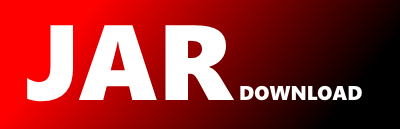
com.ibm.commons.runtime.servlet.BaseHttpServlet Maven / Gradle / Ivy
The newest version!
/*
* © Copyright IBM Corp. 2012
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.ibm.commons.runtime.servlet;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.sql.SQLException;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.ibm.commons.Platform;
import com.ibm.commons.runtime.Application;
import com.ibm.commons.runtime.util.UrlUtil;
import com.ibm.commons.util.HtmlTextUtil;
import com.ibm.commons.util.StringUtil;
/**
* Provides a base class for HTTP servlets. BaseHttpServlet
provides utility methods
* for returning standard responses and handling exceptions.
*
* @author priand
* @author mwallace
*
*/
@SuppressWarnings("deprecation")
abstract public class BaseHttpServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @param config
* @param paramToolkitUrl
* @param defaultToolkitUrl
* @return
*/
protected String getInitParameter(ServletConfig config, String name, String defaultValue) {
String value = config.getInitParameter(name);
return StringUtil.isEmpty(value) ? defaultValue : value;
}
/**
*
* @param application
* @param name
* @param defaultValue
* @return
*/
protected String getAppParameter(Application application, String name, String defaultValue) {
String value = application.getProperty(name);
return StringUtil.isEmpty(value) ? defaultValue : value;
}
protected static PrintWriter getWriter(HttpServletResponse response) throws IOException {
// Use what is available
try {
return response.getWriter();
} catch (IllegalStateException ex) {
}
return new PrintWriter(new OutputStreamWriter(response.getOutputStream(), "utf-8"));
}
protected void infoServletContext(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
response.setStatus(HttpServletResponse.SC_OK);
boolean rtl = isErrorPageRTL(request, response);
String title = getErrorPageTitle(request, response);
String lang = getErrorPageLang(request, response);
PrintWriter w = getWriter(response);
try {
writeServletContext(request, w, title, lang, rtl);
} finally {
w.flush();
}
} catch (Throwable t) {
Platform.getInstance().log(t);
}
}
private void writeServletContext(HttpServletRequest request, PrintWriter w, String title, String lang, boolean isRTL) throws IOException {
String dir = isRTL ? "rtl" : "ltr";
if (lang != null) {
w.println("");
} else {
w.println("");
}
w.println("");
w.println("" + title + " ");
w.println("");
w.println("");
w.println("");
w.println(" ");
w.println(" ServletPath ");
w.print(" ");
w.print(HtmlTextUtil.toHTMLContentString(request.getServletPath(), true));
w.println(" ");
w.println(" ");
w.println(" ");
w.println(" ContextPath ");
w.print(" ");
w.print(HtmlTextUtil.toHTMLContentString(request.getContextPath(), true));
w.println(" ");
w.println(" ");
w.println(" ");
w.println(" PathInfo ");
w.print(" ");
w.print(HtmlTextUtil.toHTMLContentString(request.getPathInfo(), true));
w.println(" ");
w.println(" ");
w.println(" ");
w.println(" QueryString ");
w.print(" ");
w.print(HtmlTextUtil.toHTMLContentString(request.getQueryString(), true));
w.println(" ");
w.println(" ");
w.println("
");
w.println("");
w.println("");
}
public static boolean isErrorPageRTL(HttpServletRequest request, HttpServletResponse response) {
return false;
}
public static String getErrorPageLang(HttpServletRequest request, HttpServletResponse response) {
return null;
}
public static String getErrorPageTitle(HttpServletRequest request, HttpServletResponse response) {
return "Error Page";
}
/**
* Send a 404 error response with the specified formatted message.
*
* @param request
* @param response
* @param fmt
* @param parameters
*
* @throws ServletException
* @throws IOException
*/
public static void service400(HttpServletRequest request, HttpServletResponse response, String fmt, Object...parameters) throws ServletException, IOException {
String s = StringUtil.format(fmt, parameters);
boolean rtl = isErrorPageRTL(request, response);
String title = getErrorPageTitle(request, response);
String lang = getErrorPageLang(request, response);
response.reset();
response.setStatus(HttpServletResponse.SC_BAD_REQUEST);
response.setContentType( "text/html" );
PrintWriter w = getWriter(response);
try {
w.println("");
w.println("");
w.println("Error 400
");
w.println(StringUtil.format("URL {0}",UrlUtil.getRequestUrl(request)));
w.println("
");
w.println("
");
w.println(HtmlTextUtil.toHTMLContentString(s, true));
w.println("");
w.println("");
} finally {
w.flush();
}
}
/**
* Send a 404 error response with the specified formatted message.
*
* @param request
* @param response
* @param fmt
* @param parameters
*
* @throws ServletException
* @throws IOException
*/
public static void service404(HttpServletRequest request, HttpServletResponse response, String fmt, Object...parameters) throws ServletException, IOException {
String s = StringUtil.format(fmt, parameters);
response.reset();
response.setStatus(HttpServletResponse.SC_NOT_FOUND);
response.setContentType( "text/html" );
PrintWriter w = getWriter(response);
try {
w.println("");
w.println("");
w.println("Error 404
");
w.println(StringUtil.format("URL {0}",UrlUtil.getRequestUrl(request)));
w.println("
");
w.println("
");
w.println(HtmlTextUtil.toHTMLContentString(s, true));
w.println("");
w.println("");
} finally {
w.flush();
}
}
/**
* Send a 500 error response with the specified formatted message.
*
* @param request
* @param response
* @param fmt
* @param parameters
*
* @throws ServletException
* @throws IOException
*/
public static void service500(HttpServletRequest request, HttpServletResponse response, String fmt, Object...parameters) throws ServletException, IOException {
String s = StringUtil.format(fmt, parameters);
response.reset();
response.setStatus(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
response.setContentType( "text/html" ); //$NON-NLS-1$
PrintWriter w = getWriter(response);
try {
w.println("");
w.println("");
w.println("Error 500
");
w.println(StringUtil.format("URL {0}",UrlUtil.getRequestUrl(request)));
w.println("
");
w.println("
");
w.println(HtmlTextUtil.toHTMLContentString(s, true));
w.println("");
w.println("");
} finally {
w.flush();
}
}
/**
* Send a service exception response message.
*
* @param request
* @param response
* @param t
* @param lang
* @param isRTL
*
* @throws IOException
* @throws ServletException
*/
public static void serviceException(HttpServletRequest request, HttpServletResponse response, Throwable t) throws IOException, ServletException {
boolean rtl = isErrorPageRTL(request, response);
String lang = getErrorPageLang(request, response);
serviceException(request, response, t, lang, rtl);
}
public static void serviceException(HttpServletRequest request, HttpServletResponse response, Throwable t, String lang, boolean isRTL) throws IOException, ServletException {
response.reset();
response.setStatus(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
response.setContentType( "text/html" ); //$NON-NLS-1$
PrintWriter writer = getWriter(response);
writeErrorPageHeader(writer, "Runtime Error", lang, isRTL);
writer.println(""+"Unexpected runtime error"+"
");
writer.println("The runtime has encountered an unexpected error."+"
");
writer.println(StringUtil.format("URL {0}",UrlUtil.getRequestUrl(request)));
writer.println("
");
writer.println("
");
boolean verbose = true;
if(verbose) {
writeExceptionMessage(writer, t);
writeExceptionTrace(writer, t, isRTL);
}
writeErrorPageFooter(writer);
writer.flush();
}
//
// Internals
//
private static void writeErrorPageHeader(PrintWriter printWriter, String title, String lang, boolean isRTL) throws IOException {
String leading = "left";
String dir = "ltr";
if(isRTL){
leading = "right";
dir = "rtl";
}
if(lang != null){
printWriter.println("");
}else{
printWriter.println("");
}
printWriter.println("");
printWriter.println(""+title+" ");
printWriter.println("");
printWriter.println("");
printWriter.println("");
printWriter.println("");
}
private static void writeErrorPageFooter(PrintWriter printWriter) throws IOException {
printWriter.println("");
printWriter.println("");
}
private static void writeExceptionMessage(PrintWriter printWriter, Throwable t) {
printWriter.println("
"+"Exception"+"
");
String lastMessage = null; // Do not duplicate messages...
for (Throwable tt=t; tt != null; tt = getCause(tt)) {
String s = tt.getLocalizedMessage();
if (StringUtil.isNotEmpty(s) && !StringUtil.equals(lastMessage, s)) {
printWriter.print(""+s+"");
printWriter.println("
");
lastMessage = s;
}
}
}
private static void writeExceptionTrace(PrintWriter writer, Throwable e, boolean isRTL) {
String leading = "left";
String stackIcon = "►";
if(isRTL){
leading = "right";
stackIcon = "◄";
}
writer.println("");
writer.println("" + stackIcon + "");
writer.println(" ");
writer.println(""+"Stack Trace"+"
");
writer.println("
");
writer.println("");
}
private static void writeExceptionTrace2(PrintWriter writer, Throwable e, int rows) {
if(e!=null) {
StackTraceElement[] elt = e.getStackTrace();
writer.println(e.toString());
for(int i=0; i200) {
return;
}
}
writeExceptionTrace2(writer, getCause(e), rows);
}
}
private static Throwable getCause(Throwable ext) {
if(ext instanceof ServletException) {
Throwable cause = ((ServletException)ext).getRootCause();
if(cause!=null) {
return cause;
}
}
if(ext instanceof SQLException) {
Throwable cause = ((SQLException)ext).getNextException();
if(cause!=null) {
return cause;
}
}
Throwable cause = ext.getCause();
return cause;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy