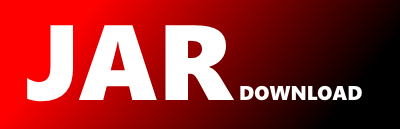
org.openpreservation.messages.Message Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odf-core Show documentation
Show all versions of odf-core Show documentation
API and library for open source validation of Open Document Format spreadsheets.
The newest version!
package org.openpreservation.messages;
import java.util.Date;
/**
* Defines behaviour of validation messages.
*
* These messages have a unique string identifier as well as
* the previous message and sub-message strings.
*
* @author Carl Wilson
* carlwilson AT github
*/
public interface Message {
/**
* An enum set that defines the severity of a message.
*/
public enum Severity {
/**
* The message is informational, equivalent to MAY
*/
INFO,
/**
* The message is a warning, equivalent to SHOULD
*/
WARNING,
/**
* The message is an error, equivalent to MUST
*/
ERROR,
/**
* The message is a fatal error, usually an system issue
*/
FATAL;
/**
* The label for the severity, lower case
*/
public final String label;
private Severity() {
this.label = this.name().toLowerCase();
}
}
/**
* Get the unique, persistent message identifier.
*
* @return the String
message id.
*/
public String getId();
/**
* Get the message timestamp
*
* @return the {@link Date} timestamp of the message, or {@code null} if not set
*/
public Date getTimestamp();
/**
* Get the message severity
*
* @return the {@link Severity} of the message
*/
public Severity getSeverity();
/**
* Get the main message
*
* @return the String
message
*/
public String getMessage();
/**
* Test whether the message has a sub-message
*
* @return true
if the message has a sub-message,
* false
otherwise
*/
public boolean hasSubMessage();
/**
* Get the sub-message
*
* @return the String
sub-message
*/
public String getSubMessage();
/**
* Test whether the message has {@link Severity} ERROR.
*
* @return true
if the message has Severity.ERROR
,
* false
otherwise
*/
public boolean isError();
/**
* Test whether the message has {@link Severity} FATAL.
*
* @return true
if the message has Severity.FATAL
,
* false
otherwise
*/
public boolean isFatal();
/**
* Test whether the message has {@link Severity} INFO.
*
* @return true
if the message has Severity.INFO
,
* false
otherwise
*/
public boolean isInfo();
/**
* Test whether the message has {@link Severity} WARNING.
*
* @return true
if the message has Severity.WARNING
,
* false
otherwise
*/
public boolean isWarning();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy