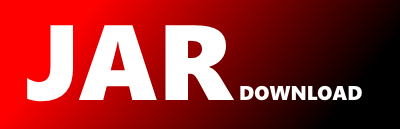
org.openprovenance.prov.template.compiler.CompilerJsonSchema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of prov-template-compiler Show documentation
Show all versions of prov-template-compiler Show documentation
A template system for PROV bundles.
The newest version!
package org.openprovenance.prov.template.compiler;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.networknt.schema.JsonSchema;
import com.networknt.schema.ValidationMessage;
import org.openprovenance.prov.model.ProvFactory;
import org.openprovenance.prov.template.descriptors.AttributeDescriptor;
import org.openprovenance.prov.template.descriptors.Descriptor;
import org.openprovenance.prov.template.descriptors.NameDescriptor;
import org.openprovenance.prov.template.descriptors.TemplateBindingsSchema;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.*;
import static org.openprovenance.prov.template.compiler.ConfigProcessor.descriptorUtils;
import static org.openprovenance.prov.template.compiler.common.Constants.ELEMENTS;
public class CompilerJsonSchema {
private final CompilerUtil compilerUtil;
private final ProvFactory pFactory;
ObjectMapper om = new ObjectMapper();
Map jsonSchemaAsAMap = initializeSchemaMap();
public CompilerJsonSchema(ProvFactory pFactory) {
this.compilerUtil=new CompilerUtil(pFactory);
this.pFactory=pFactory;
}
public void generateJSonSchemaEnd(String jsonschema, String root_dir) {
new File(root_dir).mkdirs();
final String path = root_dir + "/" + jsonschema;
jsonSchemaAsAMap.put(get$id(), "https://openprovenance.org/template/beans#" + jsonschema);
jsonSchemaAsAMap.put("type", "object");
jsonSchemaAsAMap.put("title", "The Root Schema");
try {
om.writerWithDefaultPrettyPrinter().writeValue(new File(path), jsonSchemaAsAMap);
} catch (IOException e) {
e.printStackTrace();
}
}
public Map initializeSchemaMap() {
final HashMap res = new HashMap<>();
res.put("definitions", new HashMap());
res.put("allOf", new LinkedList>());
res.put("type", "object");
return res;
}
public void generateJSonSchema(String templateName, TemplateBindingsSchema bindingsSchema, String consistsOf, String idPrefix, List sharing) {
Map> theVar=bindingsSchema.getVar();
Collection variables=descriptorUtils.fieldNames(bindingsSchema);
Map aSchema = new HashMap<>();
aSchema.put(get$id(), idPrefix + templateName);
aSchema.put("type", "object");
Map properties = new HashMap<>();
aSchema.put("properties", properties);
List requiredProperties=new LinkedList<>();
requiredProperties.add("isA");
for (String key: variables) {
requiredProperties.add(key);
Map atype = new HashMap<>();
atype.put(get$id(), idPrefix +templateName+"/properties/"+key);
atype.put("title", title(key));
final String jsonType = convertToJsonType(compilerUtil.getJavaTypeForDeclaredType(theVar, key).getName());
atype.put("type", jsonType);
//final Object defaultValue=defaultValue(jsonType);
//if (defaultValue!=null) atype.put("default", defaultValue);
//atype.put("required", true);
// LUC FIXME: should be generated for composite only, and subtype needs to be adjusted.
if (consistsOf!=null && key.equals("type")) {
atype.put("readOnly", "true");
atype.put("default", consistsOf);
}
Descriptor descriptor=theVar.get(key).get(0);
String documentation=descriptorUtils.getFromDescriptor(descriptor, AttributeDescriptor::getDocumentation, NameDescriptor::getDocumentation);
//String type=descriptorUtils.getFromDescriptor(descriptor, AttributeDescriptor::getType, NameDescriptor::getType);
if (documentation==null) documentation="";
atype.put("description", documentation + " (" + jsonType + ")" );
properties.put(key, atype);
}
if (consistsOf!=null) {
String elementKey = ELEMENTS;
requiredProperties.add(elementKey);
Map atype2 = new HashMap<>();
atype2.put(get$id(), idPrefix + templateName + "/properties/" + elementKey);
atype2.put("title", title(elementKey));
atype2.put("type", "array");
// jsonform does not support $ref in items, so we need to expand the schema
Mapsubschema= (Map) ((Map)jsonSchemaAsAMap.get("definitions")).get(consistsOf);
Map subschema2 = (subschema==null)? new HashMap<>(): new HashMap<>(subschema);
// note: the $id are not correct
subschema2.put("title", consistsOf + " {{idx}}");
Map propertiesMap = (Map) subschema2.get("properties");
for (String sharingKey: sharing) {
Map sharedKeyMap = (Map) propertiesMap.get(sharingKey);
sharedKeyMap.put("required", "true");
if ("integer".equals(sharedKeyMap.get("type"))) {
sharedKeyMap.put("maximum", -1);
sharedKeyMap.put("description", ")properties.get("count")).put("readOnly", "true");
}
String key="isA";
Map atype = new HashMap<>();
atype.put(get$id(), idPrefix +templateName+"/properties/"+key);
atype.put("title", title(key));
atype.put("type", "string");
atype.put("readOnly", "true");
atype.put("default", templateName);
atype.put("pattern", "^" + templateName + "$");
properties.put(key, atype);
atype.put("required", true);
jsonSchemaAsAMap.put("properties", Map.of("isA", Map.of("type", "string", "pattern", "^template_block$"))); // Luc Allow more templates
aSchema.put("required", requiredProperties);
aSchema.put("additionalProperties", false);
((Map)jsonSchemaAsAMap.get("definitions")).put(templateName, aSchema);
Map ifThenBlock=new HashMap<>();
Map ifBlock=new HashMap<>();
ifThenBlock.put("if",ifBlock);
ifThenBlock.put("then", Map.of("$ref", idPrefix + templateName));
ifBlock.put("type", "object");
ifBlock.put("properties", Map.of("isA", Map.of("const", templateName)));
((List " + ((String)sharedKeyMap.get("description")).replace("integer", " "));
} else {
sharedKeyMap.put("description", " " + sharedKeyMap.get("description"));
}
}
atype2.put("items",subschema2);
properties.put(elementKey, atype2);
((Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy