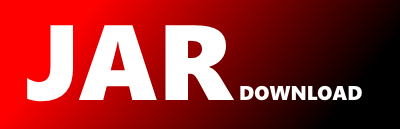
org.openrdf.model.impl.StatementImpl Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.model.impl;
import java.io.Serializable;
import org.openrdf.model.Resource;
import org.openrdf.model.Statement;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
/**
* An implementation of the Statement interface.
**/
public class StatementImpl implements Statement, Serializable {
/*---------------------------------------+
| Variables |
+---------------------------------------*/
/**
* subject
**/
private Resource _subject;
/**
* predicate
**/
private URI _predicate;
/**
* object
**/
private Value _object;
/*---------------------------------------+
| Constructors |
+---------------------------------------*/
/**
* Creates a new Statement with the supplied subject, predicate and object.
*
* @param subject The statement's subject.
* @param predicate The statement's predicate.
* @param object The statement's object.
**/
public StatementImpl(Resource subject, URI predicate, Value object) {
_subject = subject;
_predicate = predicate;
_object = object;
}
/*---------------------------------------+
| Methods |
+---------------------------------------*/
// inherit comments
public Resource getSubject() {
return _subject;
}
// inherit comments
public URI getPredicate() {
return _predicate;
}
// inherit comments
public Value getObject() {
return _object;
}
// implements Statement.equals(Object)
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other instanceof Statement) {
Statement otherSt = (Statement)other;
// The object is potentially the cheapest to check, as types
// of these references might be different.
// In general the number of different predicates in sets of
// statements is the smallest, so predicate equality is checked
// last.
return
_object.equals(otherSt.getObject()) &&
_subject.equals(otherSt.getSubject()) &&
_predicate.equals(otherSt.getPredicate());
}
return false;
}
// implements Statement.hashCode()
public int hashCode() {
return
(_subject.hashCode() & 0xFF0000) |
(_predicate.hashCode() & 0x00FF00) |
(_object.hashCode() & 0x0000FF);
}
/**
* Compares this Statement with the supplied object.
*
* @throws ClassCastException if o is not of type Statement
* @see java.lang.Comparable
**/
public int compareTo(Object o) {
if (this == o) {
return 0;
}
Statement other = (Statement)o;
int result = 0;
result = _object.compareTo(other.getObject());
if (result == 0) {
// objects are equal
result = _subject.compareTo(other.getSubject());
}
if (result == 0) {
// subjects and objects are equal
result = _predicate.compareTo(other.getPredicate());
}
return result;
}
/**
* Gives a String-representation of this Statement that can be used for
* debugging.
**/
public String toString() {
StringBuffer result = new StringBuffer(256);
result.append("(");
result.append(_subject.toString());
result.append(", ");
result.append(_predicate.toString());
result.append(", ");
result.append(_object.toString());
result.append(")");
return result.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy