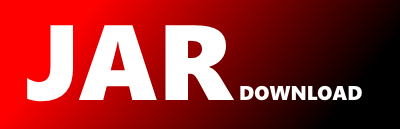
org.openprovenance.prov.template.compiler.CompilerDocumentation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prov-template-compiler Show documentation
Show all versions of prov-template-compiler Show documentation
A template system for PROV bundles.
The newest version!
package org.openprovenance.prov.template.compiler;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.networknt.schema.JsonSchema;
import com.networknt.schema.ValidationMessage;
import org.openprovenance.prov.model.ProvFactory;
import org.openprovenance.prov.template.compiler.configuration.TemplatesProjectConfiguration;
import org.openprovenance.prov.template.descriptors.AttributeDescriptor;
import org.openprovenance.prov.template.descriptors.Descriptor;
import org.openprovenance.prov.template.descriptors.NameDescriptor;
import org.openprovenance.prov.template.descriptors.TemplateBindingsSchema;
import java.io.*;
import java.util.*;
import static org.openprovenance.prov.template.compiler.CompilerJsonSchema.convertToJsonType;
import static org.openprovenance.prov.template.compiler.ConfigProcessor.descriptorUtils;
public class CompilerDocumentation {
private final CompilerUtil compilerUtil;
ObjectMapper om = new ObjectMapper();
Map jsonSchemaAsAMap = initializeSchemaMap();
public CompilerDocumentation(ProvFactory pFactory) {
this.compilerUtil=new CompilerUtil(pFactory);
}
public Map initializeSchemaMap() {
final HashMap res = new HashMap<>();
res.put("definitions", new HashMap());
res.put("allOf", new LinkedList>());
res.put("type", "object");
return res;
}
public PrintStream getOutputStream() {
return os;
}
private PrintStream os=null;
public void generateDocumentation(String documentationFile, String templateName, String root_dir, TemplateBindingsSchema bindingsSchema, List sharing) {
if (os==null) {
new File(root_dir).mkdirs();
}
final String path = root_dir + "/" + documentationFile;
try {
if (os==null) {
os = new PrintStream(new FileOutputStream(path));
os.println("");
os.println("");
os.println("");
os.println(" ");
os.println(" ");
os.println(" ");
os.println("");
}
Map> theVar= bindingsSchema.getVar();
String docString=bindingsSchema.getDocumentation();
if (docString==null) {
docString="No @documentation.";
}
os.println("" );
os.println(""+templateName+"
");
os.println("");
os.println(docString);
os.println("");
os.println("");
int column=0;
List required=new LinkedList<>();
required.add("isA");
os.println("- ");
os.println(makeSpan(String.valueOf(column), "csv_column"));
os.println(makeSpan("" + templateName+ "", "csv_field_header")+":");
os.println(makeSpan("Constant field for the name of this template","csv_doc"));
column++;
os.println("
");
for (String key: descriptorUtils.fieldNames(bindingsSchema)) {
required.add(key);
os.println("- ");
os.println(makeSpan(String.valueOf(column), "csv_column"));
os.println(makeSpan(key, "csv_field"));
final String jsonType = convertToJsonType(compilerUtil.getJavaTypeForDeclaredType(theVar, key).getName());
os.print(makeSpan(jsonType, "csv_type"));
if (descriptorUtils.isInput(key,bindingsSchema)) {
String input="";
input=input+"I";
if (descriptorUtils.isCompulsoryInput(key,bindingsSchema)) {
input=input+"*";
}
os.println(makeSpan(input, "csv_input"));
}
if (descriptorUtils.isOutput(key,bindingsSchema)) {
String output="O";
os.println(makeSpan(output, "csv_output"));
}
if (sharing!=null && sharing.contains(key)) {
os.print(makeSpan("share-able variable", "csv_shared"));
}
os.println(":");
Descriptor descriptor=theVar.get(key).get(0);
String documentation=descriptorUtils.getFromDescriptor(descriptor, AttributeDescriptor::getDocumentation, NameDescriptor::getDocumentation);
if (documentation==null) {
documentation="";
}
os.println(makeSpan(documentation,"csv_doc"));
os.println("
");
column++;
}
os.println("
");
os.println("");
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
public String makeSpan(String key, String classe) {
return ""+key+" ";
}
public Object defaultValue(String jsonType) {
switch (jsonType) {
case "integer": return 0;
case "float": return 0.0;
}
return null;
}
public String title(String key) {
//return "The Schema for " + key;
return key;
}
boolean draft04=false;
public String get$id() {
if (draft04) {
return "id";
}
return "$id";
}
public void generateDocumentationEnd(TemplatesProjectConfiguration configs, String cli_webjar_dir) {
if (os!=null) { // insure this had been initialised
os.println("");
os.println("");
getOutputStream().close();
}
}
/*
public JsonSchema setupJsonSchemaCheck() throws ProcessingException, IOException {
return setupJsonSchemaCheck("src/main/resources/schema/json-schema-v4.json");
}
public JsonSchema setupJsonSchemaCheck(String file) throws
IOException, ProcessingException {
final JsonNode schemaJSON = JsonLoader.fromPath(file);
final JsonSchemaFactory factory = JsonSchemaFactory.byDefault();
JsonSchema schema = factory.getJsonSchema(schemaJSON);
return schema;
}
public boolean checkSchema(JsonSchema schema, String file) throws IOException, ProcessingException {
System.out.println("Loading " + file);
final JsonNode fileJSON = JsonLoader.fromPath(file);
ProcessingReport report = schema.validate(fileJSON);
if (!report.isSuccess()) {
System.err.println("Cannot validate " + file + " against the PROV-JSON schema.");
System.err.println(report);
} else {
System.out.println(report);
}
return (report.isSuccess());
}
*/
static class Tester extends JsonSchemaTesting {
@Override
public JsonSchema getJsonSchemaFromClasspath(String name) {
return super.getJsonSchemaFromClasspath(name);
}
@Override
public JsonSchema getJsonSchemaFromClasspathV7(String name) {
return super.getJsonSchemaFromClasspathV7(name);
}
@Override
protected JsonSchema getJsonSchemaFromFile(String name) throws FileNotFoundException {
return super.getJsonSchemaFromFile(name);
}
}
final Tester tester=new Tester();
public JsonSchema setupJsonSchemaFromClasspath(String file) {
System.out.println("### getting schema from classpath " + file);
JsonSchema schema2=tester.getJsonSchemaFromClasspath(file);
return schema2;
}
public JsonSchema setupJsonSchemaFromClasspathV7(String file) {
System.out.println("### getting schema from classpath " + file);
JsonSchema schema2=tester.getJsonSchemaFromClasspathV7(file);
return schema2;
}
public JsonSchema setupJsonSchemaFromFile(String file) throws FileNotFoundException {
System.out.println("#### getting schema from File " + file);
JsonSchema schema2=tester.getJsonSchemaFromFile(file);
return schema2;
}
public Set checkSchema(JsonSchema schema, String file) throws IOException {
JsonNode node = om.readTree(new File(file));
Set errors = schema.validate(node);
return errors;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy