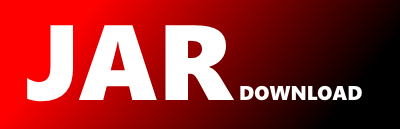
ome.xml.model.enums.UnitsPressure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ome-xml Show documentation
Show all versions of ome-xml Show documentation
A library for working with OME-XML metadata structures.
The newest version!
/*
* #%L
* OME-XML Java library for working with OME-XML metadata structures.
* %%
* Copyright (C) 2006 - 2016 Open Microscopy Environment:
* - Massachusetts Institute of Technology
* - National Institutes of Health
* - University of Dundee
* - Board of Regents of the University of Wisconsin-Madison
* - Glencoe Software, Inc.
* %%
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDERS OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
* The views and conclusions contained in the software and documentation are
* those of the authors and should not be interpreted as representing official
* policies, either expressed or implied, of any organization.
* #L%
*/
/*-----------------------------------------------------------------------------
*
* THIS IS AUTOMATICALLY GENERATED CODE. DO NOT MODIFY.
*
*-----------------------------------------------------------------------------
*/
package ome.xml.model.enums;
import ome.xml.model.enums.handlers.UnitsPressureEnumHandler;
import ome.xml.model.primitives.*;
import ome.units.quantity.Pressure;
import ome.units.unit.Unit;
import ome.units.UNITS;
@SuppressWarnings("SpellCheckingInspection")
public enum UnitsPressure implements Enumeration
{
/** yottapascal SI unit. */
YOTTAPASCAL("YPa"),
/** zettapascal SI unit. */
ZETTAPASCAL("ZPa"),
/** exapascal SI unit. */
EXAPASCAL("EPa"),
/** petapascal SI unit. */
PETAPASCAL("PPa"),
/** terapascal SI unit. */
TERAPASCAL("TPa"),
/** gigapascal SI unit. */
GIGAPASCAL("GPa"),
/** megapascal SI unit. */
MEGAPASCAL("MPa"),
/** kilopascal SI unit. */
KILOPASCAL("kPa"),
/** hectopascal SI unit. */
HECTOPASCAL("hPa"),
/** decapascal SI unit. */
DECAPASCAL("daPa"),
/** pascal SI unit. Note the C++ enum is mixed case due to PASCAL being a macro used by the Microsoft C and C++ compiler. */
PASCAL("Pa"),
/** decipascal SI unit. */
DECIPASCAL("dPa"),
/** centipascal SI unit. */
CENTIPASCAL("cPa"),
/** millipascal SI unit. */
MILLIPASCAL("mPa"),
/** micropascal SI unit. */
MICROPASCAL("µPa"),
/** nanopascal SI unit. */
NANOPASCAL("nPa"),
/** picopascal SI unit. */
PICOPASCAL("pPa"),
/** femtopascal SI unit. */
FEMTOPASCAL("fPa"),
/** attopascal SI unit. */
ATTOPASCAL("aPa"),
/** zeptopascal SI unit. */
ZEPTOPASCAL("zPa"),
/** yoctopascal SI unit. */
YOCTOPASCAL("yPa"),
/** bar SI-derived unit. */
BAR("bar"),
/** megabar SI-derived unit. */
MEGABAR("Mbar"),
/** kilobar SI-derived unit. */
KILOBAR("kbar"),
/** decibar SI-derived unit. */
DECIBAR("dbar"),
/** centibar SI-derived unit. */
CENTIBAR("cbar"),
/** millibar SI-derived unit. */
MILLIBAR("mbar"),
/** standard atmosphere SI-derived unit. */
ATMOSPHERE("atm"),
/** pound-force per square inch Imperial unit. */
PSI("psi"),
/** torr SI-derived unit. */
TORR("Torr"),
/** millitorr SI-derived unit. */
MILLITORR("mTorr"),
/** millimetre of mercury SI-derived unit */
MMHG("mm Hg");
/** C++ compatibility name for PASCAL. */
public static final UnitsPressure Pascal = PASCAL;
UnitsPressure(String value)
{
this.value = value;
}
public static UnitsPressure fromString(String value)
throws EnumerationException
{
if ("YPa".equals(value))
{
return YOTTAPASCAL;
}
if ("ZPa".equals(value))
{
return ZETTAPASCAL;
}
if ("EPa".equals(value))
{
return EXAPASCAL;
}
if ("PPa".equals(value))
{
return PETAPASCAL;
}
if ("TPa".equals(value))
{
return TERAPASCAL;
}
if ("GPa".equals(value))
{
return GIGAPASCAL;
}
if ("MPa".equals(value))
{
return MEGAPASCAL;
}
if ("kPa".equals(value))
{
return KILOPASCAL;
}
if ("hPa".equals(value))
{
return HECTOPASCAL;
}
if ("daPa".equals(value))
{
return DECAPASCAL;
}
if ("Pa".equals(value))
{
return PASCAL;
}
if ("dPa".equals(value))
{
return DECIPASCAL;
}
if ("cPa".equals(value))
{
return CENTIPASCAL;
}
if ("mPa".equals(value))
{
return MILLIPASCAL;
}
if ("µPa".equals(value))
{
return MICROPASCAL;
}
if ("nPa".equals(value))
{
return NANOPASCAL;
}
if ("pPa".equals(value))
{
return PICOPASCAL;
}
if ("fPa".equals(value))
{
return FEMTOPASCAL;
}
if ("aPa".equals(value))
{
return ATTOPASCAL;
}
if ("zPa".equals(value))
{
return ZEPTOPASCAL;
}
if ("yPa".equals(value))
{
return YOCTOPASCAL;
}
if ("bar".equals(value))
{
return BAR;
}
if ("Mbar".equals(value))
{
return MEGABAR;
}
if ("kbar".equals(value))
{
return KILOBAR;
}
if ("dbar".equals(value))
{
return DECIBAR;
}
if ("cbar".equals(value))
{
return CENTIBAR;
}
if ("mbar".equals(value))
{
return MILLIBAR;
}
if ("atm".equals(value))
{
return ATMOSPHERE;
}
if ("psi".equals(value))
{
return PSI;
}
if ("Torr".equals(value))
{
return TORR;
}
if ("mTorr".equals(value))
{
return MILLITORR;
}
if ("mm Hg".equals(value))
{
return MMHG;
}
String s = String.format("'%s' not a supported value of '%s'",
value, UnitsPressure.class);
throw new EnumerationException(s);
}
@SuppressWarnings("unused")
public String getValue()
{
return value;
}
@Override
public String toString()
{
return value;
}
public static Pressure create(T newValue, UnitsPressure newUnit)
{
Pressure theQuantity = null;
try
{
theQuantity = UnitsPressureEnumHandler.getQuantity(newValue, newUnit);
}
catch (EnumerationException ignored)
{
}
return theQuantity;
}
public static Pressure create(T newValue, UnitsPressure newUnit)
{
Pressure theQuantity = null;
try
{
theQuantity = UnitsPressureEnumHandler.getQuantity(newValue, newUnit);
}
catch (EnumerationException ignored)
{
}
return theQuantity;
}
private final String value;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy