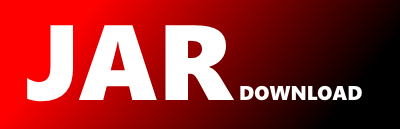
org.openrdf.model.impl.ValueFactoryImpl Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.model.impl;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import org.openrdf.model.BNode;
import org.openrdf.model.Literal;
import org.openrdf.model.Resource;
import org.openrdf.model.Statement;
import org.openrdf.model.URI;
import org.openrdf.model.Value;
import org.openrdf.model.ValueFactory;
/**
* Default implementation of the ValueFactory interface that uses the RDF model
* classes from this package.
*/
public class ValueFactoryImpl implements ValueFactory {
/*-------------------+
| Variables |
+-------------------*/
private int _lastBNodeId;
private final static String BNODE_PREFIX = "node";
/*-------------------+
| Constructors |
+-------------------*/
/**
* Creates a new ValueFactoryImpl.
*/
public ValueFactoryImpl() {
_lastBNodeId = 0;
}
/**
* Creates a new ValueFactoryImpl that uses the specified document URI to
* generate a bNode prefix.
*
* @param documentURI A document URI
* @see #setDocumentURI
*/
public ValueFactoryImpl(String documentURI) {
this();
setDocumentURI(documentURI);
}
/*-------------------+
| Methods |
+-------------------*/
// Implements ValueFactory.createURI(String)
public URI createURI(String uri) {
return new URIImpl(uri);
}
public URI createURI(String namespace, String localName) {
return new URIImpl(namespace, localName);
}
// Implements ValueFactory.createBNode()
public BNode createBNode() {
return new BNodeImpl(BNODE_PREFIX + _lastBNodeId++);
}
public BNode createBNode(String nodeId) {
return new BNodeImpl(nodeId);
}
// Implements ValueFactory.createLiteral(String)
public Literal createLiteral(String value) {
return new LiteralImpl(value);
}
// Implements ValueFactory.createLiteral(String, String)
public Literal createLiteral(String value, String language) {
return new LiteralImpl(value, language);
}
// Implements ValueFactory.createLiteral(String, URI)
public Literal createLiteral(String value, URI datatype) {
return new LiteralImpl(value, datatype);
}
// Implements ValueFactory.createLiteral(String, String, String)
public Literal createLiteral(String value, String language, String datatype) {
if (datatype != null) {
URI dtURI = createURI(datatype);
return createLiteral(value, dtURI);
}
else if (language != null) {
return createLiteral(value, language);
}
else {
return createLiteral(value);
}
}
// Implements ValueFactory.createStatement(Resource, URI, Value)
public Statement createStatement(Resource subject, URI predicate, Value object) {
return new StatementImpl(subject, predicate, object);
}
/**
* Sets the URI of the document that is currently being parsed. This URI is
* used to generate a 'unique' bNode prefix based on an MD5 (see RFC 1321)
* digest of the URI. The result of this digest is practically guaranteed to
* be unique.
*/
public void setDocumentURI(String uri) {
MessageDigest messageDigest = null;
try {
messageDigest = MessageDigest.getInstance("MD5");
}
catch (NoSuchAlgorithmException e) {
throw new RuntimeException("Unable to get MD5 instance: "
+ e.getMessage());
}
messageDigest.update(uri.getBytes());
// Create an MD5 digest code (16 bytes)
byte[] md5code = messageDigest.digest();
StringBuffer sb = new StringBuffer(32);
for (int i = 0; i < md5code.length; i++) {
int b = md5code[i];
if (b < 0) {
b += 256;
}
String hexString = Integer.toHexString(b).toUpperCase();
// make sure 2 characters are used for 'hexString'
if (hexString.length() == 1) {
sb.append('0');
}
sb.append(hexString);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy