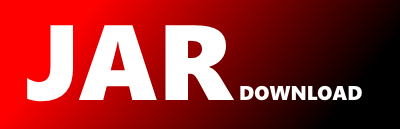
org.openrdf.vocabulary.XmlSchema Maven / Gradle / Ivy
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.vocabulary;
/**
* Defines constants for the standard XML Schema datatypes.
**/
public class XmlSchema {
/*
* The XML Schema namespace
*/
/** The XML Schema namespace (http://www.w3.org/2001/XMLSchema#). **/
public static final String NAMESPACE = "http://www.w3.org/2001/XMLSchema#";
/*
* Primitive datatypes
*/
/** http://www.w3.org/2001/XMLSchema#duration **/
public static final String DURATION = NAMESPACE + "duration";
/** http://www.w3.org/2001/XMLSchema#dateTime **/
public static final String DATETIME = NAMESPACE + "dateTime";
/** http://www.w3.org/2001/XMLSchema#time **/
public static final String TIME = NAMESPACE + "time";
/** http://www.w3.org/2001/XMLSchema#date **/
public static final String DATE = NAMESPACE + "date";
/** http://www.w3.org/2001/XMLSchema#gYearMonth **/
public static final String GYEARMONTH = NAMESPACE + "gYearMonth";
/** http://www.w3.org/2001/XMLSchema#gYear **/
public static final String GYEAR = NAMESPACE + "gYear";
/** http://www.w3.org/2001/XMLSchema#gMonthDay **/
public static final String GMONTHDAY = NAMESPACE + "gMonthDay";
/** http://www.w3.org/2001/XMLSchema#gDay **/
public static final String GDAY = NAMESPACE + "gDay";
/** http://www.w3.org/2001/XMLSchema#gMonth **/
public static final String GMONTH = NAMESPACE + "gMonth";
/** http://www.w3.org/2001/XMLSchema#string **/
public static final String STRING = NAMESPACE + "string";
/** http://www.w3.org/2001/XMLSchema#boolean **/
public static final String BOOLEAN = NAMESPACE + "boolean";
/** http://www.w3.org/2001/XMLSchema#base64Binary **/
public static final String BASE64BINARY = NAMESPACE + "base64Binary";
/** http://www.w3.org/2001/XMLSchema#hexBinary **/
public static final String HEXBINARY = NAMESPACE + "hexBinary";
/** http://www.w3.org/2001/XMLSchema#float **/
public static final String FLOAT = NAMESPACE + "float";
/** http://www.w3.org/2001/XMLSchema#decimal **/
public static final String DECIMAL = NAMESPACE + "decimal";
/** http://www.w3.org/2001/XMLSchema#double **/
public static final String DOUBLE = NAMESPACE + "double";
/** http://www.w3.org/2001/XMLSchema#anyURI **/
public static final String ANYURI = NAMESPACE + "anyURI";
/** http://www.w3.org/2001/XMLSchema#QName **/
public static final String QNAME = NAMESPACE + "QName";
/** http://www.w3.org/2001/XMLSchema#NOTATION **/
public static final String NOTATION = NAMESPACE + "NOTATION";
/*
* Derived datatypes
*/
/** http://www.w3.org/2001/XMLSchema#normalizedString **/
public static final String NORMALIZEDSTRING = NAMESPACE + "normalizedString";
/** http://www.w3.org/2001/XMLSchema#token **/
public static final String TOKEN = NAMESPACE + "token";
/** http://www.w3.org/2001/XMLSchema#language **/
public static final String LANGUAGE = NAMESPACE + "language";
/** http://www.w3.org/2001/XMLSchema#NMTOKEN **/
public static final String NMTOKEN = NAMESPACE + "NMTOKEN";
/** http://www.w3.org/2001/XMLSchema#NMTOKENS **/
public static final String NMTOKENS = NAMESPACE + "NMTOKENS";
/** http://www.w3.org/2001/XMLSchema#Name **/
public static final String NAME = NAMESPACE + "Name";
/** http://www.w3.org/2001/XMLSchema#NCName **/
public static final String NCNAME = NAMESPACE + "NCName";
/** http://www.w3.org/2001/XMLSchema#ID **/
public static final String ID = NAMESPACE + "ID";
/** http://www.w3.org/2001/XMLSchema#IDREF **/
public static final String IDREF = NAMESPACE + "IDREF";
/** http://www.w3.org/2001/XMLSchema#IDREFS **/
public static final String IDREFS = NAMESPACE + "IDREFS";
/** http://www.w3.org/2001/XMLSchema#ENTITY **/
public static final String ENTITY = NAMESPACE + "ENTITY";
/** http://www.w3.org/2001/XMLSchema#ENTITIES **/
public static final String ENTITIES = NAMESPACE + "ENTITIES";
/** http://www.w3.org/2001/XMLSchema#integer **/
public static final String INTEGER = NAMESPACE + "integer";
/** http://www.w3.org/2001/XMLSchema#long **/
public static final String LONG = NAMESPACE + "long";
/** http://www.w3.org/2001/XMLSchema#int **/
public static final String INT = NAMESPACE + "int";
/** http://www.w3.org/2001/XMLSchema#short **/
public static final String SHORT = NAMESPACE + "short";
/** http://www.w3.org/2001/XMLSchema#byte **/
public static final String BYTE = NAMESPACE + "byte";
/** http://www.w3.org/2001/XMLSchema#nonPositiveInteger **/
public static final String NON_POSITIVE_INTEGER = NAMESPACE + "nonPositiveInteger";
/** http://www.w3.org/2001/XMLSchema#negativeInteger **/
public static final String NEGATIVE_INTEGER = NAMESPACE + "negativeInteger";
/** http://www.w3.org/2001/XMLSchema#nonNegativeInteger **/
public static final String NON_NEGATIVE_INTEGER = NAMESPACE + "nonNegativeInteger";
/** http://www.w3.org/2001/XMLSchema#positiveInteger **/
public static final String POSITIVE_INTEGER = NAMESPACE + "positiveInteger";
/** http://www.w3.org/2001/XMLSchema#unsignedLong **/
public static final String UNSIGNED_LONG = NAMESPACE + "unsignedLong";
/** http://www.w3.org/2001/XMLSchema#unsignedInt **/
public static final String UNSIGNED_INT = NAMESPACE + "unsignedInt";
/** http://www.w3.org/2001/XMLSchema#unsignedShort **/
public static final String UNSIGNED_SHORT = NAMESPACE + "unsignedShort";
/** http://www.w3.org/2001/XMLSchema#unsignedByte **/
public static final String UNSIGNED_BYTE = NAMESPACE + "unsignedByte";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy