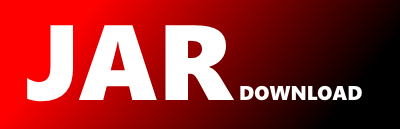
org.openrdf.model.impl.GraphImpl Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.model.impl;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import org.openrdf.model.BNode;
import org.openrdf.model.Graph;
import org.openrdf.model.Resource;
import org.openrdf.model.Statement;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import org.openrdf.model.ValueFactory;
import org.openrdf.sesame.repository.local.LocalRepository;
import org.openrdf.sesame.sail.RdfRepository;
import org.openrdf.sesame.sail.SailInitializationException;
import org.openrdf.sesame.sail.SailUpdateException;
import org.openrdf.sesame.sail.StatementIterator;
/**
* GraphImpl - an implementation of the graph interface that uses a local repository
* as storage backend.
*
* GraphImpl is not thread-safe.
*
* @author Jeen Broekstra
* @author Arjohn Kampman
*/
public class GraphImpl implements Graph {
/*----------+
| Constants |
+----------*/
/**
* Constant defining default behavior of add methods: by default identical
* blank nodes will be 'joined'.
*/
private static final boolean JOIN_BLANKNODES_DEFAULT = true;
/*----------+
| Variables |
+----------*/
private RdfRepository _sail;
/*-------------+
| Constructors |
+-------------*/
/**
* Creates a new GraphImpl. The GraphImpl uses an in-memory RdfRepository as
* storage backend.
**/
public GraphImpl() {
this(new org.openrdf.sesame.sailimpl.memory.RdfRepository());
try {
_sail.initialize(Collections.EMPTY_MAP);
}
catch (SailInitializationException e) {
// This can only happen if there is an error in the supplied config
// parameters. Since we do not supply any, it never should occur.
throw new RuntimeException(e);
}
}
/**
* Creates a new GraphImpl that uses the supplied LocalRepository as storage
* backend.
*
* @param repository The LocalRepository to be used as backend.
**/
public GraphImpl(LocalRepository repository) {
this((RdfRepository)repository.getSail());
}
/**
* Creates a new GraphImpl that uses the supplied Sail as storage backend. The constructor
* assumes the supplied Sail is initialized.
*
* @deprecated low-level SAIL api should not be exposed in access APIs;
* use constructor with LocalRepository object instead.
*
* @param sail the Sail object to be used as storage backend
*/
public GraphImpl(RdfRepository sail) {
_sail = sail;
}
/*--------+
| Methods |
+--------*/
// implements Graph.add(Resource, URI, Value)
public void add(Resource subject, URI predicate, Value object) {
_sail.startTransaction();
try {
_sail.addStatement(subject, predicate, object);
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
}
// implements Graph.add(Statement)
public void add(Statement st) {
_sail.startTransaction();
try {
_sail.addStatement(st.getSubject(), st.getPredicate(), st.getObject());
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
}
// implements Graph.add(StatementIterator)
public void add(StatementIterator iterator) {
add(iterator, JOIN_BLANKNODES_DEFAULT);
}
public void add(StatementIterator iterator, boolean joinBlankNodes) {
Map bNodesMap = null;
ValueFactory factory = null;
if (!joinBlankNodes) {
// we need to create new blank nodes to avoid accidental
// merging of blank nodes in the repository.
bNodesMap = new HashMap();
factory = _sail.getValueFactory();
}
_sail.startTransaction();
try {
while (iterator.hasNext()) {
Statement st = iterator.next();
Resource subject = st.getSubject();
URI predicate = st.getPredicate();
Value object = st.getObject();
if (!joinBlankNodes) {
if (subject instanceof BNode) {
String bNodeId = ((BNode)subject).getID();
if (bNodesMap.containsKey(bNodeId)) {
// bnode was mapped before, reuse
subject = (Resource)bNodesMap.get(bNodeId);
}
else {
// create a new blank node and add it to the mapping.
subject = factory.createBNode();
bNodesMap.put(bNodeId, subject);
}
}
if (object instanceof BNode) {
String bNodeId = ((BNode)object).getID();
if (bNodesMap.containsKey(bNodeId)) {
// bnode was mapped before, reuse
object = (Resource)bNodesMap.get(bNodeId);
}
else {
// create a new blank node and add it to the mapping.
object = factory.createBNode();
bNodesMap.put(bNodeId, object);
}
}
}
_sail.addStatement(subject, predicate, object);
}
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
}
// implements Graph.add(Collection)
public void add(Collection statements) {
add(statements, JOIN_BLANKNODES_DEFAULT);
}
public void add(Collection statements, boolean joinBlankNodes) {
Map bNodesMap = null;
ValueFactory factory = null;
if (!joinBlankNodes) {
// we need to create new blank nodes to avoid accidental
// merging of blank nodes in the repository.
bNodesMap = new HashMap();
factory = _sail.getValueFactory();
}
Iterator iter = statements.iterator();
_sail.startTransaction();
try {
while (iter.hasNext()) {
Object o = iter.next();
if (o instanceof Statement) {
Statement st = (Statement)o;
Resource subject = st.getSubject();
URI predicate = st.getPredicate();
Value object = st.getObject();
if (!joinBlankNodes) {
if (subject instanceof BNode) {
String bNodeId = ((BNode)subject).getID();
if (bNodesMap.containsKey(bNodeId)) {
// bnode was mapped before, reuse
subject = (Resource)bNodesMap.get(bNodeId);
}
else {
// create a new blank node and add it to the mapping.
subject = factory.createBNode();
bNodesMap.put(bNodeId, subject);
}
}
if (object instanceof BNode) {
String bNodeId = ((BNode)object).getID();
if (bNodesMap.containsKey(bNodeId)) {
// bnode was mapped before, reuse
object = (Resource)bNodesMap.get(bNodeId);
}
else {
// create a new blank node and add it to the mapping.
object = factory.createBNode();
bNodesMap.put(bNodeId, object);
}
}
}
_sail.addStatement(subject, predicate, object);
}
}
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
}
// implements Graph.add(Graph)
public void add(Graph graph) {
add(graph, JOIN_BLANKNODES_DEFAULT);
}
// implements Graph.add(Graph, boolean)
public void add(Graph graph, boolean joinBlankNodes) {
Map bNodesMap = null;
if (!joinBlankNodes) {
// we need to create new blank nodes to avoid accidental
// merging of blank nodes in the repository.
bNodesMap = new HashMap();
}
StatementIterator iter = graph.getStatements();
try {
ValueFactory factory = _sail.getValueFactory();
_sail.startTransaction();
while (iter.hasNext()) {
Statement st = iter.next();
Resource subject = st.getSubject();
URI predicate = st.getPredicate();
Value object = st.getObject();
if (!joinBlankNodes) {
if (subject instanceof BNode) {
String bNodeId = ((BNode)subject).getID();
if (bNodesMap.containsKey(bNodeId)) {
// bnode was mapped before, reuse
subject = (Resource)bNodesMap.get(bNodeId);
}
else {
// create a new blank node and add it to the mapping.
subject = factory.createBNode();
bNodesMap.put(bNodeId, subject);
}
}
if (object instanceof BNode) {
String bNodeId = ((BNode)object).getID();
if (bNodesMap.containsKey(bNodeId)) {
// bnode was mapped before, reuse
object = (Resource)bNodesMap.get(bNodeId);
}
else {
// create a new blank node and add it to the mapping.
object = factory.createBNode();
bNodesMap.put(bNodeId, object);
}
}
}
_sail.addStatement(subject, predicate, object);
}
}
catch (SailUpdateException e) {
// FIXME is this a correct conversion?
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
iter.close();
}
}
// implements Graph.contains(Resource, URI, Value)
public boolean contains(Resource subject, URI predicate, Value object) {
return _sail.hasStatement(subject, predicate, object);
}
// implements Graph.contains(Statement)
public boolean contains(Statement st) {
return contains(st.getSubject(), st.getPredicate(), st.getObject());
}
// implements Graph.getStatements()
public StatementIterator getStatements() {
return _sail.getStatements(null, null, null);
}
// implements Graph.getStatements(Resource, URI, Value)
public StatementIterator getStatements(Resource subject, URI predicate, Value object) {
return _sail.getStatements(subject, predicate, object);
}
// implements Graph.remove(Resource, URI, Value)
public int remove(Resource subject, URI predicate, Value object) {
int result = 0;
_sail.startTransaction();
try {
result = _sail.removeStatements(subject, predicate, object);
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
return result;
}
// implements Graph.remove(Statement)
public int remove(Statement st) {
return remove(st.getSubject(), st.getPredicate(), st.getObject());
}
// implements Graph.remove(StatementIterator)
public int remove(StatementIterator iterator) {
int result = 0;
_sail.startTransaction();
try {
while (iterator.hasNext()) {
Statement st = iterator.next();
result += _sail.removeStatements(st.getSubject(), st.getPredicate(), st.getObject());
}
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
return result;
}
// implements Graph.clear()
public void clear() {
_sail.startTransaction();
try {
_sail.clearRepository();
}
catch (SailUpdateException e) {
// only occurs if transaction is not started.
throw new RuntimeException(e);
}
finally {
_sail.commitTransaction();
}
}
// implements Graph.getStatementCollection(Resource, URI, Value)
public Collection getStatementCollection(Resource subject, URI predicate, Value object) {
Collection collection = new ArrayList();
StatementIterator iterator = getStatements(subject, predicate, object);
while (iterator.hasNext()) {
collection.add(iterator.next());
}
iterator.close();
return collection;
}
// implements Graph.remove(Graph)
public int remove(Graph graph) {
return remove(graph.getStatements());
}
// implements Graph.getValueFactory()
public ValueFactory getValueFactory() {
return _sail.getValueFactory();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy