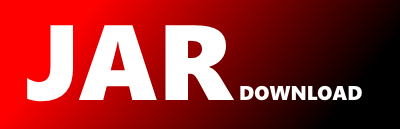
org.openrdf.rio.RDFFormat Maven / Gradle / Ivy
/*
* Licensed to Aduna under one or more contributor license agreements.
* See the NOTICE.txt file distributed with this work for additional
* information regarding copyright ownership.
*
* Aduna licenses this file to you under the terms of the Aduna BSD
* License (the "License"); you may not use this file except in compliance
* with the License. See the LICENSE.txt file distributed with this work
* for the full License.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package org.openrdf.rio;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import info.aduna.lang.FileFormat;
import org.openrdf.model.Statement;
import org.openrdf.model.IRI;
import org.openrdf.model.impl.SimpleValueFactory;
/**
* Represents the concept of an RDF data serialization format. RDF formats are
* identified by a {@link #getName() name} and can have one or more associated
* MIME types, zero or more associated file extensions and can specify a
* (default) character encoding. Some formats are able to encode context
* information while other are not; this is indicated by the value of
* {@link #supportsContexts}.
*
* @author Arjohn Kampman
*/
public class RDFFormat extends FileFormat {
/*-----------*
* Constants *
*-----------*/
/**
* Indicates that calls to {@link RDFHandler#handleNamespace(String, String)}
* may be serialised when serializing to this format.
*
* @since 2.7.0
*/
public static final boolean SUPPORTS_NAMESPACES = true;
/**
* Indicates that all calls to
* {@link RDFHandler#handleNamespace(String, String)} will be ignored when
* serializing to this format.
*
* @since 2.7.0
*/
public static final boolean NO_NAMESPACES = false;
/**
* Indicates that the {@link Statement#getContext()} URI may be serialized
* for this format.
*
* @since 2.7.0
*/
public static final boolean SUPPORTS_CONTEXTS = true;
/**
* Indicates that the {@link Statement#getContext()} URI will NOT be
* serialized for this format.
*
* @since 2.7.0
*/
public static final boolean NO_CONTEXTS = false;
/**
* The RDF/XML file
* format.
*
* Several file extensions are accepted for RDF/XML documents, including
* .rdf
, .rdfs
(for RDF Schema files),
* .owl
(for OWL ontologies), and .xml
. The media
* type is application/rdf+xml
, but application/xml
* is also accepted. The character encoding is UTF-8.
*
*
* @see RDF/XML Syntax
* Specification (Revised)
*/
public static final RDFFormat RDFXML = new RDFFormat("RDF/XML", Arrays.asList("application/rdf+xml",
"application/xml"), Charset.forName("UTF-8"), Arrays.asList("rdf", "rdfs", "owl", "xml"),
SimpleValueFactory.getInstance().createIRI("http://www.w3.org/ns/formats/RDF_XML"),
SUPPORTS_NAMESPACES, NO_CONTEXTS);
/**
* The N-Triples file format.
*
* The file extension .nt
is recommend for N-Triples documents.
* The media type is application/n-triples
and encoding is in
* UTF-8.
*
*
* @see N-Triples
*/
public static final RDFFormat NTRIPLES = new RDFFormat("N-Triples", Arrays.asList("application/n-triples",
"text/plain"), Charset.forName("UTF-8"), Arrays.asList("nt"),
SimpleValueFactory.getInstance().createIRI("http://www.w3.org/ns/formats/N-Triples"), NO_NAMESPACES,
NO_CONTEXTS);
/**
* The Turtle file format.
*
* The file extension .ttl
is recommend for Turtle documents.
* The media type is text/turtle
, but
* application/x-turtle
is also accepted. Character encoding is
* UTF-8.
*
*
* @see Turtle - Terse RDF Triple
* Language
*/
public static final RDFFormat TURTLE = new RDFFormat("Turtle", Arrays.asList("text/turtle",
"application/x-turtle"), Charset.forName("UTF-8"), Arrays.asList("ttl"),
SimpleValueFactory.getInstance().createIRI("http://www.w3.org/ns/formats/Turtle"),
SUPPORTS_NAMESPACES, NO_CONTEXTS);
/**
* The N3/Notation3 file
* format.
*
* The file extension .n3
is recommended for N3 documents. The
* media type is text/n3
, but text/rdf+n3
is also
* accepted. Character encoding is UTF-8.
*
*
* @see Notation3 (N3): A
* readable RDF syntax
*/
public static final RDFFormat N3 = new RDFFormat("N3", Arrays.asList("text/n3", "text/rdf+n3"),
Charset.forName("UTF-8"), Arrays.asList("n3"), SimpleValueFactory.getInstance().createIRI(
"http://www.w3.org/ns/formats/N3"), SUPPORTS_NAMESPACES, NO_CONTEXTS);
/**
* The TriX file format, an
* XML-based RDF serialization format that supports recording of named
* graphs.
*
* The file extension .xml
is recommended for TriX documents,
* .trix
is also accepted. The media type is
* application/trix
and the encoding is UTF-8.
*
*
* @see TriX: RDF Triples in XML
*/
public static final RDFFormat TRIX = new RDFFormat("TriX", Arrays.asList("application/trix"),
Charset.forName("UTF-8"), Arrays.asList("xml", "trix"), null, SUPPORTS_NAMESPACES, SUPPORTS_CONTEXTS);
/**
* The TriG file format, a
* Turtle-based RDF serialization format that supports recording of named
* graphs.
*
* The file extension .trig
is recommend for TriG documents. The
* media type is application/trig
and the encoding is UTF-8.
*
*
* @see The TriG Syntax
*/
public static final RDFFormat TRIG = new RDFFormat("TriG", Arrays.asList("application/trig",
"application/x-trig"), Charset.forName("UTF-8"), Arrays.asList("trig"),
SimpleValueFactory.getInstance().createIRI("http://www.w3.org/ns/formats/TriG"), SUPPORTS_NAMESPACES,
SUPPORTS_CONTEXTS);
/**
* A binary RDF format.
*
* The file extension .brf
is recommend for binary RDF
* documents. The media type is application/x-binary-rdf
.
*
*
* @see Binary
* RDF in Sesame
*/
public static final RDFFormat BINARY = new RDFFormat("BinaryRDF",
Arrays.asList("application/x-binary-rdf"), null, Arrays.asList("brf"), null, SUPPORTS_NAMESPACES,
SUPPORTS_CONTEXTS);
/**
* The N-Quads file format, an
* RDF serialization format that supports recording of named graphs.
*
* The file extension .nq
is recommended for N-Quads documents.
* The media type is application/n-quads
and the encoding is
* UTF-8.
*
*
* @see N-Quads: Extending N-Triples
* with Context
* @since 2.6.6
*/
public static final RDFFormat NQUADS = new RDFFormat("N-Quads", Arrays.asList("application/n-quads",
"text/x-nquads", "text/nquads"), Charset.forName("UTF-8"), Arrays.asList("nq"),
SimpleValueFactory.getInstance().createIRI("http://www.w3.org/ns/formats/N-Quads"), NO_NAMESPACES,
SUPPORTS_CONTEXTS);
/**
* The JSON-LD file format, an
* RDF serialization format that supports recording of named graphs.
*
* The file extension .jsonld
is recommended for JSON-LD
* documents. The media type is application/ld+json
and the
* encoding is UTF-8.
*
*
* @see JSON-LD 1.0
* @since 2.7.0
*/
public static final RDFFormat JSONLD = new RDFFormat("JSON-LD", Arrays.asList("application/ld+json"),
Charset.forName("UTF-8"), Arrays.asList("jsonld"), SimpleValueFactory.getInstance().createIRI(
"http://www.w3.org/ns/formats/JSON-LD"), SUPPORTS_NAMESPACES, SUPPORTS_CONTEXTS);
/**
* The RDF/JSON file format, an
* RDF serialization format that supports recording of named graphs.
*
* The file extension .rj
is recommended for RDF/JSON documents.
* The media type is application/rdf+json
and the encoding is
* UTF-8.
*
*
* @see RDF 1.1 JSON Alternate
* Serialization (RDF/JSON)
* @since 2.7.0
*/
public static final RDFFormat RDFJSON = new RDFFormat("RDF/JSON", Arrays.asList("application/rdf+json"),
Charset.forName("UTF-8"), Arrays.asList("rj"), SimpleValueFactory.getInstance().createIRI(
"http://www.w3.org/ns/formats/RDF_JSON"), NO_NAMESPACES, SUPPORTS_CONTEXTS);
/**
* The RDFa file format, an
* RDF serialization format.
*
* The file extension .xhtml
is recommended for RDFa documents.
* The preferred media type is application/xhtml+xml
and the
* encoding is UTF-8.
*
*
* @see XHTML+RDFa 1.1
* @since 2.7.0
*/
public static final RDFFormat RDFA = new RDFFormat("RDFa", Arrays.asList("application/xhtml+xml",
"application/html", "text/html"), Charset.forName("UTF-8"), Arrays.asList("xhtml", "html"),
SimpleValueFactory.getInstance().createIRI("http://www.w3.org/ns/formats/RDFa"), SUPPORTS_NAMESPACES,
NO_CONTEXTS);
/*----------------*
* Static methods *
*----------------*/
/**
* Processes the supplied collection of {@link RDFFormat}s and assigns
* quality values to each based on whether context must be supported and
* whether the format is preferred.
*
* @param rdfFormats
* The {@link RDFFormat}s to process.
* @param requireContext
* True to decrease the quality value for formats where
* {@link RDFFormat#supportsContexts()} returns false.
* @param preferredFormat
* The preferred RDFFormat. If it is not in the list then the quality
* of all formats will be processed as if they are not preferred.
* @return A list of strings containing the content types and an attached
* q-value specifying the quality for the format for each type.
*/
public static List getAcceptParams(Iterable rdfFormats, boolean requireContext,
RDFFormat preferredFormat)
{
List acceptParams = new ArrayList();
for (RDFFormat format : rdfFormats) {
// Determine a q-value that reflects the necessity of context
// support and the user specified preference
int qValue = 10;
if (requireContext && !format.supportsContexts()) {
// Prefer context-supporting formats over pure triple-formats
qValue -= 5;
}
if (preferredFormat != null && !preferredFormat.equals(format)) {
// Prefer specified format over other formats
qValue -= 2;
}
if (!format.supportsNamespaces()) {
// We like reusing namespace prefixes
qValue -= 1;
}
for (String mimeType : format.getMIMETypes()) {
String acceptParam = mimeType;
if (qValue < 10) {
acceptParam += ";q=0." + qValue;
}
acceptParams.add(acceptParam);
}
}
return acceptParams;
}
/*-----------*
* Variables *
*-----------*/
/**
* Flag indicating whether the RDFFormat can encode namespace information.
*/
private final boolean supportsNamespaces;
/**
* Flag indicating whether the RDFFormat can encode context information.
*/
private final boolean supportsContexts;
/**
* A standard URI published by the W3C or another standards body to uniquely
* denote this format.
*
* @see Unique URIs for File
* Formats
*/
private IRI standardURI;
/*--------------*
* Constructors *
*--------------*/
/**
* Creates a new RDFFormat object.
*
* @param name
* The name of the RDF file format, e.g. "RDF/XML".
* @param mimeType
* The MIME type of the RDF file format, e.g.
* application/rdf+xml for the RDF/XML file format.
* @param charset
* The default character encoding of the RDF file format. Specify
* null if not applicable.
* @param fileExtension
* The (default) file extension for the RDF file format, e.g.
* rdf for RDF/XML files.
* @param supportsNamespaces
* True if the RDFFormat supports the encoding of
* namespace/prefix information and false otherwise.
* @param supportsContexts
* True if the RDFFormat supports the encoding of
* contexts/named graphs and false otherwise.
*/
public RDFFormat(String name, String mimeType, Charset charset, String fileExtension,
boolean supportsNamespaces, boolean supportsContexts)
{
this(name, Arrays.asList(mimeType), charset, Arrays.asList(fileExtension), supportsNamespaces,
supportsContexts);
}
/**
* Creates a new RDFFormat object.
*
* @param name
* The name of the RDF file format, e.g. "RDF/XML".
* @param mimeType
* The MIME type of the RDF file format, e.g.
* application/rdf+xml for the RDF/XML file format.
* @param charset
* The default character encoding of the RDF file format. Specify
* null if not applicable.
* @param fileExtensions
* The RDF format's file extensions, e.g. rdf for RDF/XML
* files. The first item in the list is interpreted as the default
* file extension for the format.
* @param supportsNamespaces
* True if the RDFFormat supports the encoding of
* namespace/prefix information and false otherwise.
* @param supportsContexts
* True if the RDFFormat supports the encoding of
* contexts/named graphs and false otherwise.
*/
public RDFFormat(String name, String mimeType, Charset charset, Collection fileExtensions,
boolean supportsNamespaces, boolean supportsContexts)
{
this(name, Arrays.asList(mimeType), charset, fileExtensions, supportsNamespaces, supportsContexts);
}
/**
* Creates a new RDFFormat object.
*
* @param name
* The name of the RDF file format, e.g. "RDF/XML".
* @param mimeTypes
* The MIME types of the RDF file format, e.g.
* application/rdf+xml for the RDF/XML file format. The first
* item in the list is interpreted as the default MIME type for the
* format.
* @param charset
* The default character encoding of the RDF file format. Specify
* null if not applicable.
* @param fileExtensions
* The RDF format's file extensions, e.g. rdf for RDF/XML
* files. The first item in the list is interpreted as the default
* file extension for the format.
* @param supportsNamespaces
* True if the RDFFormat supports the encoding of
* namespace/prefix information and false otherwise.
* @param supportsContexts
* True if the RDFFormat supports the encoding of
* contexts/named graphs and false otherwise.
*/
public RDFFormat(String name, Collection mimeTypes, Charset charset,
Collection fileExtensions, boolean supportsNamespaces, boolean supportsContexts)
{
this(name, mimeTypes, charset, fileExtensions, null, supportsNamespaces, supportsContexts);
}
/**
* Creates a new RDFFormat object.
*
* @param name
* The name of the RDF file format, e.g. "RDF/XML".
* @param mimeTypes
* The MIME types of the RDF file format, e.g.
* application/rdf+xml for the RDF/XML file format. The first
* item in the list is interpreted as the default MIME type for the
* format.
* @param charset
* The default character encoding of the RDF file format. Specify
* null if not applicable.
* @param fileExtensions
* The RDF format's file extensions, e.g. rdf for RDF/XML
* files. The first item in the list is interpreted as the default
* file extension for the format.
* @param standardURI
* The standard URI that has been assigned to this format by a
* standards organisation or null if it does not currently have a
* standard URI.
* @param supportsNamespaces
* True if the RDFFormat supports the encoding of
* namespace/prefix information and false otherwise.
* @param supportsContexts
* True if the RDFFormat supports the encoding of
* contexts/named graphs and false otherwise.
* @since 2.8.0
*/
public RDFFormat(String name, Collection mimeTypes, Charset charset,
Collection fileExtensions, IRI standardURI, boolean supportsNamespaces,
boolean supportsContexts)
{
super(name, mimeTypes, charset, fileExtensions);
this.standardURI = standardURI;
this.supportsNamespaces = supportsNamespaces;
this.supportsContexts = supportsContexts;
}
/*---------*
* Methods *
*---------*/
/**
* Return true if the RDFFormat supports the encoding of
* namespace/prefix information.
*/
public boolean supportsNamespaces() {
return supportsNamespaces;
}
/**
* Return true if the RDFFormat supports the encoding of
* contexts/named graphs.
*/
public boolean supportsContexts() {
return supportsContexts;
}
/**
* @return True if a standard URI has been assigned to this format by a
* standards organisation.
* @since 2.8.0
*/
public boolean hasStandardURI() {
return standardURI != null;
}
/**
* @return The standard URI that has been assigned to this format by a
* standards organisation or null if it does not currently have a
* standard URI.
* @since 2.8.0
*/
public IRI getStandardURI() {
return standardURI;
}
}