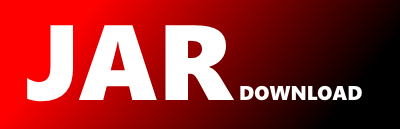
org.openrdf.console.PrintHelp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sesame-console Show documentation
Show all versions of sesame-console Show documentation
Command line user interface to Sesame repositories.
The newest version!
/*
* Licensed to Aduna under one or more contributor license agreements.
* See the NOTICE.txt file distributed with this work for additional
* information regarding copyright ownership.
*
* Aduna licenses this file to you under the terms of the Aduna BSD
* License (the "License"); you may not use this file except in compliance
* with the License. See the LICENSE.txt file distributed with this work
* for the full License.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package org.openrdf.console;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
/**
* Prints help to the console.
*
* @author Dale Visser
*/
public class PrintHelp implements Command {
private static final String USAGE = "Usage:\n";
protected static final String CONNECT = USAGE
+ "connect default Opens the default repository set for this console\n"
+ "connect Opens the repository set in the specified data dir\n"
+ "connect [user [password]] Connects to a Sesame server with optional credentials\n";
protected static final String CREATE = USAGE + "create \n"
+ " The name of a repository configuration template\n";
private static final String DISCONNECT = USAGE
+ "disconnect Disconnects from the current set of repositories or server\n";
protected static final String DROP = USAGE
+ "drop Drops the repository with the specified id\n";
protected static final String INFO = USAGE
+ "info Shows information about the console\n";
protected static final String OPEN = USAGE
+ "open Opens the repository with the specified ID\n";
protected static final String CLOSE = USAGE + "close Closes the current repository\n";
protected static final String SHOW = USAGE + "show {r, repositories} Shows all available repositories\n"
+ "show {n, namespaces} Shows all namespaces\n"
+ "show {c, contexts} Shows all context identifiers\n";
protected static final String LOAD = USAGE
+ "load [from ] [into ]\n"
+ " The path or URL identifying the data file\n"
+ " The base URI to use for resolving relative references, defaults to \n"
+ " The ID of the context to add the data to, e.g. foo:bar or _:n123\n"
+ "Loads the specified data file into the current repository\n";
protected static final String VERIFY = USAGE + "verify \n"
+ " The path or URL identifying the data file\n"
+ "Verifies the validity of the specified data file\n";
protected static final String CLEAR = USAGE + "clear Clears the entire repository\n"
+ "clear (|null)... Clears the specified context(s)\n";
protected static final String SPARQL = USAGE
+ "sparql Evaluates the SPARQL query on the currently open repository.\n"
+ "sparql Starts multi-line input for large SPARQL queries.\n"
+ "select|construct|ask|describe|prefix|base \n"
+ " Evaluates a SPARQL query on the currently open repository.\n";
protected static final String SERQL = USAGE
+ "serql Evaluates the SeRQL query on the currently open repository\n"
+ "serql Starts multi-line input for large SeRQL queries.\n" ;
protected static final String SET = USAGE
+ "set Shows all parameter values\n"
+ "set width= Set the width for query result tables\n"
+ "set log= Set the logging level (none, error, warning, info or debug)\n"
+ "set showPrefix= Toggles use of prefixed names in query results\n"
+ "set queryPrefix= Toggles automatic use of known namespace prefixes in queries\n";
protected static final String FEDERATE = USAGE
+ "federate [distinct=] [readonly=] []*\n"
+ " [distinct=] If true, uses a DISTINCT filter that suppresses duplicate results for identical quads\n"
+ " from different federation members. Default is false.\n"
+ " [readonly=] If true, sets the fedearated repository as read-only. If any member is read-only, then\n"
+ " this may only be set to true. Default is true. \n"
+ " The id to assign the federated repository.\n"
+ " The id's of at least 2 repositories to federate.\n"
+ " []* The id's of 0 or mare additional repositories to federate.\n\n"
+ "You will be prompted to enter a description for the federated repository as well.";
private final Map topics = new HashMap();
private final ConsoleIO consoleIO;
PrintHelp(ConsoleIO consoleIO) {
super();
this.consoleIO = consoleIO;
topics.put("clear", CLEAR);
topics.put("close", CLOSE);
topics.put("connect", CONNECT);
topics.put("create", CREATE);
topics.put("disconnect", DISCONNECT);
topics.put("drop", DROP);
topics.put("federate", FEDERATE);
topics.put("info", INFO);
topics.put("load", LOAD);
topics.put("open", OPEN);
topics.put("serql", SERQL);
topics.put("set", SET);
topics.put("show", SHOW);
topics.put("sparql", SPARQL);
topics.put("verify", VERIFY);
}
public void execute(String... parameters) {
if (parameters.length < 2) {
printCommandOverview();
}
else {
final String target = parameters[1].toLowerCase(Locale.ENGLISH);
if (topics.containsKey(target)) {
consoleIO.writeln(topics.get(target));
}
else {
consoleIO.writeln("No additional info available for command " + parameters[1]);
}
}
}
private void printCommandOverview() {
consoleIO.writeln("For more information on a specific command, try 'help '.");
consoleIO.writeln("List of all commands:");
consoleIO.writeln("help Displays this help message");
consoleIO.writeln("info Shows info about the console");
consoleIO.writeln("connect Connects to a (local or remote) set of repositories");
consoleIO.writeln("disconnect Disconnects from the current set of repositories");
consoleIO.writeln("create Creates a new repository");
consoleIO.writeln("federate Federate existing repositories.");
consoleIO.writeln("drop Drops a repository");
consoleIO.writeln("open Opens a repository to work on, takes a repository ID as argument");
consoleIO.writeln("close Closes the current repository");
consoleIO.writeln("show Displays an overview of various resources");
consoleIO.writeln("load Loads a data file into a repository, takes a file path or URL as argument");
consoleIO.writeln("verify Verifies the syntax of an RDF data file, takes a file path or URL as argument");
consoleIO.writeln("clear Removes data from a repository");
consoleIO.writeln("sparql Evaluate a SPARQL query");
consoleIO.writeln("serql Evaluate a SeRQL query");
consoleIO.writeln("set Allows various console parameters to be set");
consoleIO.writeln("exit, quit Exit the console");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy