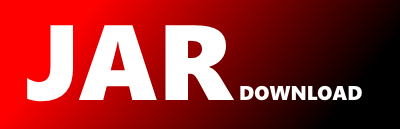
org.opennms.netmgt.model.OnmsMonitoredService Maven / Gradle / Ivy
/*
* Licensed to The OpenNMS Group, Inc (TOG) under one or more
* contributor license agreements. See the LICENSE.md file
* distributed with this work for additional information
* regarding copyright ownership.
*
* TOG licenses this file to You under the GNU Affero General
* Public License Version 3 (the "License") or (at your option)
* any later version. You may not use this file except in
* compliance with the License. You may obtain a copy of the
* License at:
*
* https://www.gnu.org/licenses/agpl-3.0.txt
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific
* language governing permissions and limitations under the
* License.
*/
package org.opennms.netmgt.model;
import static org.opennms.core.utils.InetAddressUtils.toInteger;
import java.io.Serializable;
import java.math.BigInteger;
import java.net.InetAddress;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import javax.persistence.CascadeType;
import javax.persistence.CollectionTable;
import javax.persistence.Column;
import javax.persistence.ElementCollection;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.JoinTable;
import javax.persistence.ManyToMany;
import javax.persistence.ManyToOne;
import javax.persistence.OneToMany;
import javax.persistence.SequenceGenerator;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import javax.persistence.Transient;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlIDREF;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlTransient;
import org.codehaus.jackson.annotate.JsonIgnore;
import org.codehaus.jackson.annotate.JsonIgnoreProperties;
import org.codehaus.jackson.annotate.JsonProperty;
import org.hibernate.annotations.Where;
import com.google.common.base.MoreObjects;
@XmlRootElement(name = "service")
@Entity
@Table(name="ifServices")
@JsonIgnoreProperties({"hibernateLazyInitializer", "handler"})
public class OnmsMonitoredService extends OnmsEntity implements Serializable, Comparable {
private static final long serialVersionUID = 7899180234592272274L;
private Integer m_id;
private Date m_lastGood;
private Date m_lastFail;
private String m_qualifier;
private String m_status;
private String m_source;
private String m_notify;
private OnmsServiceType m_serviceType;
private OnmsIpInterface m_ipInterface;
/*
* This is a set only because we want it to be lazy
* and we need a better query language (i.e. HQL)
* to make this work. In this case, the Set size
* will always be 1 or empty because there can only
* be one outage at a time on a service.
*
* With distributed monitoring, there will probably
* be a model change were one service can be represented
* by more than one outage.
*/
private Set m_currentOutages = new LinkedHashSet<>();
private Set m_applications = new LinkedHashSet<>();
private List m_metaData = new ArrayList<>();
public static final Map STATUS_MAP;
static {
STATUS_MAP = new HashMap();
STATUS_MAP.put("A", "Managed");
STATUS_MAP.put("U", "Unmanaged");
STATUS_MAP.put("D", "Deleted");
STATUS_MAP.put("F", "Forced Unmanaged");
STATUS_MAP.put("N", "Not Monitored");
STATUS_MAP.put("R", "Rescan to Resume");
STATUS_MAP.put("S", "Rescan to Suspend");
STATUS_MAP.put("X", "Remotely Monitored");
}
/**
* Constructor for OnmsMonitoredService.
*/
public OnmsMonitoredService() {
}
/**
* Constructor for OnmsMonitoredService.
*
* @param ipIf a {@link org.opennms.netmgt.model.OnmsIpInterface} object.
* @param serviceType a {@link org.opennms.netmgt.model.OnmsServiceType} object.
*/
public OnmsMonitoredService(OnmsIpInterface ipIf, OnmsServiceType serviceType) {
m_ipInterface = ipIf;
m_ipInterface.getMonitoredServices().add(this);
m_serviceType = serviceType;
}
/**
* Unique identifier for ifService.
*
* @return a {@link java.lang.Integer} object.
*/
@Id
@Column(nullable=false)
@SequenceGenerator(name="opennmsSequence", sequenceName="opennmsNxtId")
@GeneratedValue(generator="opennmsSequence")
@XmlTransient
public Integer getId() {
return m_id;
}
@Transient
@JsonProperty("id")
public Integer getJsonId() {
return m_id;
}
/**
* setId
*
* @param id a {@link java.lang.Integer} object.
*/
public void setId(Integer id) {
m_id = id;
}
/**
* This id is used for the serialized representation such as json, xml etc.
*/
@XmlID
@XmlAttribute(name="id")
@Transient
@JsonIgnore
public String getXmlId() {
return getId() == null? null : getId().toString();
}
public void setXmlId(final String id) {
setId(Integer.valueOf(id));
}
/**
* getIpAddress
*
* @return a {@link java.lang.String} object.
*/
@XmlTransient
@Transient
@JsonIgnore
public InetAddress getIpAddress() {
return m_ipInterface.getIpAddress();
}
/**
* getIpAddress
*
* @return a {@link java.lang.String} object.
*
* @deprecated
*/
@XmlTransient
@Transient
@JoinColumn(name="ipInterfaceId")
@JsonProperty("ipAddress")
public String getIpAddressAsString() {
return m_ipInterface.getIpAddressAsString();
}
/**
* getIfIndex
*
* @return a {@link java.lang.Integer} object.
*/
@XmlTransient
@Transient
@JsonIgnore
public Integer getIfIndex() {
return m_ipInterface.getIfIndex();
}
/**
* getLastGood
*
* @return a {@link java.util.Date} object.
*/
@Temporal(TemporalType.TIMESTAMP)
@Column(name="lastGood")
public Date getLastGood() {
return m_lastGood;
}
/**
* setLastGood
*
* @param lastgood a {@link java.util.Date} object.
*/
public void setLastGood(Date lastgood) {
m_lastGood = lastgood;
}
/**
* getLastFail
*
* @return a {@link java.util.Date} object.
*/
@Temporal(TemporalType.TIMESTAMP)
@Column(name="lastFail")
public Date getLastFail() {
return m_lastFail;
}
/**
* setLastFail
*
* @param lastfail a {@link java.util.Date} object.
*/
public void setLastFail(Date lastfail) {
m_lastFail = lastfail;
}
/**
* getQualifier
*
* @return a {@link java.lang.String} object.
*/
@Column(name="qualifier", length=16)
public String getQualifier() {
return m_qualifier;
}
/**
* setQualifier
*
* @param qualifier a {@link java.lang.String} object.
*/
public void setQualifier(String qualifier) {
m_qualifier = qualifier;
}
/**
* getStatus
*
* @return a {@link java.lang.String} object.
*/
@XmlAttribute
@Column(name="status", length=1)
public String getStatus() {
return m_status;
}
/**
* setStatus
*
* @param status a {@link java.lang.String} object.
*/
public void setStatus(String status) {
m_status = status;
}
@Transient
@XmlAttribute
public String getStatusLong() {
return STATUS_MAP.get(getStatus());
}
/**
* getSource
*
* @return a {@link java.lang.String} object.
*/
@XmlAttribute
@Column(name="source", length=1)
public String getSource() {
return m_source;
}
/**
* setSource
*
* @param source a {@link java.lang.String} object.
*/
public void setSource(String source) {
m_source = source;
}
/**
* getNotify
*
* @return a {@link java.lang.String} object.
*/
@Column(name="notify", length=1)
public String getNotify() {
return m_notify;
}
/**
* setNotify
*
* @param notify a {@link java.lang.String} object.
*/
public void setNotify(String notify) {
m_notify = notify;
}
@JsonIgnore
@XmlTransient
@ElementCollection(fetch = FetchType.LAZY)
@CollectionTable(name="ifServices_metadata", joinColumns = @JoinColumn(name = "id"))
public List getMetaData() {
return m_metaData;
}
public void setMetaData(final List metaData) {
m_metaData = metaData;
}
public void addMetaData(final String context, final String key, final String value) {
Objects.requireNonNull(context);
Objects.requireNonNull(key);
Objects.requireNonNull(value);
final Optional entry = getMetaData().stream()
.filter(m -> m.getContext().equals(context))
.filter(m -> m.getKey().equals(key))
.findFirst();
// Update the value if present, otherwise create a new entry
if (entry.isPresent()) {
entry.get().setValue(value);
} else {
getMetaData().add(new OnmsMetaData(context, key, value));
}
}
public void removeMetaData(final String context, final String key) {
Objects.requireNonNull(context);
Objects.requireNonNull(key);
final Iterator iterator = getMetaData().iterator();
while (iterator.hasNext()) {
final OnmsMetaData onmsNodeMetaData = iterator.next();
if (context.equals(onmsNodeMetaData.getContext()) && key.equals(onmsNodeMetaData.getKey())) {
iterator.remove();
}
}
}
public void removeMetaData(final String context) {
Objects.requireNonNull(context);
final Iterator iterator = getMetaData().iterator();
while (iterator.hasNext()) {
final OnmsMetaData onmsNodeMetaData = iterator.next();
if (context.equals(onmsNodeMetaData.getContext())) {
iterator.remove();
}
}
}
/**
* getIpInterface
*
* @return a {@link org.opennms.netmgt.model.OnmsIpInterface} object.
*/
@XmlIDREF
@JsonIgnore
@XmlElement(name="ipInterfaceId")
@ManyToOne(optional=false, fetch=FetchType.LAZY)
@JoinColumn(name="ipInterfaceId")
public OnmsIpInterface getIpInterface() {
return m_ipInterface;
}
@JsonProperty("ipInterfaceId")
@Transient
public Integer getIpInterfaceId() {
return m_ipInterface.getId();
}
/**
* setIpInterface
*
* @param ipInterface a {@link org.opennms.netmgt.model.OnmsIpInterface} object.
*/
public void setIpInterface(OnmsIpInterface ipInterface) {
m_ipInterface = ipInterface;
}
/**
* getNodeId
*
* @return a {@link java.lang.Integer} object.
*/
@XmlTransient
@Transient
@JsonProperty("nodeId")
public Integer getNodeId() {
return m_ipInterface.getNode().getId();
}
@XmlTransient
@Transient
@JsonProperty("nodeLabel")
public String getNodeLabel() {
return m_ipInterface.getNode().getLabel();
}
/**
* getServiceType
*
* @return a {@link org.opennms.netmgt.model.OnmsServiceType} object.
*/
@ManyToOne(optional=false)
@JoinColumn(name="serviceId")
public OnmsServiceType getServiceType() {
return m_serviceType;
}
/**
* setServiceType
*
* @param service a {@link org.opennms.netmgt.model.OnmsServiceType} object.
*/
public void setServiceType(OnmsServiceType service) {
m_serviceType = service;
}
/**
* toString
*
* @return a {@link java.lang.String} object.
*/
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("id", m_id)
.add("lastGood", m_lastGood)
.add("lastFail", m_lastFail)
.add("qualifier", m_qualifier)
.add("status", m_status)
.add("source", m_source)
.add("notify", m_notify)
.add("serviceType", m_serviceType)
// cannot include these since the require db queries
// .add("ipInterface", m_ipInterface)
// .add("currentOutages", m_currentOutages)
// .add("applications", m_applications)
.toString();
}
/**
* getServiceId
*
* @return a {@link java.lang.Integer} object.
*/
@Transient
@JsonIgnore
public Integer getServiceId() {
return getServiceType().getId();
}
/** {@inheritDoc} */
@Override
public void visit(EntityVisitor visitor) {
visitor.visitMonitoredService(this);
visitor.visitMonitoredServiceComplete(this);
}
/**
* getServiceName
*
* @return a {@link java.lang.String} object.
*/
@Transient
@JsonIgnore
public String getServiceName() {
return getServiceType().getName();
}
/**
* isDown
*
* @return a boolean.
*/
@Transient
@XmlAttribute(name="down")
public boolean isDown() {
boolean down = true;
if (!"A".equals(getStatus()) || m_currentOutages.isEmpty()) {
return !down;
}
return down;
}
/**
* getCurrentOutages
*
* @return a {@link java.util.Set} object.
*/
@XmlTransient
@OneToMany(mappedBy="monitoredService", fetch=FetchType.LAZY)
@Where(clause="ifRegainedService is null")
@JsonIgnore
public Set getCurrentOutages() {
return m_currentOutages;
}
/**
* setCurrentOutages
*
* @param currentOutages a {@link java.util.Set} object.
*/
public void setCurrentOutages(Set currentOutages) {
m_currentOutages = currentOutages;
}
/**
* getApplications
*
* @return a {@link java.util.Set} object.
*/
@ManyToMany(
cascade={CascadeType.PERSIST, CascadeType.MERGE}
)
@JoinTable(
name="application_service_map",
joinColumns={@JoinColumn(name="ifserviceid")},
inverseJoinColumns={@JoinColumn(name="appid")}
)
@XmlTransient
@JsonIgnore
public Set getApplications() {
return m_applications;
}
/**
* setApplications
*
* @param applications a {@link java.util.Set} object.
*/
public void setApplications(Set applications) {
m_applications = applications;
}
/**
* addApplication
*
* @param application a {@link org.opennms.netmgt.model.OnmsApplication} object.
* @return a boolean.
*/
public boolean addApplication(OnmsApplication application) {
return getApplications().add(application);
}
/**
* removeApplication
*
* @param application a {@link org.opennms.netmgt.model.OnmsApplication} object.
* @return a boolean.
*/
public boolean removeApplication(OnmsApplication application) {
return getApplications().remove(application);
}
/**
* compareTo
*
* @param o a {@link org.opennms.netmgt.model.OnmsMonitoredService} object.
* @return a int.
*/
@Override
public int compareTo(OnmsMonitoredService o) {
int diff;
diff = getIpInterface().getNode().getLabel().compareToIgnoreCase(o.getIpInterface().getNode().getLabel());
if (diff != 0) {
return diff;
}
BigInteger a = toInteger(getIpAddress());
BigInteger b = toInteger(o.getIpAddress());
diff = a.compareTo(b);
if (diff != 0) {
return diff;
}
return getServiceName().compareToIgnoreCase(o.getServiceName());
}
/**
* mergeServiceAttributes
*
* @param scanned a {@link org.opennms.netmgt.model.OnmsMonitoredService} object.
*/
public void mergeServiceAttributes(OnmsMonitoredService scanned) {
if (hasNewValue(scanned.getQualifier(), getQualifier())) {
setQualifier(scanned.getQualifier());
}
if (hasNewStatusValue(scanned.getStatus(), getStatus())) {
setStatus(scanned.getStatus());
}
if (hasNewValue(scanned.getSource(), getSource())) {
setSource(scanned.getSource());
}
if (hasNewValue(scanned.getNotify(), getNotify())) {
setNotify(scanned.getNotify());
}
}
public void mergeMetaData(OnmsMonitoredService scanned) {
if (!getMetaData().equals(scanned.getMetaData())) {
setMetaData(scanned.getMetaData());
}
}
private boolean hasNewStatusValue(String newStatus, String oldStatus)
{
/*
* Don't overwrite the 'Not Monitored' in the database when provisioning the
* node. The Poller will update it when scheduling it packages.
*/
return !"N".equals(oldStatus) && newStatus != null && !newStatus.equals(oldStatus);
}
@Transient
@XmlTransient
@JsonIgnore
public String getForeignSource() {
if (getIpInterface() != null) {
return getIpInterface().getForeignSource();
}
return null;
}
@Transient
@XmlTransient
@JsonIgnore
public String getForeignId() {
if (getIpInterface() != null) {
return getIpInterface().getForeignId();
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy