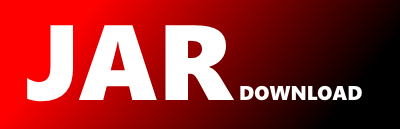
org.openrdf.util.http.CookieManager Maven / Gradle / Ivy
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.util.http;
import java.net.URLConnection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* An HTTP cookie manager.
*/
public class CookieManager {
/*----------------------+
| Variables |
+----------------------*/
/**
* A Map of cookies per host. The key is a host name (String),
* the value is a Map mapping cookie names to Cookie objects.
**/
private Map _cookiesPerHost;
/*----------------------+
| Constructors |
+----------------------*/
/**
* Creates a new CookieManager.
*/
public CookieManager() {
_cookiesPerHost = new HashMap();
}
/*----------------------+
| Methods |
+----------------------*/
/**
* Extracts any cookies from the supplied URLConnection and stores
* them internally.
*
* @param urlConnection A URLConnection.
**/
public void extractCookies(URLConnection urlConnection) {
Map headerFields = urlConnection.getHeaderFields();
List cookieHeaders = (List)headerFields.get("Set-Cookie");
if (!cookieHeaders.isEmpty()) {
String hostName = urlConnection.getURL().getHost();
Map cookieMap = (Map)_cookiesPerHost.get(hostName);
if (cookieMap == null) {
cookieMap = new HashMap();
_cookiesPerHost.put(hostName, cookieMap);
}
for (int i = 0; i < cookieHeaders.size(); i++) {
String cookieHeader = (String)cookieHeaders.get(i);
Cookie cookie = _parseCookieHeader(cookieHeader);
cookieMap.put(cookie.getName(), cookie);
//System.err.println("Got cookie: " + cookie.getName() + " = " + cookie.getValue());
}
}
}
/**
* Sets any cookies that are relevant for the supplied
* URLConnection.
*
* @param urlConnection A URLConnection.
**/
public void setCookies(URLConnection urlConnection) {
String hostName = urlConnection.getURL().getHost();
Map cookieMap = (Map)_cookiesPerHost.get(hostName);
if (cookieMap != null && !cookieMap.isEmpty()) {
StringBuffer headerValue = new StringBuffer(64);
Iterator cookieIter = cookieMap.values().iterator();
while (cookieIter.hasNext()) {
Cookie cookie = (Cookie)cookieIter.next();
headerValue.append(cookie.getName());
headerValue.append('=');
headerValue.append(cookie.getValue());
if (cookieIter.hasNext()) {
headerValue.append("; ");
}
}
urlConnection.setRequestProperty(
"Cookie", headerValue.toString());
//System.err.println("Cookie set: Cookie = " + headerValue.toString());
}
}
/**
* Removes all cookie information from this cookie manager.
**/
public void clear() {
_cookiesPerHost.clear();
}
/**
* Removes all cookie information for the specified host from this
* cookie manager.
*
* @param hostName A server's host name.
**/
public void clear(String hostName) {
_cookiesPerHost.remove(hostName);
}
/*----------------------+
| Private methods |
+----------------------*/
private Cookie _parseCookieHeader(String headerValue) {
// Example value: JSESSIONID=C2CA7C6C2DD15CE3551FEB34F7107815;Path=/sesame
int equalsIdx = headerValue.indexOf('=');
if (equalsIdx == -1) {
throw new IllegalArgumentException(
"Unable to parse header value: " + headerValue);
}
int semiColonIdx = headerValue.indexOf(';', equalsIdx);
if (semiColonIdx != -1) {
// Truncate path info
headerValue = headerValue.substring(0, semiColonIdx);
}
String name = headerValue.substring(0, equalsIdx);
String value = headerValue.substring(equalsIdx + 1);
return new Cookie(name, value);
}
/*----------------------+
| Inner class Cookie |
+----------------------*/
static class Cookie {
/** The name of the cookie. **/
private String _name;
/** The value of the cookie. **/
private String _value;
/**
* Creates a new Cookie.
*
* @param name The cookie name.
* @param value The cookie value.
*/
public Cookie(String name, String value) {
_name = name;
_value = value;
}
/**
* Gets the name of the cookie.
*
* @return The name of the cookie.
*/
public String getName() {
return _name;
}
/**
* Gets the value of the cookie.
*
* @return The value of the cookie.
*/
public String getValue() {
return _value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy