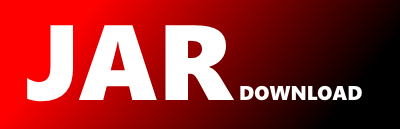
com.ibm.commons.runtime.Context Maven / Gradle / Ivy
The newest version!
/*
* © Copyright IBM Corp. 2012
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.ibm.commons.runtime;
import java.io.IOException;
import java.util.AbstractMap;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.ibm.commons.Platform;
/**
* SBT Context.
*
* This class encapsulate a context that can be reused by all the helper classes.
*
* @author Philippe Riand
*/
public abstract class Context {
public static final String ANONYMOUS = "anonymous";
public static Context init(Application application, Object request, Object response) {
return RuntimeFactory.get().initContext(application, request, response);
}
public static Context init(Context context) {
return RuntimeFactory.get().initContext(context);
}
public static void destroy(Context context) {
RuntimeFactory.get().destroyContext(context);
}
public static Context get() {
Context ctx = RuntimeFactory.get().getContextUnchecked();
if(ctx==null) {
throw new IllegalStateException("SBT context is not initialized for the request");
}
return ctx;
}
public static Context getUnchecked() {
return RuntimeFactory.get().getContextUnchecked();
}
private Application application;
private List listeners;
protected Context(Application application) {
this.application = application;
}
public synchronized void addListener(ContextListener listener) {
if(listeners==null) {
listeners = new ArrayList();
}
listeners.add(listener);
}
public synchronized void removeListener(ContextListener listener) {
if(listeners!=null) {
listeners.remove(listener);
if(listeners.isEmpty()) {
listeners = null;
}
}
}
public void close() {
if(listeners!=null) {
for(ContextListener l: listeners) {
try {
l.close(this);
} catch(Exception ex) {
Platform.getInstance().log(ex);
}
}
}
}
public ClassLoader getClassLoader() {
return getApplication().getClassLoader();
}
public abstract String getCurrentUserId();
public boolean isCurrentUserAnonymous() {
return getCurrentUserId().equals(ANONYMOUS);
}
public abstract Object getRequest();
public abstract Object getResponse();
public final HttpServletRequest getHttpRequest() {
return (HttpServletRequest)getRequest();
}
public final HttpServletResponse getHttpResponse() {
return (HttpServletResponse)getResponse();
}
public Application getApplication() {
return application;
}
public Application getApplicationUnchecked() {
if(application==null) {
application = Application.getUnchecked();
}
return application;
}
public abstract String getProperty(String propertyName);
public abstract String getProperty(String propertyName, String defaultValue);
public abstract void setProperty(String propertyName, String value);
public abstract Object getBean(String beanName);
public abstract void sendRedirect(String redirectUrl) throws IOException;
// Find a better name for this method - getActionUrl()?
public abstract String encodeUrl(String url);
//
// Access to the scopes
//
public static final int SCOPE_NONE = 0;
public static final int SCOPE_GLOBAL = 1;
public static final int SCOPE_APPLICATION = 2;
public static final int SCOPE_SESSION = 3;
public static final int SCOPE_REQUEST = 4;
// Extensions
public static final int SCOPE_GLOBALUSER = 5;
public static final int SCOPE_APPLICATIONUSER = 6;
public Map getScope(int scope) {
switch(scope) {
case SCOPE_GLOBAL: return getGlobalMap();
case SCOPE_APPLICATION: return getApplicationMap();
case SCOPE_SESSION: return getSessionMap();
case SCOPE_REQUEST: return getRequestMap();
case SCOPE_GLOBALUSER: return getGlobalUserMap();
case SCOPE_APPLICATIONUSER: return getApplicationUserMap();
}
return null;
}
private static HashMap globalScope = new HashMap();
public Map getGlobalMap() {
return globalScope;
}
private static Map> globalUserScope = new HashMap>();
public Map getGlobalUserMap() {
String id = getCurrentUserId();
Map map = globalUserScope.get(id);
if(map==null) {
map = new HashMap();
globalUserScope.put(id, map);
}
return map;
}
protected Map createGlobalUserMap() {
return new ApplicationMap(this);
}
private Map applicationMap;
public Map getApplicationMap() {
if(applicationMap==null) {
applicationMap = createApplicationMap();
}
return applicationMap;
}
protected Map createApplicationMap() {
return new ApplicationMap(this);
}
public Map getApplicationUserMap() {
Map appMap = getApplicationMap();
String id = "_appscope_"+getCurrentUserId();
Map map = (Map)appMap.get(id);
if(map==null) {
map = new HashMap();
appMap.put(id, map);
}
return map;
}
protected Map createApplicationUserMap() {
return new ApplicationMap(this);
}
private Map sessionMap;
public Map getSessionMap() {
if(sessionMap==null) {
sessionMap = createSessionMap();
}
return sessionMap;
}
protected abstract Map createSessionMap();
private Map requestMap;
public Map getRequestMap() {
if(requestMap==null) {
requestMap = createRequestMap();
}
return requestMap;
}
protected abstract Map createRequestMap();
private Map requestParameterMap;
public Map getRequestParameterMap() {
if(requestParameterMap==null) {
requestParameterMap = createRequestParameterMap();
}
return requestParameterMap;
}
protected abstract Map createRequestParameterMap();
private Map requestCookieMap;
public Map getRequestCookieMap() {
if(requestCookieMap==null) {
requestCookieMap = createRequestCookieMap();
}
return requestCookieMap;
}
protected abstract Map createRequestCookieMap();
/**
* Utility class for creating maps.
* @author priand
*/
protected static abstract class AbstractScopeMap extends AbstractMap {
@Override
public void clear() {
throw new UnsupportedOperationException();
}
@Override
public void putAll(Map extends String,? extends Object> p) {
throw new UnsupportedOperationException();
}
@Override
public Object remove(Object key) {
throw new UnsupportedOperationException();
}
@Override
public boolean equals(Object obj) {
if (obj.getClass()==this.getClass()) {
return super.equals(obj);
}
return false;
}
protected static class MapEntry implements Map.Entry {
private final String key;
private final Object value;
public MapEntry(String key, Object value) {
this.key = key;
this.value = value;
}
@Override
public String getKey() {
return key;
}
@Override
public Object getValue() {
return value;
}
@Override
public Object setValue(Object value) {
throw new UnsupportedOperationException();
}
@Override
public int hashCode() {
return ((key == null ? 0 : key.hashCode()) ^ (value == null ? 0 : value.hashCode()));
}
@Override
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof Map.Entry)) {
return false;
}
@SuppressWarnings("unchecked")
Map.Entry input = (Map.Entry) obj;
String inputKey = input.getKey();
Object inputValue = input.getValue();
if (inputKey == key || (inputKey != null && inputKey.equals(key))) {
if (inputValue == value || (inputValue != null && inputValue.equals(value))) {
return true;
}
}
return false;
}
}
}
private static class ApplicationMap extends AbstractScopeMap {
private Context context;
ApplicationMap(Context context) {
this.context = context;
}
@Override
public Object get(Object key) {
Application app = context.getApplicationUnchecked();
return app!=null ? app.getScope().get((String)key) : null;
}
@Override
public Object put(String key, Object value) {
Application app = context.getApplication();
return app.getScope().put(key, value);
}
@Override
public Object remove(Object key) {
Application app = context.getApplicationUnchecked();
if(app!=null) {
return app.getScope().remove(key);
}
return null;
}
@Override
public Set> entrySet() {
Application app = context.getApplicationUnchecked();
if(app!=null) {
return app.getScope().entrySet();
} else {
return Collections.emptySet();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy