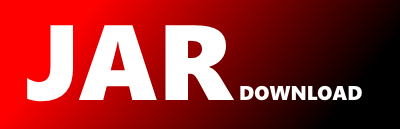
org.openpnp.capture.library.OpenpnpCaptureLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openpnp-capture-java Show documentation
Show all versions of openpnp-capture-java Show documentation
OpenPnP Capture Java with all binaries included, packaged for Maven.
package org.openpnp.capture.library;
import com.sun.jna.Library;
import com.sun.jna.Native;
import com.sun.jna.NativeLibrary;
import com.sun.jna.Pointer;
import com.sun.jna.ptr.IntByReference;
import java.nio.IntBuffer;
/**
* JNA Wrapper for library openpnp-capture
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java , Rococoa, or JNA.
*/
public interface OpenpnpCaptureLibrary extends Library {
public static final String JNA_LIBRARY_NAME = "openpnp-capture";
public static final NativeLibrary JNA_NATIVE_LIB = NativeLibrary.getInstance(OpenpnpCaptureLibrary.JNA_LIBRARY_NAME);
public static final OpenpnpCaptureLibrary INSTANCE = (OpenpnpCaptureLibrary)Native.loadLibrary(OpenpnpCaptureLibrary.JNA_LIBRARY_NAME, OpenpnpCaptureLibrary.class);
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_EXPOSURE = (int)1;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_FOCUS = (int)2;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_ZOOM = (int)3;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_WHITEBALANCE = (int)4;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_GAIN = (int)5;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_BRIGHTNESS = (int)6;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_CONTRAST = (int)7;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_SATURATION = (int)8;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_GAMMA = (int)9;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPPROPID_LAST = (int)10;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPRESULT_OK = (int)0;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPRESULT_ERR = (int)1;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPRESULT_DEVICENOTFOUND = (int)2;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPRESULT_FORMATNOTSUPPORTED = (int)3;
/** native declaration : openpnp-capture/include/openpnp-capture.h */
public static final int CAPRESULT_PROPERTYNOTSUPPORTED = (int)4;
/**
* Initialize the capture library
* @return The context ID.
* Original signature : CapContext Cap_createContext()
* native declaration : openpnp-capture/include/openpnp-capture.h:75
*/
Pointer Cap_createContext();
/**
* Un-initialize the capture library context
* @param ctx The ID of the context to destroy.
* @return The context ID.
* Original signature : CapResult Cap_releaseContext(CapContext)
* native declaration : openpnp-capture/include/openpnp-capture.h:81
*/
int Cap_releaseContext(Pointer ctx);
/**
* Get the number of capture devices on the system.
* note: this can change dynamically due to the
* pluggin and unplugging of USB devices.
* @param ctx The ID of the context.
* @return The number of capture devices found.
* Original signature : uint32_t Cap_getDeviceCount(CapContext)
* native declaration : openpnp-capture/include/openpnp-capture.h:89
*/
int Cap_getDeviceCount(Pointer ctx);
/**
* Get the name of a capture device.
* This name is meant to be displayed in GUI applications,
* i.e. its human readable.
* if a device with the given index does not exist,
* NULL is returned.
* @param ctx The ID of the context.
* @param index The device index of the capture device.
* @return a pointer to an UTF-8 string containting the name of the capture device.
* Original signature : char* Cap_getDeviceName(CapContext, CapDeviceID)
* native declaration : openpnp-capture/include/openpnp-capture.h:101
*/
Pointer Cap_getDeviceName(Pointer ctx, int index);
/**
* Get the unique name of a capture device.
* The string contains a unique concatenation
* of the device name and other parameters.
* These parameters are platform dependent.
* Note: when a USB camera does not expose a serial number,
* platforms might have trouble uniquely identifying
* a camera. In such cases, the USB port location can
* be used to add a unique feature to the string.
* This, however, has the down side that the ID of
* the camera changes when the USB port location
* changes. Unfortunately, there isn't much to
* do about this.
* if a device with the given index does not exist,
* NULL is returned.
* @param ctx The ID of the context.
* @param index The device index of the capture device.
* @return a pointer to an UTF-8 string containting the unique ID of the capture device.
* Original signature : char* Cap_getDeviceUniqueID(CapContext, CapDeviceID)
* native declaration : openpnp-capture/include/openpnp-capture.h:123
*/
Pointer Cap_getDeviceUniqueID(Pointer ctx, int index);
/**
* Returns the number of formats supported by a certain device.
* returns -1 if device does not exist.
* @param ctx The ID of the context.
* @param index The device index of the capture device.
* @return The number of formats supported or -1 if the device does not exist.
* Original signature : int32_t Cap_getNumFormats(CapContext, CapDeviceID)
* native declaration : openpnp-capture/include/openpnp-capture.h:133
*/
int Cap_getNumFormats(Pointer ctx, int index);
/**
* Get the format information from a device.
* @param ctx The ID of the context.
* @param index The device index of the capture device.
* @param id The index/ID of the frame buffer format (0 .. number returned by Cap_getNumFormats() minus 1 ).
* @param info pointer to a CapFormatInfo structure to be filled with data.
* @return The CapResult.
* Original signature : CapResult Cap_getFormatInfo(CapContext, CapDeviceID, CapFormatID, CapFormatInfo*)
* native declaration : openpnp-capture/include/openpnp-capture.h:142
*/
int Cap_getFormatInfo(Pointer ctx, int index, int id, CapFormatInfo info);
/**
* Open a capture stream to a device with specific format requirements
* Although the (internal) frame buffer format is set via the fourCC ID,
* the frames returned by Cap_captureFrame are always 24-bit RGB.
* @param ctx The ID of the context.
* @param index The device index of the capture device.
* @param formatID The index/ID of the frame buffer format (0 .. number returned by Cap_getNumFormats() minus 1 ).
* @return The stream ID or -1 if the device does not exist or the stream format ID is incorrect.
* Original signature : CapStream Cap_openStream(CapContext, CapDeviceID, CapFormatID)
* native declaration : openpnp-capture/include/openpnp-capture.h:159
*/
int Cap_openStream(Pointer ctx, int index, int formatID);
/**
* Close a capture stream
* @param ctx The ID of the context.
* @param stream The stream ID.
* @return CapResult
* Original signature : CapResult Cap_closeStream(CapContext, CapStream)
* native declaration : openpnp-capture/include/openpnp-capture.h:166
*/
int Cap_closeStream(Pointer ctx, int stream);
/**
* Check if a stream is open, i.e. is capturing data.
* @param ctx The ID of the context.
* @param stream The stream ID.
* @return 1 if the stream is open and capturing, else 0.
* Original signature : uint32_t Cap_isOpenStream(CapContext, CapStream)
* native declaration : openpnp-capture/include/openpnp-capture.h:173
*/
int Cap_isOpenStream(Pointer ctx, int stream);
/**
* this function copies the most recent RGB frame data
* to the given buffer.
* Original signature : CapResult Cap_captureFrame(CapContext, CapStream, void*, uint32_t)
* native declaration : openpnp-capture/include/openpnp-capture.h:182
*/
int Cap_captureFrame(Pointer ctx, int stream, Pointer RGBbufferPtr, int RGBbufferBytes);
/**
* returns 1 if a new frame has been captured, 0 otherwise
* Original signature : uint32_t Cap_hasNewFrame(CapContext, CapStream)
* native declaration : openpnp-capture/include/openpnp-capture.h:185
*/
int Cap_hasNewFrame(Pointer ctx, int stream);
/**
* returns the number of frames captured during the lifetime of the stream.
* For debugging purposes
* Original signature : uint32_t Cap_getStreamFrameCount(CapContext, CapStream)
* native declaration : openpnp-capture/include/openpnp-capture.h:189
*/
int Cap_getStreamFrameCount(Pointer ctx, int stream);
/**
* get the min/max limits of a camera/stream property (e.g. zoom, exposure etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid.
* Original signature : CapResult Cap_getPropertyLimits(CapContext, CapStream, CapPropertyID, int32_t*, int32_t*)
* native declaration : openpnp-capture/include/openpnp-capture.h:202
* @deprecated use the safer methods {@link #Cap_getPropertyLimits(com.sun.jna.Pointer, int, int, java.nio.IntBuffer, java.nio.IntBuffer)} and {@link #Cap_getPropertyLimits(com.sun.jna.Pointer, int, int, com.sun.jna.ptr.IntByReference, com.sun.jna.ptr.IntByReference)} instead
*/
@Deprecated
int Cap_getPropertyLimits(Pointer ctx, int stream, int propID, IntByReference min, IntByReference max);
/**
* get the min/max limits of a camera/stream property (e.g. zoom, exposure etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid.
* Original signature : CapResult Cap_getPropertyLimits(CapContext, CapStream, CapPropertyID, int32_t*, int32_t*)
* native declaration : openpnp-capture/include/openpnp-capture.h:202
*/
int Cap_getPropertyLimits(Pointer ctx, int stream, int propID, IntBuffer min, IntBuffer max);
/**
* set the value of a camera/stream property (e.g. zoom, exposure etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid.
* Original signature : CapResult Cap_setProperty(CapContext, CapStream, CapPropertyID, int32_t)
* native declaration : openpnp-capture/include/openpnp-capture.h:210
*/
int Cap_setProperty(Pointer ctx, int stream, int propID, int value);
/**
* set the automatic flag of a camera/stream property (e.g. zoom, focus etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid.
* Original signature : CapResult Cap_setAutoProperty(CapContext, CapStream, CapPropertyID, uint32_t)
* native declaration : openpnp-capture/include/openpnp-capture.h:218
*/
int Cap_setAutoProperty(Pointer ctx, int stream, int propID, int bOnOff);
/**
* get the value of a camera/stream property (e.g. zoom, exposure etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid or outValue == NULL.
* Original signature : CapResult Cap_getProperty(CapContext, CapStream, CapPropertyID, int32_t*)
* native declaration : openpnp-capture/include/openpnp-capture.h:226
* @deprecated use the safer methods {@link #Cap_getProperty(com.sun.jna.Pointer, int, int, java.nio.IntBuffer)} and {@link #Cap_getProperty(com.sun.jna.Pointer, int, int, com.sun.jna.ptr.IntByReference)} instead
*/
@Deprecated
int Cap_getProperty(Pointer ctx, int stream, int propID, IntByReference outValue);
/**
* get the value of a camera/stream property (e.g. zoom, exposure etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid or outValue == NULL.
* Original signature : CapResult Cap_getProperty(CapContext, CapStream, CapPropertyID, int32_t*)
* native declaration : openpnp-capture/include/openpnp-capture.h:226
*/
int Cap_getProperty(Pointer ctx, int stream, int propID, IntBuffer outValue);
/**
* get the automatic flag of a camera/stream property (e.g. zoom, focus etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid.
* Original signature : CapResult Cap_getAutoProperty(CapContext, CapStream, CapPropertyID, uint32_t*)
* native declaration : openpnp-capture/include/openpnp-capture.h:234
* @deprecated use the safer methods {@link #Cap_getAutoProperty(com.sun.jna.Pointer, int, int, java.nio.IntBuffer)} and {@link #Cap_getAutoProperty(com.sun.jna.Pointer, int, int, com.sun.jna.ptr.IntByReference)} instead
*/
@Deprecated
int Cap_getAutoProperty(Pointer ctx, int stream, int propID, IntByReference outValue);
/**
* get the automatic flag of a camera/stream property (e.g. zoom, focus etc)
* returns: CAPRESULT_OK if all is well.
* CAPRESULT_PROPERTYNOTSUPPORTED if property not available.
* CAPRESULT_ERR if context, stream are invalid.
* Original signature : CapResult Cap_getAutoProperty(CapContext, CapStream, CapPropertyID, uint32_t*)
* native declaration : openpnp-capture/include/openpnp-capture.h:234
*/
int Cap_getAutoProperty(Pointer ctx, int stream, int propID, IntBuffer outValue);
/**
* Set the logging level.
* LOG LEVEL ID | LEVEL
* ------------- | -------------
* LOG_EMERG | 0
* LOG_ALERT | 1
* LOG_CRIT | 2
* LOG_ERR | 3
* LOG_WARNING | 4
* LOG_NOTICE | 5
* LOG_INFO | 6
* LOG_DEBUG | 7
* LOG_VERBOSE | 8
* Original signature : void Cap_setLogLevel(uint32_t)
* native declaration : openpnp-capture/include/openpnp-capture.h:256
*/
void Cap_setLogLevel(int level);
/**
* Return the version of the library as a string.
* In addition to a version number, this should
* contain information on the platform,
* e.g. Win32/Win64/Linux32/Linux64/OSX etc,
* wether or not it is a release or debug
* build and the build date.
* When building the library, please set the
* following defines in the build environment:
* __LIBVER__
* __PLATFORM__
* __BUILDTYPE__
* Original signature : char* Cap_getLibraryVersion()
* native declaration : openpnp-capture/include/openpnp-capture.h:274
*/
Pointer Cap_getLibraryVersion();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy