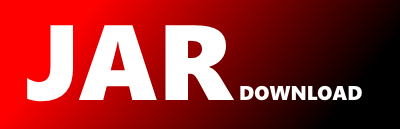
org.openrdf.util.http.HttpServerUtil Maven / Gradle / Ivy
The newest version!
/* Sesame - Storage and Querying architecture for RDF and RDF Schema
* Copyright (C) 2001-2006 Aduna
*
* Contact:
* Aduna
* Prinses Julianaplein 14 b
* 3817 CS Amersfoort
* The Netherlands
* tel. +33 (0)33 465 99 87
* fax. +33 (0)33 465 99 87
*
* http://aduna-software.com/
* http://www.openrdf.org/
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.openrdf.util.http;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.zip.GZIPOutputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.FileUploadException;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
/**
* Utility methods for HTTP servers/servlets.
**/
public class HttpServerUtil {
private static File _tmpDir = null;
private static DiskFileItemFactory _fileItemFactory = null;
private static ServletFileUpload _fileUpload = null;
private static ServletFileUpload _getServletFileUpload() {
if (_fileUpload == null) {
_fileItemFactory = new DiskFileItemFactory();
if (_tmpDir != null) {
// Try setting the tmp directory for _fileUpload
_fileItemFactory.setRepository(_tmpDir);
}
_fileUpload = new ServletFileUpload(_fileItemFactory);
}
return _fileUpload;
}
public static void setTmpDir(File tmpDir) {
_tmpDir = tmpDir;
if (_fileItemFactory != null) {
_fileItemFactory.setRepository(tmpDir);
}
}
/**
* Checks whether the supplied request is a multipart/form-data
* POST request.
**/
public static boolean isMultipartFormRequest(HttpServletRequest request) {
return ServletFileUpload.isMultipartContent(request);
}
/**
* Gets the trimmed value of a request parameter as a String. If the
* specified parameter does not exist or if it has a white-space-only value,
* null will be returned.
*
* @param request The request object to get the parameter from.
* @param paramName The name of the parameter.
* @return The trimmed value, or null if the specified parameter
* does not have a value.
**/
public static String getParameter(HttpServletRequest request, String paramName) {
return getParameter(request, paramName, null);
}
/**
* Gets the trimmed value of a request parameter as a String. If the
* specified parameter does not exist or if it has a white-space-only value,
* the supplied defaultValue will be returned.
*
* @param request The request object to get the parameter from.
* @param paramName The name of the parameter.
* @param defaultValue The value that should be returned when the specified
* parameter did not have a value.
* @return The trimmed value, or defaultValue if the specified
* parameter does not have a value.
**/
public static String getParameter(HttpServletRequest request, String paramName, String defaultValue) {
String result = request.getParameter(paramName);
if (result == null) {
result = defaultValue;
}
else {
result = result.trim();
if (result.length() == 0) {
result = defaultValue;
}
}
return result;
}
/**
* Parses a multipart/form-data POST request and returns its
* parameters as set of FileItems, mapped using their field name.
*
* @param request The request.
* @return a Map of FileItem objects, mapped using their field name (Strings).
* @exception IOException If the request could not be read/parsed.
**/
public static Map parseMultipartFormRequest(HttpServletRequest request)
throws IOException
{
try {
List fileItems = _getServletFileUpload().parseRequest(request);
Map resultMap = new HashMap(fileItems.size() * 2);
Iterator iter = fileItems.iterator();
while (iter.hasNext()) {
FileItem fileItem = (FileItem)iter.next();
resultMap.put(fileItem.getFieldName(), fileItem);
}
return resultMap;
}
catch (FileUploadException e) {
IOException ioe = new IOException();
ioe.initCause(e);
throw ioe;
}
}
/**
* Gets the trimmed value of a request parameter from a Map of FileItem
* objects, as returned by parseMultipartFormRequest(). If the
* specified parameter does not exist or if it has a white-space-only value,
* null will be returned. The values are assumed to be using the
* UTF-8 encoding.
*
* @param fileItemMap A Map of FileItem objects, mapped using their field
* name (Strings).
* @param paramName The name of the parameter.
* @return The trimmed value, or null if the specified parameter
* does not have a value.
**/
public static String getParameter(Map fileItemMap, String paramName) {
return getParameter(fileItemMap, paramName, null);
}
/**
* Gets the trimmed value of a request parameter from a Map of FileItem
* objects, as returned by parseMultipartFormRequest(). If the
* specified parameter does not exist or if it has a white-space-only value,
* the supplied defaultValue will be returned. The values are
* assumed to be using the UTF-8 encoding.
*
* @param fileItemMap A Map of FileItem objects, mapped using their field
* name (Strings).
* @param paramName The name of the parameter.
* @param defaultValue The value that should be returned when the specified
* parameter did not have a value.
* @return The trimmed value, or null if the specified parameter
* does not have a value.
**/
public static String getParameter(Map fileItemMap, String paramName, String defaultValue) {
String result = defaultValue;
FileItem fileItem = (FileItem)fileItemMap.get(paramName);
if (fileItem != null) {
try {
result = fileItem.getString("UTF-8").trim();
}
catch (UnsupportedEncodingException e) {
// UTF-8 must be supported by all compliant JVM's,
// this exception should never be thrown.
throw new RuntimeException("UTF-8 character encoding not supported on this platform");
}
if (result.length() == 0) {
result = defaultValue;
}
}
return result;
}
/**
* Gets the binary value of a request parameter from a Map of FileItem
* objects, as returned by parseMultipartFormRequest().
*
* @param fileItemMap A Map of FileItem objects, mapped using their field
* name (Strings).
* @param paramName The name of the parameter.
* @return The binary value, or null if the specified parameter
* does not have a value.
**/
public static byte[] getBinaryParameter(Map fileItemMap, String paramName) {
byte[] result = null;
FileItem fileItem = (FileItem)fileItemMap.get(paramName);
if (fileItem != null) {
result = fileItem.get();
}
return result;
}
/**
* Gets the value of a request parameter as an InputStream from a Map of
* FileItem objects, as returned by parseMultipartFormRequest().
*
* @param fileItemMap A Map of FileItem objects, mapped using their field
* name (Strings).
* @param paramName The name of the parameter.
* @return The value, or null if the specified parameter does not
* have a value.
**/
public static InputStream getStreamParameter(Map fileItemMap, String paramName)
throws IOException
{
InputStream result = null;
FileItem fileItem = (FileItem)fileItemMap.get(paramName);
if (fileItem != null) {
result = fileItem.getInputStream();
}
return result;
}
/**
* Sets headers on the supplied response that prevent all kinds of
* browsers to cache it.
**/
public static void setNoCacheHeaders(HttpServletResponse response) {
//
// according to http://vancouver-webpages.com/META/FAQ.html
//
response.setHeader("Cache-Control", "must-revalidate");
response.setHeader("Cache-Control", "max-age=1");
response.setHeader("Cache-Control", "no-cache");
response.setIntHeader("Expires", 0);
response.setHeader("Pragma", "no-cache");
}
/**
* Checks whether the sender of the supplied request can handle
* gzip-encoded data.
*
* @param request A HTTP request
* @return true if the sender of the request can handle
* gzip-encoded data, false otherwise.
**/
public static boolean acceptsGZIPEncoding(HttpServletRequest request) {
boolean result = false;
String acceptEncoding = request.getHeader("accept-encoding");
if (acceptEncoding != null) {
// Microsoft Internet Explorer indicates that it accepts gzip
// encoding, but it fails to handle it properly (see issue
// [SES-28] in Sesame issue tracker).
boolean isInternetExplorer = false;
String userAgent = request.getHeader("user-agent");
if (userAgent != null) {
isInternetExplorer = userAgent.indexOf("MSIE") != -1;
}
result = isInternetExplorer == false &&
acceptEncoding.toLowerCase().indexOf("gzip") != -1;
}
return result;
}
/**
* Opens an gzip-encoded output stream for the specified response. This
* method also sets the required header(s) to indicate that the response
* is gzip-encoded.
**/
public static OutputStream openGZIPOutputStream(HttpServletResponse response)
throws IOException
{
response.setHeader("Content-Encoding", "gzip");
return new GZIPOutputStream(response.getOutputStream(), 4096);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy