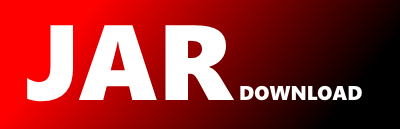
org.openrdf.model.Graph Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.model;
import java.util.Collection;
import org.openrdf.sesame.sail.StatementIterator;
/**
* Graph is an interface for fast manipulation of collections of RDF statements in code.
*
* @author Jeen Broekstra
* @version $Revision: 1.4.2.3 $
*/
public interface Graph {
/**
* Adds a single statement to the graph. If the supplied parameters contain
* any blank nodes that are shared with this graph, these will be joined.
*
* @param subject the statement subject
* @param predicate the statement predicate
* @param object the statement object
*/
public void add(Resource subject, URI predicate, Value object);
/**
* Adds a single statement to the graph. If the supplied
* statements contains any blank nodes that are shared with this graph,
* these will be joined.
*
* @param statement the statement to be added
*/
public void add(Statement statement);
/**
* Adds all statements in the iterator to the graph. If the supplied
* iterator contains any blank nodes that are shared with this graph,
* these will be joined.
*
* @param iterator an iterator containing statements
*/
public void add(StatementIterator iterator);
/**
* Adds all statements in the iterator to the graph.
*
* @param iterator an iterator containing statements
* @param joinBlankNodes specifies whether blank nodes in the supplied iterator should be
* joined with this graph. If false, new blank nodes will be created for each blank node
* in the supplied iterator.
*
*/
public void add(StatementIterator iterator, boolean joinBlankNodes);
/**
* Adds all statements in the Collection to the graph.
* If the supplied collection contains any blank nodes that are shared
* with this graph, these will be joined.
*
* @param statements a Collection containing Statement objects.
*/
public void add(Collection statements);
/**
* Adds all statements in the Collection to the graph.
*
* @param statements a Collection containing statements
* @param joinBlankNodes specifies whether blank nodes in the supplied Collection should be
* joined with this graph. If false, new blank nodes will be created for each blank node
* in the supplied Collection.
*
*/
public void add(Collection statements, boolean joinBlankNodes);
/**
* Adds all statements in the supplied graph to this graph. If the supplied graph
* contains any blank nodes that are shared with this graph, these will be joined.
*
* @param graph the supplied graph, to be added to this graph.
*/
public void add(Graph graph);
/**
* Adds all statements in the supplied graph to this graph.
*
* @param graph the supplied graph, to be added to this graph.
* @param joinBlankNodes specifies whether blank nodes in the supplied graph should be
* joined with this graph. If false, new blank nodes will be created for each blank node
* in the supplied graph.
*/
public void add(Graph graph, boolean joinBlankNodes);
/**
* Checks if a statement matching the (subject, predicate, object) pattern is
* present in the graph.
*
* @param subject the statement subject, or null for a wildcard.
* @param predicate the statement predicate, or null for a wildcard.
* @param object the statement object, or null for a wildcard.
* @return true if a matching statement is found, false otherwise.
*/
public boolean contains(Resource subject, URI predicate, Value object);
/**
* Checks if the supplied statement is present in the graph.
* @param statement the statement for which presence is checked.
* @return true if the statement is present in the graph, false otherwise.
*/
public boolean contains(Statement statement);
/**
* Retrieves all statements in the graph as an iterator
* @return a StatementIterator containing all statements in the graph.
*/
public StatementIterator getStatements();
/**
* Retrieves all statements matching the (subject, predicate, object) pattern
* in the graph as an iterator.
*
* @param subject the statement subject, or null for a wildcard
* @param predicate the statement predicate, or null for a wildcard
* @param object the statement object, or null for a wildcard
* @return a StatementIterator containing all matching statements in the graph.
*/
public StatementIterator getStatements(Resource subject, URI predicate, Value object);
/**
* Retrieves all statements matching the (subject, predicate, object) pattern
* in the graph as a Collection.
*
* @param subject the statement subject, or null for a wildcard.
* @param predicate the statement predicate, or null for a wildcard.
* @param object the statement object, or null for a wildcard.
* @return a Collection of matching statements.
*/
public Collection getStatementCollection(Resource subject, URI predicate, Value object);
/**
* Remove all statements matching the (subject, predicate, object) pattern from the graph.
*
* @param subject the statement subject, or null for a wildcard.
* @param predicate the statement predicate, or null for a wildcard.
* @param object the statement object, or null for a wildcard.
* @return the number of statements that were removed.
*/
public int remove(Resource subject, URI predicate, Value object);
/**
* Remove the supplied statement from the graph.
*
* @param statement the statement to be removed.
* @return the number of statements removed. If the statement was not
* present in the graph, 0 is returned.
*
*/
public int remove(Statement statement);
/**
* Remove all statements in the StatementIterator from the graph.
*
* @param iterator A StatementIterator containing all statements to be removed.
* @return the number of statements removed.
*/
public int remove(StatementIterator iterator);
/**
* Remove all statements in the supplied graph from this graph.
* @param graph
* @return the number of statements removed.
*/
public int remove(Graph graph);
/**
* Clears the graph. All statements are removed from the graph.
*
*/
public void clear();
/**
* Retrieves a ValueFactory for this graph.
*
* @return a ValueFactory.
*/
public ValueFactory getValueFactory();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy