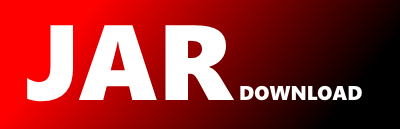
org.openrdf.util.StringUtil Maven / Gradle / Ivy
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.util;
public class StringUtil {
/**
* Substitute String "old" by String "new" in String "text" everywhere.
*
* This is static util function that I could not place anywhere more
* appropriate. The name of this function is from the good-old awk time.
*
* @param olds The String to be substituted.
* @param news The String is the new content.
* @param text The String in which the substitution is done.
* @return The result String containing the substitutions; if no
* substitutions were made, the result is 'text'.
*/
public static String gsub(String olds, String news, String text) {
if (olds == null || olds.length() == 0) {
// Nothing to substitute.
return text;
}
// Search for any occurences of 'olds'.
int oldsIndex = text.indexOf(olds);
if (oldsIndex == -1) {
// Nothing to substitute.
return text;
}
// We're going to do some substitutions.
StringBuffer buf = new StringBuffer(text.length());
int prevIndex = 0;
while (oldsIndex >= 0) {
// First, add the text between the previous and the current
// occurence.
buf.append(text.substring(prevIndex, oldsIndex));
// Then add the substition pattern
buf.append(news);
// Remember the index for the next loop.
prevIndex = oldsIndex + olds.length();
// Search for the next occurence.
oldsIndex = text.indexOf(olds, prevIndex);
}
// Add the part after the last occurence.
buf.append(text.substring(prevIndex));
return buf.toString();
}
/**
* Searches for the first occurrence of a word, which must be surrounded
* by whitespace characters, in a text.
**/
public static int indexOfWord(String text, String word) {
return indexOfWord(text, word, 0);
}
/**
* Searches for the first occurrence of a word, which must be surrounded
* by whitespace characters, that appears in a text after 'fromIndex'.
**/
public static int indexOfWord(String text, String word, int fromIndex) {
int wordIdx = fromIndex - 1;
while (true) {
wordIdx = text.indexOf(word, wordIdx + 1);
if (wordIdx == -1) {
// word not found
break;
}
// Verify that the character before the word is whitespace
int beforeIdx = wordIdx - 1;
if (beforeIdx >= 0 &&
!Character.isWhitespace(text.charAt(beforeIdx)))
{
// character was not whitespace
continue;
}
// Verify that the character after the word is whitespace
int afterIdx = wordIdx + word.length();
if (afterIdx < text.length() &&
!Character.isWhitespace(text.charAt(afterIdx)))
{
// character was not whitespace
continue;
}
// All checks passed,
break;
}
return wordIdx;
}
/**
* Searches for the last occurrence of a word, which must be surrounded
* by whitespace characters, in a text.
**/
public static int lastIndexOfWord(String text, String word) {
return lastIndexOfWord(text, word, text.length());
}
/**
* Searches for the last occurrence of a word, which must be surrounded
* by whitespace characters, in a text. The algorithm searches the text
* backwards, starting from 'fromIndex'.
**/
public static int lastIndexOfWord(String text, String word, int fromIndex) {
int wordIdx = fromIndex + 1;
while (true) {
wordIdx = text.lastIndexOf(word, wordIdx - 1);
if (wordIdx == -1) {
// word not found
break;
}
// Verify that the character before the word is whitespace
int beforeIdx = wordIdx - 1;
if (beforeIdx >= 0 &&
!Character.isWhitespace(text.charAt(beforeIdx)))
{
// character was not whitespace
continue;
}
// Verify that the character after the word is whitespace
int afterIdx = wordIdx + word.length();
if (afterIdx < text.length() &&
!Character.isWhitespace(text.charAt(afterIdx)))
{
// character was not whitespace
continue;
}
// All checks passed,
break;
}
return wordIdx;
}
} //end class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy