure any base annotations add their Elements first
super.asXMLElement(document, Reagent_element);
if (id != null)
{
// Attribute property ID
Reagent_element.setAttribute("ID", id);
}
if (name != null)
{
// Attribute property Name
Reagent_element.setAttribute("Name", name);
}
if (reagentIdentifier != null)
{
// Attribute property ReagentIdentifier
Reagent_element.setAttribute("ReagentIdentifier", reagentIdentifier);
}
if (description != null)
{
// Element property Description which is not complex (has no
// sub-elements)
Element description_element =
document.createElementNS(NAMESPACE, "Description");
description_element.setTextContent(description);
Reagent_element.appendChild(description_element);
}
if (annotationLinks != null)
{
// Reference property AnnotationRef which occurs more than once
for (Annotation annotationLinks_value : annotationLinks)
{
AnnotationRef o = new AnnotationRef();
o.setID(annotationLinks_value.getID());
Element child =
document.createElementNS(NAMESPACE, "AnnotationRef");
o.asXMLElement(document, child);
Reagent_element.appendChild(child);
}
}
// *** IGNORING *** Skipped back reference Screen_BackReference
// *** IGNORING *** Skipped back reference Well_BackReference
return Reagent_element;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy